- LeetCode周赛——383
duanyq666
LeetCode周赛java算法开发语言leetcode
1.边界上的蚂蚁(模拟)classSolution{public:intreturnToBoundaryCount(vector&nums){intn=nums.size();intres=0,cnt=0;for(inti=0;iz(n);z[0]=n-1;//z函数匹配for(inti=1,l=0,r=0;ir)l=i,r=i+z[i]-1;}for(inti=1;i>resultGrid(ve
- LeetCode周赛384 题解
嘗_
算法leetcode
AK第384场周赛-力扣(LeetCode)前两题都是签到,略。第三题:回文字符串的最大数量1、题意:给定一个字符串数组,总字符数量不超过1e6,可以交换其中的任意两个字符,问能构造最多几个回文字符串。2、题解:首先我们要知道,无论怎么交换,字符数组内的各个字符串的长度不能发生改变,只是原来的字符布局发生了改变,那我们不妨先将所有的字符拿出来然后依次发出去,问题此时转化为了怎么分配字符使得回文字符
- LeetCode周赛——384
duanyq666
LeetCode周赛leetcode算法职场和发展
1.修改矩阵(模拟)classSolution{public:vector>modifiedMatrix(vector>&matrix){intn=matrix.size();intm=matrix[0].size();vectorans(m);for(inti=0;i&nums,vector&pattern){intn=nums.size();intm=pattern.size();intans
- [LeetCode周赛复盘] 第 384 场周赛20240211
七水shuliang
力扣周赛复盘leetcode算法职场和发展
[LeetCode周赛复盘]第384场周赛20240211一、本周周赛总结100230.修改矩阵1.题目描述2.思路分析3.代码实现100219.回文字符串的最大数量1.题目描述2.思路分析3.代码实现100198.匹配模式数组的子数组数目II1.题目描述2.思路分析3.代码实现参考链接一、本周周赛总结赛后半小时才做出T4,比赛时就没交T1模拟。T3贪心。T2/T4Z函数/KMP。100230.修
- leetcode周赛打卡题
weixin_44235070
总结
第179-180场周赛灯泡开关(1375题):所有连续的灯是左边连续的一段,即数组的前缀构成连续的一段。通知所有员工所需的时间(1376题):dfs的方法。T秒后青蛙的位置(1377题):dfs或者bfs的方法。矩阵中的幸运数(1380题)增量的栈(1381题)将二叉搜索树变平衡(1382题):1.dfs遍历生成有序数组;2.将有序数组变成平衡二叉树。最大的团队表现值(1383题):小根堆第29场
- 2020-03-15时间记录
296b871d5cd0
(1)8:00-10:30划水(2)10:30-11:06写leetcode周赛(3)11:06-13:52划水。。。(4)13:52-14:17写时时发布项目,emmmm这是不是体现了代码积累的重要性,哈哈哈。看来我之前对代码的复盘确实不够。感觉也写了许多代码了,但很多都没用过了。以后要好好梳理出来,填入我的个人代码库中。(5)14:17-写创新实践项目今日任务表:时时发布项目创新实践项目根据简
- 复盘成长——2024.1月复盘
吴代庄
未来规划复盘javaleetcode职场和发展学习
复盘的目的和范围每月一次的精心复盘,不仅是对自身工作成就、学习进步与成长轨迹的深度梳理,更是一种对未来路径的细致规划。通过这一过程,我期望能够更加精准地把握生活的节奏和职场的航向,从中汲取经验、聚焦目标,并以此为契机,迈向更加辉煌璀璨的未来。本月的目标与成果1.文章输出目标:每月输出至少1篇文章完成情况:共输出21篇文章,其中11篇技术文章,3篇读书笔记,3篇LeetCode周赛笔记,1篇复盘文章
- 2020-01-19时间记录
296b871d5cd0
(1)9:00-10:14起床洗脸吃饭看漫画问学车的事上厕所(2)10:14-10:21刷牙(3)10:21-12:00leetcode周赛(4)12:00-12:33吃饭(5)12:33-15:00划水和配眼镜(6)15:00-15:20继续刷leetcode周赛没做出来的最后一题,做出来了,果然是贪心算法,早上前面花的时间太长了,加上太久没写算法题,生疏了。(7)15:20-19:11搞一下时
- leetcode周赛373场
菜菜小堡
leetcode算法排序算法
leetcode周赛373场第三题2948题评论区的解题思路找到了很好的解法,当时没有想到,给原始数组排序后,分段再给数组位置排序。classSolution{publicint[]lexicographicallySmallestArray(int[]nums,intlimit){intn=nums.length;Integer[]ids=newInteger[n];for(inti=0;inu
- 2020-01-26时间记录
296b871d5cd0
(1)8:30-10:30起床看小说(2)10:30-11:30leetcode周赛,只做了两题,第三题图论忘记怎么做了,第四题估计是贪心或动态规划思考不出。。看来算法是要好好加强了。(3)11:30-12:15休息(4)12:15-看超实用笔记读书法emmm又划水了。。。服务外包项目再说吧。又没啥钱。明天找个vue视频项目看看。然后实习计划要提上日程了,其他一切都不重要,目前最重要的就是找到实习
- 字符串题目杂记
cwtnice
算法学习字符串
前言:主要是字符串处理的题目,有时候感觉很简单却处理不好-=这里就作为我的字符串题目笔记吧,主要来自于leetcode周赛的第一题。题目就放英文吧,也锻炼一下英文阅读能力,小陈,加油!——2021.8.8LeetCode5838.检查字符串是否为数组前缀(check-if-string-is-a-prefix-of-array)Givenastringsandanarrayofstringswor
- 【LeetCode周赛】第379场周赛
爱喝牛奶的男孩
LeetCodeleetcode算法职场和发展
LeetCode第379场周赛100170.对角线最长的矩形的面积简单100187.捕获黑皇后需要的最少移动次数中等100150.移除后集合的最多元素数中等100170.对角线最长的矩形的面积简单100170.对角线最长的矩形的面积分析:根据题意模拟即可。遍历数组,计算矩形对角线长度。如果对角线更长,则更新新的矩形面积。如果对角线一样长,则判断是否新的矩形面积更大代码:classSolution{
- 【LeetCode周赛】第373场周赛
爱喝牛奶的男孩
LeetCodeleetcode算法
LeetCode第373场周赛2946.循环移位后的矩阵相似检查简单2947.统计美丽子字符串I中等2948.交换得到字典序最小的数组中等2949.统计美丽子字符串II困难2946.循环移位后的矩阵相似检查简单2946.循环移位后的矩阵相似检查分析:对于循环位移k,当k=1k=1k=1,此时是向左或者向右循环移动、当k=n+1k=n+1k=n+1,此时与k=1k=1k=1情况类似,最终也是想左或向
- [LeetCode周赛复盘] 第 379 场周赛20240107
七水shuliang
力扣周赛复盘leetcode算法职场和发展
[LeetCode周赛复盘]第379场周赛20240107一、本周周赛总结100170.对角线最长的矩形的面积1.题目描述2.思路分析3.代码实现100187.捕获黑皇后需要的最少移动次数1.题目描述2.思路分析3.代码实现100150.移除后集合的最多元素数1.题目描述2.思路分析3.代码实现100154.执行操作后的最大分割数量1.题目描述2.思路分析3.代码实现参考链接一、本周周赛总结赛后半
- 关于0 1背包问题的详细解读-(不断更新中)
暗=里
算法
1.前言第368场leetcode周赛第二题使用到了01背包问题的解法,由于当时对01背包问题理解不是很透彻,导致这题丢分。在b站上看了启蒙课程再加上自己理解,于是有了这篇博客,一是方便自己复习总结,二是为算法小白提供帮助。2.状态方程及其推导的说明01背包的状态方程是:dp[i][j]=MAX{dp[i−1][j],dp[i−1][j−weight[i]]+value[i]}dp[i][j]=M
- leetcode周赛 第 370 场周赛
Stray_Lambs
leetcode算法
2923.找到冠军I一场比赛中共有n支队伍,按从0到n-1编号。给你一个下标从0开始、大小为n*n的二维布尔矩阵grid。对于满足0>mp=newArrayList>();privateint[]v;publiclongmaximumScoreAfterOperations(int[][]edges,int[]values){v=values;mp.clear();intn=edges.lengt
- [LeetCode周赛复盘] 第 299 场周赛20220626
英雄星球七水请求出战
@[TOC]([LeetCode周赛复盘]第299场周赛20220626)一、本周周赛总结再次感觉到自己的菜。最后一题图论,是真的不会,打死都不会。赛后看大佬讲解,照着大佬代码复写,大概理解了,但是再给我一道新题,估计还是做不出来。得练。在这里插入图片描述二、[Easy]6101.判断矩阵是否是一个X矩阵链接:6101.判断矩阵是否是一个X矩阵1.题目描述判断矩阵是否是一个X矩阵难度:简单如果一个
- [LeetCode周赛复盘] 第 119 场双周赛20231209
七水shuliang
力扣周赛复盘leetcode算法职场和发展
[LeetCode周赛复盘]第119场双周赛20231209一、本周周赛总结100130.找到两个数组中的公共元素1.题目描述2.思路分析3.代码实现100152.消除相邻近似相等字符1.题目描述2.思路分析3.代码实现100147.最多K个重复元素的最长子数组1.题目描述2.思路分析3.代码实现100140.关闭分部的可行集合数目1.题目描述2.思路分析3.代码实现参考链接一、本周周赛总结T1模
- [LeetCode周赛复盘] 第 375 场周赛20231210
七水shuliang
力扣周赛复盘leetcode算法职场和发展
[LeetCode周赛复盘]第375场周赛20231210一、本周周赛总结100143.统计已测试设备1.题目描述2.思路分析3.代码实现100155.双模幂运算1.题目描述2.思路分析3.代码实现100137.统计最大元素出现至少K次的子数组1.题目描述2.思路分析3.代码实现100136.统计好分割方案的数目1.题目描述2.思路分析3.代码实现参考链接一、本周周赛总结T1模拟。T2快速幂模拟。
- [LeetCode周赛复盘] 第 374 场周赛20231203
七水shuliang
力扣周赛复盘leetcode算法职场和发展
[LeetCode周赛复盘]第374场周赛20231203一、本周周赛总结100144.找出峰值1.题目描述2.思路分析3.代码实现100153.需要添加的硬币的最小数量1.题目描述2.思路分析3.代码实现100145.统计完全子字符串1.题目描述2.思路分析3.代码实现100146.统计感冒序列的数目1.题目描述2.思路分析3.代码实现参考链接一、本周周赛总结比赛后才做出T3,没敢交T1模拟。T
- [LeetCode周赛复盘] 第 376 场周赛20231217
七水shuliang
力扣周赛复盘leetcode算法职场和发展
[LeetCode周赛复盘]第376场周赛20231217一、本周周赛总结100149.找出缺失和重复的数字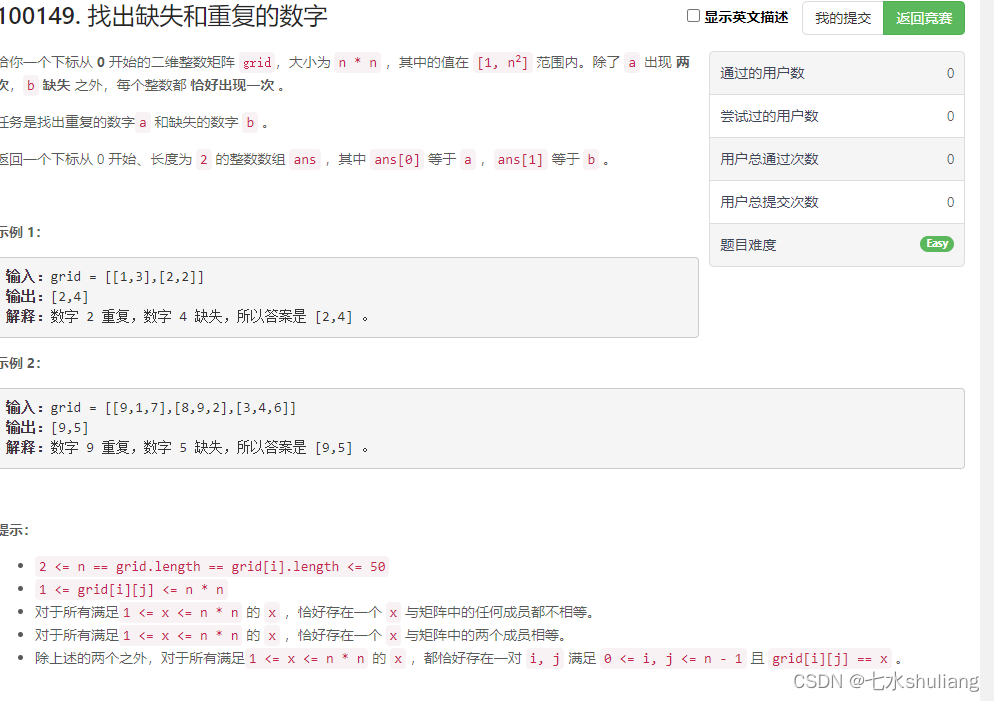2.思路分析3.代码实现100161.划分数组并满足最大差限制1.题目描述2.思路分析3.代码实现100151.使数组成为
- Leetcode周赛374补题(3 / 3) - EA专场
Roye_ack
leetcode周赛leetcode算法职场和发展贪心java滑动窗口分组循环
不愧是EA的题,我最爱的模拟人生……好难,呜呜目录1、找出峰值-暴力枚举2、需要添加的硬币的最小数量-思维+贪心3、统计完全子字符串-滑窗+分组循环1、找出峰值-暴力枚举2951.找出峰值classSolution{publicListfindPeaks(int[]m){Listres=newArrayListm[i+1])res.add(i);returnres;}}2、需要添加的硬币的最小数量
- LeetCode周赛第184场 5382. HTML 实体解析器
岛屿绕城
LeetCodeleetcodehtml字符串
题目链接「HTML实体解析器」是一种特殊的解析器,它将HTML代码作为输入,并用字符本身替换掉所有这些特殊的字符实体。HTML里这些特殊字符和它们对应的字符实体包括:双引号:字符实体为",对应的字符是"。单引号:字符实体为',对应的字符是'。与符号:字符实体为&,对应对的字符是&。大于号:字符实体为>,对应的字符是>。小于号:字符实体为<,对应的字符是y&&
- 第四题-abb 【第六届传智杯程序设计挑战赛解题分析详解复盘】(Java&Python&C++实现)
一见已难忘
ACM算法题库javapythonc++abb传智杯abb传智杯
欢迎来到ACM算法题库专栏在ACM算法题库专栏,热情推崇算法之美,精心整理了各类比赛题目的详细解法,包括但不限于ICPC、CCPC、蓝桥杯、LeetCode周赛、传智杯等等。无论您是刚刚踏入算法领域,还是经验丰富的竞赛选手,这里都是提升技能和知识的理想之地。✨经典必会题目:我们提供了精选的算法学习必会题目,帮助您构建坚实的算法基础。✨详细题目解法:每道题目都附带了详尽的解法,帮助您理解并掌握解题思
- 第五题-kotori和素因子【第六届传智杯程序设计挑战赛解题分析详解复盘】(Java&Python&C++实现)
一见已难忘
ACM算法题库javapythonc++kotori和素因子传智杯
欢迎来到ACM算法题库专栏在ACM算法题库专栏,热情推崇算法之美,精心整理了各类比赛题目的详细解法,包括但不限于ICPC、CCPC、蓝桥杯、LeetCode周赛、传智杯等等。无论您是刚刚踏入算法领域,还是经验丰富的竞赛选手,这里都是提升技能和知识的理想之地。✨经典必会题目:我们提供了精选的算法学习必会题目,帮助您构建坚实的算法基础。✨详细题目解法:每道题目都附带了详尽的解法,帮助您理解并掌握解题思
- 第六题-红和蓝【第六届传智杯程序设计挑战赛解题分析详解复盘】(Java&Python&C++实现)
一见已难忘
ACM算法题库javapythonc++红和蓝传智杯红和蓝传智杯
欢迎来到ACM算法题库专栏在ACM算法题库专栏,热情推崇算法之美,精心整理了各类比赛题目的详细解法,包括但不限于ICPC、CCPC、蓝桥杯、LeetCode周赛、传智杯等等。无论您是刚刚踏入算法领域,还是经验丰富的竞赛选手,这里都是提升技能和知识的理想之地。✨经典必会题目:我们提供了精选的算法学习必会题目,帮助您构建坚实的算法基础。✨详细题目解法:每道题目都附带了详尽的解法,帮助您理解并掌握解题思
- 第一题-字符串拼接【第六届传智杯程序设计挑战赛解题分析详解复盘】(C/C++实现)
一见已难忘
ACM算法题库c语言c++开发语言字符串拼接传智杯
欢迎来到ACM算法题库专栏在ACM算法题库专栏,热情推崇算法之美,精心整理了各类比赛题目的详细解法,包括但不限于ICPC、CCPC、蓝桥杯、LeetCode周赛、传智杯等等。无论您是刚刚踏入算法领域,还是经验丰富的竞赛选手,这里都是提升技能和知识的理想之地。✨经典必会题目:我们提供了精选的算法学习必会题目,帮助您构建坚实的算法基础。✨详细题目解法:每道题目都附带了详尽的解法,帮助您理解并掌握解题思
- 3.红色和紫色-【第六届传智杯程序设计挑战赛解题分析详解复盘】(Java&Python&C++实现)
一见已难忘
ACM算法题库javapythonc++传智杯红色和紫色
欢迎来到ACM算法题库专栏在ACM算法题库专栏,热情推崇算法之美,精心整理了各类比赛题目的详细解法,包括但不限于ICPC、CCPC、蓝桥杯、LeetCode周赛、传智杯等等。无论您是刚刚踏入算法领域,还是经验丰富的竞赛选手,这里都是提升技能和知识的理想之地。✨经典必会题目:我们提供了精选的算法学习必会题目,帮助您构建坚实的算法基础。✨详细题目解法:每道题目都附带了详尽的解法,帮助您理解并掌握解题思
- leetcode周赛5418.二叉树中的伪回文路径
CPeony
leetcode
leetcode周赛5418.二叉树中的伪回文路径给你一棵二叉树,每个节点的值为1到9。我们称二叉树中的一条路径是「伪回文」的,当它满足:路径经过的所有节点值的排列中,存在一个回文序列。请你返回从根到叶子节点的所有路径中伪回文路径的数目。示例1输入:root=[2,3,1,3,1,null,1]输出:2解释:上图为给定的二叉树。总共有3条从根到叶子的路径:红色路径[2,3,3],绿色路径[2,1,
- Leetcode周赛--2021.8.15
譕訫_
Java学习Leetcode周赛leetcodejava
emmm,昨天忘记今早实验室上午断电,没带电脑回宿舍,实验室没电自然也打不开指纹锁,所以在得知断电之后,直接取消报名睡大觉zzz下午补上今早的周赛题解,emm总体还是比较简单的,前三道都挺基础的,第四道还是没看。。。第一题—5843.作为子字符串出现在单词中的字符串数目思路判断patterns数组内的元素有多少个是字符串word的子字符串,因为求的是连续的子字符串而不是子序列,所以直接使用Stri
- html
周华华
html
js
1,数组的排列
var arr=[1,4,234,43,52,];
for(var x=0;x<arr.length;x++){
for(var y=x-1;y<arr.length;y++){
if(arr[x]<arr[y]){
&
- 【Struts2 四】Struts2拦截器
bit1129
struts2拦截器
Struts2框架是基于拦截器实现的,可以对某个Action进行拦截,然后某些逻辑处理,拦截器相当于AOP里面的环绕通知,即在Action方法的执行之前和之后根据需要添加相应的逻辑。事实上,即使struts.xml没有任何关于拦截器的配置,Struts2也会为我们添加一组默认的拦截器,最常见的是,请求参数自动绑定到Action对应的字段上。
Struts2中自定义拦截器的步骤是:
- make:cc 命令未找到解决方法
daizj
linux命令未知make cc
安装rz sz程序时,报下面错误:
[root@slave2 src]# make posix
cc -O -DPOSIX -DMD=2 rz.c -o rz
make: cc:命令未找到
make: *** [posix] 错误 127
系统:centos 6.6
环境:虚拟机
错误原因:系统未安装gcc,这个是由于在安
- Oracle之Job应用
周凡杨
oracle job
最近写服务,服务上线后,需要写一个定时执行的SQL脚本,清理并更新数据库表里的数据,应用到了Oracle 的 Job的相关知识。在此总结一下。
一:查看相关job信息
1、相关视图
dba_jobs
all_jobs
user_jobs
dba_jobs_running 包含正在运行
- 多线程机制
朱辉辉33
多线程
转至http://blog.csdn.net/lj70024/archive/2010/04/06/5455790.aspx
程序、进程和线程:
程序是一段静态的代码,它是应用程序执行的蓝本。进程是程序的一次动态执行过程,它对应了从代码加载、执行至执行完毕的一个完整过程,这个过程也是进程本身从产生、发展至消亡的过程。线程是比进程更小的单位,一个进程执行过程中可以产生多个线程,每个线程有自身的
- web报表工具FineReport使用中遇到的常见报错及解决办法(一)
老A不折腾
web报表finereportjava报表报表工具
FineReport使用中遇到的常见报错及解决办法(一)
这里写点抛砖引玉,希望大家能把自己整理的问题及解决方法晾出来,Mark一下,利人利己。
出现问题先搜一下文档上有没有,再看看度娘有没有,再看看论坛有没有。有报错要看日志。下面简单罗列下常见的问题,大多文档上都有提到的。
1、address pool is full:
含义:地址池满,连接数超过并发数上
- mysql rpm安装后没有my.cnf
林鹤霄
没有my.cnf
Linux下用rpm包安装的MySQL是不会安装/etc/my.cnf文件的,
至于为什么没有这个文件而MySQL却也能正常启动和作用,在这儿有两个说法,
第一种说法,my.cnf只是MySQL启动时的一个参数文件,可以没有它,这时MySQL会用内置的默认参数启动,
第二种说法,MySQL在启动时自动使用/usr/share/mysql目录下的my-medium.cnf文件,这种说法仅限于r
- Kindle Fire HDX root并安装谷歌服务框架之后仍无法登陆谷歌账号的问题
aigo
root
原文:http://kindlefireforkid.com/how-to-setup-a-google-account-on-amazon-fire-tablet/
Step 4: Run ADB command from your PC
On the PC, you need install Amazon Fire ADB driver and instal
- javascript 中var提升的典型实例
alxw4616
JavaScript
// 刚刚在书上看到的一个小问题,很有意思.大家一起思考下吧
myname = 'global';
var fn = function () {
console.log(myname); // undefined
var myname = 'local';
console.log(myname); // local
};
fn()
// 上述代码实际上等同于以下代码
m
- 定时器和获取时间的使用
百合不是茶
时间的转换定时器
定时器:定时创建任务在游戏设计的时候用的比较多
Timer();定时器
TImerTask();Timer的子类 由 Timer 安排为一次执行或重复执行的任务。
定时器类Timer在java.util包中。使用时,先实例化,然后使用实例的schedule(TimerTask task, long delay)方法,设定
- JDK1.5 Queue
bijian1013
javathreadjava多线程Queue
JDK1.5 Queue
LinkedList:
LinkedList不是同步的。如果多个线程同时访问列表,而其中至少一个线程从结构上修改了该列表,则它必须 保持外部同步。(结构修改指添加或删除一个或多个元素的任何操作;仅设置元素的值不是结构修改。)这一般通过对自然封装该列表的对象进行同步操作来完成。如果不存在这样的对象,则应该使用 Collections.synchronizedList 方
- http认证原理和https
bijian1013
httphttps
一.基础介绍
在URL前加https://前缀表明是用SSL加密的。 你的电脑与服务器之间收发的信息传输将更加安全。
Web服务器启用SSL需要获得一个服务器证书并将该证书与要使用SSL的服务器绑定。
http和https使用的是完全不同的连接方式,用的端口也不一样,前者是80,后
- 【Java范型五】范型继承
bit1129
java
定义如下一个抽象的范型类,其中定义了两个范型参数,T1,T2
package com.tom.lang.generics;
public abstract class SuperGenerics<T1, T2> {
private T1 t1;
private T2 t2;
public abstract void doIt(T
- 【Nginx六】nginx.conf常用指令(Directive)
bit1129
Directive
1. worker_processes 8;
表示Nginx将启动8个工作者进程,通过ps -ef|grep nginx,会发现有8个Nginx Worker Process在运行
nobody 53879 118449 0 Apr22 ? 00:26:15 nginx: worker process
- lua 遍历Header头部
ronin47
lua header 遍历
local headers = ngx.req.get_headers()
ngx.say("headers begin", "<br/>")
ngx.say("Host : ", he
- java-32.通过交换a,b中的元素,使[序列a元素的和]与[序列b元素的和]之间的差最小(两数组的差最小)。
bylijinnan
java
import java.util.Arrays;
public class MinSumASumB {
/**
* Q32.有两个序列a,b,大小都为n,序列元素的值任意整数,无序.
*
* 要求:通过交换a,b中的元素,使[序列a元素的和]与[序列b元素的和]之间的差最小。
* 例如:
* int[] a = {100,99,98,1,2,3
- redis
开窍的石头
redis
在redis的redis.conf配置文件中找到# requirepass foobared
把它替换成requirepass 12356789 后边的12356789就是你的密码
打开redis客户端输入config get requirepass
返回
redis 127.0.0.1:6379> config get requirepass
1) "require
- [JAVA图像与图形]现有的GPU架构支持JAVA语言吗?
comsci
java语言
无论是opengl还是cuda,都是建立在C语言体系架构基础上的,在未来,图像图形处理业务快速发展,相关领域市场不断扩大的情况下,我们JAVA语言系统怎么从这么庞大,且还在不断扩大的市场上分到一块蛋糕,是值得每个JAVAER认真思考和行动的事情
- 安装ubuntu14.04登录后花屏了怎么办
cuiyadll
ubuntu
这个情况,一般属于显卡驱动问题。
可以先尝试安装显卡的官方闭源驱动。
按键盘三个键:CTRL + ALT + F1
进入终端,输入用户名和密码登录终端:
安装amd的显卡驱动
sudo
apt-get
install
fglrx
安装nvidia显卡驱动
sudo
ap
- SSL 与 数字证书 的基本概念和工作原理
darrenzhu
加密ssl证书密钥签名
SSL 与 数字证书 的基本概念和工作原理
http://www.linuxde.net/2012/03/8301.html
SSL握手协议的目的是或最终结果是让客户端和服务器拥有一个共同的密钥,握手协议本身是基于非对称加密机制的,之后就使用共同的密钥基于对称加密机制进行信息交换。
http://www.ibm.com/developerworks/cn/webspher
- Ubuntu设置ip的步骤
dcj3sjt126com
ubuntu
在单位的一台机器完全装了Ubuntu Server,但回家只能在XP上VM一个,装的时候网卡是DHCP的,用ifconfig查了一下ip是192.168.92.128,可以ping通。
转载不是错:
Ubuntu命令行修改网络配置方法
/etc/network/interfaces打开后里面可设置DHCP或手动设置静态ip。前面auto eth0,让网卡开机自动挂载.
1. 以D
- php包管理工具推荐
dcj3sjt126com
PHPComposer
http://www.phpcomposer.com/
Composer是 PHP 用来管理依赖(dependency)关系的工具。你可以在自己的项目中声明所依赖的外部工具库(libraries),Composer 会帮你安装这些依赖的库文件。
中文文档
入门指南
下载
安装包列表
Composer 中国镜像
- Gson使用四(TypeAdapter)
eksliang
jsongsonGson自定义转换器gsonTypeAdapter
转载请出自出处:http://eksliang.iteye.com/blog/2175595 一.概述
Gson的TypeAapter可以理解成自定义序列化和返序列化 二、应用场景举例
例如我们通常去注册时(那些外国网站),会让我们输入firstName,lastName,但是转到我们都
- JQM控件之Navbar和Tabs
gundumw100
htmlxmlcss
在JQM中使用导航栏Navbar是简单的。
只需要将data-role="navbar"赋给div即可:
<div data-role="navbar">
<ul>
<li><a href="#" class="ui-btn-active&qu
- 利用归并排序算法对大文件进行排序
iwindyforest
java归并排序大文件分治法Merge sort
归并排序算法介绍,请参照Wikipeida
zh.wikipedia.org/wiki/%E5%BD%92%E5%B9%B6%E6%8E%92%E5%BA%8F
基本思想:
大文件分割成行数相等的两个子文件,递归(归并排序)两个子文件,直到递归到分割成的子文件低于限制行数
低于限制行数的子文件直接排序
两个排序好的子文件归并到父文件
直到最后所有排序好的父文件归并到输入
- iOS UIWebView URL拦截
啸笑天
UIWebView
本文译者:candeladiao,原文:URL filtering for UIWebView on the iPhone说明:译者在做app开发时,因为页面的javascript文件比较大导致加载速度很慢,所以想把javascript文件打包在app里,当UIWebView需要加载该脚本时就从app本地读取,但UIWebView并不支持加载本地资源。最后从下文中找到了解决方法,第一次翻译,难免有
- 索引的碎片整理SQL语句
macroli
sql
SET NOCOUNT ON
DECLARE @tablename VARCHAR (128)
DECLARE @execstr VARCHAR (255)
DECLARE @objectid INT
DECLARE @indexid INT
DECLARE @frag DECIMAL
DECLARE @maxfrag DECIMAL
--设置最大允许的碎片数量,超过则对索引进行碎片
- Angularjs同步操作http请求with $promise
qiaolevip
每天进步一点点学习永无止境AngularJS纵观千象
// Define a factory
app.factory('profilePromise', ['$q', 'AccountService', function($q, AccountService) {
var deferred = $q.defer();
AccountService.getProfile().then(function(res) {
- hibernate联合查询问题
sxj19881213
sqlHibernateHQL联合查询
最近在用hibernate做项目,遇到了联合查询的问题,以及联合查询中的N+1问题。
针对无外键关联的联合查询,我做了HQL和SQL的实验,希望能帮助到大家。(我使用的版本是hibernate3.3.2)
1 几个常识:
(1)hql中的几种join查询,只有在外键关联、并且作了相应配置时才能使用。
(2)hql的默认查询策略,在进行联合查询时,会产
- struts2.xml
wuai
struts
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE struts PUBLIC
"-//Apache Software Foundation//DTD Struts Configuration 2.3//EN"
"http://struts.apache