关于Visual C++2010编译器的问题
今天使用
visual C++ 2010
编译器编写
程序逆波兰表达式求值
但是遭遇了以前从未看到的问题,在此 求助各位,谢谢
头 文件Stack.h
1
template
<
class
T
>
2
class
Stack
3
{
4
public:
5
Stack(int size=0);//构造大小为size的堆栈
6
~Stack()
{delete p;}//撤销堆栈
7
bool IsFull();//判断堆栈是否已满
8
bool IsEmpty();//判断堆栈是否为空
9
void Push(T &x);//将x压入堆栈
10
T Pop(T &x);//将栈顶元素弹出用x保存
11
private:
12
int Size;//大小
13
int top;//栈顶
14
T *p;//数组指针
15
}
;
实现源文件Stack.cpp

2

3



4

5

6



7

8

9

10

11

12

13

14

15

1
#include
"
Stack.h
"
2
3
template
<
class
T
>
4
void
Stack
<
T
>
::Push(T
&
x)
5
{
6
p[top++]=x;//将x保存于栈顶,栈顶+1
7
}
8
9
template
<
class
T
>
10
T Stack
<
T
>
::Pop(T
&
x)
11
{
12
x=p[--top];//x保存栈顶元素,栈顶-1
13
return x;
14
}
15
16
template
<
class
T
>
17
Stack
<
T
>
::Stack(
int
size)
18
{
19
Size=size;//栈大小为size
20
top=0;//栈顶top指向0
21
p=new T[Size];//为p开辟Size大小的空间
22
}
23
24
template
<
class
T
>
25
bool
Stack
<
T
>
::IsFull()
26
{
27
return top==Size;
28
}
//
判断堆栈是否已满
29
30
template
<
class
T
>
31
bool
Stack
<
T
>
::IsEmpty()
32
{
33
return top==0;
34
}
//
判断堆栈是否为空

2

3

4

5



6

7

8

9

10

11



12

13

14

15

16

17

18



19

20

21

22

23

24

25

26



27

28

29

30

31

32



33

34

main.cpp
1
#include
<
iostream
>
2
#include
<
stdio.h
>
3
#include
"
Stack.h
"
4
const
int
MaxSize
=
40
;
//
由于定义数组时开辟空间大小
5
using
namespace
std;
6
7
/**/
/*
8
*逆波兰表达式求值函数
9
*/
10
float
LiBolan(
char
*
array)
{//array存储表达式
11
Stack<float> sta(MaxSize);//建立大小为Maxsize的堆栈
12
int i=0;
13
if(array[0]=='.')
{
14
cout<<"表达式错误:小数表示错误"<<endl;
15
return 0;
16
}
17
while(array[i]!='\0')
{//当表达式没有结束
18
if(array[i]=='.')
{//数字第一个字符为小数点或运算符后是小数点
19
cout<<"表达式错误:小数表示错误"<<endl;
20
return 0;
21
}
22
if(i==0&&array[i]=='-')
{//判断第一个是否负数,后面的没有必要判断,如果是
23
i++;//处理下一个字符
24
float tem=0;
25
while(array[i]>='0'&&array[i]<='9')
{
26
tem=(float)array[i]-48+tem*10;//先计算该负数的绝对值
27
i++;
28
}
29
if(array[i]=='.')
{//若存在小数
30
i++;//忽略该位
31
if(!(array[i]>='0'&&array[i]<='9'))
{//小数点后不是数字
32
cout<<"表达式错误:小数表示错误"<<endl;
33
return 0;
34
}
35
}
36
Stack<char> s(7);//用于存储小数字符
37
while(array[i]>='0'&&array[i]<='9')
{
38
s.Push(array[i]);
39
i++;
40
}
41
float item=0;
42
char x;
43
while(!s.IsEmpty())
44
item=((float)s.Pop(x)-48)*0.1+item*0.1;//计算小数
45
tem+=item;
46
tem=-tem;//取相反数得到原数
47
sta.Push(tem);//将该数压入栈中
48
}
49
if(array[i]>='0'&&array[i]<='9')
{//其他时候,判断是否为数字
50
float tem=0;
51
while(array[i]>='0'&&array[i]<='9')
{//计算整数部分
52
tem=(float)array[i]-48+tem*10;
53
i++;
54
}
55
if(array[i]=='.')
{//若存在小数
56
i++;//忽略该位
57
if(!(array[i]>='0'&&array[i]<='9'))
{//小数点后不是数字
58
cout<<"表达式错误:小数表示错误"<<endl;
59
return 0;
60
}
61
}
62
Stack<char> s(7);//用于存储小数字符
63
while(array[i]>='0'&&array[i]<='9')
{
64
s.Push(array[i]);
65
i++;
66
}
67
float item=0;
68
char x;
69
while(!s.IsEmpty())
70
item=((float)s.Pop(x)-48)*0.1+item*0.1;//计算小数
71
tem+=item;
72
sta.Push(tem);
73
}
74
if(array[i]=='+'||array[i]=='-'||array[i]=='*'||array[i]=='/')
{
75
float it1,it21,it22;//it21栈顶元素,it22倒数第二个,it1是it21,it22对应运算的值
76
char ch=array[i];
77
switch(ch)
{
78
case '+':it21=sta.Pop(it21);
79
if(sta.IsEmpty())
{
80
cout<<"表达是错误:运算符比对应所需的运算数多"<<endl;//弹出一个运算数后为空
81
return 0;
82
}
83
it22=sta.Pop(it22);
84
it1=it21+it22;
85
sta.Push(it1);break;
86
case '-':it21=sta.Pop(it21);
87
if(sta.IsEmpty())
{
88
cout<<"表达式错误:运算符比对应所需的运算数多"<<endl;
89
return 0;
90
}
91
it22=sta.Pop(it22);
92
it1=it22-it21;
93
sta.Push(it1);break;
94
case '*':it21=sta.Pop(it21);
95
if(sta.IsEmpty())
{
96
cout<<"表达式错误:运算符比对应所需的运算数多"<<endl;
97
return 0;
98
}
99
it22=sta.Pop(it22);
100
it1=it21*it22;
101
sta.Push(it1);break;
102
case '/':it21=sta.Pop(it21);
103
if(sta.IsEmpty())
{
104
cout<<"表达式错误:运算符比对应所需的运算数多"<<endl;
105
return 0;
106
}
107
it22=sta.Pop(it22);
108
it1=it22/it21;
109
sta.Push(it1);break;
110
default:break;
111
}
112
i++;
113
}
114
else
115
i++;
116
}
117
float value;
118
sta.Pop(value);
119
if(!sta.IsEmpty())
{
120
cout<<"表达式错误:运算数多于所需的运算符"<<endl;//最后栈不为空
121
return 0;
122
}
123
return value;
124
}
125
void
main()
{
126
printf("请输入一个后缀表达式:");
127
char str[MaxSize];
128
gets(str);
129
float value=LiBolan(str);
130
printf("%2.2f\n",value);
131
}

2

3

4

5

6

7


8

9

10



11

12

13



14

15

16

17



18



19

20

21

22



23

24

25



26

27

28

29



30

31



32

33

34

35

36

37



38

39

40

41

42

43

44

45

46

47

48

49



50

51



52

53

54

55



56

57



58

59

60

61

62

63



64

65

66

67

68

69

70

71

72

73

74



75

76

77



78

79



80

81

82

83

84

85

86

87



88

89

90

91

92

93

94

95



96

97

98

99

100

101

102

103



104

105

106

107

108

109

110

111

112

113

114

115

116

117

118

119



120

121

122

123

124

125



126

127

128

129

130

131

当用Visual C++ 6.0编译时正确,用visualC++201编译时,如果不分头文件源文件,即直接把这三个文件放在man.cpp中编译正确,当时当把他们分开后
其文件结构图如下时编译通不过
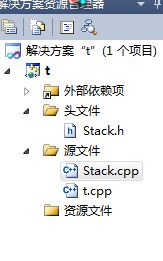
其问题描述如下
1>t.obj : error LNK2019: 无法解析的外部符号 "public: bool __thiscall Stack<float>::IsEmpty(void)" ( ?IsEmpty@?$Stack@M@@QAE_NXZ),该符号在函数 "float __cdecl LiBolan(char *)" ( ?LiBolan@@YAMPAD@Z) 中被引用
1>t.obj : error LNK2019: 无法解析的外部符号 "public: float __thiscall Stack<float>::Pop(float &)" ( ?Pop@?$Stack@M@@QAEMAAM@Z),该符号在函数 "float __cdecl LiBolan(char *)" ( ?LiBolan@@YAMPAD@Z) 中被引用
1>t.obj : error LNK2019: 无法解析的外部符号 "public: void __thiscall Stack<float>::Push(float &)" ( ?Push@?$Stack@M@@QAEXAAM@Z),该符号在函数 "float __cdecl LiBolan(char *)" ( ?LiBolan@@YAMPAD@Z) 中被引用
1>t.obj : error LNK2019: 无法解析的外部符号 "public: char __thiscall Stack<char>::Pop(char &)" ( ?Pop@?$Stack@D@@QAEDAAD@Z),该符号在函数 "float __cdecl LiBolan(char *)" ( ?LiBolan@@YAMPAD@Z) 中被引用
1>t.obj : error LNK2019: 无法解析的外部符号 "public: bool __thiscall Stack<char>::IsEmpty(void)" ( ?IsEmpty@?$Stack@D@@QAE_NXZ),该符号在函数 "float __cdecl LiBolan(char *)" ( ?LiBolan@@YAMPAD@Z) 中被引用
1>t.obj : error LNK2019: 无法解析的外部符号 "public: void __thiscall Stack<char>::Push(char &)" ( ?Push@?$Stack@D@@QAEXAAD@Z),该符号在函数 "float __cdecl LiBolan(char *)" ( ?LiBolan@@YAMPAD@Z) 中被引用
1>t.obj : error LNK2019: 无法解析的外部符号 "public: __thiscall Stack<char>::Stack<char>(int)" ( ??0?$Stack@D@@QAE@H@Z),该符号在函数 "float __cdecl LiBolan(char *)" ( ?LiBolan@@YAMPAD@Z) 中被引用
1>t.obj : error LNK2019: 无法解析的外部符号 "public: __thiscall Stack<float>::Stack<float>(int)" ( ??0?$Stack@M@@QAE@H@Z),该符号在函数 "float __cdecl LiBolan(char *)" ( ?LiBolan@@YAMPAD@Z) 中被引用
1>E:\Visual2010\t\Debug\t.exe : fatal error LNK1120: 8 个无法解析的外部命令
1>
1>生成失败。
1>
1>已用时间 00:00:00.79
========== 生成: 成功 0 个,失败 1 个,最新 0 个,跳过 0 个 ==========