import numpy as np
from scipy import signal
import cv2
import random
import math
def getClosenessWeight(sigma_g,H,W):
r,c=np.mgrid[0:H:1,0:W:1]
r -= (H - 1) // 2
c -= int(W - 1) // 2
closeWeight=np.exp(-0.5*(np.power(r,2)+np.power(c,2))/math.pow(sigma_g,2))
return closeWeight
def bfltGray(I,H,W,sigma_g,sigma_d):
closenessWeight=getClosenessWeight(sigma_g,H,W)
cH = (H - 1) // 2
cW = (W - 1) // 2
rows,cols=I.shape
bfltGrayImage=np.zeros(I.shape,np.float32)
for r in range(rows):
for c in range(cols):
pixel=I[r][c]
rTop=0 if r-cH<0 else r-cH
rBottom=rows-1 if r+cH>rows-1 else r+cH
cLeft=0 if c-cW<0 else c-cW
cRight=cols-1 if c+cW>cols-1 else c+cW
region=I[rTop:rBottom+1,cLeft:cRight+1]
similarityWeightTemp=np.exp(-0.5*np.power(region-pixel,2.0)/math.pow(sigma_d,2))
closenessWeightTemp=closenessWeight[rTop-r+cH:rBottom-r+cH+1,cLeft-c+cW:cRight-c+cW+1]
weightTemp=similarityWeightTemp*closenessWeightTemp
weightTemp=weightTemp/np.sum(weightTemp)
bfltGrayImage[r][c]=np.sum(region*weightTemp)
return bfltGrayImage
if __name__=='__main__':
a= cv2.imread('D:/2.png', cv2.IMREAD_UNCHANGED)
image1 = cv2.split(a)[0]
cv2.imshow("image1",image1)
image1=image1/255.0
bfltImage=bfltGray(image1,3,3,19,0.2)
cv2.imshow("增强后图",bfltImage)
cv2.waitKey(0)
cv2.destroyAllWindows()
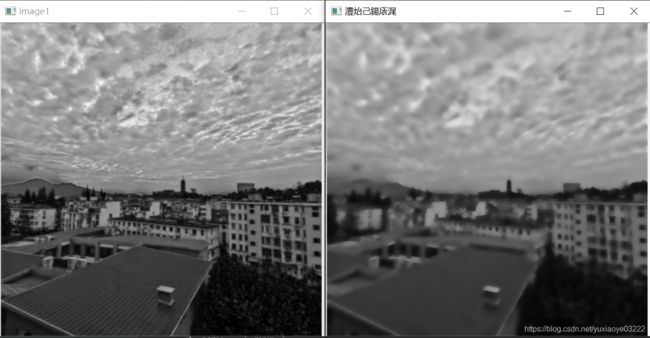
左原图,右面处理后的