如需转载请评论或简信,并注明出处,未经允许不得转载
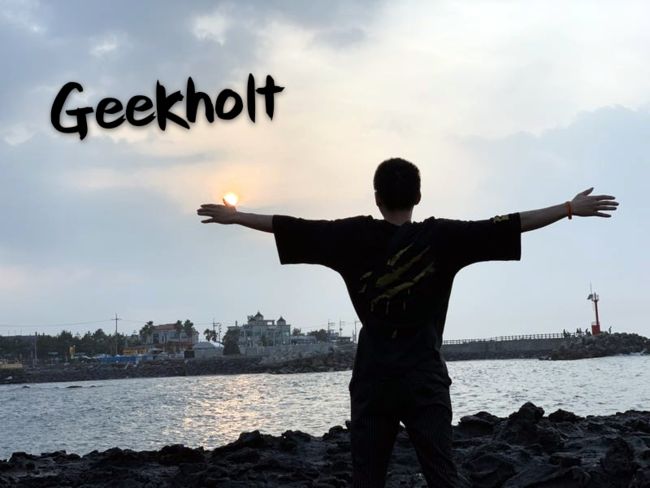
Kotlin系列导读
Kotlin学习手册(一)类与继承
Kotlin学习手册(二)属性与字段
Kotlin学习手册(三)接口
Kotlin学习手册(四)内部类
Kotlin学习手册(五)函数
Kotlin学习手册(六)数组与集合
Kotlin学习手册(七)for循环
Kotlin学习手册(八)内联函数let、with、run、apply、also
Kotlin学习手册(九)空类型安全
Kotlin学习手册(十)带你真正理解什么是Kotlin协程
目录
函数声明
返回值void的函数
java
public void printStr() {
System.out.print("Hello World");
}
kotlin
//kotlin用fun关键字声明一个函数,Unit表示返回值类型为空
fun printStr(): Unit {
print("Hello world")
}
或
//当返回值类型为空时,Unit也可以省略
fun printStr() {
print("Hello world")
}
返回值为Int的函数
java
public int sum(int a, int b) {
return a + b;
}
kotlin
fun sum(a: Int, b: Int): Int {
return a + b
}
或
//将表达式作为函数体、返回值类型自动推断
fun sum(a: Int, b: Int) = a + b
静态函数
java
public static int sum(int a, int b) {
return a + b;
}
kotlin
//使用companion object来声明静态的方法或变量
companion object {
fun sum3(a: Int, b: Int) = a + b
}
函数调用
调用类成员方法
java
int sum = new JavaDemo().sum(1,2);
kotlin
val sum = KotlinDemo().sum(1, 2)
调用类静态方法
java
int sum = JavaDemo.sum(1,2);
kotlin
val sum = KotlinDemo.sum(1,2)
函数参数
默认参数
java
public void read(List b, int off, int len) { /*……*/ }
//有时候我们为了给函数提供默认参数,会写一个重载的方法
public void read(List b) {
read(b, 0, b.size());
}
kotlin
//函数参数可以有默认值,当省略相应的参数时使用默认值。这可以减少重载数量
fun read(b: Array, off: Int = 0, len: Int = b.size) { /*……*/ }
这里要注意的是,重写的方法不可以有默认值,如
open class A {
open fun foo(i: Int = 10) { /*……*/ }
}
class B : A() {
override fun foo(i: Int) { /*……*/ } // 不能有默认值
}
如果一个默认参数在一个无默认值的参数之前,那么该默认值只能通过使用命名参数调用该函数来使用:
fun foo(bar: Int = 0, baz: Int) { /*……*/ }
foo(baz = 1) // 使用默认值 bar = 0
如果在默认参数之后的最后一个参数是 lambda 表达式,那么它既可以作为命名参数在括号内传入,也可以在括号外传入:
fun foo(bar: Int = 0, baz: Int = 1, qux: () -> Unit) { /*……*/ }
foo(1) { println("hello") } // 使用默认值 baz = 1
foo(qux = { println("hello") }) // 使用两个默认值 bar = 0 与 baz = 1
foo { println("hello") } // 使用两个默认值 bar = 0 与 baz = 1
可变数量的参数(Varargs)
函数的参数(通常是最后一个)可以用 varargs
修饰符标记:
fun asList(vararg ts: T): List {
val result = ArrayList()
for (t in ts) // ts is an Array
result.add(t)
return result
}
允许将可变数量的参数传递给函数
val list = asList(1, 2, 3)
函数类型
成员函数
成员函数是在类或对象内部定义的函数
class Sample() {
fun foo() { print("Foo") }
}
局部函数
Kotlin 支持局部函数,即一个函数在另一个函数内部:
fun dfs(graph: Graph) {
fun dfs(current: Vertex, visited: MutableSet) {
if (!visited.add(current)) return
for (v in current.neighbors)
dfs(v, visited)
}
dfs(graph.vertices[0], HashSet())
}
局部函数可以访问外部函数(即闭包)的局部变量,所以在上例中,visited 可以是局部变量
fun dfs(graph: Graph) {
val visited = HashSet()
fun dfs(current: Vertex) {
if (!visited.add(current)) return
for (v in current.neighbors)
dfs(v)
}
dfs(graph.vertices[0])
}
泛型函数
函数可以有泛型参数,通过在函数名前使用尖括号指定
fun singletonList(item: T): List { /*……*/ }
扩展函数
声明一个扩展函数,我们需要用一个 接收者类型 也就是被扩展的类型来作为他的前缀。 下面代码为 MutableList
添加一个swap
函数
fun MutableList.swap(index1: Int, index2: Int) {
val tmp = this[index1] // “this”对应该列表
this[index1] = this[index2]
this[index2] = tmp
}
这个 this
关键字在扩展函数内部对应到接收者对象(传过来的在点符号前的对象)
现在,我们对任意 MutableList
调用该函数了
val list = mutableListOf(1, 2, 3)
list.swap(0, 2) // “swap()”内部的“this”会保存“list”的值