主要代码:
/****************************************************
@Title: 数据结构实验
@Name: <实验7-1> 图的遍历
@Object:
[实验目的]
实现图的存储结构;
实现图的深度优先和广度优先遍历
[实验提示]
1. 在 graph.h 中实现图的基本操作
2. 在 graph.h 中实现图的深度优先遍历和广度
优先遍历
@Include:
ds.h
类C通用模块
graph.h [*]
图的实现
@Usage:
请查看"TO-DO列表",根据要求完成代码
@Copyright: BTC 2004, Zhuang Bo
@Author: Zhuang Bo
@Date: 2004
@Description:
*****************************************************/
#include
#include
#include "ds.h"
#include "graph.h"
Status printvex(VexType val);
void main()
{
MGraph g;
CreateGraph(g);
PrintAdjMatrix(g);
printf("\n深度优先遍历:\n");
DFSTraverse(g,0,printvex);
printf("\n广度优先遍历:\n");
BFSTraverse(g,0,printvex);
DestroyGraph(g);
system("pause");
}
Status printvex(VexType val)
{
write(val);
return OK;
}
#ifndef GRAPH_H_INCLUDED
#define GRAPH_H_INCLUDED
#define MAXQSIZE 64
#include
#include "ds.h"
#define MAX_VERTEX_NUM 20 //最大顶点数
#define VexType char //顶点类型
#define ArcType int
#define INFINITY INT_MAX //无穷大
#define ElemType int
typedef struct {
VexType vexs[MAX_VERTEX_NUM];
ArcType arcs[MAX_VERTEX_NUM][MAX_VERTEX_NUM];
int vexnum,arcnum;
} MGraph;
typedef struct Sq{
ElemType *base;
int front;
int rear;
} SqQueue;
int visited[100];
Status CreateGraph(MGraph &G);
Status DestroyGraph(MGraph &G);
VexType GetVex(MGraph G, int v);
Status PutVex(MGraph &G, int v, VexType val);
Status InsertArc(MGraph &G, int v, int w, ArcType arc);
Status DeleteArc(MGraph &G, int v, int w);
Status InsertVex(MGraph &G, VexType val);
Status DeleteVex(MGraph &G, int v);
int FirstAdjVex(MGraph G, int v);
int NextAdjVex(MGraph G, int v, int w);
void DFSTraverse(MGraph G, int v, Status(*Visit)(VexType));
Status BFSTraverse(MGraph G, int v, Status(*Visit)(VexType));
void PrintAdjMatrix(MGraph G);
int locateVex(MGraph G, VexType v);
void PrintAdjMatrix(MGraph G) ;
void DFS (MGraph G, int v);
Status InitQueue(SqQueue &Q);
EnQueue(SqQueue &Q, ElemType e);
Status QueueEmpty(SqQueue Q);
Status DeQueue(SqQueue &Q, ElemType &e);
Status DeQueue(SqQueue &Q, ElemType &e)
{
if(Q.front==Q.rear){
return ERROR;
}
e=Q.base[Q.front];
Q.front=(Q.front+1)%MAXQSIZE;
return OK ;
}
Status QueueEmpty(SqQueue Q)
{
if(Q.rear==Q.front){
return TRUE;
}
return FALSE;
}
Status InitQueue(SqQueue &Q)
{
Q.base=(ElemType*)malloc(MAXQSIZE*sizeof(ElemType));
if(!Q.base){
exit(0);
}
Q.front=Q.rear=0;
return OK;
}
Status EnQueue(SqQueue &Q, ElemType e)
{
if((Q.rear+1)%MAXQSIZE==Q.front){
return ERROR;
}
Q.base[Q.rear]=e;
Q.rear=(Q.rear+1)%MAXQSIZE;
return OK;
}
int locateVex(MGraph G, VexType v){
for(int i = 0; i < G.vexnum; i++){
if(G.vexs[i] == v)
return i;
}
return -1;
}
Status CreateGraph(MGraph &G)
{
printf("输入顶点数和弧数如:(5,3):");
scanf("%d,%d", &G.vexnum, &G.arcnum);
printf("输入%d个顶点(以空格隔开如:v1 v2 v3):", G.vexnum);
getchar();
for(int m = 0; m < G.vexnum; m++){
scanf("%c", &G.vexs[m]);
getchar();
}
for( m = 0; m < G.vexnum; m++){
printf("%c", G.vexs[m]);
printf("\n");
}
int i=0, j=0;
for(i = 0; i < G.vexnum; i++){
for(j = 0; j < G.vexnum; j++)
G.arcs[i][j] = 0;
}
VexType v1, v2;
int w;
printf("\n每行输入一条弧依附的顶点(先弧尾后弧头)和权值(如:v1 v2 3):\n");
fflush(stdin);
for(int k = 0; k < G.arcnum; k++){
scanf("%c %c %d",&v1, &v2, &w);
fflush(stdin);
i = locateVex(G, v1);
j = locateVex(G, v2);
G.arcs[i][j] = w;
}
return OK;
}
Status DestroyGraph(MGraph &G)
{
return OK;
}
Status GetVex(MGraph G, int v, VexType &val)
{
if(v<0||v>=G.vexnum) return ERROR;
val = G.vexs[v];
return OK;
}
Status PutVex(MGraph &G, int v, VexType val)
{
if(v<0||v>=G.vexnum) return ERROR;
G.vexs[v] = val;
return OK;
}
Status InsertArc(MGraph &G, int v, int w, ArcType arc)
{
if(v<0||v>=G.vexnum||w<0||w>=G.vexnum) return ERROR;
G.arcs[v][w] = arc;
return OK;
}
Status DeleteArc(MGraph &G, int v, int w)
{
if(v<0||v>=G.vexnum||w<0||w>=G.vexnum) return ERROR;
G.arcs[v][w] = 0;
return OK;
}
Status InsertVex(MGraph &G, VexType val)
{
return ERROR;
}
Status DeleteVex(MGraph &G, int v)
{
return ERROR;
}
int FirstAdjVex(MGraph G, int v)
{
int i;
for(i=0;iif(G.arcs[v][i]){
return i;
}
}
return -1;
}
int NextAdjVex(MGraph G, int v, int w)
{
int i;
for(i=w+1;iif(G.arcs[v][i]){
return i;
}
}
return -1;
}
void DFSTraverse(MGraph G, int v, Status(*Visit)(VexType))
{
for(v=0;vfor(v=0;vif(!visited[v]){
DFS (G, v);
}
}
}
void DFS (MGraph G, int v){
visited[v]= TRUE;
write (v);
for(int w=FirstAdjVex(G, v);w>0;w=NextAdjVex(G, v, w)){
if(!visited[w])
DFS (G, w);
}
}
Status BFSTraverse(MGraph G, int v, Status(*Visit)(VexType))
{
int u;
SqQueue Q;
for(v=0;vfor(v=0;vif(!visited[v]){
visited[v]=TRUE;
write (v);
EnQueue(Q, v);
while(!QueueEmpty(Q)){
DeQueue(Q, u);
for(int w=FirstAdjVex(G, u);w>=0;w=NextAdjVex(G, u, w))
if(!visited[w]){
visited[w]=TRUE;
write (w);
EnQueue(Q, w);
}
}
}
return 1;
}
void PrintAdjMatrix(MGraph G)
{
int i,j;
for(i=0; ifor(j=0; j"%5d", G.arcs[i][j]);
printf("\n");
}
}
#endif //GRAPH_H_INCLUDED
演示效果图:
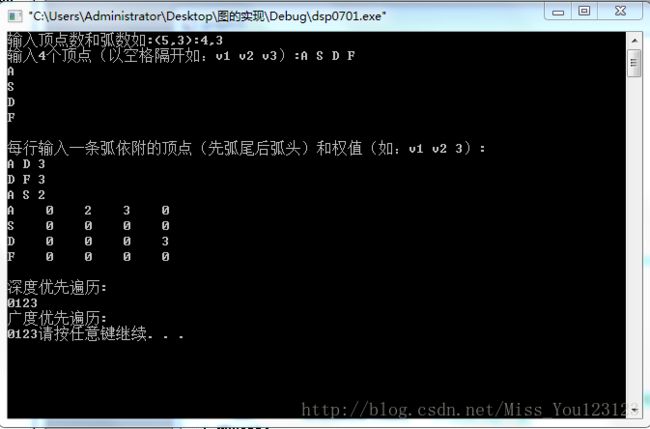