13.3 利用BIRT设计引擎API生成报表
前面我们在讲解BIRT报表工作模式的时候实现过一个简单的利用BIRT设计引擎API生成报表,在这一节我们详细讲解实现过程。
BIRT报表的设计引擎API是BIRT报表的核心,不论是任何形式的BIRT设计器(eclipse插件,osgi独立,standalone)还是任何形式的BIRT展示器(web viewer,用户自定义servlet viewer,rcp)都必须包含BIRT设计引擎。
BIRT design API包含如下包和类:
- Packages
- org.eclipse.birt.report.model.api
- org.eclipse.birt.report.model.api.activity
- org.eclipse.birt.report.model.api.command
- org.eclipse.birt.report.model.api.core
- org.eclipse.birt.report.model.api.css
- org.eclipse.birt.report.model.api.elements
- org.eclipse.birt.report.model.api.elements.structures
- org.eclipse.birt.report.model.api.elements.table
- org.eclipse.birt.report.model.api.extension
- org.eclipse.birt.report.model.api.impl
- org.eclipse.birt.report.model.api.metadata
- org.eclipse.birt.report.model.api.olap
- org.eclipse.birt.report.model.api.scripts
- org.eclipse.birt.report.model.api.simpleapi
- org.eclipse.birt.report.model.api.util
- org.eclipse.birt.report.model.api.validators
用户在用java设计BIRT报表的时候都会或多或少的用到其中的4到5个包;
我们先看一个简单的例子:
- package birt;
-
- import java.io.IOException;
- import java.util.ArrayList;
- import java.util.List;
-
- import org.eclipse.birt.core.framework.Platform;
- import org.eclipse.birt.report.model.api.CellHandle;
- import org.eclipse.birt.report.model.api.DataItemHandle;
- import org.eclipse.birt.report.model.api.DesignConfig;
- import org.eclipse.birt.report.model.api.ElementFactory;
- import org.eclipse.birt.report.model.api.IDesignEngine;
- import org.eclipse.birt.report.model.api.IDesignEngineFactory;
- import org.eclipse.birt.report.model.api.LabelHandle;
- import org.eclipse.birt.report.model.api.OdaDataSetHandle;
- import org.eclipse.birt.report.model.api.OdaDataSourceHandle;
- import org.eclipse.birt.report.model.api.PropertyHandle;
- import org.eclipse.birt.report.model.api.ReportDesignHandle;
- import org.eclipse.birt.report.model.api.RowHandle;
- import org.eclipse.birt.report.model.api.SessionHandle;
- import org.eclipse.birt.report.model.api.StructureFactory;
- import org.eclipse.birt.report.model.api.TableHandle;
- import org.eclipse.birt.report.model.api.activity.SemanticException;
- import org.eclipse.birt.report.model.api.elements.structures.ComputedColumn;
-
- import com.ibm.icu.util.ULocale;
-
-
-
-
-
- public class DECreateDynamicTable {
-
- ReportDesignHandle designHandle = null;
- ElementFactory designFactory = null;
- StructureFactory structFactory = null;
-
- public static void main( String[] args )
- {
- try
- {
- DECreateDynamicTable de = new DECreateDynamicTable();
- List al = new ArrayList();
- al.add("OFFICECODE");
- al.add("CITY");
- al.add("COUNTRY");
- de.buildReport(al, "From Offices" );
- }
- catch( IOException e )
- {
- e.printStackTrace();
- }
- catch( SemanticException e )
- {
- e.printStackTrace();
- }
- }
-
- void buildDataSource( ) throws SemanticException
- {
- OdaDataSourceHandle dsHandle = designFactory.newOdaDataSource( "Data Source", "org.eclipse.birt.report.data.oda.jdbc" );
- dsHandle.setProperty( "odaDriverClass","org.eclipse.birt.report.data.oda.sampledb.Driver" );
- dsHandle.setProperty( "odaURL", "jdbc:classicmodels:sampledb" );
- dsHandle.setProperty( "odaUser", "ClassicModels" );
- dsHandle.setProperty( "odaPassword", "" );
-
- designHandle.getDataSources( ).add( dsHandle );
- }
-
- void buildDataSet(List al, String fromClause ) throws SemanticException
- {
- OdaDataSetHandle dsHandle = designFactory.newOdaDataSet( "ds","org.eclipse.birt.report.data.oda.jdbc.JdbcSelectDataSet" );
- dsHandle.setDataSource( "Data Source" );
- String qry = "Select ";
- for( int i=0; i < al.size(); i++)
- {
- qry += " " + al.get(i);
- if( i != (al.size() -1) )
- {
- qry += ",";
- }
- }
- qry += " " + fromClause;
- dsHandle.setQueryText( qry );
-
- designHandle.getDataSets( ).add( dsHandle );
- }
-
- void buildReport(List al, String fromClause ) throws IOException, SemanticException
- {
-
- DesignConfig config = new DesignConfig( );
-
- config.setProperty("BIRT_HOME", "E:\\birt汉化包\\birt-runtime-3_7_2\\ReportEngine");
- IDesignEngine engine = null;
- try
- {
- Platform.startup( config );
- IDesignEngineFactory factory = (IDesignEngineFactory) Platform.createFactoryObject( IDesignEngineFactory.EXTENSION_DESIGN_ENGINE_FACTORY );
- engine = factory.createDesignEngine( config );
- }
- catch( Exception ex )
- {
- ex.printStackTrace();
- }
- SessionHandle session = engine.newSessionHandle( ULocale.ENGLISH ) ;
- try
- {
-
- designHandle = session.openDesign("d:\\sample.rptdesign");
-
- designFactory = designHandle.getElementFactory( );
-
- buildDataSource();
- buildDataSet(al, fromClause);
-
- TableHandle table = designFactory.newTableItem( "table", al.size() );
- table.setWidth( "100%" );
- table.setDataSet( designHandle.findDataSet( "ds" ) );
- PropertyHandle computedSet = table.getColumnBindings( );
- ComputedColumn cs1 = null;
- for( int i=0; i < al.size(); i++)
- {
- cs1 = StructureFactory.createComputedColumn();
- cs1.setName((String)al.get(i));
- cs1.setExpression("dataSetRow[\"" + (String)al.get(i) + "\"]");
- computedSet.addItem(cs1);
- }
-
- RowHandle tableheader = (RowHandle) table.getHeader( ).get( 0 );
- for( int i=0; i < al.size(); i++ )
- {
- LabelHandle label1 = designFactory.newLabel( (String)al.get(i) );
- label1.setText((String)al.get(i));
- CellHandle cell = (CellHandle) tableheader.getCells( ).get( i );
- cell.getContent( ).add( label1 );
- }
-
-
- RowHandle tabledetail = (RowHandle) table.getDetail( ).get( 0 );
- for( int i=0; i < al.size(); i++ )
- {
- CellHandle cell = (CellHandle) tabledetail.getCells( ).get( i );
- DataItemHandle data = designFactory.newDataItem( "data_"+(String)al.get(i) );
- data.setResultSetColumn( (String)al.get(i));
- cell.getContent( ).add( data );
- }
-
- designHandle.getBody( ).add( table );
-
-
- designHandle.saveAs( "d:\\sample3.rptdesign" );
- designHandle.close( );
- System.out.println("Finished");
- }
- catch (Exception e)
- {
- e.printStackTrace();
- }
- }
- }
这个例子一共有四个函数 :
1 . Main 函数: 这个例子简单之处在与它可以直接的运行,只要你修改了
config.setProperty("BIRT_HOME", "E:\\birt汉化包\\birt-runtime-3_7_2\\ReportEngine"); 指向你自己的Birt Runtime 解压后的ReportEngine 目录.
designHandle = session.openDesign("d:\\sample.rptdesign"); 你可以从Birt 里面建立一个新的Report template.然后指向这个report 就可以了
designHandle.saveAs( "d:\\sample3.rptdesign" ); //$NON-NLS-1$ 指定一个你想保存的位置,c:/temp 目录存在你才能够保存到c:/temp 目录下.
2 . buildDataSource 函数把一个ReportDesignHandle 的 Data Source 初始化, setProperties 左边的String 是不能变的,Data Source 的名字可以随便取,取DataSet 的时候要根据这个名字来取.
3 . buildDataSet 通过拼sql 的方式 ,来build DataSet, 注意sql 别拼错了.
4 . buildReport 注意element 的初始化顺序.在所有的DataItem 外面都是一层Cell,Cell 外面才是row .这个例子使用的row 来拼成table 的,也可以用column 来拼,相对应的数据处理也是一个column 一个 column 的处理的了
这个程序打开了一个名为sample的BIRT设计文件,往其中插入了一个1行3列的网格,列名分别为OFFICECODE,CITY,COUNTRY,没有做任何美化,另存为sample3.rptdesign
我们看看改变,sample.rptdesign预览如下:
sample3.rptdesign预览如下:
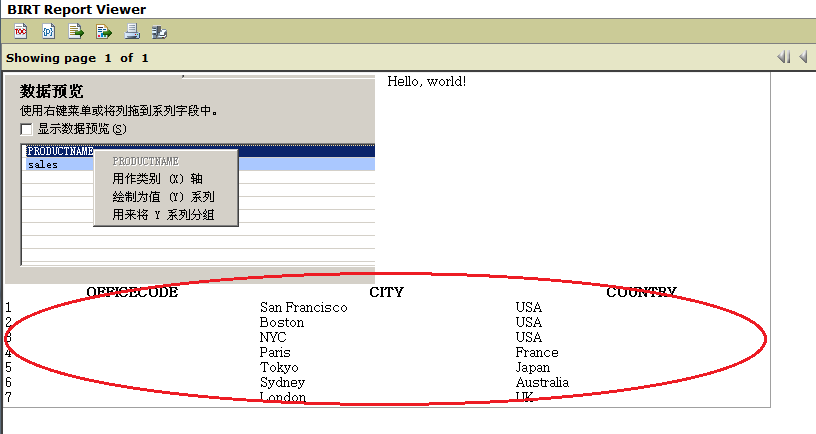
这种修改不仅能表现在设计阶段,我们在调用报表引擎进行展示的时候同样可以修改,源码如下:
- package birt;
-
- import java.util.ArrayList;
- import java.util.HashMap;
- import java.util.logging.Level;
-
- import org.eclipse.birt.core.framework.Platform;
- import org.eclipse.birt.report.engine.api.EngineConfig;
- import org.eclipse.birt.report.engine.api.EngineConstants;
- import org.eclipse.birt.report.engine.api.EngineException;
- import org.eclipse.birt.report.engine.api.HTMLActionHandler;
- import org.eclipse.birt.report.engine.api.HTMLEmitterConfig;
- import org.eclipse.birt.report.engine.api.HTMLRenderContext;
- import org.eclipse.birt.report.engine.api.HTMLRenderOption;
- import org.eclipse.birt.report.engine.api.HTMLServerImageHandler;
- import org.eclipse.birt.report.engine.api.IReportEngine;
- import org.eclipse.birt.report.engine.api.IReportEngineFactory;
- import org.eclipse.birt.report.engine.api.IReportRunnable;
- import org.eclipse.birt.report.engine.api.IRunAndRenderTask;
- import org.eclipse.birt.report.model.api.CellHandle;
- import org.eclipse.birt.report.model.api.DataItemHandle;
- import org.eclipse.birt.report.model.api.ElementFactory;
- import org.eclipse.birt.report.model.api.LabelHandle;
- import org.eclipse.birt.report.model.api.OdaDataSetHandle;
- import org.eclipse.birt.report.model.api.OdaDataSourceHandle;
- import org.eclipse.birt.report.model.api.PropertyHandle;
- import org.eclipse.birt.report.model.api.ReportDesignHandle;
- import org.eclipse.birt.report.model.api.RowHandle;
- import org.eclipse.birt.report.model.api.StructureFactory;
- import org.eclipse.birt.report.model.api.TableHandle;
- import org.eclipse.birt.report.model.api.activity.SemanticException;
- import org.eclipse.birt.report.model.api.elements.structures.ComputedColumn;
-
- public class ExecuteModifedReport {
-
- public void runReport() throws EngineException
- {
- IReportEngine engine=null;
- EngineConfig config = null;
-
- try
- {
-
- config = new EngineConfig( );
- config.setEngineHome( "E:\\birt汉化包\\birt-runtime-3_7_2\\ReportEngine" );
- config.setLogConfig(null, Level.FINE);
-
- Platform.startup( config );
- IReportEngineFactory factory = (IReportEngineFactory) Platform.createFactoryObject( IReportEngineFactory.EXTENSION_REPORT_ENGINE_FACTORY );
- engine = factory.createReportEngine( config );
- engine.changeLogLevel( Level.WARNING );
- }
- catch( Exception ex )
- {
- ex.printStackTrace();
- }
-
-
- HTMLEmitterConfig emitterConfig = new HTMLEmitterConfig( );
- emitterConfig.setActionHandler( new HTMLActionHandler( ) );
- HTMLServerImageHandler imageHandler = new HTMLServerImageHandler( );
- emitterConfig.setImageHandler( imageHandler );
- config.getEmitterConfigs( ).put( "html", emitterConfig );
-
- IReportRunnable design = null;
-
-
- design = engine.openReportDesign("d:\\sample.rptdesign");
-
- ReportDesignHandle report = (ReportDesignHandle) design.getDesignHandle( );
- buildReport( report );
-
-
- IRunAndRenderTask task = engine.createRunAndRenderTask(design);
-
-
- HTMLRenderContext renderContext = new HTMLRenderContext();
-
- renderContext.setBaseURL("baseurl");
-
- renderContext.setBaseImageURL("urltoimages");
-
- renderContext.setImageDirectory("d:\\myimages");
-
-
- renderContext.setSupportedImageFormats("JPG;PNG;BMP;SVG");
- HashMap contextMap = new HashMap();
- contextMap.put( EngineConstants.APPCONTEXT_HTML_RENDER_CONTEXT, renderContext );
- task.setAppContext( contextMap );
-
-
-
-
-
-
- HTMLRenderOption options = new HTMLRenderOption();
-
-
- options.setOutputFileName("d:\\output.html");
-
-
- options.setOutputFormat("html");
- task.setRenderOption(options);
-
-
-
- task.run();
- task.close();
- engine.destroy();
- Platform.shutdown();
- System.out.println("Finished");
- }
-
- public void buildReport(ReportDesignHandle designHandle)
- {
- try
- {
- ElementFactory designFactory = designHandle.getElementFactory( );
- buildDataSource(designFactory, designHandle);
-
- ArrayList cols = new ArrayList();
- cols.add("OFFICECODE");
- cols.add("CITY");
- cols.add("COUNTRY");
-
- buildDataSet(cols, "From Offices", designFactory, designHandle);
-
- TableHandle table = designFactory.newTableItem( "table", cols.size() );
- table.setWidth( "100%" );
- table.setDataSet( designHandle.findDataSet( "ds" ) );
-
- PropertyHandle computedSet = table.getColumnBindings( );
- ComputedColumn cs1 = null;
-
- for( int i=0; i < cols.size(); i++ )
- {
- cs1 = StructureFactory.createComputedColumn();
- cs1.setName((String)cols.get(i));
- cs1.setExpression("dataSetRow[\"" + (String)cols.get(i) + "\"]");
- computedSet.addItem(cs1);
- }
-
-
- RowHandle tableheader = (RowHandle) table.getHeader( ).get( 0 );
-
- for( int i=0; i < cols.size(); i++ )
- {
- LabelHandle label1 = designFactory.newLabel( (String)cols.get(i) );
- label1.setText((String)cols.get(i));
- CellHandle cell = (CellHandle) tableheader.getCells( ).get( i );
- cell.getContent( ).add( label1 );
- }
-
-
- RowHandle tabledetail = (RowHandle) table.getDetail( ).get( 0 );
- for( int i=0; i < cols.size(); i++ )
- {
- CellHandle cell = (CellHandle) tabledetail.getCells( ).get( i );
- DataItemHandle data = designFactory.newDataItem( "data_"+(String)cols.get(i) );
- data.setResultSetColumn( (String)cols.get(i));
- cell.getContent( ).add( data );
- }
-
- designHandle.getBody( ).add( table );
- }
- catch(Exception e)
- {
- e.printStackTrace();
- }
- }
-
- void buildDataSource( ElementFactory designFactory, ReportDesignHandle designHandle ) throws SemanticException
- {
- OdaDataSourceHandle dsHandle = designFactory.newOdaDataSource( "Data Source", "org.eclipse.birt.report.data.oda.jdbc" );
- dsHandle.setProperty( "odaDriverClass", "org.eclipse.birt.report.data.oda.sampledb.Driver" );
- dsHandle.setProperty( "odaURL", "jdbc:classicmodels:sampledb" );
- dsHandle.setProperty( "odaUser", "ClassicModels" );
- dsHandle.setProperty( "odaPassword", "" );
-
- designHandle.getDataSources( ).add( dsHandle );
- }
-
- void buildDataSet(ArrayList cols, String fromClause, ElementFactory designFactory, ReportDesignHandle designHandle ) throws SemanticException
- {
- OdaDataSetHandle dsHandle = designFactory.newOdaDataSet( "ds", "org.eclipse.birt.report.data.oda.jdbc.JdbcSelectDataSet" );
- dsHandle.setDataSource( "Data Source" );
- String qry = "Select ";
- for( int i=0; i < cols.size(); i++ )
- {
- qry += " " + cols.get(i);
- if( i != (cols.size() -1) )
- {
- qry += ",";
- }
- }
- qry += " " + fromClause;
- dsHandle.setQueryText( qry );
- designHandle.getDataSets( ).add( dsHandle );
- }
-
-
-
-
- public static void main(String[] args)
- {
- try
- {
- ExecuteModifedReport ex = new ExecuteModifedReport( );
- ex.runReport();
- }
- catch ( Exception e )
- {
- e.printStackTrace();
- }
- }
- }
产生的output.html内容如下:
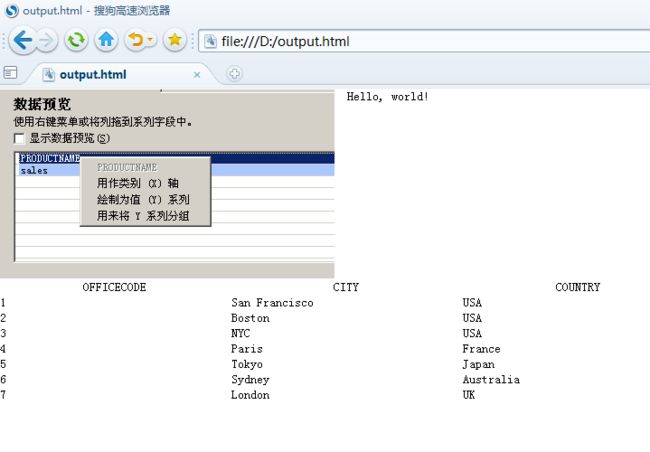
为什么能在运行展示报表之前进行设计上的修改,这是由报表展示器的设计架构决定的,前面提过,BIRT的展示器是包含了BIRT 设计引擎的,主要是在产生报表引擎阶段,如下图所示:
BIRT设计引擎API包含了报表设计所有元素的handle类,其中最重要的一些handle类结构如下:
- org.eclipse.birt.report.model.api.DesignElementHandle (implements org.eclipse.birt.report.model.elements.interfaces.IDesignElementModel)
- org.eclipse.birt.report.model.api.AccessControlHandle (implements org.eclipse.birt.report.model.elements.interfaces.IAccessControlModel)
- org.eclipse.birt.report.model.api.ValueAccessControlHandle (implements org.eclipse.birt.report.model.elements.interfaces.IValueAccessControlModel)
- org.eclipse.birt.report.model.api.FilterConditionElementHandle (implements org.eclipse.birt.report.model.elements.interfaces.IFilterConditionElementModel)
- org.eclipse.birt.report.model.api.MemberValueHandle (implements org.eclipse.birt.report.model.elements.interfaces.IMemberValueModel)
- org.eclipse.birt.report.model.api.ModuleHandle (implements org.eclipse.birt.report.model.api.core.IModuleModel)
- org.eclipse.birt.report.model.api.LibraryHandle (implements org.eclipse.birt.report.model.elements.interfaces.ILibraryModel)
- org.eclipse.birt.report.model.api.ReportDesignHandle (implements org.eclipse.birt.report.model.elements.interfaces.IReportDesignModel)
- org.eclipse.birt.report.model.api.MultiViewsHandle (implements org.eclipse.birt.report.model.elements.interfaces.IMultiViewsModel)
- org.eclipse.birt.report.model.api.ReportElementHandle
- org.eclipse.birt.report.model.api.CellHandle (implements org.eclipse.birt.report.model.elements.interfaces.ICellModel)
- org.eclipse.birt.report.model.api.ColumnHandle (implements org.eclipse.birt.report.model.elements.interfaces.ITableColumnModel)
- org.eclipse.birt.report.model.api.olap.CubeHandle (implements org.eclipse.birt.report.model.elements.interfaces.ICubeModel)
- org.eclipse.birt.report.model.api.olap.OdaCubeHandle (implements org.eclipse.birt.report.model.elements.interfaces.IOdaOlapElementModel)
- org.eclipse.birt.report.model.api.olap.TabularCubeHandle (implements org.eclipse.birt.report.model.elements.interfaces.ITabularCubeModel)
- org.eclipse.birt.report.model.api.DataSetHandle (implements org.eclipse.birt.report.model.elements.interfaces.IDataSetModel, org.eclipse.birt.report.model.elements.interfaces.ISimpleDataSetModel)
- org.eclipse.birt.report.model.api.JointDataSetHandle (implements org.eclipse.birt.report.model.elements.interfaces.IJointDataSetModel)
- org.eclipse.birt.report.model.api.OdaDataSetHandle (implements org.eclipse.birt.report.model.elements.interfaces.IOdaDataSetModel)
- org.eclipse.birt.report.model.api.ScriptDataSetHandle (implements org.eclipse.birt.report.model.elements.interfaces.IScriptDataSetModel)
- org.eclipse.birt.report.model.api.DataSourceHandle (implements org.eclipse.birt.report.model.elements.interfaces.IDataSourceModel)
- org.eclipse.birt.report.model.api.OdaDataSourceHandle (implements org.eclipse.birt.report.model.elements.interfaces.IOdaDataSourceModel, org.eclipse.birt.report.model.elements.interfaces.IOdaExtendableElementModel)
- org.eclipse.birt.report.model.api.ScriptDataSourceHandle (implements org.eclipse.birt.report.model.elements.interfaces.IScriptDataSourceModel)
- org.eclipse.birt.report.model.api.olap.DimensionHandle (implements org.eclipse.birt.report.model.elements.interfaces.IDimensionModel)
- org.eclipse.birt.report.model.api.olap.OdaDimensionHandle (implements org.eclipse.birt.report.model.elements.interfaces.IOdaOlapElementModel)
- org.eclipse.birt.report.model.api.olap.TabularDimensionHandle
- org.eclipse.birt.report.model.api.GroupHandle (implements org.eclipse.birt.report.model.elements.interfaces.IGroupElementModel)
- org.eclipse.birt.report.model.api.ListGroupHandle
- org.eclipse.birt.report.model.api.TableGroupHandle
- org.eclipse.birt.report.model.api.olap.HierarchyHandle (implements org.eclipse.birt.report.model.elements.interfaces.IHierarchyModel)
- org.eclipse.birt.report.model.api.olap.OdaHierarchyHandle (implements org.eclipse.birt.report.model.elements.interfaces.IOdaOlapElementModel)
- org.eclipse.birt.report.model.api.olap.TabularHierarchyHandle (implements org.eclipse.birt.report.model.elements.interfaces.ITabularHierarchyModel)
- org.eclipse.birt.report.model.api.olap.LevelHandle (implements org.eclipse.birt.report.model.elements.interfaces.ILevelModel)
- org.eclipse.birt.report.model.api.olap.OdaLevelHandle
- org.eclipse.birt.report.model.api.olap.TabularLevelHandle (implements org.eclipse.birt.report.model.elements.interfaces.ITabularLevelModel)
- org.eclipse.birt.report.model.api.MasterPageHandle (implements org.eclipse.birt.report.model.elements.interfaces.IMasterPageModel)
- org.eclipse.birt.report.model.api.GraphicMasterPageHandle (implements org.eclipse.birt.report.model.elements.interfaces.IGraphicMaterPageModel)
- org.eclipse.birt.report.model.api.SimpleMasterPageHandle (implements org.eclipse.birt.report.model.elements.interfaces.ISimpleMasterPageModel)
- org.eclipse.birt.report.model.api.olap.MeasureGroupHandle (implements org.eclipse.birt.report.model.elements.interfaces.IMeasureGroupModel)
- org.eclipse.birt.report.model.api.olap.OdaMeasureGroupHandle
- org.eclipse.birt.report.model.api.olap.TabularMeasureGroupHandle
- org.eclipse.birt.report.model.api.olap.MeasureHandle (implements org.eclipse.birt.report.model.elements.interfaces.IMeasureModel)
- org.eclipse.birt.report.model.api.olap.OdaMeasureHandle
- org.eclipse.birt.report.model.api.olap.TabularMeasureHandle
- org.eclipse.birt.report.model.api.ParameterGroupHandle (implements org.eclipse.birt.report.model.elements.interfaces.IParameterGroupModel)
- org.eclipse.birt.report.model.api.CascadingParameterGroupHandle (implements org.eclipse.birt.report.model.elements.interfaces.ICascadingParameterGroupModel)
- org.eclipse.birt.report.model.api.ParameterHandle (implements org.eclipse.birt.report.model.elements.interfaces.IParameterModel)
- org.eclipse.birt.report.model.api.ScalarParameterHandle (implements org.eclipse.birt.report.model.elements.interfaces.IScalarParameterModel)
- org.eclipse.birt.report.model.api.ReportItemHandle (implements org.eclipse.birt.report.model.api.elements.IReportItemMethodContext, org.eclipse.birt.report.model.elements.interfaces.IReportItemModel, org.eclipse.birt.report.model.elements.interfaces.IStyledElementModel)
- org.eclipse.birt.report.model.api.AutoTextHandle (implements org.eclipse.birt.report.model.elements.interfaces.IAutoTextModel)
- org.eclipse.birt.report.model.api.DataItemHandle (implements org.eclipse.birt.report.model.elements.interfaces.IDataItemModel)
- org.eclipse.birt.report.model.api.ExtendedItemHandle (implements org.eclipse.birt.report.model.elements.interfaces.IExtendedItemModel, org.eclipse.birt.report.model.api.elements.IReportItemMethodContext)
- org.eclipse.birt.report.model.api.FreeFormHandle (implements org.eclipse.birt.report.model.elements.interfaces.IFreeFormModel)
- org.eclipse.birt.report.model.api.GridHandle (implements org.eclipse.birt.report.model.elements.interfaces.IGridItemModel)
- org.eclipse.birt.report.model.api.ImageHandle (implements org.eclipse.birt.report.model.elements.interfaces.IImageItemModel)
- org.eclipse.birt.report.model.api.LabelHandle (implements org.eclipse.birt.report.model.elements.interfaces.ILabelModel)
- org.eclipse.birt.report.model.api.LineHandle (implements org.eclipse.birt.report.model.elements.interfaces.ILineItemModel)
- org.eclipse.birt.report.model.api.ListingHandle (implements org.eclipse.birt.report.model.elements.interfaces.IListingElementModel)
- org.eclipse.birt.report.model.api.ListHandle
- org.eclipse.birt.report.model.api.TableHandle (implements org.eclipse.birt.report.model.elements.interfaces.ITableItemModel)
- org.eclipse.birt.report.model.api.RectangleHandle
- org.eclipse.birt.report.model.api.TextDataHandle (implements org.eclipse.birt.report.model.elements.interfaces.ITextDataItemModel)
- org.eclipse.birt.report.model.api.MultiLineDataHandle
- org.eclipse.birt.report.model.api.TextItemHandle (implements org.eclipse.birt.report.model.elements.interfaces.ITextItemModel)
- org.eclipse.birt.report.model.api.RowHandle (implements org.eclipse.birt.report.model.elements.interfaces.ITableRowModel)
- org.eclipse.birt.report.model.api.StyleHandle (implements org.eclipse.birt.report.model.elements.interfaces.IStyleModel)
- org.eclipse.birt.report.model.api.PrivateStyleHandle
- org.eclipse.birt.report.model.api.SharedStyleHandle
- org.eclipse.birt.report.model.api.CssSharedStyleHandle
- org.eclipse.birt.report.model.api.TemplateElementHandle
- org.eclipse.birt.report.model.api.TemplateDataSetHandle
- org.eclipse.birt.report.model.api.TemplateReportItemHandle
- org.eclipse.birt.report.model.api.TemplateParameterDefinitionHandle (implements org.eclipse.birt.report.model.elements.interfaces.ITemplateParameterDefinitionModel)
- org.eclipse.birt.report.model.api.ThemeHandle (implements org.eclipse.birt.report.model.elements.interfaces.IThemeModel)
- org.eclipse.birt.report.model.api.SortElementHandle (implements org.eclipse.birt.report.model.elements.interfaces.ISortElementModel)
- org.eclipse.birt.report.model.api.VariableElementHandle (implements org.eclipse.birt.report.model.elements.interfaces.IVariableElementModel)
报表设计器本身包含所有的这些引擎类,当然也包含用于数据源,图表,展示的引擎:
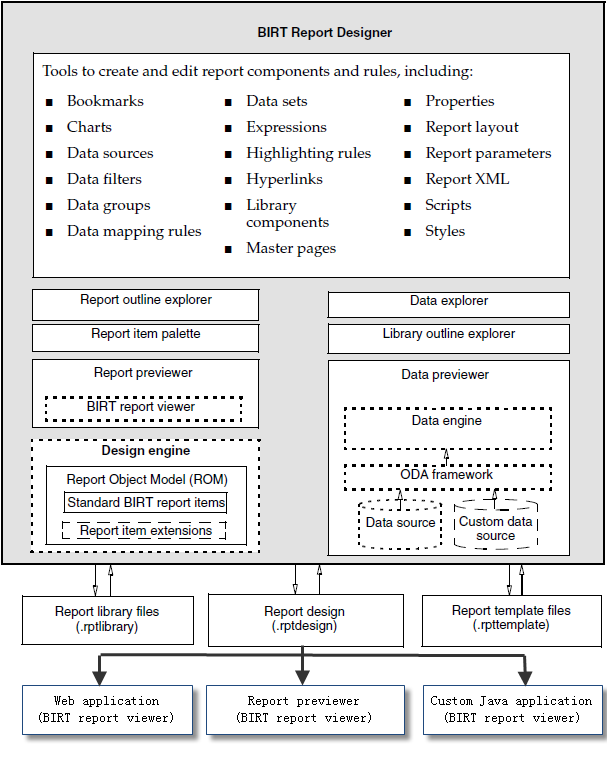
设计引擎中的主要类如下:
ReportDesignHandle类:
代表整个报表设计,报表设计定义了用于描述整个设计的特征,基础等等的一组属性。除了属性之外,报表设计也包含一些组成报表的元素。
这些元素包括:
Code Modules,适用于整个设计的全局脚本
Parameters,一个用于描述用户在运行报表时输入的数据参数元素的集合(list)
Data Sources,报表使用的数据源
Data Sets,报表定义的数据集
Color Palette,一个自定义的颜色名称的集合(set),并且是报表的一部分
Styles,用户在报表中自定义的用于格式化元素的样式文件,在这个样式文件的集合中,每种样式必须有唯一的名字。
Page Setup,代表报表内的母版页布局
Components,报表中定义的可重复使用的报表项目中。报表项目可以扩大这些项目。可以为这个设计定义了一个“私人图书馆”
Body,Visual报表的内容清单。内容是由一个或多个节组成的。一个节是一个报表项目填补了页面的宽度。它可以包含文字,表格,列表,表格等元素
Scratch Pad,当重建报表时需要提供一个临时的地方移动报表项目。
Translations,针对报表的额外的消息列表集合
Images 报表中嵌入的图像列表集合
模块允许使用在库中定义的组件
-
-
- ReportDesignHandle designHandle = ...;
- designHandle.includeLibrary( "libA.rptlibrary", "LibA" );
- LibraryHandle libraryHandle = designHandle.getLibrary("LibA");
-
-
-
- LabelHandle labelHandle = (LabelHandle) libraryHandle.findElement("companyNameLabel");
- LabelHandle myLabelHandle = (LabelHandle) designHandle.getElementFactory().newElementFrom( labelHandle, "myLabel" );
-
-
-
- designHandle.getBody().add(myLabelHandle);
除了继承自org.eclipse.birt.report.model.api.ModuleHandle的方法,继承自org.eclipse.birt.report.model.api.DesignElementHandle的方法,LibraryHandle还有自有的方法:
ElementFactory类
创建一个新的报表元素,并返回处理它。使用这个类来创建报表元素。创建一个元素后,通过SlotHandle类中的增加方法将它添加到设计中。通过调用这个类的getElementFactory方法来获取一个实例
SessionHandle类
代表报表设计的状态 – 一个用户有一个session。在Eclipse环境中,这代表了开放的设计集。在网络环境中,这代表了开放设计的session和语言环境。session有一个默认的样式属性集合和默认单位。session还拥有一些方法来创建和打开的报表设计。
OdaDataSourceHandle类
代表数据源
OdaDataSetHandle类
代表数据集
DesignConfig类
报表设计引擎配置设置。允许引擎提供图像处理,超链接处理和字体处理等个性化的实现
IdesignEngine类
代表作为一个整体BIRT的设计引擎。用于创建新的会话session
IDesignEngineFactory类
工厂类,用于创建一个新的设计引擎实例。
TableHandle类
代表一个table元素。一个table有一个本地化的标题,并且可以重复其在每一页上的标题。一个table是一个结构化的行和列的清单集合。这些列被定义为整个表,这些行被聚集成集。
PropertyHandle类
一个用于处理一个元素的顶级物业工作的处理器
ComputedColumn类
描述了一个为数据集或报表查询而定义的计算列。计算列有一个名字,和一个JavaScript表达式,用于计算这个列的值
StructureFactory类
提供一个工厂方法,用于创建一个空的结构
RowHandle类
代表一个网格或者表格中的一行。每一行包含多个cell,每一行可以定义它的高度。
CellHandle类
代表了一个表或表格里的单元格cell。一个单元格cell可以跨越多个行或列。一个单元格cell可以包含零个,一个或多个内容content。不过,由于BIRT的多个项目将自动定位,如果单元格cell拥有多个项目,应用程序一般应提供其自己的集装箱container。
应用程序一般不直接创造cell处理器。相反,它使用的导航方法对其他元素提供一个处理诸如RowHandle。
DataItemHandle类
代表一个数据项元素。一个数据项有一个动作,值表达和帮助文本
EngineConfig类
环绕的报表引擎的配置设置。允许开发人员指定在何处寻找引擎插件,数据驱动,并在写图像文件。允许用户自定义数据相关的属性(即数据引擎)。也可以让引擎提供的图像处理,超链接处理和字体处理等个性化的实现
IReportEngineFactory
一个工厂类,用于创建报表引擎。
IReportEngine
代表一个报表引擎
一个报表引擎提供了报表功能的切入点。这是全球定制的报表生成和渲染过程。这也是引擎收集统计数据的地方。通过报表引擎,报表生成,并能提供不同的输出格式。查询也可以执行预览的目的,不涉及一个完整的报告生成
HTMLRenderOption
用于输出网页格式的报表
用户可以通过查询API文档查询每个handle的用法,如何修改设计,在此我不在把API文档粘贴过来,如下:
下面的例子演示了SessionHandle,ReportDesignHandle,IncludedCssStyleSheetHandle,SlotHandle,DesignElementHandle,PropertyHandle,StructureHandle,MemberHandle,GridHandle,ListingHandle,ImageHandle,ExtendedItemHandle的用法
上面的源码读取一个设计文档TopNPercent.rptdesign,并未进行修改,在控制台打印设计信息如下:
- Contents Count: 3
- Slot Count for a report design 11
- StandardProperty Refresh rate--0
- StandardProperty Event handler class--null
- StandardProperty New handler on each event--false
- StandardProperty Layout preference--auto layout
- StandardProperty Report orientation--ltr
- StandardProperty Enable Data Security--false
- StandardProperty Cascade ACL--true
- StandardProperty ACL expression--null
- StandardProperty Image DPI--null
- StandardProperty Page variables--null
- StandardProperty Data objects--null
- StandardProperty Locale--null
- StandardProperty Initialize--null
- StandardProperty On prepare--null
- StandardProperty Before factory--null
- StandardProperty After factory--null
- StandardProperty Before render--null
- StandardProperty After render--null
- StandardProperty On page start--null
- StandardProperty On page end--null
- StandardProperty Display name--null
- StandardProperty Display name key--null
- StandardProperty Icon file--null
- StandardProperty Cheat sheet--null
- StandardProperty Thumbnail--null
- StandardProperty Subject--null
- StandardProperty Help guide--null
- StandardProperty Base--null
- StandardProperty Units--in
- StandardProperty Theme--null
- StandardProperty Author--Mark Coggins
- StandardProperty Title--Top Classic Models Inc. Customers by Revenue
- StandardProperty Title key--null
- StandardProperty Description--null
- StandardProperty Description key--null
- StandardProperty Comments--null
- StandardProperty Include resource--null
- StandardProperty Created by--Eclipse BIRT Designer Version 2.5.2.v20100208 Build <2.5.2.v20100210-0630>
- StandardProperty External Meta Data--null
- C:\Documents and Settings\pclenahan\My Documents\ClassicModels\logos\Classic-Models-Full-M.jpg
- Found chart of type Bar Chart
- Finished
下面是一个综合利用各种报表元素handle生成的一个相对复杂的报表,加入了突出显示,报表参数,动态排序,聚合等等
- package birtbird;
-
- import java.io.IOException;
- import java.util.ArrayList;
- import java.util.List;
- import java.io.BufferedInputStream;
- import java.io.IOException;
- import java.io.InputStream;
-
- import org.eclipse.birt.core.framework.Platform;
- import org.eclipse.birt.report.model.api.CellHandle;
- import org.eclipse.birt.report.model.api.ColumnHandle;
- import org.eclipse.birt.report.model.api.DataItemHandle;
- import org.eclipse.birt.report.model.api.DesignConfig;
- import org.eclipse.birt.report.model.api.ElementFactory;
- import org.eclipse.birt.report.model.api.IDesignEngine;
- import org.eclipse.birt.report.model.api.IDesignEngineFactory;
- import org.eclipse.birt.report.model.api.LabelHandle;
- import org.eclipse.birt.report.model.api.ImageHandle;
- import org.eclipse.birt.report.model.api.ActionHandle;
- import org.eclipse.birt.report.model.api.ReportElementHandle;
- import org.eclipse.birt.report.model.api.RowOperationParameters;
- import org.eclipse.birt.report.model.api.ScalarParameterHandle;
- import org.eclipse.birt.report.model.api.ScriptLibHandle;
- import org.eclipse.birt.report.model.api.TextItemHandle;
- import org.eclipse.birt.report.model.elements.JointDataSet;
-
- import org.eclipse.birt.report.model.api.OdaDataSetHandle;
- import org.eclipse.birt.report.model.api.JointDataSetHandle;
- import org.eclipse.birt.report.model.api.JoinConditionHandle;
-
- import org.eclipse.birt.report.model.api.OdaDataSourceHandle;
- import org.eclipse.birt.report.model.api.PropertyHandle;
- import org.eclipse.birt.report.model.api.ReportDesignHandle;
- import org.eclipse.birt.report.model.api.RowHandle;
- import org.eclipse.birt.report.model.api.SessionHandle;
- import org.eclipse.birt.report.model.api.StructureFactory;
- import org.eclipse.birt.report.model.api.TableHandle;
- import org.eclipse.birt.report.model.api.activity.SemanticException;
- import org.eclipse.birt.report.model.api.elements.structures.ComputedColumn;
- import org.eclipse.birt.report.model.api.PropertyHandle;
- import org.eclipse.birt.report.model.elements.ReportItem;
-
- import org.eclipse.birt.report.model.api.elements.structures.EmbeddedImage;
-
- import org.eclipse.birt.report.model.elements.Style;
- import org.eclipse.birt.report.model.elements.ReportDesign;
-
- import org.eclipse.birt.report.model.api.StyleHandle;
-
- import org.eclipse.birt.report.model.api.elements.structures.AggregationArgument;
- import org.eclipse.birt.report.model.api.elements.structures.DateTimeFormatValue;
- import org.eclipse.birt.report.model.api.elements.structures.FormatValue;
- import org.eclipse.birt.report.model.api.elements.structures.MapRule;
- import org.eclipse.birt.report.model.api.elements.structures.HideRule;
- import org.eclipse.birt.report.model.api.elements.structures.PropertyBinding;
- import org.eclipse.birt.report.model.api.elements.structures.TOC;
- import org.eclipse.birt.report.model.api.elements.structures.JoinCondition;
- import org.eclipse.birt.report.model.api.elements.structures.ParamBinding;
-
- import org.eclipse.birt.report.model.api.elements.DesignChoiceConstants;
-
- import org.eclipse.birt.report.model.api.elements.structures.HighlightRule;
- import org.eclipse.birt.report.model.elements.interfaces.IJointDataSetModel;
-
- import org.eclipse.birt.report.model.api.elements.structures.SortKey;
- import org.eclipse.birt.report.model.api.SortKeyHandle;
-
- import org.eclipse.birt.report.model.api.elements.structures.FilterCondition;
- import org.eclipse.birt.report.model.api.elements.structures.Action;
- import org.eclipse.birt.report.model.api.elements.structures.IncludeScript;
- import java.util.Iterator;
-
- import com.ibm.icu.util.ULocale;
-
-
-
-
-
- public class StructFactoryTest {
- ReportDesignHandle designHandle = null;
- ElementFactory designFactory = null;
- StructureFactory structFactory = null;
-
- public static void main(String[] args) {
- try {
- StructFactoryTest de = new StructFactoryTest();
- de.buildReport();
- } catch (IOException e) {
-
- e.printStackTrace();
- } catch (SemanticException e) {
-
- e.printStackTrace();
- }
- }
-
- void buildDataSource() throws SemanticException {
-
- OdaDataSourceHandle dsHandle = designFactory.newOdaDataSource(
- "Data Source", "org.eclipse.birt.report.data.oda.jdbc");
- dsHandle.setProperty("odaDriverClass",
- "org.eclipse.birt.report.data.oda.sampledb.Driver");
- dsHandle.setProperty("odaURL", "jdbc:classicmodels:sampledb");
- dsHandle.setProperty("odaUser", "ClassicModels");
- dsHandle.setProperty("odaPassword", "");
-
- PropertyBinding pb = new PropertyBinding();
-
- designHandle.getDataSources().add(dsHandle);
- long currid = dsHandle.getID();
-
- }
-
- void buildDataSet() throws SemanticException {
-
- OdaDataSetHandle dsHandle = designFactory.newOdaDataSet("ds",
- "org.eclipse.birt.report.data.oda.jdbc.JdbcSelectDataSet");
- dsHandle.setDataSource("Data Source");
- String qry = "Select * from customers";
-
- dsHandle.setQueryText(qry);
-
- addFilterCondition(dsHandle);
-
- designHandle.getDataSets().add(dsHandle);
-
- }
-
- void buildDataSet2() throws SemanticException {
-
- OdaDataSetHandle dsHandle = designFactory.newOdaDataSet("ds2",
- "org.eclipse.birt.report.data.oda.jdbc.JdbcSelectDataSet");
- dsHandle.setDataSource("Data Source");
- String qry = "Select * from orderdetails where ordernumber = ?";
-
- dsHandle.setQueryText(qry);
-
- addFilterCondition(dsHandle);
-
- designHandle.getDataSets().add(dsHandle);
-
- }
-
- void buildJointDataSet() throws SemanticException {
- OdaDataSetHandle dsHandle1 = designFactory.newOdaDataSet("ds1",
- "org.eclipse.birt.report.data.oda.jdbc.JdbcSelectDataSet");
- dsHandle1.setDataSource("Data Source");
- String qry1 = "Select * from customers";
-
- dsHandle1.setQueryText(qry1);
-
- OdaDataSetHandle dsHandle2 = designFactory.newOdaDataSet("ds2",
- "org.eclipse.birt.report.data.oda.jdbc.JdbcSelectDataSet");
- dsHandle2.setDataSource("Data Source");
- String qry2 = "Select * from orders";
-
- dsHandle2.setQueryText(qry2);
-
- JointDataSetHandle jds = designFactory.newJointDataSet("test");
-
- designHandle.getDataSets().add(dsHandle1);
- designHandle.getDataSets().add(dsHandle2);
-
- jds.addDataSet("ds1");
- jds.addDataSet("ds2");
-
- String leftExpression = "dataSetRow[\"CUSTOMERNUMBER\"]";
- String rightExpression = "dataSetRow[\"CUSTOMERNUMBER\"]";
- JoinCondition condition = StructureFactory.createJoinCondition();
- condition.setJoinType(DesignChoiceConstants.JOIN_TYPE_LEFT_OUT);
- condition.setOperator(DesignChoiceConstants.JOIN_OPERATOR_EQALS);
- condition.setLeftDataSet("ds1");
- condition.setRightDataSet("ds2");
- condition.setLeftExpression(leftExpression);
- condition.setRightExpression(rightExpression);
-
- PropertyHandle conditionHandle = jds
- .getPropertyHandle(JointDataSet.JOIN_CONDITONS_PROP);
- conditionHandle.addItem(condition);
-
- designHandle.getDataSets().add(jds);
-
- }
-
- void addMapRule(TableHandle th) {
- try {
-
- MapRule mr = structFactory.createMapRule();
- mr.setTestExpression("row[\"CustomerCreditLimit\"]");
- mr.setOperator(DesignChoiceConstants.MAP_OPERATOR_EQ);
- mr.setValue1("0");
- mr.setDisplay("N/A");
-
- PropertyHandle ph = th
- .getPropertyHandle(StyleHandle.MAP_RULES_PROP);
- ph.addItem(mr);
- } catch (Exception e) {
- e.printStackTrace();
- }
- }
-
- void addVisRule(ReportElementHandle rh) {
- try {
- HideRule hr = structFactory.createHideRule();
- hr.setFormat("pdf");
- hr.setExpression("true");
-
- PropertyHandle ph = rh
- .getPropertyHandle(ReportItem.VISIBILITY_PROP);
- ph.addItem(hr);
- } catch (Exception e) {
- e.printStackTrace();
- }
- }
-
- void addBottomBorder(ReportElementHandle rh) {
- try {
-
- rh.setProperty(StyleHandle.BORDER_BOTTOM_COLOR_PROP, "#000000");
- rh.setProperty(StyleHandle.BORDER_BOTTOM_STYLE_PROP, "solid");
- rh.setProperty(StyleHandle.BORDER_BOTTOM_WIDTH_PROP, "2px");
-
- } catch (Exception e) {
- e.printStackTrace();
- }
- }
-
- void addHighLightRule(RowHandle th) {
- try {
- HighlightRule hr = structFactory.createHighlightRule();
-
- hr.setOperator(DesignChoiceConstants.MAP_OPERATOR_GT);
- hr.setTestExpression("row[\"CustomerCreditLimit\"]");
- hr.setValue1("100000");
- hr.setProperty(HighlightRule.BACKGROUND_COLOR_MEMBER, "blue");
-
- PropertyHandle ph = th
- .getPropertyHandle(StyleHandle.HIGHLIGHT_RULES_PROP);
-
- ph.addItem(hr);
- } catch (Exception e) {
- e.printStackTrace();
- }
- }
-
- void addSortKey(TableHandle th) {
- try {
- SortKey sk = structFactory.createSortKey();
-
- sk.setDirection(DesignChoiceConstants.SORT_DIRECTION_ASC);
- sk.setKey("if( params[\"srt\"].value){ if( params[\"srt\"].value == 'a' ){ row[\"CustomerName\"]; }else{ row[\"CustomerCity\"];}}");
-
- PropertyHandle ph = th.getPropertyHandle(TableHandle.SORT_PROP);
- ph.addItem(sk);
- } catch (Exception e) {
- e.printStackTrace();
- }
- }
-
- void modSortKey(TableHandle th) {
- try {
- SortKeyHandle sk;
- PropertyHandle ph = th.getPropertyHandle(TableHandle.SORT_PROP);
-
- sk = (SortKeyHandle) ph.get(0);
- sk.setDirection(DesignChoiceConstants.SORT_DIRECTION_DESC);
- } catch (Exception e) {
- e.printStackTrace();
- }
- }
-
- void addFilterCondition(OdaDataSetHandle dh) {
- try {
- FilterCondition fc = structFactory.createFilterCond();
- fc.setExpr("row[\"COUNTRY\"]");
- fc.setOperator(DesignChoiceConstants.MAP_OPERATOR_EQ);
- fc.setValue1("'USA'");
-
- dh.addFilter(fc);
-
- } catch (Exception e) {
- e.printStackTrace();
- }
- }
-
- void addFilterCondition(TableHandle th) {
- try {
-
- FilterCondition fc = structFactory.createFilterCond();
- fc.setExpr("row[\"CustomerCountry\"]");
- fc.setOperator(DesignChoiceConstants.MAP_OPERATOR_EQ);
- fc.setValue1("'USA'");
-
- PropertyHandle ph = th.getPropertyHandle(TableHandle.FILTER_PROP);
-
- ph.addItem(fc);
- } catch (Exception e) {
- e.printStackTrace();
- }
- }
-
- void addHyperlink(LabelHandle lh) {
- try {
- Action ac = structFactory.createAction();
-
- ActionHandle actionHandle = lh.setAction(ac);
-
- actionHandle
- .setLinkType(DesignChoiceConstants.ACTION_LINK_TYPE_DRILL_THROUGH);
- actionHandle.setReportName("d:\\TopNPercent.rptdesign");
- actionHandle.setTargetFileType("report-design");
- actionHandle.setTargetWindow("_blank");
- actionHandle.getMember("paramBindings");
- ParamBinding pb = structFactory.createParamBinding();
- pb.setParamName("order");
- pb.setExpression("row[\"ORDERNUMBER\"]");
- actionHandle.addParamBinding(pb);
-
- } catch (Exception e) {
- e.printStackTrace();
- }
- }
-
- void addToc(DataItemHandle dh) {
- try {
- TOC myToc = structFactory.createTOC("row[\"CustomerName\"]");
-
- dh.addTOC(myToc);
- } catch (Exception e) {
- e.printStackTrace();
- }
- }
-
- void addImage() {
- try {
- EmbeddedImage image = structFactory.createEmbeddedImage();
- image.setType(DesignChoiceConstants.IMAGE_TYPE_IMAGE_JPEG);
- image.setData(load("logo3.jpg"));
- image.setName("mylogo");
-
- designHandle.addImage(image);
- } catch (Exception e) {
- e.printStackTrace();
- }
- }
-
- public byte[] load(String fileName) throws IOException {
- InputStream is = null;
-
- is = new BufferedInputStream(this.getClass().getResourceAsStream(
- fileName));
- byte data[] = null;
- if (is != null) {
- try {
- data = new byte[is.available()];
- is.read(data);
- } catch (IOException e1) {
- throw e1;
- }
- }
- return data;
- }
-
- void addScript(ReportDesignHandle rh) {
- try {
- IncludeScript is = structFactory.createIncludeScript();
- is.setFileName("test.js");
-
-
-
-
- } catch (Exception e) {
- e.printStackTrace();
- }
- }
-
- void buildReport() throws IOException, SemanticException {
-
- DesignConfig config = new DesignConfig();
- config.setBIRTHome("E:\\birt汉化包\\birt-runtime-3_7_2\\ReportEngine");
- IDesignEngine engine = null;
- try {
-
- Platform.startup(config);
- IDesignEngineFactory factory = (IDesignEngineFactory) Platform
- .createFactoryObject(IDesignEngineFactory.EXTENSION_DESIGN_ENGINE_FACTORY);
- engine = factory.createDesignEngine(config);
-
- } catch (Exception ex) {
- ex.printStackTrace();
- }
-
- SessionHandle session = engine.newSessionHandle(ULocale.ENGLISH);
-
- ReportDesignHandle design = null;
-
- try {
-
- designHandle = session.createDesign();
- addScript(designHandle);
- designFactory = designHandle.getElementFactory();
- buildDataSource();
- buildDataSet();
- buildJointDataSet();
-
- TableHandle table = designFactory.newTableItem("table", 3);
- table.setWidth("100%");
- table.setDataSet(designHandle.findDataSet("ds"));
-
-
- RowOperationParameters ro = new RowOperationParameters(2, -1, 0);
- table.insertRow(ro);
-
- PropertyHandle computedSet = table.getColumnBindings();
- ComputedColumn cs1, cs2, cs3, cs4, cs5;
-
- cs1 = StructureFactory.createComputedColumn();
- cs1.setName("CustomerName");
- cs1.setExpression("dataSetRow[\"CUSTOMERNAME\"]");
- computedSet.addItem(cs1);
- cs2 = StructureFactory.createComputedColumn();
- cs2.setName("CustomerCity");
- cs2.setExpression("dataSetRow[\"CITY\"]");
-
- computedSet.addItem(cs2);
- cs3 = StructureFactory.createComputedColumn();
- cs3.setName("CustomerCountry");
- cs3.setExpression("dataSetRow[\"COUNTRY\"]");
- computedSet.addItem(cs3);
- cs4 = StructureFactory.createComputedColumn();
- cs4.setName("CustomerCreditLimit");
- cs4.setExpression("dataSetRow[\"CREDITLIMIT\"]");
- computedSet.addItem(cs4);
-
- cs5 = StructureFactory.createComputedColumn();
- cs5.setName("CustomerCreditLimitSum");
- cs5.setExpression("dataSetRow[\"CREDITLIMIT\"]");
- cs5.setAggregateFunction("sum");
- computedSet.addItem(cs5);
-
-
- RowHandle tableheader = (RowHandle) table.getHeader().get(0);
-
- ColumnHandle ch = (ColumnHandle) table.getColumns().get(0);
- ch.setProperty("width", "50%");
-
- ColumnHandle ch2 = (ColumnHandle) table.getColumns().get(1);
- ch2.setSuppressDuplicates(true);
-
- LabelHandle label1 = designFactory.newLabel("Label1");
- label1.setOnRender("var x = 3;");
- addBottomBorder(label1);
- label1.setText("Customer");
- CellHandle cell = (CellHandle) tableheader.getCells().get(0);
-
- cell.getContent().add(label1);
- LabelHandle label2 = designFactory.newLabel("Label2");
- label2.setText("City");
- cell = (CellHandle) tableheader.getCells().get(1);
- cell.getContent().add(label2);
- LabelHandle label3 = designFactory.newLabel("Label3");
- label3.setText("Credit Limit");
- cell = (CellHandle) tableheader.getCells().get(2);
-
- cell.getContent().add(label3);
-
-
- RowHandle tabledetail = (RowHandle) table.getDetail().get(1);
-
- cell = (CellHandle) tabledetail.getCells().get(0);
- DataItemHandle data = designFactory.newDataItem("data1");
- data.setResultSetColumn("CustomerName");
-
- addToc(data);
-
- cell.getContent().add(data);
- cell = (CellHandle) tabledetail.getCells().get(1);
- data = designFactory.newDataItem("data2");
- data.setResultSetColumn("CustomerCity");
- cell.getContent().add(data);
- cell = (CellHandle) tabledetail.getCells().get(2);
- data = designFactory.newDataItem("data3");
- TextItemHandle tih = designFactory.newTextItem("mytextitem");
-
-
- data.setResultSetColumn("CustomerCreditLimit");
- cell.getContent().add(data);
-
- FormatValue fv = structFactory.newFormatValue();
- fv.setPattern("#,##0.00{RoundingMode=HALF_UP}");
- fv.setCategory("custom");
- data.setProperty("numberFormat", fv);
-
- addHyperlink(label1);
- addMapRule(table);
- addHighLightRule(tabledetail);
- addSortKey(table);
- modSortKey(table);
- addFilterCondition(table);
-
-
- RowHandle tablefooter = (RowHandle) table.getFooter().get(0);
- cell = (CellHandle) tablefooter.getCells().get(0);
-
- ImageHandle image1 = designFactory.newImage("mylogo");
- image1.setImageName("mylogo");
-
-
-
-
- cell = (CellHandle) tablefooter.getCells().get(2);
- data = designFactory.newDataItem("datasum");
- data.setResultSetColumn("CustomerCreditLimitSum");
-
- cell.getContent().add(data);
-
- ScalarParameterHandle sph = designFactory.newScalarParameter("srt");
- sph.setIsRequired(false);
-
-
- sph.setValueType(DesignChoiceConstants.PARAM_VALUE_TYPE_STATIC);
- sph.setDataType(DesignChoiceConstants.PARAM_TYPE_STRING);
- designHandle.getParameters().add(sph);
-
-
- table.getDetail().get(0).drop();
- designHandle.getBody().add(table);
-
-
-
-
- designHandle.saveAs("d:\\structfactorytest.rptdesign");
- designHandle.close();
- Platform.shutdown();
- System.out.println("Finished");
- } catch (Exception e) {
- e.printStackTrace();
- }
-
- }
- }
生成的structfactorytest.rptdesign预览如下:
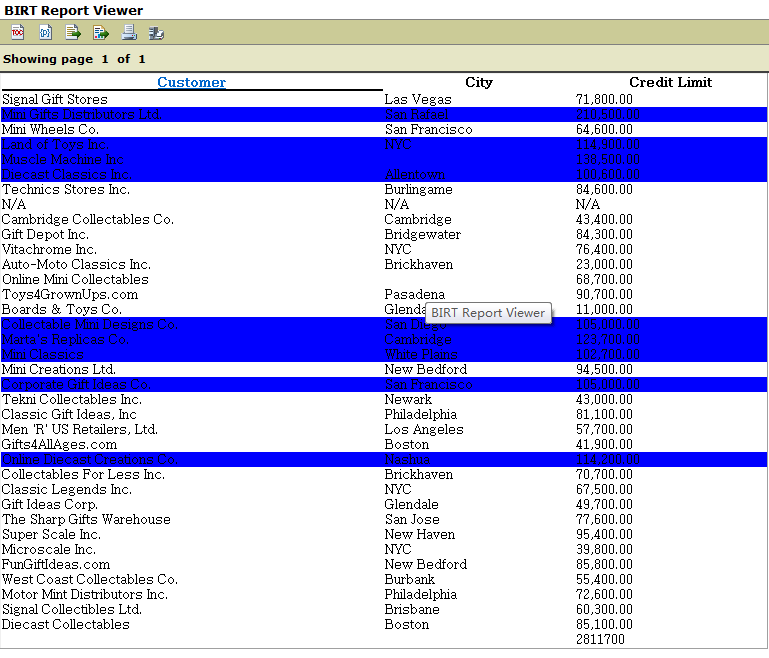
输入参数srt为a之后的预览如下(故意显示了目录,srt等于a意味着要根据customer逆序排序):
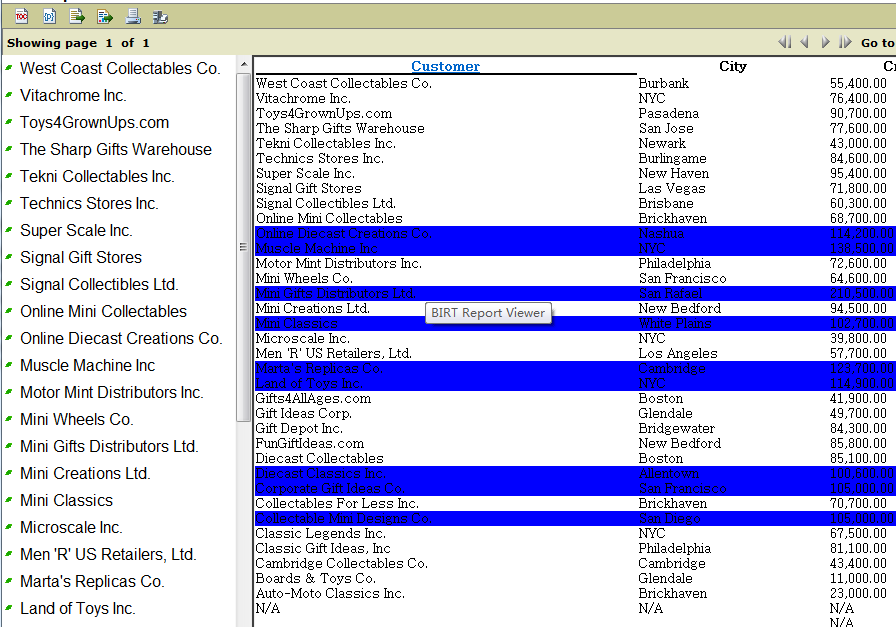
当然我们还能设计交叉报表和分组报表,下面就是一个设计交叉报表的案例:
- package birtbird;
-
-
- import java.io.IOException;
-
- import org.eclipse.birt.core.framework.Platform;
- import org.eclipse.birt.report.item.crosstab.core.ICrosstabConstants;
- import org.eclipse.birt.report.item.crosstab.core.de.CrosstabCellHandle;
- import org.eclipse.birt.report.item.crosstab.core.de.CrosstabReportItemHandle;
- import org.eclipse.birt.report.item.crosstab.core.de.DimensionViewHandle;
- import org.eclipse.birt.report.item.crosstab.core.de.LevelViewHandle;
- import org.eclipse.birt.report.item.crosstab.core.de.MeasureViewHandle;
- import org.eclipse.birt.report.item.crosstab.core.util.CrosstabExtendedItemFactory;
- import org.eclipse.birt.report.model.api.ComputedColumnHandle;
- import org.eclipse.birt.report.model.api.DataItemHandle;
- import org.eclipse.birt.report.model.api.DataSetHandle;
- import org.eclipse.birt.report.model.api.DesignConfig;
- import org.eclipse.birt.report.model.api.DesignElementHandle;
- import org.eclipse.birt.report.model.api.ElementFactory;
- import org.eclipse.birt.report.model.api.ExtendedItemHandle;
- import org.eclipse.birt.report.model.api.IDesignEngine;
- import org.eclipse.birt.report.model.api.IDesignEngineFactory;
- import org.eclipse.birt.report.model.api.LabelHandle;
- import org.eclipse.birt.report.model.api.OdaDataSetHandle;
- import org.eclipse.birt.report.model.api.OdaDataSourceHandle;
- import org.eclipse.birt.report.model.api.ReportDesignHandle;
- import org.eclipse.birt.report.model.api.ReportItemHandle;
- import org.eclipse.birt.report.model.api.SessionHandle;
- import org.eclipse.birt.report.model.api.StructureFactory;
- import org.eclipse.birt.report.model.api.activity.SemanticException;
- import org.eclipse.birt.report.model.api.elements.DesignChoiceConstants;
- import org.eclipse.birt.report.model.api.elements.structures.ComputedColumn;
- import org.eclipse.birt.report.model.api.extension.IReportItem;
- import org.eclipse.birt.report.model.api.olap.MeasureGroupHandle;
- import org.eclipse.birt.report.model.api.olap.MeasureHandle;
- import org.eclipse.birt.report.model.api.olap.TabularCubeHandle;
- import org.eclipse.birt.report.model.api.olap.TabularDimensionHandle;
- import org.eclipse.birt.report.model.api.olap.TabularHierarchyHandle;
- import org.eclipse.birt.report.model.api.olap.TabularLevelHandle;
- import org.eclipse.birt.report.model.elements.interfaces.ICubeModel;
-
- import com.ibm.icu.util.ULocale;
-
- public class CreateDataCube
- {
- ReportDesignHandle designHandle = null;
- ElementFactory designFactory = null;
- StructureFactory structFactory = null;
-
- public static void main( String[] args )
- {
- try
- {
- CreateDataCube de = new CreateDataCube();
- de.buildReport();
- }
- catch ( IOException e )
- {
- e.printStackTrace();
- }
- catch ( SemanticException e )
- {
- e.printStackTrace();
- }
- }
-
- void buildDataSource( ) throws SemanticException
- {
- OdaDataSourceHandle dsHandle = designFactory.newOdaDataSource(
- "Data Source", "org.eclipse.birt.report.data.oda.jdbc" );
- dsHandle.setProperty( "odaDriverClass",
- "org.eclipse.birt.report.data.oda.sampledb.Driver" );
- dsHandle.setProperty( "odaURL", "jdbc:classicmodels:sampledb" );
- dsHandle.setProperty( "odaUser", "ClassicModels" );
- dsHandle.setProperty( "odaPassword", "" );
- designHandle.getDataSources( ).add( dsHandle );
- }
-
- void buildDataSet( ) throws SemanticException
- {
- OdaDataSetHandle dsHandle = designFactory.newOdaDataSet( "ds",
- "org.eclipse.birt.report.data.oda.jdbc.JdbcSelectDataSet" );
- dsHandle.setDataSource( "Data Source" );
- String qry = "Select * from customers";
- dsHandle.setQueryText( qry );
- designHandle.getDataSets( ).add( dsHandle );
- }
- void buidCube() throws SemanticException
- {
- TabularCubeHandle cubeHandle = designHandle.getElementFactory( )
- .newTabularCube( "MyCube" );
- cubeHandle.setDataSet((DataSetHandle)designHandle.getDataSets().get(0));
- designHandle.getCubes( ).add( cubeHandle );
-
-
- MeasureGroupHandle measureGroupHandle = designHandle
- .getElementFactory( ).newTabularMeasureGroup(
- "testMeasureGroup" );
- cubeHandle.setProperty( ICubeModel.MEASURE_GROUPS_PROP,
- measureGroupHandle );
-
- measureGroupHandle.add( MeasureGroupHandle.MEASURES_PROP, designFactory.newTabularMeasure( null ) );
- MeasureHandle measure = (MeasureHandle) measureGroupHandle.getContent(MeasureGroupHandle.MEASURES_PROP, 0 );
- measure.setName( "CREDITLIMIT" );
- measure.setMeasureExpression( "dataSetRow['CREDITLIMIT']" );
- measure.setFunction( DesignChoiceConstants.MEASURE_FUNCTION_SUM );
- measure.setCalculated( false );
- measure.setDataType( DesignChoiceConstants.COLUMN_DATA_TYPE_FLOAT );
-
- TabularDimensionHandle dimension = designFactory.newTabularDimension( null );
- cubeHandle.add(TabularCubeHandle.DIMENSIONS_PROP, dimension );
- dimension.setTimeType( false );
-
- dimension.add( TabularDimensionHandle.HIERARCHIES_PROP, designFactory.newTabularHierarchy( null ) );
- TabularHierarchyHandle hierarchy = (TabularHierarchyHandle) dimension.getContent( TabularDimensionHandle.HIERARCHIES_PROP, 0 );
-
- hierarchy.setDataSet( (DataSetHandle)designHandle.getDataSets().get(0) );
-
- hierarchy.add( TabularHierarchyHandle.LEVELS_PROP, designFactory.newTabularLevel( dimension, null ) );
- TabularLevelHandle level = (TabularLevelHandle) hierarchy.getContent(TabularHierarchyHandle.LEVELS_PROP, 0 );
- level.setName( "testlevel" );
- level.setColumnName( "CUSTOMERNAME" );
- level.setDataType( DesignChoiceConstants.COLUMN_DATA_TYPE_STRING );
-
- ExtendedItemHandle xtab = CrosstabExtendedItemFactory.createCrosstabReportItem(designHandle, cubeHandle, "MyCrosstab" );
- designHandle.getBody().add(xtab);
-
-
- IReportItem reportItem = xtab.getReportItem( );
- CrosstabReportItemHandle xtabHandle = (CrosstabReportItemHandle) reportItem;
- DimensionViewHandle dvh = xtabHandle.insertDimension(dimension, ICrosstabConstants.ROW_AXIS_TYPE, 0);
- LevelViewHandle levelViewHandle =dvh.insertLevel(level, 0);
- CrosstabCellHandle cellHandle = levelViewHandle.getCell( );
- DesignElementHandle eii = xtabHandle.getModelHandle( );
-
- ComputedColumn bindingColumn = StructureFactory.newComputedColumn( eii, level.getName( ) );
- ComputedColumnHandle bindingHandle = ((ReportItemHandle) eii).addColumnBinding( bindingColumn,false );
- bindingColumn.setDataType( level.getDataType( ) );
- String exp = "dimension['" + dimension.getName()+"']['"+level.getName()+"']";
- bindingColumn.setExpression( exp);
-
- DataItemHandle dataHandle = designFactory.newDataItem( level.getName( ) );
- dataHandle.setResultSetColumn( bindingHandle.getName( ) );
- cellHandle.addContent( dataHandle );
-
- MeasureViewHandle mvh = xtabHandle.insertMeasure(measure, 0);
-
-
- LabelHandle labelHandle = designFactory.newLabel( null );
- labelHandle.setText( measure.getName() );
- mvh.getHeader( ).addContent( labelHandle );
-
- }
-
-
- void buildReport() throws IOException, SemanticException
- {
-
- DesignConfig config = new DesignConfig( );
- config.setBIRTHome("E:\\birt汉化包\\birt-runtime-3_7_2\\ReportEngine");
- IDesignEngine engine = null;
- try{
- Platform.startup( config );
- IDesignEngineFactory factory = (IDesignEngineFactory) Platform
- .createFactoryObject( IDesignEngineFactory.EXTENSION_DESIGN_ENGINE_FACTORY );
- engine = factory.createDesignEngine( config );
- }catch( Exception ex){
- ex.printStackTrace();
- }
-
- SessionHandle session = engine.newSessionHandle( ULocale.ENGLISH ) ;
-
- try{
-
- designHandle = session.createDesign();
-
- designFactory = designHandle.getElementFactory( );
-
- buildDataSource();
- buildDataSet();
- buidCube();
-
-
-
- designHandle.saveAs("d:\\cubetest.rptdesign" );
- designHandle.close( );
- Platform.shutdown();
- System.out.println("Finished");
- }catch (Exception e){
- e.printStackTrace();
- }
-
- }
- }
生成的cubetest.rptdesign预览如下:
下面是一个设计图表的例子: