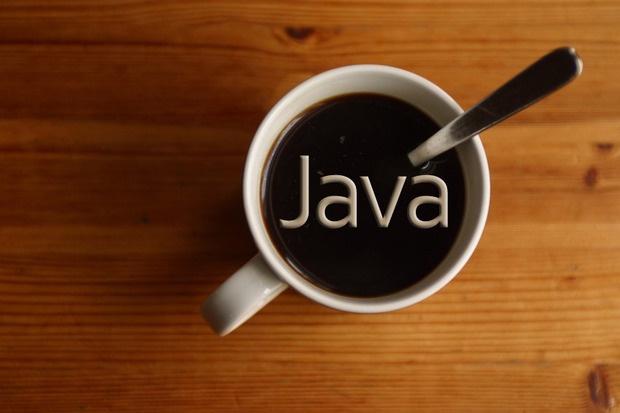
Java
线程阻塞
join
public class joinThread extends Thread {
@Override
public void run() {
for (int i = 0; i < 1000; i++) {
System.out.println("join......");
}
}
}
public void test1Thread() throws InterruptedException {
joinThread thread = new joinThread(); // 新生
thread.start(); // 就绪
for (int i = 0; i < 1000; i++) {
if (50 == i) {
thread.join(); // main方法阻塞
}
System.out.println("main......");
}
}
join:一个线程在占有CPU资源期间,可以让其他线程调用join方法和本线程联合。当前线程等待调用该方法的线程结束后,在重新排队等待CPU资源,以便恢复执行。
yield
public class yieldThread extends Thread {
@Override
public void run() {
for (int i = 0; i < 1000; i++) {
System.out.println("yield......");
}
}
}
public void test2Thread() {
yieldThread thread = new yieldThread();
thread.start();
for (int i = 0; i < 1000; i++) {
if (i % 20 == 0) {
Thread.yield();// 这个方法写在哪个线程,就暂停哪个线程
}
}
}
yield:指当前线程愿意让出当前的CPU资源,但是让出多久并没有规定,所以并不能打乱CPU的调度。此方法在哪个线程中调用,就暂时性的暂停哪个线程。此方法是一个静态方法。
sleep
静态方法,sleep:休眠,不释放锁,放弃CPU资源。
- 与时间相关:倒计时
- 模拟网络延时
/**
* 倒计时
* 倒数10个数,一秒内打印一个
* @throws InterruptedException
*/
public void threadSleep1() throws InterruptedException {
int num = 10;
while (true) {
Log.d("WM",(num--) + "");
Thread.sleep(1000); // 暂停一秒
if (num <= 0) {
break;
}
}
}
public void threadSleep2() throws InterruptedException {
Date endTime = new Date(System.currentTimeMillis() + 10 * 1000);
long end = endTime.getTime();
while (true) {
// 输出
Log.d("WM",new SimpleDateFormat("mm:ss").format(endTime) + "");
// 等待一秒
Thread.sleep(1000);
// 构建下一秒时间
endTime = new Date(endTime.getTime() - 1000);
// 10秒以内继续
if (end - 10000 > endTime.getTime()) {
break;
}
}
}
线程的优先级
isAlive()
判断线程是否还"活着",即线程是否还未终止。getPriority()
获得线程的优先级数值setPriority()
设置线程的优先级数值setName()
给线程一个名字getName()
取得线程的名字currentThread()
取得当前正在运行的线程对象也就是取得自己本身
public class MyThread implements Runnable {
private boolean flag = true;
private int number = 0;
@Override
public void run() {
while (flag) {
Log.d("WM",(Thread.currentThread().getName()) + "--->" +(number++) + "");
}
}
public void stop() {
this.flag = !this.flag;
}
}
给线程设置名字
public void test() throws InterruptedException {
MyThread it = new MyThread();
Thread proxy = new Thread(it);
proxy.setName("test1");
Log.d("WM",proxy.getName());
proxy.start();
Log.d("WM",Thread.currentThread().getName()); // 主线程 main
Thread.sleep(200);
it.stop();
}
默认优先级
public void test() throws InterruptedException {
MyThread it1 = new MyThread();
Thread p1 = new Thread(it1);
MyThread it2 = new MyThread();
Thread p2 = new Thread(it2);
p1.start();
p2.start();
Thread.sleep(100);
it1.stop();
it2.stop();
}
线程的优先级
/**
* 优先级:概率,不是绝对的先后顺序,而是运行的概率
* MAX_PRIORITY 最大优先级 (10)
* NORM_PRIORITY 默认优先级(5)
* MIN_PRIORITY 最小优先级 (0)
* @throws InterruptedException
*/
public void test() throws InterruptedException {
MyThread it1 = new MyThread();
Thread p1 = new Thread(it1);
MyThread it2 = new MyThread();
Thread p2 = new Thread(it2);
p1.setPriority(Thread.MAX_PRIORITY);
p2.setPriority(Thread.MIN_PRIORITY);
p1.start();
p2.start();
Thread.sleep(100);
it1.stop();
it2.stop();
}