文章目录
- 第五节 搭建环境:辅助功能
-
- 1、常量类
- 2、MD5 加密工具方法
- 3、日志配置文件
- 第六节 业务功能:登录
-
- 1、显示首页
-
- ①流程图
- ②创建 PortalServlet
-
- ③在 index.html 中编写登录表单
- 2、登录操作
-
- ①流程图
- ②创建 EmpService
- ③创建登录失败异常
- ④增加常量声明
- ⑤创建 AuthServlet
-
- ⑥EmpService 方法
- ⑦EmpDao 方法
- ⑧临时页面
- 3、退出登录
-
- ①在临时页面编写超链接
- ②在 AuthServlet 编写退出逻辑
第五节 搭建环境:辅助功能
1、常量类
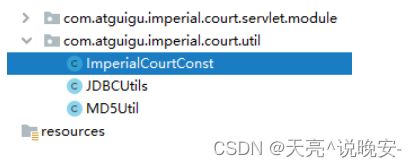
public class ImperialCourtConst {
public static final String LOGIN_FAILED_MESSAGE = "账号、密码错误,不可进宫!";
public static final String ACCESS_DENIED_MESSAGE = "宫闱禁地,不得擅入!";
}
2、MD5 加密工具方法
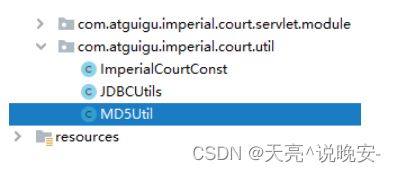
public class MD5Util {
public static String encode(String source) {
if (source == null || "".equals(source)) {
throw new RuntimeException("用于加密的明文不可为空");
}
String algorithm = "md5";
MessageDigest messageDigest = null;
try {
messageDigest = MessageDigest.getInstance(algorithm);
} catch (NoSuchAlgorithmException e) {
e.printStackTrace();
}
byte[] input = source.getBytes();
byte[] output = messageDigest.digest(input);
int signum = 1;
BigInteger bigInteger = new BigInteger(signum, output);
int radix = 16;
String encoded = bigInteger.toString(radix).toUpperCase();
return encoded;
}
}
3、日志配置文件
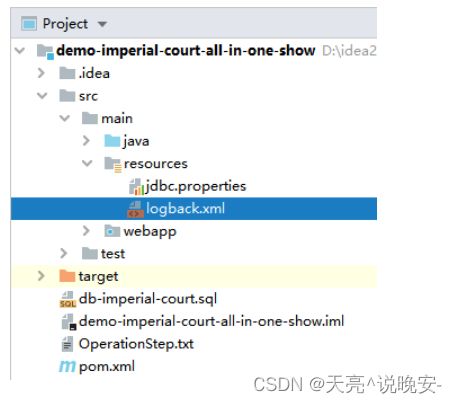
<configuration debug="true">
<appender name="STDOUT"
class="ch.qos.logback.core.ConsoleAppender">
<encoder>
<pattern>[%d{HH:mm:ss.SSS}] [%-5level] [%thread] [%logger] [%msg]%npattern>
<charset>UTF-8charset>
encoder>
appender>
<root level="INFO">
<appender-ref ref="STDOUT" />
root>
<logger name="com.atguigu" level="DEBUG" additivity="false">
<appender-ref ref="STDOUT" />
logger>
configuration>
第六节 业务功能:登录
1、显示首页
①流程图
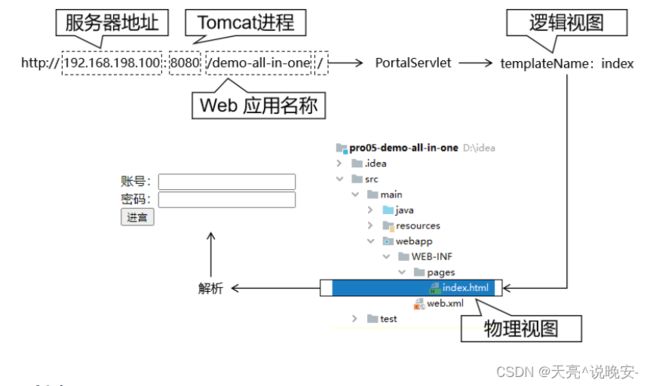
②创建 PortalServlet
[1]创建 Java 类
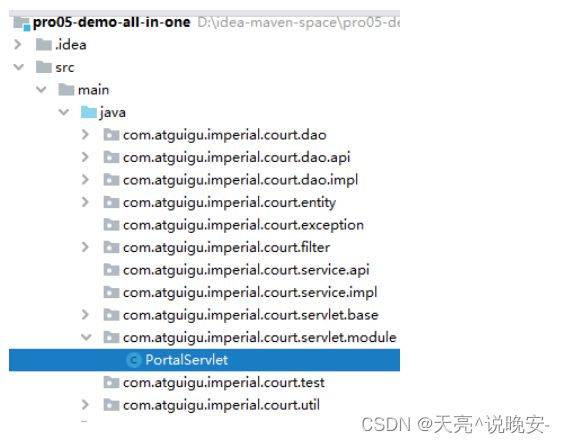
public class PortalServlet extends ViewBaseServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
doPost(req, resp);
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
String templateName = "index";
processTemplate(templateName, req, resp);
}
}
[2]注册
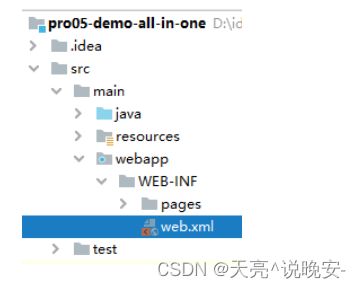
<servlet>
<servlet-name>portalServletservlet-name>
<servlet-class>com.atguigu.imperial.court.servlet.module.PortalServletservlet-class>
servlet>
<servlet-mapping>
<servlet-name>portalServletservlet-name>
<url-pattern>/url-pattern>
servlet-mapping>
③在 index.html 中编写登录表单
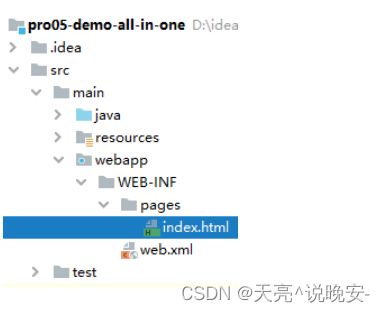
DOCTYPE html>
<html lang="en" xml:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Titletitle>
head>
<body>
<form th:action="@{/auth}" method="post">
<input type="hidden" name="method" value="login" />
<p th:text="${message}">p>
<p th:text="${systemMessage}">p>
账号:<input type="text" name="loginAccount"/><br/>
密码:<input type="password" name="loginPassword"><br/>
<button type="submit">进宫button>
form>
body>
html>
2、登录操作
①流程图
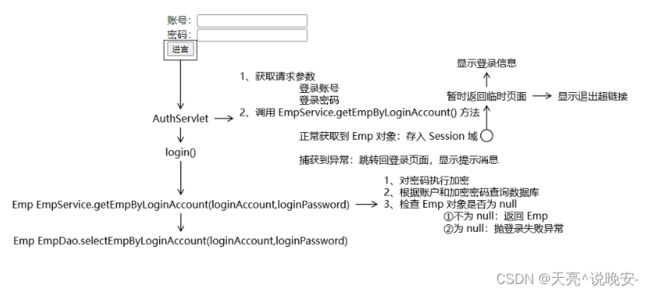
②创建 EmpService
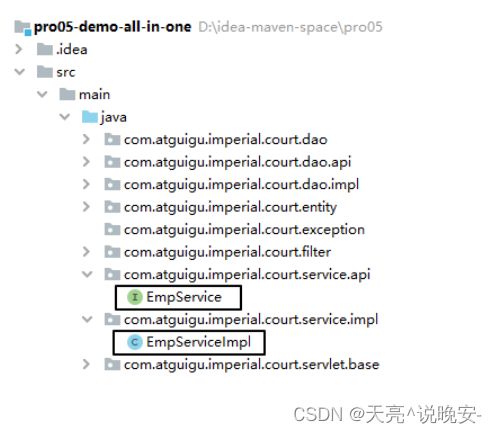
③创建登录失败异常

public class LoginFailedException extends RuntimeException {
public LoginFailedException() {
}
public LoginFailedException(String message) {
super(message);
}
public LoginFailedException(String message, Throwable cause) {
super(message, cause);
}
public LoginFailedException(Throwable cause) {
super(cause);
}
public LoginFailedException(String message, Throwable cause, boolean enableSuppression, boolean writableStackTrace) {
super(message, cause, enableSuppression, writableStackTrace);
}
}
④增加常量声明
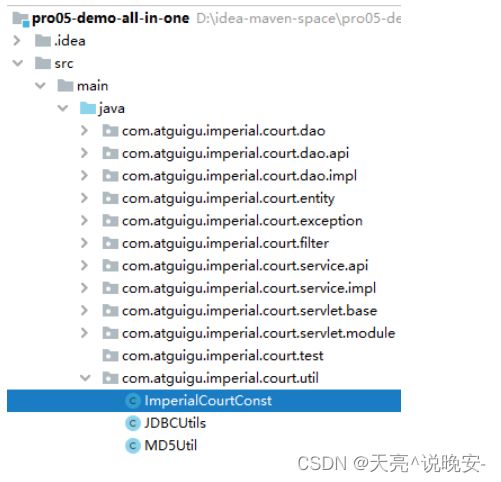
public class ImperialCourtConst {
public static final String LOGIN_FAILED_MESSAGE = "账号、密码错误,不可进宫!";
public static final String ACCESS_DENIED_MESSAGE = "宫闱禁地,不得擅入!";
public static final String LOGIN_EMP_ATTR_NAME = "loginInfo";
}
⑤创建 AuthServlet
[1]创建 Java 类
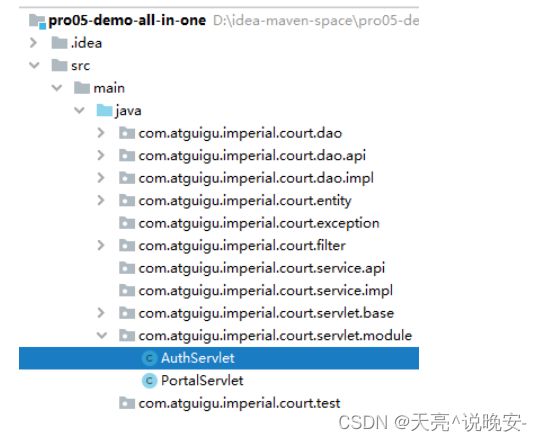
public class AuthServlet extends ModelBaseServlet {
private EmpService empService = new EmpServiceImpl();
protected void login(
HttpServletRequest request,
HttpServletResponse response)
throws ServletException, IOException {
try {
String loginAccount = request.getParameter("loginAccount");
String loginPassword = request.getParameter("loginPassword");
Emp emp = empService.getEmpByLoginAccount(loginAccount, loginPassword);
HttpSession session = request.getSession();
session.setAttribute(ImperialCourtConst.LOGIN_EMP_ATTR_NAME, emp);
String templateName = "temp";
processTemplate(templateName, request, response);
} catch (Exception e) {
e.printStackTrace();
if (e instanceof LoginFailedException) {
request.setAttribute("message", e.getMessage());
processTemplate("index", request, response);
}else {
throw new RuntimeException(e);
}
}
}
}
[2]注册
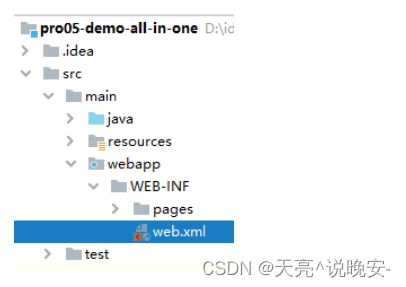
<servlet>
<servlet-name>authServletservlet-name>
<servlet-class>com.atguigu.imperial.court.servlet.module.AuthServletservlet-class>
servlet>
<servlet-mapping>
<servlet-name>authServletservlet-name>
<url-pattern>/authurl-pattern>
servlet-mapping>
⑥EmpService 方法
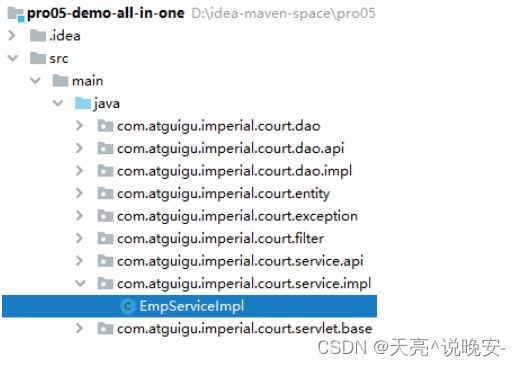
public class EmpServiceImpl implements EmpService {
private EmpDao empDao = new EmpDaoImpl();
@Override
public Emp getEmpByLoginAccount(String loginAccount, String loginPassword) {
String encodedLoginPassword = MD5Util.encode(loginPassword);
Emp emp = empDao.selectEmpByLoginAccount(loginAccount, encodedLoginPassword);
if (emp != null) {
return emp;
} else {
throw new LoginFailedException(ImperialCourtConst.LOGIN_FAILED_MESSAGE);
}
}
}
⑦EmpDao 方法
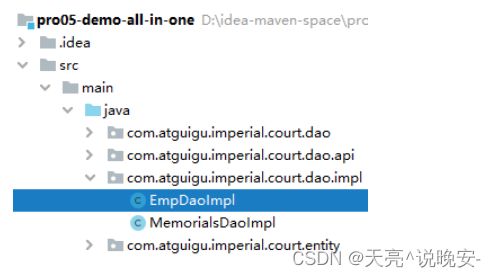
public class EmpDaoImpl extends BaseDao<Emp> implements EmpDao {
@Override
public Emp selectEmpByLoginAccount(String loginAccount, String encodedLoginPassword) {
String sql = "select emp_id empId," +
"emp_name empName," +
"emp_position empPosition," +
"login_account loginAccount," +
"login_password loginPassword " +
"from t_emp where login_account=? and login_password=?";
return super.getSingleBean(sql, Emp.class, loginAccount, encodedLoginPassword);
}
}
⑧临时页面
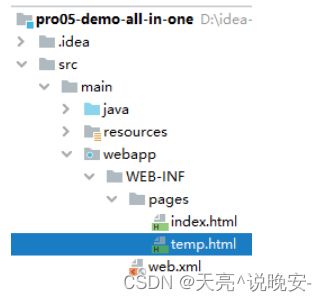
DOCTYPE html>
<html lang="en" xml:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>临时title>
head>
<body>
<p th:text="${session.loginInfo}">p>
body>
html>
3、退出登录
①在临时页面编写超链接
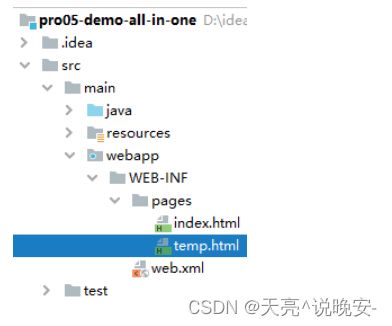
<a th:href="@{/auth?method=logout}">退朝a>
②在 AuthServlet 编写退出逻辑
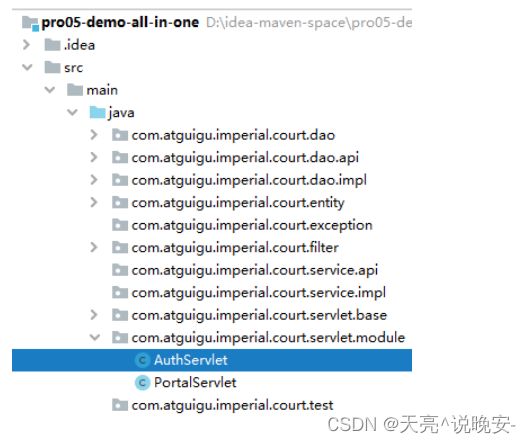
protected void logout(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
HttpSession session = request.getSession();
session.invalidate();
String templateName = "index";
processTemplate(templateName, request, response);
}