- 深入理解 Java 中的 ArrayList
^辞安
java开发语言idea
1.引言ArrayList是Java集合框架中最常用的数据结构之一。它基于动态数组实现,提供了快速的随机访问和高效的尾部插入操作。无论是初学者还是资深开发者,`ArrayList`都是日常开发中不可或缺的工具。本文将深入探讨`ArrayList`的实现原理、常见操作及其性能特点,并结合源码解析其内部机制。2.ArrayList的基本概念2.1什么是ArrayList?ArrayList是Java集
- 面试必问之缓存击穿、穿透、雪崩及常用解决方案
就要学Java
RedisSpringMysql缓存面试redis数据库java
缓存击穿、穿透、雪崩及解决方案Redis是一种高性能的键值型数据库,它可以用来实现缓存功能,提高应用的响应速度和承载能力。但是,使用Redis缓存也会遇到一些常见的问题,比如缓存击穿、缓存穿透、缓存雪崩。这些问题都会影响缓存的效率和稳定性,所以需要了解它们的原因和解决方案,保障Redis能够正常运行。击穿、穿透、雪崩的意思击穿、穿透、雪崩这三个词语很容易混淆,本文先对词语进行理解,再讲解技术击穿:
- C语言考研机试(自用)
海参的学习小屋
c语言开发语言考研学习方法visualstudio
一、注意事项%c是一个格式化转换说明符,用于读取或输出一个字符;%s是字符串%f:表示输出一个浮点数;%lf:表示将输入的值解释为双精度浮点数。%.2f:表示输出一个浮点数并保留两位小数,对应的变量是y。A=a-32,A的ASCII是65,a是97scanf_s("%c",&a,1);#includesqrt(x);//求平方根abs(x);//绝对值pow(x,y);//x的y次方最大公约数。欧
- 关于idea中新建springboot项目Java版本不能选择11和8的解决办法
aniceperson999
intellij-ideajavaide
原因:spring2.X版本在2023年11月24日停止维护了,因此创建spring项目时不再有2.X版本的选项,只能从3.1.X版本开始选择而Spring3.X版本不支持JDK8,JDK11,最低支持JDK17,因此JDK11也无法选择了当然,停止维护只代表我们无法用idea主动创建spring2.X版本的项目了,不代表我们无法使用,该使用依然能使用,丝毫不受影响目前阿里云还是支持创建Sprin
- python网络爬虫——爬取新发地农产品数据
张謹礧
python网络爬虫python爬虫开发语言
这段代码是一个爬取新发地蔬菜价格信息的程序,它使用了多线程来加快数据获取和解析的速度。具体的步骤如下:导入所需的库:json、requests、threading和pandas。初始化一些变量,包括页数、商品总列表以及存放json数据的列表。定义了一个函数url_parse(),用于发送请求并解析网页数据。函数使用requests.post()方法发送POST请求,获取商品信息,并将其保存到jso
- python pip怎么升级_使用Python pip怎么升级pip
weixin_39608118
pythonpip怎么升级
Pip是一个Python的包管理工具,实际上它也可以被看待为是一个包,Pip相当于Linux上的yum,对python的开发者来说相当方便。我们再也无需去焦头烂额的寻找whl包,直接通过pip就可以在线安装(前提是有网络+pip版本合适的情况下)可见pip的版本更新是相当重要的,今天小编就来教大家怎么升级pip方法/步骤我们首先来看看pip的版本。pipshowpip可以看到,小编这里的pip版本
- 中科院C语言应聘机试编程题6,中科院计算所保研笔试+机试+面试经验分享
力气气
中科院C语言应聘机试编程题6
计算所JDL(先进人机交互)实验室9月10号开始联系计算所导师,12号收到导师的回复,大致意思是老师让我提供三位本校推荐老师的联系方式,又问了是否有读博的打算,让我准备到计算所JDL面试,16号收到了他们的正式通知。老实说我这次的复试基本属于裸面,时间很仓促,后来才知道很多同学在暑假之前就开始联系导师了,提前见了导师,咨询清楚了复试内容,暑假有针对性性地复习了一遍。这样的话录取的可能性就很大了,所
- python数据分析之爬虫基础:爬虫介绍以及urllib详解
web13765607643
python数据分析爬虫
前言在数据分析中,爬虫有着很大作用,可以自动爬取网页中提取的大量的数据,比如从电商网站手机商品信息,为市场分析提供数据基础。也可以补充数据集、检测动态变化等一系列作用。可以说在数据分析中有着相当大的作用!页面结构介绍这里主要介绍HTML的一些简单结构,需要一点前端的知识,可以根据情况直接跳过。Title姓名年龄性别张三18男铁锅炖大鹅小鸡炖蘑菇锅包肉奖励自己睡觉起床读书学习爬虫相关概念1、爬虫的概
- 使用Idea创建springboot项目
奔跑吧邓邓子
SpringBoot深入浅出常见问题解答(FAQ)高效运维javaidea
提示:“奔跑吧邓邓子”的高效运维专栏聚焦于各类运维场景中的实际操作与问题解决。内容涵盖服务器硬件(如IBMSystem3650M5)、云服务平台(如腾讯云、华为云)、服务器软件(如Nginx、Apache、GitLab、Redis、Elasticsearch、Kubernetes、Docker等)、开发工具(如Git、HBuilder)以及网络安全(如挖矿病毒排查、SSL证书配置)等多个方面。无论
- 人事管理系统设计与实现
AI天才研究院
DeepSeekR1&大数据AI人工智能大模型AI大模型企业级应用开发实战计算计算科学神经计算深度学习神经网络大数据人工智能大型语言模型AIAGILLMJavaPython架构设计AgentRPA
人事管理系统设计与实现1.背景介绍在现代企业管理中,人力资源管理是一个非常重要的环节。传统的人事管理方式效率低下,无法满足企业快速发展的需求。因此,开发一套功能完善、高效实用的人事管理系统就显得尤为重要。本文将详细介绍一个基于Web的人事管理系统的设计与实现过程,该系统采用B/S架构,后端使用Java语言,前端采用Vue.js框架。系统主要包括员工信息管理、招聘管理、考勤管理、薪酬管理、绩效考核等
- MeanShift聚类分割算法
点云学习
c++pcl点云处理聚类算法pcl点云处理PCL3D视觉
目录1MeanShift算法的数学原理1.密度估计2.均值向量计算3.位置更新4.收敛条件2MeanShift算法的详细步骤1初始化2迭代过程3聚类3示例代码1MeanShift算法的数学原理MeanShift算法的核心思想是通过在高维空间中计算密度梯度并进行移动,找到数据点的密度峰值,从而实现聚类。下面详细介绍该算法的数学原理和每一步的推理公式。1.密度估计MeanShift算法通过核密度估计(
- [特殊字符]【CVPR2024新突破】Logit标准化:知识蒸馏中的自适应温度革命[特殊字符]
☞黑心萝卜三条杠☜
论文人工智能论文阅读
文章信息题目:LogitStandardizationinKnowledgeDistillation论文地址:paper代码地址:code年份:2024年发表于CVPR文章主题文章的核心目标是改进知识蒸馏(KD)中的一个关键问题:传统KD方法假设教师和学生模型共享一个全局温度参数(temperature),这导致学生模型需要精确匹配教师模型的logit范围和方差。这种假设不仅限制了学生模型的性能,
- 【学习】电脑上有多个GPU,命令行指定GPU进行训练。
超好的小白
学习人工智能深度学习
使用如下指令可以指定使用的GPU。CUDA_VISIBLE_DEVICES=1假设要使用第二个GPU进行训练。CUDA_VISIBLE_DEVICES=1pythontrain.py
- 深度学习开源数据集大全:从入门到前沿
念九_ysl
AI人工智能
在深度学习中,数据是模型训练的基石。本文整理了当前最常用且高质量的开源数据集,涵盖图像、视频、自然语言处理(NLP)、语音与音频等方向,帮助研究者和开发者快速定位所需资源。一、图像类数据集1.MNIST简介:手写数字识别领域的“HelloWorld”,包含6万张训练图像和1万张测试图像,尺寸为28×28的灰度图。特点:适合入门级图像分类任务,支持快速验证算法原型28。下载地址:MNIST官网2.I
- React + TypeScript 实现 SQL 脚本生成全栈实践
i建模
数据建模数据管理前端开发数学建模
React+TypeScript实现数据模型驱动SQL脚本生成全栈实践引言:数据模型与SQL的桥梁革命在现代化全栈开发中,数据模型与数据库的精准映射已成为提升开发效率的关键。传统手动编写SQL脚本的方式存在模式漂移风险高(SchemaDrift)和维护成本大两大痛点。本文将结合React+TypeScript技术栈,解析如何构建智能化的SQL脚本生成系统,并给出2025年最新企业级解决方案。一、技
- 每日一题之屏蔽信号
Ace'
算法c++
问题描述在与三体文明的对抗中,人类联邦探测到了两个重要的信号源,分别用非负整数aa和bb来表示。为了抵御三体舰队的入侵,科学家们制定出一项关键策略——屏蔽信号,目标是要让aa、bb这两个信号源其中之一的数值归零。在实施屏蔽操作时,有着一套既定规则:每次操作,科学家们需要先对比两个信号源的数值大小,然后用较大的那个数减去较小的数,得出差值之后,再把原本较大的那个数替换成这个差值。就这样反复操作,一轮
- 基于Python豆瓣电影评论的数据处理与分析
AI智能涌现深度研究
DeepSeekR1&大数据AI人工智能Python入门实战计算科学神经计算深度学习神经网络大数据人工智能大型语言模型AIAGILLMJavaPython架构设计AgentRPA
基于Python豆瓣电影评论的数据处理与分析作者:禅与计算机程序设计艺术1.背景介绍1.1豆瓣电影评论数据的价值1.1.1反映观众观影偏好1.1.2影响电影市场走向1.1.3为推荐系统提供数据支持1.2Python在数据处理与分析中的优势1.2.1丰富的数据处理库1.2.2强大的数据分析和可视化能力1.2.3简洁高效的语法1.3本文的研究目的和意义1.3.1探索豆瓣电影评论数据的特点1.3.2实践
- VUE实现日历记录事件
yu_zheng5163
vue.jsjavascriptecmascript
el-calendarHTML代码段可以根据自己的需要显示内容,样式-1?'lar-is-selected':'lar-no-selected'"@click="holidayUpdate(data,date)">班-1&&queryDate.indexOf(data.day)!=-1"style="color:#f73131">休 -1?'color:#F73131;text-alig
- 用idea创建低版本springboot2.X的项目
詹皇wm
问题总结intellij-ideajava
文章目录我的环境:创建项目:进入创建项目页面:进入Springboot版本选择和添加依赖页面进入项目打开pom.xml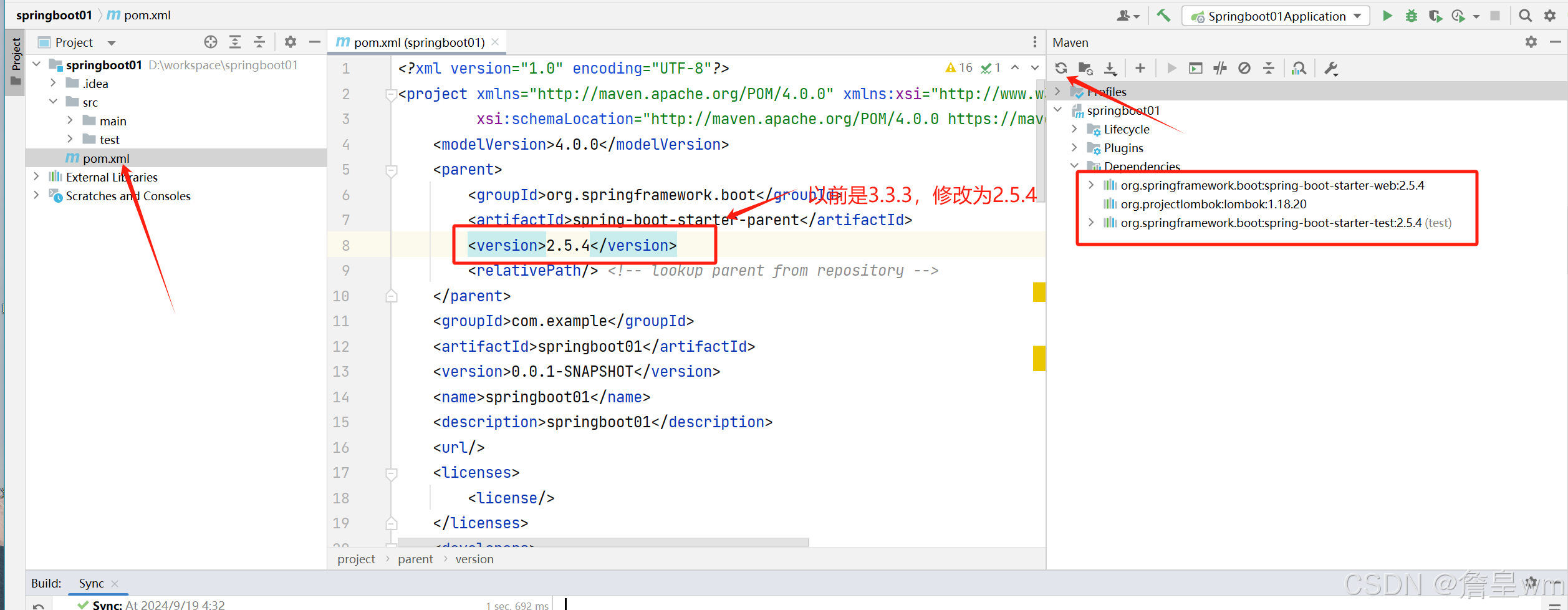*补充*_本文章主要是对于现在idea配置大部分都是自带SpringBoot3.X,怎么创建低版本springboo
- 【Golang】Go语言Web开发之模板渲染
景天科技苑
Go语言开发零基础到高阶实战golang前端开发语言Go语言模板渲染模板渲染golang模板渲染
✨✨欢迎大家来到景天科技苑✨✨养成好习惯,先赞后看哦~作者简介:景天科技苑《头衔》:大厂架构师,华为云开发者社区专家博主,阿里云开发者社区专家博主,CSDN全栈领域优质创作者,掘金优秀博主,51CTO博客专家等。《博客》:Python全栈,Golang开发,PyQt5和Tkinter桌面开发,小程序开发,人工智能,js逆向,App逆向,网络系统安全,数据分析,Django,fastapi,flas
- 【第八节】C++设计模式(结构型模式)-Decorator(装饰器)模式
攻城狮7号
c++版本设计模式c++设计模式装饰器模式
目录一、问题引出二、模式选择三、代码实现四、总结讨论一、问题引出装饰器模式:动态扩展对象功能的设计模式在面向对象(OO)设计与开发中,我们常面临为已有类添加新职责的需求。传统方法是通过继承创建子类来实现功能扩展,但这种方式容易导致继承层次过深,显著增加系统复杂度。装饰器模式(DecoratorPattern)应运而生,其通过组合替代继承的机制,为功能扩展提供了更灵活的解决方案,从而避免了继承层次过
- Springboot 整合 Java DL4J 实现企业门禁人脸识别系统
伏羲栈
人工智能深度学习JavaDL4J-深度学习实战springbootjavaDeeplearning4jdeeplearning人工智能深度学习spring
博主简介:历代文学网(PC端可以访问:https://literature.sinhy.com/#/literature?__c=1000,移动端可微信小程序搜索“历代文学”)总架构师,15年工作经验,精通Java编程,高并发设计,Springboot和微服务,熟悉Linux,ESXI虚拟化以及云原生Docker和K8s,热衷于探索科技的边界,并将理论知识转化为实际应用。保持对新技术的好奇心,乐于
- Redis---LRU原理与算法实现
lh_freak
redis算法数据库
文章目录LRU概念理解LRU原理基于HashMap和双向链表实现LRURedis中的LRU的实现LRU时钟淘汰策略近似LRU的实现LRU算法的优化RedisLRU的核心代码逻辑RedisLRU的核心代码逻辑RedisLRU的配置参数RedisLRU的优缺点RedisLRU的优缺点LRU概念理解LRU(LeastRecentlyUsed)最近最少使用算法,是一种常用的页面置换算法,广泛应用于操作系统
- HTML---css选择器
lh_freak
htmlcss前端
CSS指层叠样式表,是一种用来为结构化文档(如HTML文档或XML应用)添加样式(字体、间距和颜色等)的计算机语言,CSS文件扩展名为.css。使用CSS我们可以大大提升网页开发的工作效率!插入样式表的方法有三种:外部样式(Externalstylesheet)内部样式(Internalstylesheet)内联样式(Inlinestyle)内联样式(行内样式)hello样式直接写入到本元素标签中
- 或许我们都被分库分表约束了思维
京东云开发者
京东云
作者:张俊杰概述这篇文章没什么太多的干货,纯纯是一篇讨论和思考帖。从业数据库领域三年有余了,从分库分表中间件到数据库团队内核学到了很多东西。也接触了很多项目,包括TiDB、Vitess、Polardb、StarDB等等。国内的项目好像很多都聚焦于分库分表的概念,包括很多的数据库团队都在尝试这个概念的落地和沉溺于性能的跑分。最近我在预览MySQL官方,看到了Partitioning的概念,而且占据了
- spark为什么比mapreduce快?
京东云开发者
sparkmapreduce大数据
作者:京东零售吴化斌spark为什么比mapreduce快?首先澄清几个误区:1:两者都是基于内存计算的,任何计算框架都肯定是基于内存的,所以网上说的spark是基于内存计算所以快,显然是错误的2;DAG计算模型减少的是磁盘I/O次数(相比于mapreduce计算模型而言),而不是shuffle次数,因为shuffle是根据数据重组的次数而定,所以shuffle次数不能减少所以总结spark比ma
- 程序员未来的出路:行业趋势与职业发展分析
guzhoumingyue
AIpython
随着技术的发展和行业需求的变化,程序员的职业出路也在不断演变。以下是程序员未来可能的职业发展方向及具体建议:一、技术深耕路线AI与机器学习专家趋势:AI技术在各行业的应用日益广泛,从自动驾驶到智能客服,需求持续增长。技能要求:Python、TensorFlow、PyTorch、数据挖掘、算法优化。发展路径:从机器学习工程师做起,积累项目经验。深入研究深度学习、强化学习等前沿技术。成为AI架构师或数
- 在麻将 AI 的迷宫中,我用 Python 函数组合探寻最优解:精髓与穷举
fxrz12
AI人工智能python开发语言
我,一个对人工智能充满热情的程序员,带着对麻将策略的浓厚兴趣,踏上了开发AI麻将服务器的征程。这不仅仅是一次技术挑战,更是一次对思维方式和问题解决能力的深度探索。麻将,这个看似简单的游戏,实则蕴含着无穷的策略和变化。AI需要在瞬息万变的牌局中,做出最优的决策,这需要它:洞察牌局:精准分析手牌,评估牌型的潜在价值。预判风险:计算打出某张牌可能带来的风险。布局未来:预测后续牌局的走向,制定长远策略。为
- 磁力链接那些事:开源工具大盘点
fxrz12
开源软件github
什么是磁力链接?磁力链接(MagnetURI)是一种用于表示文件或文件夹的超链接文本,通常用于P2P网络中的文件共享。与传统的HTTP链接不同,磁力链接并不直接指向文件存储位置,而是包含了文件的哈希值、跟踪器信息等元数据。这种方式使得文件分享更加高效、安全,也更难以被审查。为什么选择开源磁力工具?自由定制:开源工具的代码是公开的,用户可以根据自己的需求进行修改和扩展。安全可靠:开源社区的监督和审查
- 谈一谈无服务架构降本增效
fxrz12
架构运维云计算serverless无服务器
在当今数字化转型的浪潮中,企业不断寻求创新的方法来优化IT基础设施,降低运营成本并提升业务效率。无服务架构(ServerlessArchitecture)作为一种新兴的计算模式,正在成为众多企业的首选解决方案。本文将探讨无服务架构如何帮助企业实现降本增效,并通过图表对比无服务架构和常规架构。什么是无服务架构?无服务架构是一种云计算执行模型,开发者可以部署代码而无需管理服务器。云服务提供商(如AWS
- java的(PO,VO,TO,BO,DAO,POJO)
Cb123456
VOTOBOPOJODAO
转:
http://www.cnblogs.com/yxnchinahlj/archive/2012/02/24/2366110.html
-------------------------------------------------------------------
O/R Mapping 是 Object Relational Mapping(对象关系映
- spring ioc原理(看完后大家可以自己写一个spring)
aijuans
spring
最近,买了本Spring入门书:spring In Action 。大致浏览了下感觉还不错。就是入门了点。Manning的书还是不错的,我虽然不像哪些只看Manning书的人那样专注于Manning,但怀着崇敬 的心情和激情通览了一遍。又一次接受了IOC 、DI、AOP等Spring核心概念。 先就IOC和DI谈一点我的看法。IO
- MyEclipse 2014中Customize Persperctive设置无效的解决方法
Kai_Ge
MyEclipse2014
高高兴兴下载个MyEclipse2014,发现工具条上多了个手机开发的按钮,心生不爽就想弄掉他!
结果发现Customize Persperctive失效!!
有说更新下就好了,可是国内Myeclipse访问不了,何谈更新...
so~这里提供了更新后的一下jar包,给大家使用!
1、将9个jar复制到myeclipse安装目录\plugins中
2、删除和这9个jar同包名但是版本号较
- SpringMvc上传
120153216
springMVC
@RequestMapping(value = WebUrlConstant.UPLOADFILE)
@ResponseBody
public Map<String, Object> uploadFile(HttpServletRequest request,HttpServletResponse httpresponse) {
try {
//
- Javascript----HTML DOM 事件
何必如此
JavaScripthtmlWeb
HTML DOM 事件允许Javascript在HTML文档元素中注册不同事件处理程序。
事件通常与函数结合使用,函数不会在事件发生前被执行!
注:DOM: 指明使用的 DOM 属性级别。
1.鼠标事件
属性  
- 动态绑定和删除onclick事件
357029540
JavaScriptjquery
因为对JQUERY和JS的动态绑定事件的不熟悉,今天花了好久的时间才把动态绑定和删除onclick事件搞定!现在分享下我的过程。
在我的查询页面,我将我的onclick事件绑定到了tr标签上同时传入当前行(this值)参数,这样可以在点击行上的任意地方时可以选中checkbox,但是在我的某一列上也有一个onclick事件是用于下载附件的,当
- HttpClient|HttpClient请求详解
7454103
apache应用服务器网络协议网络应用Security
HttpClient 是 Apache Jakarta Common 下的子项目,可以用来提供高效的、最新的、功能丰富的支持 HTTP 协议的客户端编程工具包,并且它支持 HTTP 协议最新的版本和建议。本文首先介绍 HTTPClient,然后根据作者实际工作经验给出了一些常见问题的解决方法。HTTP 协议可能是现在 Internet 上使用得最多、最重要的协议了,越来越多的 Java 应用程序需
- 递归 逐层统计树形结构数据
darkranger
数据结构
将集合递归获取树形结构:
/**
*
* 递归获取数据
* @param alist:所有分类
* @param subjname:对应统计的项目名称
* @param pk:对应项目主键
* @param reportList: 最后统计的结果集
* @param count:项目级别
*/
public void getReportVO(Arr
- 访问WEB-INF下使用frameset标签页面出错的原因
aijuans
struts2
<frameset rows="61,*,24" cols="*" framespacing="0" frameborder="no" border="0">
- MAVEN常用命令
avords
Maven库:
http://repo2.maven.org/maven2/
Maven依赖查询:
http://mvnrepository.com/
Maven常用命令: 1. 创建Maven的普通java项目: mvn archetype:create -DgroupId=packageName 
- PHP如果自带一个小型的web服务器就好了
houxinyou
apache应用服务器WebPHP脚本
最近单位用PHP做网站,感觉PHP挺好的,不过有一些地方不太习惯,比如,环境搭建。PHP本身就是一个网站后台脚本,但用PHP做程序时还要下载apache,配置起来也不太很方便,虽然有好多配置好的apache+php+mysq的环境,但用起来总是心里不太舒服,因为我要的只是一个开发环境,如果是真实的运行环境,下个apahe也无所谓,但只是一个开发环境,总有一种杀鸡用牛刀的感觉。如果php自己的程序中
- NoSQL数据库之Redis数据库管理(list类型)
bijian1013
redis数据库NoSQL
3.list类型及操作
List是一个链表结构,主要功能是push、pop、获取一个范围的所有值等等,操作key理解为链表的名字。Redis的list类型其实就是一个每个子元素都是string类型的双向链表。我们可以通过push、pop操作从链表的头部或者尾部添加删除元素,这样list既可以作为栈,又可以作为队列。
&nbs
- 谁在用Hadoop?
bingyingao
hadoop数据挖掘公司应用场景
Hadoop技术的应用已经十分广泛了,而我是最近才开始对它有所了解,它在大数据领域的出色表现也让我产生了兴趣。浏览了他的官网,其中有一个页面专门介绍目前世界上有哪些公司在用Hadoop,这些公司涵盖各行各业,不乏一些大公司如alibaba,ebay,amazon,google,facebook,adobe等,主要用于日志分析、数据挖掘、机器学习、构建索引、业务报表等场景,这更加激发了学习它的热情。
- 【Spark七十六】Spark计算结果存到MySQL
bit1129
mysql
package spark.examples.db
import java.sql.{PreparedStatement, Connection, DriverManager}
import com.mysql.jdbc.Driver
import org.apache.spark.{SparkContext, SparkConf}
object SparkMySQLInteg
- Scala: JVM上的函数编程
bookjovi
scalaerlanghaskell
说Scala是JVM上的函数编程一点也不为过,Scala把面向对象和函数型编程这两种主流编程范式结合了起来,对于熟悉各种编程范式的人而言Scala并没有带来太多革新的编程思想,scala主要的有点在于Java庞大的package优势,这样也就弥补了JVM平台上函数型编程的缺失,MS家.net上已经有了F#,JVM怎么能不跟上呢?
对本人而言
- jar打成exe
bro_feng
java jar exe
今天要把jar包打成exe,jsmooth和exe4j都用了。
遇见几个问题。记录一下。
两个软件都很好使,网上都有图片教程,都挺不错。
首先肯定是要用自己的jre的,不然不能通用,其次别忘了把需要的lib放到classPath中。
困扰我很久的一个问题是,我自己打包成功后,在一个同事的没有装jdk的电脑上运行,就是不行,报错jvm.dll为无效的windows映像,如截图
最后发现
- 读《研磨设计模式》-代码笔记-策略模式-Strategy
bylijinnan
java设计模式
声明: 本文只为方便我个人查阅和理解,详细的分析以及源代码请移步 原作者的博客http://chjavach.iteye.com/
/*
策略模式定义了一系列的算法,并将每一个算法封装起来,而且使它们还可以相互替换。策略模式让算法独立于使用它的客户而独立变化
简单理解:
1、将不同的策略提炼出一个共同接口。这是容易的,因为不同的策略,只是算法不同,需要传递的参数
- cmd命令值cvfM命令
chenyu19891124
cmd
cmd命令还真是强大啊。今天发现jar -cvfM aa.rar @aaalist 就这行命令可以根据aaalist取出相应的文件
例如:
在d:\workspace\prpall\test.java 有这样一个文件,现在想要将这个文件打成一个包。运行如下命令即可比如在d:\wor
- OpenJWeb(1.8) Java Web应用快速开发平台
comsci
java框架Web项目管理企业应用
OpenJWeb(1.8) Java Web应用快速开发平台的作者是我们技术联盟的成员,他最近推出了新版本的快速应用开发平台 OpenJWeb(1.8),我帮他做做宣传
OpenJWeb快速开发平台以快速开发为核心,整合先进的java 开源框架,本着自主开发+应用集成相结合的原则,旨在为政府、企事业单位、软件公司等平台用户提供一个架构透
- Python 报错:IndentationError: unexpected indent
daizj
pythontab空格缩进
IndentationError: unexpected indent 是缩进的问题,也有可能是tab和空格混用啦
Python开发者有意让违反了缩进规则的程序不能通过编译,以此来强制程序员养成良好的编程习惯。并且在Python语言里,缩进而非花括号或者某种关键字,被用于表示语句块的开始和退出。增加缩进表示语句块的开
- HttpClient 超时设置
dongwei_6688
httpclient
HttpClient中的超时设置包含两个部分:
1. 建立连接超时,是指在httpclient客户端和服务器端建立连接过程中允许的最大等待时间
2. 读取数据超时,是指在建立连接后,等待读取服务器端的响应数据时允许的最大等待时间
在HttpClient 4.x中如下设置:
HttpClient httpclient = new DefaultHttpC
- 小鱼与波浪
dcj3sjt126com
一条小鱼游出水面看蓝天,偶然间遇到了波浪。 小鱼便与波浪在海面上游戏,随着波浪上下起伏、汹涌前进。 小鱼在波浪里兴奋得大叫:“你每天都过着这么刺激的生活吗?简直太棒了。” 波浪说:“岂只每天过这样的生活,几乎每一刻都这么刺激!还有更刺激的,要有潮汐变化,或者狂风暴雨,那才是兴奋得心脏都会跳出来。” 小鱼说:“真希望我也能变成一个波浪,每天随着风雨、潮汐流动,不知道有多么好!” 很快,小鱼
- Error Code: 1175 You are using safe update mode and you tried to update a table
dcj3sjt126com
mysql
快速高效用:SET SQL_SAFE_UPDATES = 0;下面的就不要看了!
今日用MySQL Workbench进行数据库的管理更新时,执行一个更新的语句碰到以下错误提示:
Error Code: 1175
You are using safe update mode and you tried to update a table without a WHERE that
- 枚举类型详细介绍及方法定义
gaomysion
enumjavaee
转发
http://developer.51cto.com/art/201107/275031.htm
枚举其实就是一种类型,跟int, char 这种差不多,就是定义变量时限制输入的,你只能够赋enum里面规定的值。建议大家可以看看,这两篇文章,《java枚举类型入门》和《C++的中的结构体和枚举》,供大家参考。
枚举类型是JDK5.0的新特征。Sun引进了一个全新的关键字enum
- Merge Sorted Array
hcx2013
array
Given two sorted integer arrays nums1 and nums2, merge nums2 into nums1 as one sorted array.
Note:You may assume that nums1 has enough space (size that is
- Expression Language 3.0新特性
jinnianshilongnian
el 3.0
Expression Language 3.0表达式语言规范最终版从2013-4-29发布到现在已经非常久的时间了;目前如Tomcat 8、Jetty 9、GlasshFish 4已经支持EL 3.0。新特性包括:如字符串拼接操作符、赋值、分号操作符、对象方法调用、Lambda表达式、静态字段/方法调用、构造器调用、Java8集合操作。目前Glassfish 4/Jetty实现最好,对大多数新特性
- 超越算法来看待个性化推荐
liyonghui160com
超越算法来看待个性化推荐
一提到个性化推荐,大家一般会想到协同过滤、文本相似等推荐算法,或是更高阶的模型推荐算法,百度的张栋说过,推荐40%取决于UI、30%取决于数据、20%取决于背景知识,虽然本人不是很认同这种比例,但推荐系统中,推荐算法起的作用起的作用是非常有限的。
就像任何
- 写给Javascript初学者的小小建议
pda158
JavaScript
一般初学JavaScript的时候最头痛的就是浏览器兼容问题。在Firefox下面好好的代码放到IE就不能显示了,又或者是在IE能正常显示的代码在firefox又报错了。 如果你正初学JavaScript并有着一样的处境的话建议你:初学JavaScript的时候无视DOM和BOM的兼容性,将更多的时间花在 了解语言本身(ECMAScript)。只在特定浏览器编写代码(Chrome/Fi
- Java 枚举
ShihLei
javaenum枚举
注:文章内容大量借鉴使用网上的资料,可惜没有记录参考地址,只能再传对作者说声抱歉并表示感谢!
一 基础 1)语法
枚举类型只能有私有构造器(这样做可以保证客户代码没有办法新建一个enum的实例)
枚举实例必须最先定义
2)特性
&nb
- Java SE 6 HotSpot虚拟机的垃圾回收机制
uuhorse
javaHotSpotGC垃圾回收VM
官方资料,关于Java SE 6 HotSpot虚拟机的garbage Collection,非常全,英文。
http://www.oracle.com/technetwork/java/javase/gc-tuning-6-140523.html
Java SE 6 HotSpot[tm] Virtual Machine Garbage Collection Tuning
&