这里写目录标题
- 一、绝对值不等式
-
- 二、推公式
-
- 1、题目内容——耍杂技的牛
- 2、算法思路
- 3、题解
一、绝对值不等式
1、题目内容——货仓选址
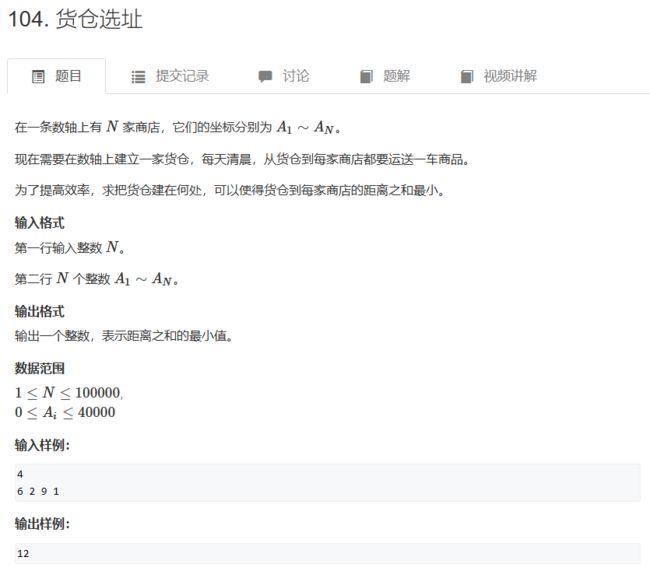
2、算法思路
(1)绝对值不等式
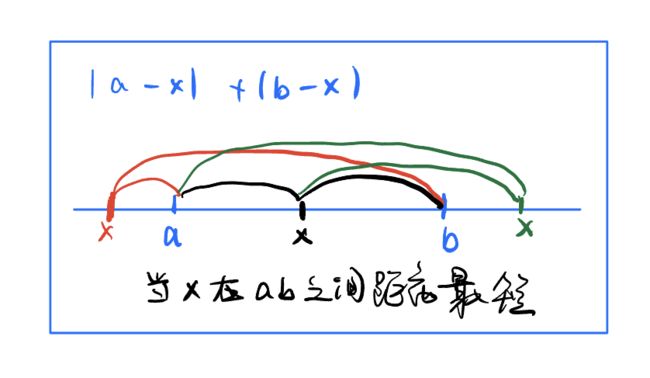
(2)算法思路
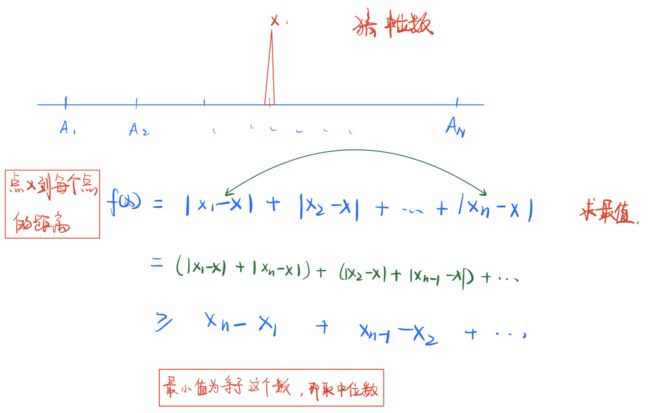
3、题解
import java.util.*;
import java.io.*;
public class Main{
static int N = 100010;
static int[] a = new int[N];
public static void main(String[] args) throws IOException {
BufferedReader in = new BufferedReader(new InputStreamReader(System.in));
String str1 = in.readLine();
int n = Integer.parseInt(str1);
String[] str2 = in.readLine().split(" ");
for(int i = 0; i < n; i++){
a[i] = Integer.parseInt(str2[i]);
}
Arrays.sort(a, 0, n);
long res = 0;
for(int i = 0; i < n; i++){
res += Math.abs(a[i] - a[n / 2]);
}
System.out.println(res);
}
}
二、推公式
1、题目内容——耍杂技的牛
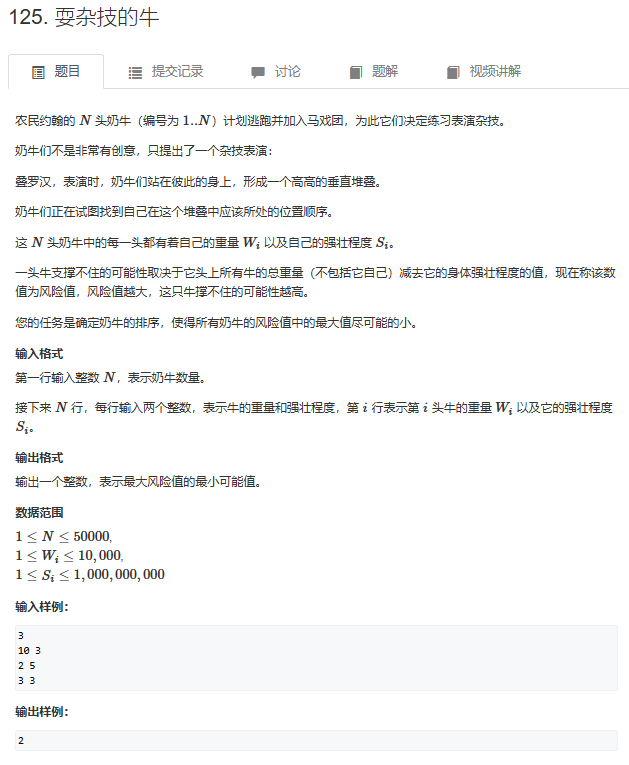
2、算法思路
- 排序方法:、
-
- 按照 wi + si 从小到大的顺序进行排序,值最大的,危险系数一定是最小的
3、题解
import java.util.*;
import java.io.*;
public class Main{
public static void main(String[] args) throws IOException {
BufferedReader in = new BufferedReader(new InputStreamReader(System.in));
List<PIIs> list = new ArrayList<PIIs>();
String str1 = in.readLine();
int n = Integer.parseInt(str1);
for(int i = 0; i < n; i++){
String[] str2 = in.readLine().split(" ");
int w = Integer.parseInt(str2[0]);
int s = Integer.parseInt(str2[1]);
list.add(new PIIs(w + s, w));
}
Collections.sort(list);
int res = Integer.MIN_VALUE;
int sumWeigt = 0;
for(PIIs item:list){
int w = item.getSecond();
int s = item.getFirst() - w;
res = Math.max(res, sumWeigt - s);
sumWeigt += w;
}
System.out.println(res);
}
}
class PIIs implements Comparable<PIIs>{
private int first;
private int second;
public PIIs(int first, int second){
this.first = first;
this.second = second;
}
public int getFirst(){
return this.first;
}
public int getSecond(){
return this.second;
}
public int compareTo(PIIs o){
return Integer.compare(this.first, o.first);
}
}