1 店铺信息编辑DAO
package com.tzb.o2o.dao;
import com.tzb.o2o.entity.Shop;
public interface ShopDao {
Shop queryByShopId(long shopId);
int insertShop(Shop shop);
int updateShop(Shop shop);
}
<resultMap id="shopMap" type="com.tzb.o2o.entity.Shop">
<id column="shop_id" property="shopId" />
<result column="shop_name" property="shopName" />
<result column="shop_desc" property="shopDesc" />
<result column="shop_addr" property="shopAddr" />
<result column="phone" property="phone" />
<result column="priority" property="priority"/>
<result column="shop_img" property="shopImg" />
<result column="create_time" property="createTime" />
<result column="last_edit_time" property="lastEditTime" />
<result column="enable_status" property="enableStatus" />
<result column="advice" property="advice" />
<association column="owner_id" property="owner" javaType="PersonInfo">
<id column="user_id" property="userId" />
<result column="name" property="name" />
association>
<association column="area_id" property="area" javaType="Area">
<id column="area_id" property="areaId" />
<result column="area_name" property="areaName" />
association>
<association property="shopCategory" column="shop_category_id" javaType="ShopCategory">
<id column="shop_category_id" property="shopCategoryId" />
<result column="shop_category_name" property="shopCategoryName" />
association>
resultMap>
<select id="queryByShopId" resultMap="shopMap" parameterType="long">
SELECT
s.shop_id,
s.shop_name,
s.shop_desc,
s.shop_addr,
s.phone,
s.shop_img,
s.priority,
s.create_time,
s.last_edit_time,
s.enable_status,
s.advice,
a.area_id,
a.area_name,
sc.shop_category_id,
sc.shop_category_name
FROM
tb_shop s,
tb_area a,
tb_shop_category sc
WHERE
s.area_id = a.area_id
AND s.shop_category_id = sc.shop_category_id
AND
s.shop_id
= #{shopId}
select>
1.1 单元测试

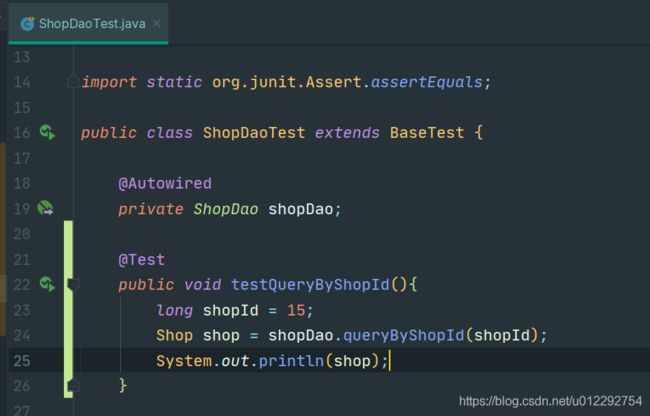
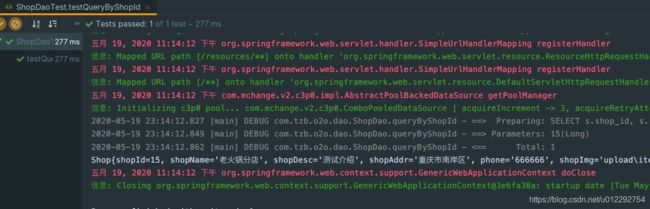
2 店铺编辑 Service
package com.tzb.o2o.service;
import com.tzb.o2o.dto.ShopExecution;
import com.tzb.o2o.entity.Shop;
import com.tzb.o2o.exceptions.ShopOperationException;
import java.io.InputStream;
public interface ShopService {
Shop getByShopId(long shopId);
ShopExecution modifyShop(Shop shop,InputStream shopImgInputStream,String fileName) throws ShopOperationException;
ShopExecution addShop(Shop shop, InputStream shopImgInputStream,String fileName);
}
public static void deleteFileOrPath(String storePath) {
File fileOrPath = new File(PathUtil.getImgBasePath() + storePath);
if(fileOrPath.exists()){
if (fileOrPath.isDirectory()){
File files[] = fileOrPath.listFiles();
for (File file : files) {
file.delete();
}
}
fileOrPath.delete();
}
}
@Override
public ShopExecution modifyShop(Shop shop, InputStream shopImgInputStream, String fileName) throws ShopOperationException {
if (shop == null || shop.getShopId() == null) {
return new ShopExecution(ShopStateEnum.NULL_SHOP);
} else {
try {
if (shopImgInputStream != null && fileName != null && !"".equals(fileName)) {
Shop tempShop = shopDao.queryByShopId(shop.getShopId());
if (tempShop.getShopImg() != null) {
ImageUtil.deleteFileOrPath(tempShop.getShopImg());
}
addShopImg(shop, shopImgInputStream, fileName);
}
shop.setLastEditTime(new Date());
int effectedNum = shopDao.updateShop(shop);
if (effectedNum <= 0) {
return new ShopExecution(ShopStateEnum.INNER_ERROR);
} else {
shop = shopDao.queryByShopId(shop.getShopId());
return new ShopExecution(ShopStateEnum.SUCCESS,shop);
}
} catch (Exception e) {
throw new ShopOperationException("modifyShop error:" + e.getMessage());
}
}
}
2.1 单元测试
@Test
public void testModifyShop() throws ShopOperationException, FileNotFoundException {
Shop shop = new Shop();
shop.setShopId(15L);
shop.setShopName("修改后的店铺名称");
File shopImg = new File("D://test2.jpg");
InputStream is = new FileInputStream(shopImg);
ShopExecution shopExecution = shopService.modifyShop(shop, is, "test2.jpg");
System.out.println("新图片地址:" + shopExecution.getShop().getShopImg());
}
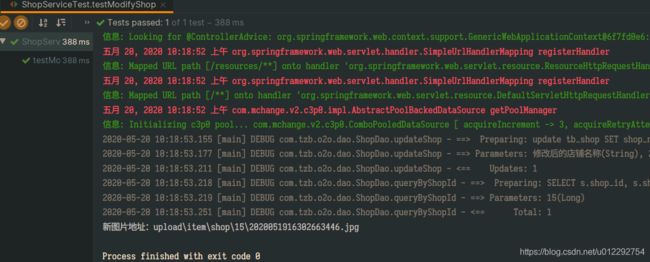

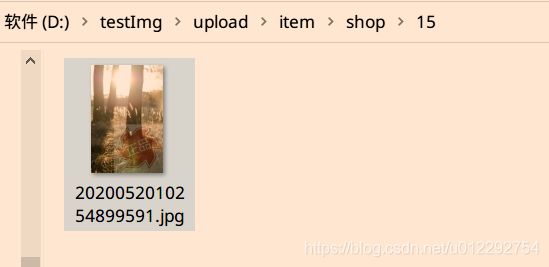