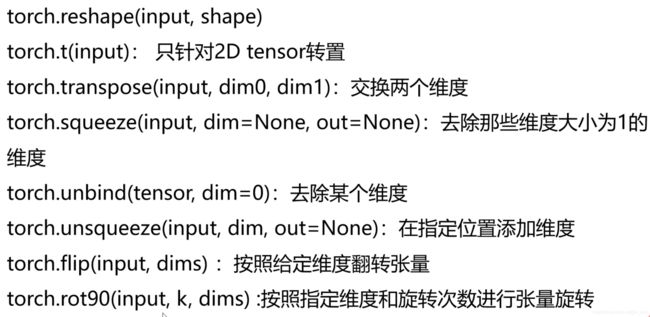
reshape transpose
import torch
a = torch.rand(2, 3)
print('a\n', a)
out = torch.reshape(a, (3, 2))
print('out\n', out)
print(torch.t(out))
print(torch.transpose(out, 0, 1))
输出结果:
a
tensor([[0.1375, 0.3036, 0.2777],
[0.7585, 0.9363, 0.4981]])
out
tensor([[0.1375, 0.3036],
[0.2777, 0.7585],
[0.9363, 0.4981]])
tensor([[0.1375, 0.2777, 0.9363],
[0.3036, 0.7585, 0.4981]])
tensor([[0.1375, 0.2777, 0.9363],
[0.3036, 0.7585, 0.4981]])
unbind
import torch
a = torch.rand(1, 2, 3)
print('a\n', a)
out = torch.unbind(a, dim=1)
print('out1\n', out)
print('out1.shape\n', out[0].shape)
out = torch.unbind(a, dim=2)
print('out\n', out)
print('out.shape\n', out[0].shape)
输出结果:
a
tensor([[[0.3706, 0.0505, 0.0693],
[0.2060, 0.7625, 0.0705]]])
out1
(tensor([[0.3706, 0.0505, 0.0693]]), tensor([[0.2060, 0.7625, 0.0705]]))
out1.shape
torch.Size([1, 3])
out
(tensor([[0.3706, 0.2060]]), tensor([[0.0505, 0.7625]]), tensor([[0.0693, 0.0705]]))
out.shape
torch.Size([1, 2])
flip
a = torch.rand(1, 2, 3)
print('a\n', a)
print('dims=0\n', torch.flip(a, dims=[0]))
print('dims=1\n', torch.flip(a, dims=[1]))
print('dims=1 shape\n', torch.flip(a, dims=[1]).shape)
print('a\n', a)
print('dims=2\n', torch.flip(a, dims=[2]))
print('dims=2 shape\n', torch.flip(a, dims=[2]).shape)
print('a\n', a)
print('dims=1, 2\n', torch.flip(a, dims=[1, 2]))
print('dims=1, 2 shape\n', torch.flip(a, dims=[1, 2]).shape)
out = torch.flip(a, dims=[1])
out2 = torch.flip(out, dims=[2])
print('out2\n', out2)
输出结果:
a
tensor([[[0.7758, 0.6811, 0.1429],
[0.2851, 0.7585, 0.1430]]])
dims=0
tensor([[[0.7758, 0.6811, 0.1429],
[0.2851, 0.7585, 0.1430]]])
dims=1
tensor([[[0.2851, 0.7585, 0.1430],
[0.7758, 0.6811, 0.1429]]])
dims=1 shape
torch.Size([1, 2, 3])
a
tensor([[[0.7758, 0.6811, 0.1429],
[0.2851, 0.7585, 0.1430]]])
dims=2
tensor([[[0.1429, 0.6811, 0.7758],
[0.1430, 0.7585, 0.2851]]])
dims=2 shape
torch.Size([1, 2, 3])
a
tensor([[[0.7758, 0.6811, 0.1429],
[0.2851, 0.7585, 0.1430]]])
dims=1, 2
tensor([[[0.1430, 0.7585, 0.2851],
[0.1429, 0.6811, 0.7758]]])
dims=1, 2 shape
torch.Size([1, 2, 3])
out2
tensor([[[0.1430, 0.7585, 0.2851],
[0.1429, 0.6811, 0.7758]]])
rot90
import torch
a = torch.rand(1, 2, 3)
print('a\n', a)
out = torch.rot90(a)
print('out90\n', out)
print('out.shape\n', out.shape)
out = torch.rot90(a, 2)
print('out180\n', out)
print('out.shape\n', out.shape)
out = torch.rot90(a, 4)
print('out360\n', out)
print('out.shape\n', out.shape)
out = torch.rot90(a, -1, [0, 2])
print('out-90\n', out)
print('out.sha pe\n', out.shape)
输出结果:
a
tensor([[[0.8526, 0.7337, 0.5586],
[0.7688, 0.5023, 0.9327]]])
out90
tensor([[[0.7688, 0.5023, 0.9327]],
[[0.8526, 0.7337, 0.5586]]])
out.shape
torch.Size([2, 1, 3])
out180
tensor([[[0.7688, 0.5023, 0.9327],
[0.8526, 0.7337, 0.5586]]])
out.shape
torch.Size([1, 2, 3])
out360
tensor([[[0.8526, 0.7337, 0.5586],
[0.7688, 0.5023, 0.9327]]])
out.shape
torch.Size([1, 2, 3])
out-90
tensor([[[0.8526],
[0.7688]],
[[0.7337],
[0.5023]],
[[0.5586],
[0.9327]]])
out.sha pe
torch.Size([3, 2, 1])