1
using
System;
2
using
System.Drawing;
3
using
System.Drawing.Imaging;
4
using
System.Windows.Forms;
5
6
namespace
GraphicsCopyright
7
{
8
///
<summary>
9
///
Summary description for Form1.
10
///
</summary>
11
public
class
Form1 : System.Windows.Forms.Form
12
{
13
Image originalimage;
14
15
private
System.Windows.Forms.OpenFileDialog openFileDialog1;
16
private
System.Windows.Forms.PictureBox pictureBox1;
17
private
System.Windows.Forms.GroupBox groupBox1;
18
private
System.Windows.Forms.Button btnAddCopyright;
19
private
System.Windows.Forms.Button btnOpenFile;
20
///
<summary>
21
///
Required designer variable.
22
///
</summary>
23
private
System.ComponentModel.Container components
=
null
;
24
25
public
Form1()
26
{
27
//
28
//
Required for Windows Form Designer support
29
//
30
InitializeComponent();
31
32
//
33
//
TODO: Add any constructor code after InitializeComponent call
34
//
35
}
36
37
///
<summary>
38
///
Clean up any resources being used.
39
///
</summary>
40
protected
override
void
Dispose(
bool
disposing )
41
{
42
if
( disposing )
43
{
44
if
(components
!=
null
)
45
{
46
components.Dispose();
47
}
48
}
49
base
.Dispose( disposing );
50
}
51
52
#region
Windows Form Designer generated code
53
///
<summary>
54
///
Required method for Designer support - do not modify
55
///
the contents of this method with the code editor.
56
///
</summary>
57
private
void
InitializeComponent()
58
{
59
this
.openFileDialog1
=
new
System.Windows.Forms.OpenFileDialog();
60
this
.btnAddCopyright
=
new
System.Windows.Forms.Button();
61
this
.btnOpenFile
=
new
System.Windows.Forms.Button();
62
this
.pictureBox1
=
new
System.Windows.Forms.PictureBox();
63
this
.groupBox1
=
new
System.Windows.Forms.GroupBox();
64
this
.SuspendLayout();
65
//
66
//
btnAddCopyright
67
//
68
this
.btnAddCopyright.Enabled
=
false
;
69
this
.btnAddCopyright.Location
=
new
System.Drawing.Point(
216
,
240
);
70
this
.btnAddCopyright.Name
=
"
btnAddCopyright
"
;
71
this
.btnAddCopyright.Size
=
new
System.Drawing.Size(
164
,
37
);
72
this
.btnAddCopyright.TabIndex
=
0
;
73
this
.btnAddCopyright.Text
=
"
添加版权信息
"
;
74
this
.btnAddCopyright.Click
+=
new
System.EventHandler(
this
.btnAddCopyright_Click);
75
//
76
//
btnOpenFile
77
//
78
this
.btnOpenFile.Location
=
new
System.Drawing.Point(
32
,
240
);
79
this
.btnOpenFile.Name
=
"
btnOpenFile
"
;
80
this
.btnOpenFile.Size
=
new
System.Drawing.Size(
164
,
37
);
81
this
.btnOpenFile.TabIndex
=
0
;
82
this
.btnOpenFile.Text
=
"
打开图像文件
"
;
83
this
.btnOpenFile.Click
+=
new
System.EventHandler(
this
.btnOpenFile_Click);
84
//
85
//
pictureBox1
86
//
87
this
.pictureBox1.BorderStyle
=
System.Windows.Forms.BorderStyle.Fixed3D;
88
this
.pictureBox1.Location
=
new
System.Drawing.Point(
8
,
0
);
89
this
.pictureBox1.Name
=
"
pictureBox1
"
;
90
this
.pictureBox1.Size
=
new
System.Drawing.Size(
400
,
224
);
91
this
.pictureBox1.TabIndex
=
0
;
92
this
.pictureBox1.TabStop
=
false
;
93
//
94
//
groupBox1
95
//
96
this
.groupBox1.Location
=
new
System.Drawing.Point(
24
,
224
);
97
this
.groupBox1.Name
=
"
groupBox1
"
;
98
this
.groupBox1.Size
=
new
System.Drawing.Size(
368
,
64
);
99
this
.groupBox1.TabIndex
=
1
;
100
this
.groupBox1.TabStop
=
false
;
101
//
102
//
Form1
103
//
104
this
.AutoScaleBaseSize
=
new
System.Drawing.Size(
6
,
14
);
105
this
.ClientSize
=
new
System.Drawing.Size(
416
,
301
);
106
this
.Controls.Add(
this
.pictureBox1);
107
this
.Controls.Add(
this
.btnAddCopyright);
108
this
.Controls.Add(
this
.btnOpenFile);
109
this
.Controls.Add(
this
.groupBox1);
110
this
.Name
=
"
Form1
"
;
111
this
.Text
=
"
给图像添加版权信息
"
;
112
this
.Load
+=
new
System.EventHandler(
this
.Form1_Load);
113
this
.ResumeLayout(
false
);
114
115
}
116
#endregion
117
118
///
<summary>
119
///
The main entry point for the application.
120
///
</summary>
121
[STAThread]
122
static
void
Main()
123
{
124
Application.Run(
new
Form1());
125
}
126
127
private
void
btnOpenFile_Click(
object
sender, System.EventArgs e)
128
{
129
//
Stream myStream;
130
OpenFileDialog openFileDialog1
=
new
OpenFileDialog();
131
132
openFileDialog1.InitialDirectory
=
"
c:\\
"
;
133
openFileDialog1.Filter
=
"
All files (*.*)|*.*
"
;
134
openFileDialog1.FilterIndex
=
2
;
135
openFileDialog1.RestoreDirectory
=
true
;
136
137
if
(openFileDialog1.ShowDialog()
==
DialogResult.OK)
138
{
139
originalimage
=
System.Drawing.Image.FromFile(openFileDialog1.FileName.ToString());
140
Image ithumbnail
=
originalimage.GetThumbnailImage(
200
,
200
,
null
,
new
IntPtr());
141
pictureBox1.Image
=
ithumbnail;
142
btnAddCopyright.Enabled
=
true
;
143
}
144
}
145
146
private
void
btnAddCopyright_Click(
object
sender, System.EventArgs e)
147
{
148
int
imagewidth;
149
int
imageheight;
150
int
fontsize
=
300
;
151
int
x,y;
152
int
a,re,gr,bl,x1,y1,z1;
153
int
size;
154
Bitmap pattern;
155
SizeF sizeofstring;
156
bool
foundfont;
157
imagewidth
=
originalimage.Width;
158
imageheight
=
originalimage.Height;
159
size
=
imagewidth
*
imageheight;
160
pattern
=
new
Bitmap(imagewidth,imageheight);
161
Bitmap temp
=
new
Bitmap(originalimage);
162
Graphics g
=
Graphics.FromImage(pattern);
163
Graphics tempg
=
Graphics.FromImage(originalimage);
164
//
find a font size that will fit in the bitmap
165
foundfont
=
false
;
166
g.Clear(Color.White);
167
while
(foundfont
==
false
)
168
{
169
Font fc
=
new
Font(
"
Georgia
"
, fontsize, System.Drawing.FontStyle.Bold);
170
171
sizeofstring
=
new
SizeF(imagewidth,imageheight);
172
sizeofstring
=
g.MeasureString(
"
DOTNET
"
,fc);
173
if
(sizeofstring.Width
<
pattern.Width)
174
{
175
if
(sizeofstring.Height
<
pattern.Height)
176
{
177
foundfont
=
true
;
178
g.DrawString(
"
DOTNET
"
, fc,
new
SolidBrush(Color.Black),
1
,
1
);
179
}
180
181
}
182
else
183
fontsize
=
fontsize
-
1
;
184
}
185
MessageBox.Show(
"
已创建新文件
"
,
"
给图像添加版权信息
"
);
186
for
(x
=
1
;x
<
pattern.Width;x
++
)
187
{
188
for
(y
=
1
;y
<
pattern.Height;y
++
)
//
189
{
190
if
(pattern.GetPixel(x,y).ToArgb()
==
Color.Black.ToArgb())
191
{
192
a
=
temp.GetPixel(x,y).A;
193
re
=
temp.GetPixel(x,y).R;
194
gr
=
temp.GetPixel(x,y).G;
195
bl
=
temp.GetPixel(x,y).B;
196
197
x1
=
re;
198
y1
=
gr;
199
z1
=
bl;
200
201
if
(bl
+
25
<
255
)
202
bl
=
bl
+
25
;
203
if
(gr
+
25
<
255
)
204
gr
=
gr
+
25
;
205
if
(re
+
25
<
255
)
206
re
=
re
+
25
;
207
if
(x1
-
25
>
0
)
208
x1
=
x1
-
25
;
209
if
(y1
-
25
>
0
)
210
y1
=
y1
-
25
;
211
if
(z1
-
25
>
0
)
212
z1
=
z1
-
25
;
213
214
tempg.DrawEllipse(
new
Pen(
new
SolidBrush(Color.Black)),x,y
+
1
,
3
,
3
);
215
tempg.DrawEllipse(
new
Pen(
new
SolidBrush(Color.FromArgb(a,x1,y1,z1))),x,y,
1
,
1
);
216
}
217
}
218
}
219
MessageBox.Show(
"
输出文件是c:\\output.jpeg
"
,
"
给图像添加版权信息
"
);
220
tempg.Save();
221
originalimage.Save(
"
c:\\output.jpeg
"
,ImageFormat.Jpeg);
222
}
223
224
private
void
Form1_Load(
object
sender, System.EventArgs e)
225
{
226
227
}
228
}
229
}
230
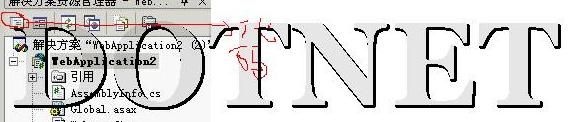