Elasticsearch ( ES ) 分布式全文搜索引擎介绍、安装与使用;SpringBoot 整合 ES 代码示例
+ Elasticsearch ( ES ) 分布式全文搜索引擎介绍、安装与使用
- 以windows环境为例:
- 下载 ES :
https://artifacts.elastic.co/downloads/elasticsearch/elasticsearch-7.16.2-windows-x86_64.zip
- 解压完成后,执行 bin 下 elasticsearch.bat 启动 ES 服务:
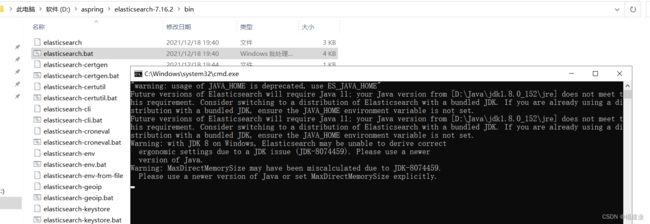
- 验证是否启动成功:访问 localhost:9200,返回如下图所示 JSON 信息,则表示启动成功:
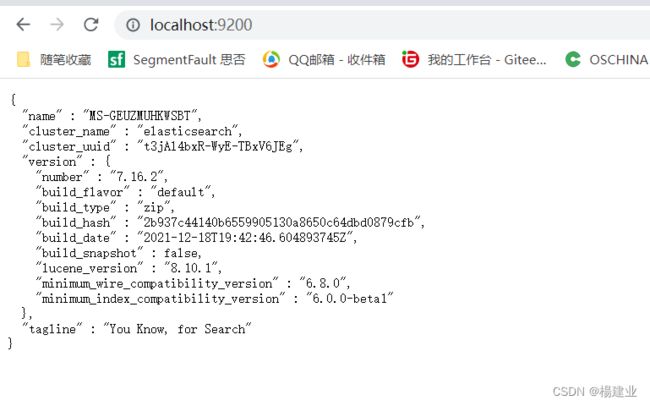
- 下载 IK 分词器:
- https://github.com/medcl/elasticsearch-analysis-ik/releases/download/v7.16.2/elasticsearch-analysis-ik-7.16.2.zip
- 将压缩包中的文件解压到下图所示目录后,重启 ES 服务:
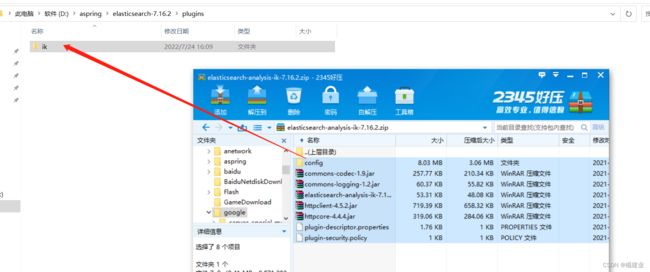
- 通过 Postman 创建索引(PUT请求):
{
"mappings":{
"properties":{
"id":{
"type":"keyword"
},
"name":{
"type":"text",
"analyzer":"ik_max_word",
"copy_to":"all"
},
"description":{
"type":"text",
"analyzer":"ik_max_word",
"copy_to":"all"
},
"price":{
"type":"keyword"
},
"all":{
"type":"text",
"analyzer":"ik_max_word"
}
}
}
}
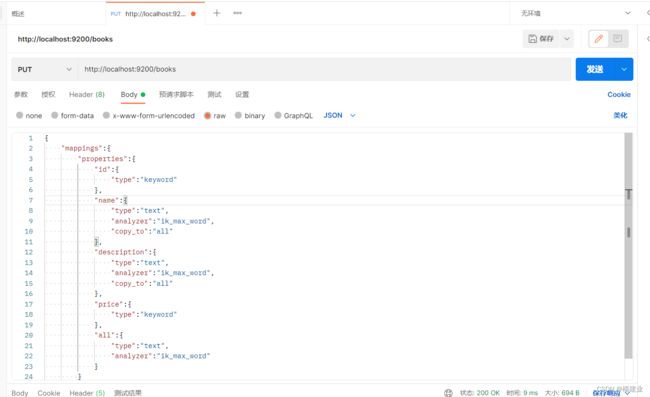
- 通过 Postman 查询索引(GET请求):
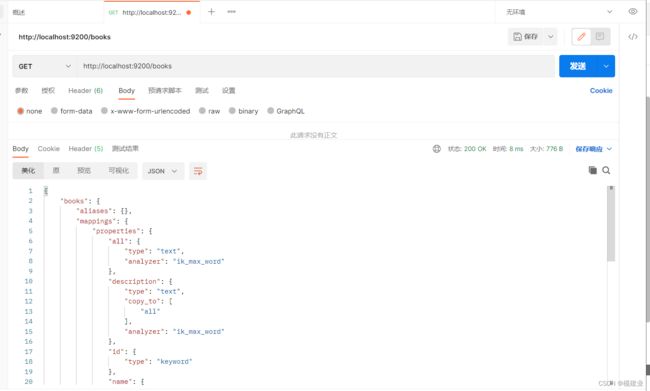
- 通过 Postman 添加文档(POST请求):
- 方式一:http://localhost:9200/books/_doc/id,此方式也可在URL中携带参数 id ,也可不在URL中携带 id ,不在URL中携带 id时,id会自动生成。在请求体中携带参数id无效。
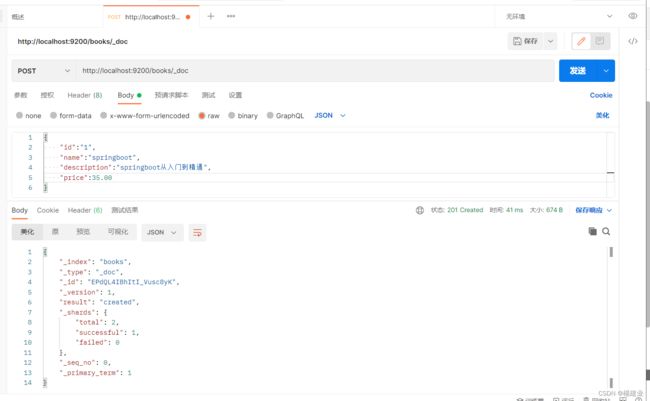
- 方式二:http://localhost:9200/books/_create/id,在请求体中携带参数id无效。
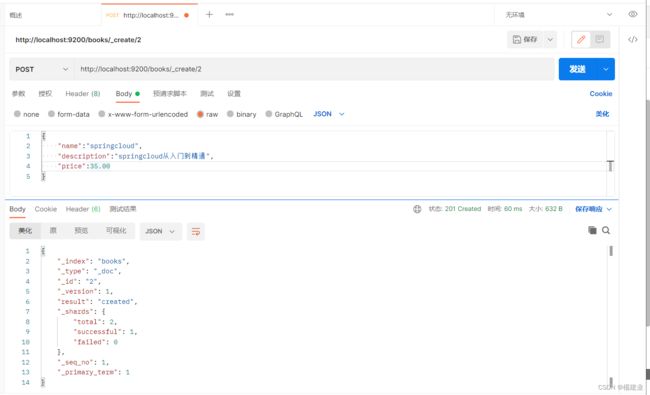
- 通过 Postman 查询文档(GET请求):
- http://localhost:9200/books/_doc/2
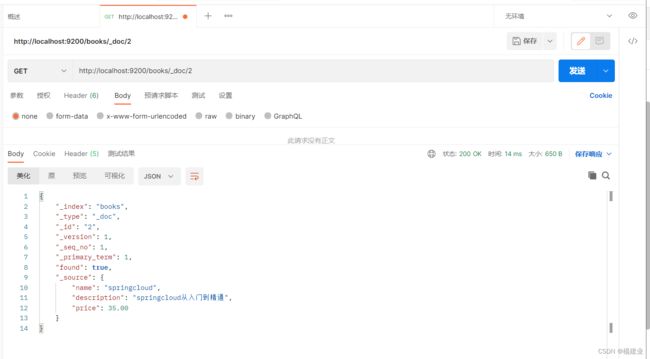
- 通过 Postman 查询全部文档(GET请求):
- http://localhost:9200/books/_search
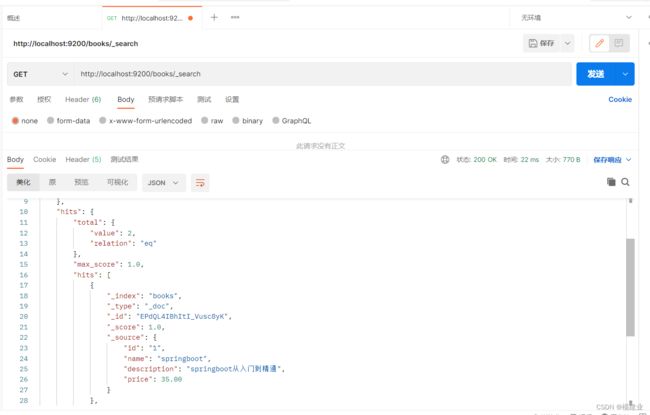
- 通过 Postman 按条件查询文档(GET请求):
- http://localhost:9200/books/_search?q=name:springcloud
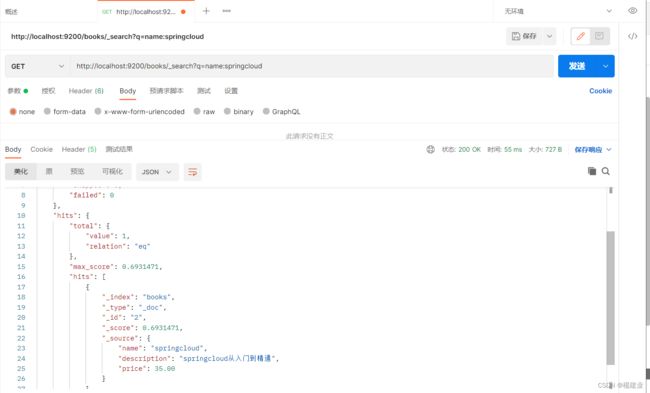
- 通过 Postman 删除文档(DELETE请求):
- http://localhost:9200/books/_doc/2
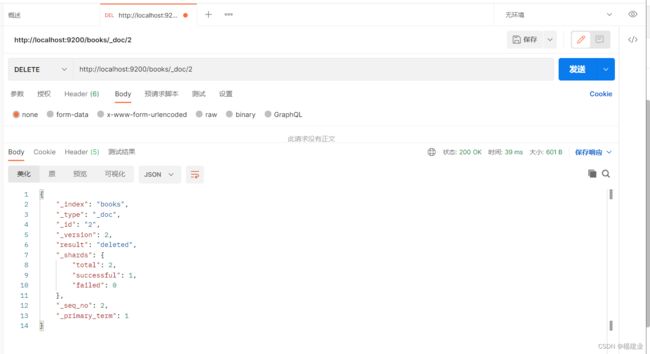
- 通过 Postman 修改文档(POST请求):
- http://localhost:9200/books/_update/2,且要携带要修改的参数信息:
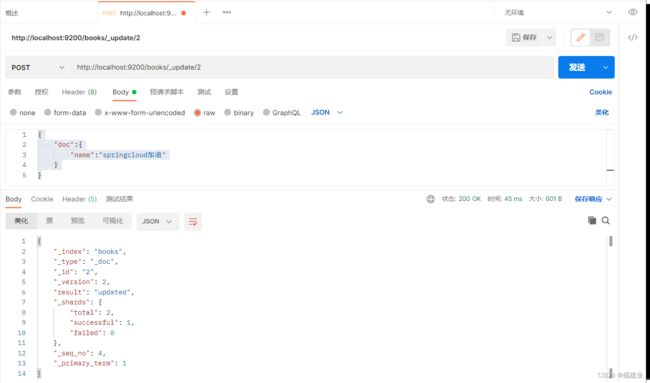
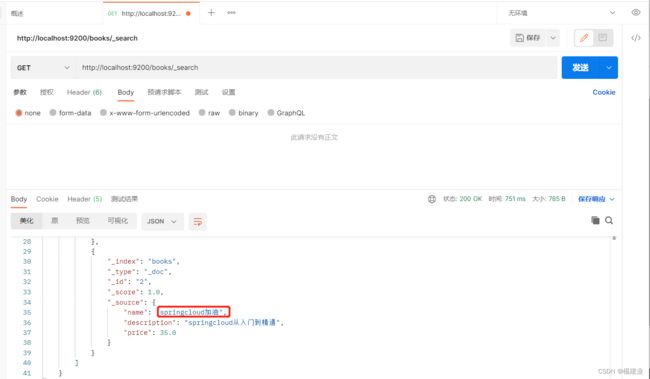
+ SpringBoot 整合 Elasticsearch ( ES ) 代码示例
- pom.xml添加相关依赖:
<dependency>
<groupId>org.projectlombokgroupId>
<artifactId>lombokartifactId>
<optional>trueoptional>
dependency>
<dependency>
<groupId>org.springframework.bootgroupId>
<artifactId>spring-boot-starter-testartifactId>
<scope>testscope>
dependency>
<dependency>
<groupId>mysqlgroupId>
<artifactId>mysql-connector-javaartifactId>
<version>8.0.28version>
dependency>
<dependency>
<groupId>com.baomidougroupId>
<artifactId>mybatis-plus-boot-starterartifactId>
<version>3.4.3version>
dependency>
<dependency>
<groupId>com.alibabagroupId>
<artifactId>druid-spring-boot-starterartifactId>
<version>1.2.6version>
dependency>
<dependency>
<groupId>org.elasticsearch.clientgroupId>
<artifactId>elasticsearch-rest-high-level-clientartifactId>
dependency>
- yml配置:
spring:
datasource:
druid:
driver-class-name: com.mysql.cj.jdbc.Driver
url: jdbc:mysql://localhost:3306/springboot?serverTimezone=UTC
username: user
password: 123456
mybatis-plus:
global-config:
db-config:
table-prefix: tbl_
id-type: assign_uuid
configuration:
log-impl: org.apache.ibatis.logging.stdout.StdOutImpl
- 创建索引:
import com.example.springboot.dao.BookDao;
import org.apache.http.HttpHost;
import org.elasticsearch.action.admin.indices.create.CreateIndexRequest;
import org.elasticsearch.client.RequestOptions;
import org.elasticsearch.client.RestClient;
import org.elasticsearch.client.RestClientBuilder;
import org.elasticsearch.client.RestHighLevelClient;
import org.elasticsearch.xcontent.XContentType;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
@SpringBootTest
public class SpringBootESTest {
private RestHighLevelClient client;
@Test
void testCreateIndexByIK() {
try {
HttpHost host = HttpHost.create("http://localhost:9200");
RestClientBuilder builder = RestClient.builder(host);
client = new RestHighLevelClient(builder);
CreateIndexRequest request = new CreateIndexRequest("books");
String json = "{\n" +
" \"mappings\":{\n" +
" \"properties\":{\n" +
" \"id\":{\n" +
" \"type\":\"keyword\"\n" +
" },\n" +
" \"name\":{\n" +
" \"type\":\"text\",\n" +
" \"analyzer\":\"ik_max_word\",\n" +
" \"copy_to\":\"all\"\n" +
" },\n" +
" \"description\":{\n" +
" \"type\":\"text\",\n" +
" \"analyzer\":\"ik_max_word\",\n" +
" \"copy_to\":\"all\"\n" +
" },\n" +
" \"price\":{\n" +
" \"type\":\"keyword\"\n" +
" },\n" +
" \"all\":{\n" +
" \"type\":\"text\",\n" +
" \"analyzer\":\"ik_max_word\"\n" +
" }\n" +
" }\n" +
" }\n" +
"}";
request.source(json, XContentType.JSON);
client.indices().create(request, RequestOptions.DEFAULT);
client.close();
}catch (Exception e){
e.printStackTrace();
}
}
}
- 执行测试代码后,通过 Postman 测试:(备注,测试类抛异常了,json无法被正常解析,需要调试下);
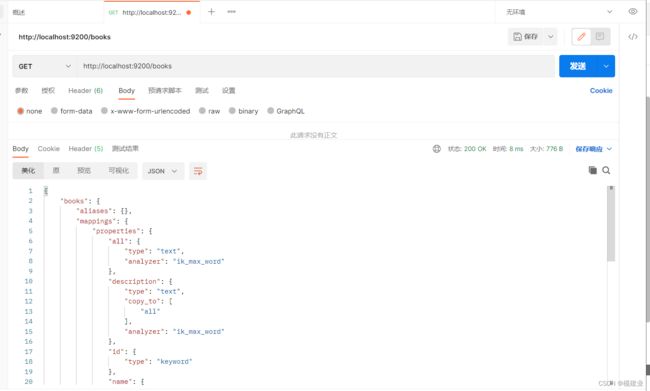
- 添加文档
import com.alibaba.fastjson.JSON;
import com.baomidou.mybatisplus.extension.handlers.FastjsonTypeHandler;
import com.example.springboot.dao.BookDao;
import com.example.springboot.entity.Book;
import org.apache.http.HttpHost;
import org.elasticsearch.action.admin.indices.create.CreateIndexRequest;
import org.elasticsearch.action.bulk.BulkRequest;
import org.elasticsearch.action.index.IndexRequest;
import org.elasticsearch.client.RequestOptions;
import org.elasticsearch.client.RestClient;
import org.elasticsearch.client.RestClientBuilder;
import org.elasticsearch.client.RestHighLevelClient;
import org.elasticsearch.xcontent.XContentType;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import java.util.List;
@SpringBootTest
public class SpringBootESTest {
@Autowired
private BookDao bookDao;
private RestHighLevelClient client;
@Test
void testCreateIndexByIK() {
try {
HttpHost host = HttpHost.create("http://localhost:9200");
RestClientBuilder builder = RestClient.builder(host);
client = new RestHighLevelClient(builder);
CreateIndexRequest request = new CreateIndexRequest("books");
String json = "{" +
" \"mappings\":{" +
" \"properties\":{" +
" \"id\":{" +
" \"type\":\"keyword\"" +
" }," +
" \"name\":{" +
" \"type\":\"text\"," +
" \"analyzer\":\"ik_max_word\"," +
" \"copy_to\":\"all\"" +
" }," +
" \"description\":{" +
" \"type\":\"text\"," +
" \"analyzer\":\"ik_max_word\"," +
" \"copy_to\":\"all\"" +
" }," +
" \"price\":{" +
" \"type\":\"keyword\"" +
" }," +
" \"all\":{" +
" \"type\":\"text\"," +
" \"analyzer\":\"ik_max_word\"" +
" }" +
" }" +
" }" +
"}";
request.source(json, XContentType.JSON);
client.indices().create(request, RequestOptions.DEFAULT);
client.close();
}catch (Exception e){
e.printStackTrace();
}
}
@Test
void createDoc(){
try {
HttpHost host = HttpHost.create("http://localhost:9200");
RestClientBuilder builder = RestClient.builder(host);
client = new RestHighLevelClient(builder);
Book book = bookDao.selectById("1");
String json = JSON.toJSONString(book);
IndexRequest indexRequest = new IndexRequest("books").id("1");
indexRequest.source(json, XContentType.JSON);
client.index(indexRequest, RequestOptions.DEFAULT);
client.close();
}catch (Exception e){
e.printStackTrace();
}
}
@Test
void createDocBulk(){
try {
HttpHost host = HttpHost.create("http://localhost:9200");
RestClientBuilder builder = RestClient.builder(host);
client = new RestHighLevelClient(builder);
List<Book> books = bookDao.selectList(null);
BulkRequest bulkRequest = new BulkRequest();
for (Book book : books) {
String json = JSON.toJSONString(book);
IndexRequest indexRequest = new IndexRequest("books").id(book.getId());
indexRequest.source(json, XContentType.JSON);
bulkRequest.add(indexRequest);
}
client.bulk(bulkRequest, RequestOptions.DEFAULT);
client.close();
}catch (Exception e){
e.printStackTrace();
}
}
}
- 通过 Postman 测试如下:
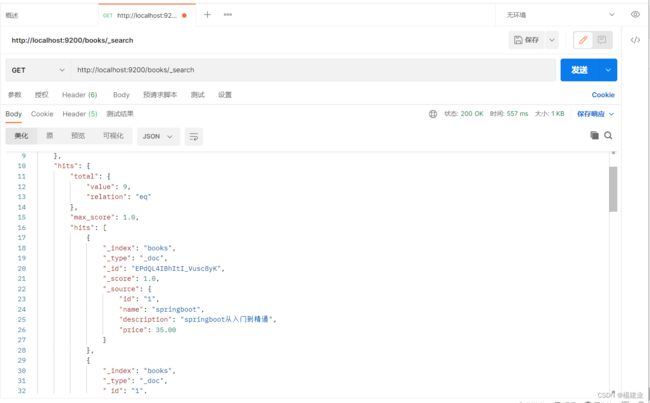
- 查询文档
import com.alibaba.fastjson.JSON;
import com.baomidou.mybatisplus.extension.handlers.FastjsonTypeHandler;
import com.example.springboot.dao.BookDao;
import com.example.springboot.entity.Book;
import org.apache.http.HttpHost;
import org.elasticsearch.action.admin.indices.create.CreateIndexRequest;
import org.elasticsearch.action.bulk.BulkRequest;
import org.elasticsearch.action.get.GetRequest;
import org.elasticsearch.action.get.GetResponse;
import org.elasticsearch.action.index.IndexRequest;
import org.elasticsearch.action.search.SearchRequest;
import org.elasticsearch.action.search.SearchResponse;
import org.elasticsearch.client.RequestOptions;
import org.elasticsearch.client.RestClient;
import org.elasticsearch.client.RestClientBuilder;
import org.elasticsearch.client.RestHighLevelClient;
import org.elasticsearch.index.query.QueryBuilder;
import org.elasticsearch.index.query.QueryBuilders;
import org.elasticsearch.search.SearchHit;
import org.elasticsearch.search.SearchHits;
import org.elasticsearch.search.builder.SearchSourceBuilder;
import org.elasticsearch.xcontent.XContentType;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import javax.naming.directory.SearchResult;
import java.util.List;
@SpringBootTest
public class SpringBootESTest {
@Autowired
private BookDao bookDao;
private RestHighLevelClient client;
@Test
void queryDocById(){
try {
HttpHost host = HttpHost.create("http://localhost:9200");
RestClientBuilder builder = RestClient.builder(host);
client = new RestHighLevelClient(builder);
GetRequest request = new GetRequest("books", "1");
GetResponse response = client.get(request, RequestOptions.DEFAULT);
String json = response.getSourceAsString();
System.out.println(json);
client.close();
}catch (Exception e){
e.printStackTrace();
}
}
@Test
void queryDocSearch(){
try {
HttpHost host = HttpHost.create("http://localhost:9200");
RestClientBuilder builder = RestClient.builder(host);
client = new RestHighLevelClient(builder);
SearchRequest searchRequest = new SearchRequest("books");
SearchSourceBuilder searchSourceBuilder = new SearchSourceBuilder();
searchSourceBuilder.query(QueryBuilders.termQuery("name", "springcloud"));
searchRequest.source(searchSourceBuilder);
SearchResponse searchResponse = client.search(searchRequest, RequestOptions.DEFAULT);
SearchHits hits = searchResponse.getHits();
for (SearchHit hit : hits) {
System.out.println(hit.getSourceAsString());
}
client.close();
}catch (Exception e){
e.printStackTrace();
}
}
}