JSF+Spring+Hibernate做的login
源文件结构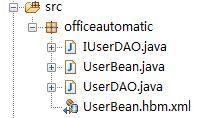
web文件结构
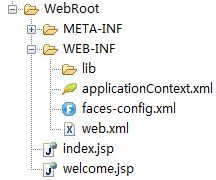
1 UserBean.java
1
package
officeautomatic;
2
3 import javax.faces.event.ActionEvent;
4
5 public class UserBean {
6 private Integer id;
7 private String name;
8 private String pass;
9 private String err;
10 private UserDAO userDAO;
11 private String outcome;
12
13 public void setId(Integer id) {
14 this .id = id;
15 }
16
17 public Integer getId() {
18 return id;
19 }
20
21 public void setName(String name) {
22 this .name = name;
23 }
24
25 public String getName() {
26 return name;
27 }
28
29 public void setPass(String pass) {
30 this .pass = pass;
31 }
32
33 public String getPass() {
34 return pass;
35 }
36
37 public void setErr(String err) {
38 this .err = err;
39 }
40
41 public String getErr() {
42 return err;
43 }
44
45 public void setUserDAO(UserDAO userDAO) {
46 this .userDAO = userDAO;
47 }
48
49 public UserDAO getUserDAO() {
50 return userDAO;
51 }
52
53 public void checkValue(ActionEvent e) {
54 if ((getUserDAO().find(id) != null ) && pass.equals(getUserDAO().find( new Integer(id)).getPass())){
55 setName(getUserDAO().find( new Integer(id)).getName());
56 outcome = " success " ;
57 }
58 else {
59 setErr( " error! " );
60 outcome = " failure " ;
61 }
62 }
63
64 public String outcome(){
65 return outcome;
66 }
67 }
checkValue方法里由id搜索,核对password的过程想改成单独的UserValidator类
2
3 import javax.faces.event.ActionEvent;
4
5 public class UserBean {
6 private Integer id;
7 private String name;
8 private String pass;
9 private String err;
10 private UserDAO userDAO;
11 private String outcome;
12
13 public void setId(Integer id) {
14 this .id = id;
15 }
16
17 public Integer getId() {
18 return id;
19 }
20
21 public void setName(String name) {
22 this .name = name;
23 }
24
25 public String getName() {
26 return name;
27 }
28
29 public void setPass(String pass) {
30 this .pass = pass;
31 }
32
33 public String getPass() {
34 return pass;
35 }
36
37 public void setErr(String err) {
38 this .err = err;
39 }
40
41 public String getErr() {
42 return err;
43 }
44
45 public void setUserDAO(UserDAO userDAO) {
46 this .userDAO = userDAO;
47 }
48
49 public UserDAO getUserDAO() {
50 return userDAO;
51 }
52
53 public void checkValue(ActionEvent e) {
54 if ((getUserDAO().find(id) != null ) && pass.equals(getUserDAO().find( new Integer(id)).getPass())){
55 setName(getUserDAO().find( new Integer(id)).getName());
56 outcome = " success " ;
57 }
58 else {
59 setErr( " error! " );
60 outcome = " failure " ;
61 }
62 }
63
64 public String outcome(){
65 return outcome;
66 }
67 }

2 IUserDAO.java
1
package
officeautomatic;
2
3 public interface IUserDAO {
4 public UserBean find(Integer id);
5 }
3
UserDAO.java2
3 public interface IUserDAO {
4 public UserBean find(Integer id);
5 }
1
package
officeautomatic;
2
3 import org.hibernate.Hibernate;
4 import org.hibernate.Session;
5 import org.hibernate.SessionFactory;
6
7 public class UserDAO implements IUserDAO {
8 private SessionFactory sessionFactory;
9
10 public UserDAO() {
11
12 }
13
14 public UserDAO(SessionFactory sessionFactory) {
15 this .setSessionFactory(sessionFactory);
16 }
17
18 public void setSessionFactory(SessionFactory sessionFactory) {
19 this .sessionFactory = sessionFactory;
20 }
21
22 public UserBean find(Integer id) {
23 Session session = sessionFactory.openSession();
24
25 UserBean userBean = (UserBean) session.get(UserBean. class , id);
26 Hibernate.initialize(userBean);
27
28 session.close();
29
30 return userBean;
31 }
32 }
4
index.jsp
2
3 import org.hibernate.Hibernate;
4 import org.hibernate.Session;
5 import org.hibernate.SessionFactory;
6
7 public class UserDAO implements IUserDAO {
8 private SessionFactory sessionFactory;
9
10 public UserDAO() {
11
12 }
13
14 public UserDAO(SessionFactory sessionFactory) {
15 this .setSessionFactory(sessionFactory);
16 }
17
18 public void setSessionFactory(SessionFactory sessionFactory) {
19 this .sessionFactory = sessionFactory;
20 }
21
22 public UserBean find(Integer id) {
23 Session session = sessionFactory.openSession();
24
25 UserBean userBean = (UserBean) session.get(UserBean. class , id);
26 Hibernate.initialize(userBean);
27
28 session.close();
29
30 return userBean;
31 }
32 }
1
<%
@ page language
=
"
java
"
contentType
=
"
text.html; charset=BIG5
"
2 pageEncoding = "BIG5 " %>
3 <% @taglib uri = " http://java.sun.com/jsf/core " prefix = " f " %>
4 <% @taglib uri = " http://java.sun.com/jsf/html " prefix = " h " %>
5
6 <! DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" >
7 < html >
8 < head >
9 < meta http-equiv ="Content-Type" content ="text/html; charset=BIG5" >
10 < title > Login </ title >
11 </ head >
12
13 < body >
14 < f:view locale ="zh_CN" >
15 < h:form id ="loginForm" >
16 < h:message for ="userNameInput" ></ h:message >
17 < h:outputText value ="#{userBean.err}" ></ h:outputText >
18 < p >
19 < h:outputLabel for ="userNameInput" >
20 < h:outputText id ="userNameLabel" value ="User ID:" ></ h:outputText >
21 </ h:outputLabel >
22 < h:inputText id ="userNameInput" value ="#{userBean.id}" required ="true" ></ h:inputText >
23 </ p >
24 < p >
25 < h:outputLabel for ="passwordInput" >
26 < h:outputText id ="passwordLabel" value ="Password:" ></ h:outputText >
27 </ h:outputLabel >
28 < h:inputSecret id ="passwordInput" value ="#{userBean.pass}" required ="true" ></ h:inputSecret >
29 </ p >
30 < h:commandButton id ="submitButton" value ="Submmit" actionListener ="#{userBean.checkValue}" action ="#{userBean.outcome}" ></ h:commandButton >
31 </ h:form >
32 </ f:view >
33 </ body >
34 </ html >
actionListener作为JSF的监听器,返回void。
2 pageEncoding = "BIG5 " %>
3 <% @taglib uri = " http://java.sun.com/jsf/core " prefix = " f " %>
4 <% @taglib uri = " http://java.sun.com/jsf/html " prefix = " h " %>
5
6 <! DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" >
7 < html >
8 < head >
9 < meta http-equiv ="Content-Type" content ="text/html; charset=BIG5" >
10 < title > Login </ title >
11 </ head >
12
13 < body >
14 < f:view locale ="zh_CN" >
15 < h:form id ="loginForm" >
16 < h:message for ="userNameInput" ></ h:message >
17 < h:outputText value ="#{userBean.err}" ></ h:outputText >
18 < p >
19 < h:outputLabel for ="userNameInput" >
20 < h:outputText id ="userNameLabel" value ="User ID:" ></ h:outputText >
21 </ h:outputLabel >
22 < h:inputText id ="userNameInput" value ="#{userBean.id}" required ="true" ></ h:inputText >
23 </ p >
24 < p >
25 < h:outputLabel for ="passwordInput" >
26 < h:outputText id ="passwordLabel" value ="Password:" ></ h:outputText >
27 </ h:outputLabel >
28 < h:inputSecret id ="passwordInput" value ="#{userBean.pass}" required ="true" ></ h:inputSecret >
29 </ p >
30 < h:commandButton id ="submitButton" value ="Submmit" actionListener ="#{userBean.checkValue}" action ="#{userBean.outcome}" ></ h:commandButton >
31 </ h:form >
32 </ f:view >
33 </ body >
34 </ html >
5 welcome.jsp
1
<%
@ page language
=
"
java
"
contentType
=
"
text.html; charset=BIG5
"
2 pageEncoding = " BIG5 " %>
3 <% @taglib uri = " http://java.sun.com/jsf/core " prefix = " f " %>
4 <% @taglib uri = " http://java.sun.com/jsf/html " prefix = " h " %>
5
6 <! DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" >
7 < html >
8 < head >
9 < meta http-equiv ="Content-Type" content ="text/html; charset=BIG5" >
10 < title > welcome </ title >
11 </ head >
12
13 < body >
14 < f:view >
15 < h:outputText value ="#{userBean.name}" />
16 < h3 > login successfully </ h3 >
17 </ f:view >
18 </ body >
19 </ html >
6
faces-config.xml
2 pageEncoding = " BIG5 " %>
3 <% @taglib uri = " http://java.sun.com/jsf/core " prefix = " f " %>
4 <% @taglib uri = " http://java.sun.com/jsf/html " prefix = " h " %>
5
6 <! DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" >
7 < html >
8 < head >
9 < meta http-equiv ="Content-Type" content ="text/html; charset=BIG5" >
10 < title > welcome </ title >
11 </ head >
12
13 < body >
14 < f:view >
15 < h:outputText value ="#{userBean.name}" />
16 < h3 > login successfully </ h3 >
17 </ f:view >
18 </ body >
19 </ html >
1
<?
xml version="1.0" encoding="UTF-8"
?>
2 <! DOCTYPE faces-config PUBLIC
3 "-//Sun Microsystems, Inc.//DTD JavaServer Faces Config 1.0//EN"
4 "http://java.sun.com/dtd/web-facesconfig_1_0.dtd" >
5
6 < faces-config >
7 < application >
8 < variable-resolver >
9 org.springframework.web.jsf.DelegatingVariableResolver
10 </ variable-resolver >
11 </ application >
12 < navigation-rule >
13 < from-view-id > /index.jsp </ from-view-id >
14 < navigation-case >
15 < from-outcome > success </ from-outcome >
16 < to-view-id > /welcome.jsp </ to-view-id >
17 </ navigation-case >
18 < navigation-case >
19 < from-outcome > failure </ from-outcome >
20 < to-view-id > /index.jsp </ to-view-id >
21 </ navigation-case >
22
23 </ navigation-rule >
24
25 < managed-bean >
26 < managed-bean-name > userBean </ managed-bean-name >
27 < managed-bean-class > officeautomatic.UserBean </ managed-bean-class >
28 < managed-bean-scope > session </ managed-bean-scope >
29 < managed-property >
30 < property-name > userDAO </ property-name >
31 < value > #{userDAO} </ value >
32 </ managed-property >
33
34 </ managed-bean >
35
36 </ faces-config >
2 <! DOCTYPE faces-config PUBLIC
3 "-//Sun Microsystems, Inc.//DTD JavaServer Faces Config 1.0//EN"
4 "http://java.sun.com/dtd/web-facesconfig_1_0.dtd" >
5
6 < faces-config >
7 < application >
8 < variable-resolver >
9 org.springframework.web.jsf.DelegatingVariableResolver
10 </ variable-resolver >
11 </ application >
12 < navigation-rule >
13 < from-view-id > /index.jsp </ from-view-id >
14 < navigation-case >
15 < from-outcome > success </ from-outcome >
16 < to-view-id > /welcome.jsp </ to-view-id >
17 </ navigation-case >
18 < navigation-case >
19 < from-outcome > failure </ from-outcome >
20 < to-view-id > /index.jsp </ to-view-id >
21 </ navigation-case >
22
23 </ navigation-rule >
24
25 < managed-bean >
26 < managed-bean-name > userBean </ managed-bean-name >
27 < managed-bean-class > officeautomatic.UserBean </ managed-bean-class >
28 < managed-bean-scope > session </ managed-bean-scope >
29 < managed-property >
30 < property-name > userDAO </ property-name >
31 < value > #{userDAO} </ value >
32 </ managed-property >
33
34 </ managed-bean >
35
36 </ faces-config >
7 beans-config.xml
1
<?
xml version="1.0" encoding="UTF-8"
?>
2 < beans xmlns ="http://www.springframework.org/schema/beans"
3 xmlns:xsi ="http://www.w3.org/2001/XMLSchema-instance"
4 xsi:schemaLocation ="http://www.springframework.org/schema/beans
5 http://www.springframework.org/schema/beans/spring-beans-2.0.xsd" >
6
7 < bean id ="dataSource" class ="org.springframework.jdbc.datasource.DriverManagerDataSource" >
8 < property name ="driverClassName" value ="com.mysql.jdbc.Driver" />
9 < property name ="url" value ="jdbc:mysql://localhost:3306/cie" />
10 < property name ="username" value ="root" />
11 < property name ="password" value ="123456" />
12 </ bean >
13
14 < bean id ="sessionFactory"
15 class ="org.springframework.orm.hibernate3.LocalSessionFactoryBean"
16 destroy-method ="close" >
17 < property name ="dataSource" ref ="dataSource" />
18 < property name ="mappingResources" >
19 < list >
20 < value >officeautomatic/ UserBean.hbm.xml </ value >
21 </ list >
22 </ property >
23 < property name ="hibernateProperties" >
24 < props >
25 < prop key ="hibernate.dialect" >
26 org.hibernate.dialect.MySQLDialect
27 </ prop >
28 </ props >
29 </ property >
30 </ bean >
31
32 < bean id ="userDAO" class ="officeautomatic.UserDAO" >
33 < property name ="sessionFactory" ref ="sessionFactory" ></ property >
34 </ bean >
35
36 </ beans >
2 < beans xmlns ="http://www.springframework.org/schema/beans"
3 xmlns:xsi ="http://www.w3.org/2001/XMLSchema-instance"
4 xsi:schemaLocation ="http://www.springframework.org/schema/beans
5 http://www.springframework.org/schema/beans/spring-beans-2.0.xsd" >
6
7 < bean id ="dataSource" class ="org.springframework.jdbc.datasource.DriverManagerDataSource" >
8 < property name ="driverClassName" value ="com.mysql.jdbc.Driver" />
9 < property name ="url" value ="jdbc:mysql://localhost:3306/cie" />
10 < property name ="username" value ="root" />
11 < property name ="password" value ="123456" />
12 </ bean >
13
14 < bean id ="sessionFactory"
15 class ="org.springframework.orm.hibernate3.LocalSessionFactoryBean"
16 destroy-method ="close" >
17 < property name ="dataSource" ref ="dataSource" />
18 < property name ="mappingResources" >
19 < list >
20 < value >officeautomatic/ UserBean.hbm.xml </ value >
21 </ list >
22 </ property >
23 < property name ="hibernateProperties" >
24 < props >
25 < prop key ="hibernate.dialect" >
26 org.hibernate.dialect.MySQLDialect
27 </ prop >
28 </ props >
29 </ property >
30 </ bean >
31
32 < bean id ="userDAO" class ="officeautomatic.UserDAO" >
33 < property name ="sessionFactory" ref ="sessionFactory" ></ property >
34 </ bean >
35
36 </ beans >
cie:数据库名。
sessionFactory的value要带包名,officeautomatic/UserBean.hbm.xml这错误查了好久,唉……
8 UserBean.hbm.xml
1
<?
xml version="1.0" encoding="UTF-8"
?>
2 <! DOCTYPE hibernate-mapping
3 PUBLIC "-//Hibernate/Hibernate Mapping DTD 3.0//EN"
4 "http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd" >
5
6 < hibernate-mapping >
7 < class name ="officeautomatic.UserBean" table ="user" >
8
9 < id name ="id" column ="id" >
10 < generator class ="native" ></ generator >
11 </ id >
12
13 < property name ="name" column ="username" ></ property >
14
15 < property name ="pass" column ="password" ></ property >
16
17 </ class >
18 </ hibernate-mapping >
UserBean类与数据库中user表格对应。
2 <! DOCTYPE hibernate-mapping
3 PUBLIC "-//Hibernate/Hibernate Mapping DTD 3.0//EN"
4 "http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd" >
5
6 < hibernate-mapping >
7 < class name ="officeautomatic.UserBean" table ="user" >
8
9 < id name ="id" column ="id" >
10 < generator class ="native" ></ generator >
11 </ id >
12
13 < property name ="name" column ="username" ></ property >
14
15 < property name ="pass" column ="password" ></ property >
16
17 </ class >
18 </ hibernate-mapping >
9 web.xml
1
<?
xml version="1.0" encoding="UTF-8"
?>
2 < web-app xmlns ="http://java.sun.com/xml/ns/javaee"
3 xmlns:xsi ="http://www.w3.org/2001/XMLSchema-instance" version ="2.5"
4 xsi:schemaLocation ="http://java.sun.com/xml/ns/javaee
5 http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" >
6
7 < session-config >
8 < session-timeout > 30 </ session-timeout >
9 </ session-config >
10
11 < servlet >
12 < servlet-name > context </ servlet-name >
13 < servlet-class >
14 org.springframework.web.context.ContextLoaderServlet
15 </ servlet-class >
16 < load-on-startup > 1 </ load-on-startup >
17 </ servlet >
18 < listener >
19 < listener-class >
20 org.apache.myfaces.webapp.StartupServletContextListener
21 </ listener-class >
22 </ listener >
23 < context-param >
24 < param-name > contextConfigLocation </ param-name >
25 < param-value > /WEB-INF/applicationContext.xml </ param-value >
26 </ context-param >
27 < context-param >
28 < param-name > javax.faces.CONFIG_FILES </ param-name >
29 < param-value > /WEB-INF/faces-config.xml </ param-value >
30 </ context-param >
31
32 < servlet >
33 < servlet-name > Faces Servlet </ servlet-name >
34 < servlet-class > javax.faces.webapp.FacesServlet </ servlet-class >
35 < load-on-startup > 0 </ load-on-startup >
36 </ servlet >
37 < servlet-mapping >
38 < servlet-name > Faces Servlet </ servlet-name >
39 < url-pattern > *.faces </ url-pattern >
40 </ servlet-mapping >
41
42 </ web-app >
43
用了myface的listener,要加个myface的包。
2 < web-app xmlns ="http://java.sun.com/xml/ns/javaee"
3 xmlns:xsi ="http://www.w3.org/2001/XMLSchema-instance" version ="2.5"
4 xsi:schemaLocation ="http://java.sun.com/xml/ns/javaee
5 http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" >
6
7 < session-config >
8 < session-timeout > 30 </ session-timeout >
9 </ session-config >
10
11 < servlet >
12 < servlet-name > context </ servlet-name >
13 < servlet-class >
14 org.springframework.web.context.ContextLoaderServlet
15 </ servlet-class >
16 < load-on-startup > 1 </ load-on-startup >
17 </ servlet >
18 < listener >
19 < listener-class >
20 org.apache.myfaces.webapp.StartupServletContextListener
21 </ listener-class >
22 </ listener >
23 < context-param >
24 < param-name > contextConfigLocation </ param-name >
25 < param-value > /WEB-INF/applicationContext.xml </ param-value >
26 </ context-param >
27 < context-param >
28 < param-name > javax.faces.CONFIG_FILES </ param-name >
29 < param-value > /WEB-INF/faces-config.xml </ param-value >
30 </ context-param >
31
32 < servlet >
33 < servlet-name > Faces Servlet </ servlet-name >
34 < servlet-class > javax.faces.webapp.FacesServlet </ servlet-class >
35 < load-on-startup > 0 </ load-on-startup >
36 </ servlet >
37 < servlet-mapping >
38 < servlet-name > Faces Servlet </ servlet-name >
39 < url-pattern > *.faces </ url-pattern >
40 </ servlet-mapping >
41
42 </ web-app >
43