【SpringBoot】DEMO:上传头像,路径存入数据库,展示到页面上(解决了重启服务器才可以显示图片的问题)
- 零、效果展示
- 一、分析需求
- 二、工具准备
- 三、功能实现
- 四、github链接
零、效果展示
- 需要原码的小伙伴,找点击github链接,原码+数据表文件已上传到github
一、分析需求
- 上传图片到服务器指定的文件夹
- 把图片的路径和名称存入数据库,图片名称加密
- 在html页面通过id搜索查询图片(不需要重启服务器,立即显示)
二、工具准备
- IDEA
- mysql 5.7
- SpringBoot
- Thymeleaf
三、功能实现
- 数据库设计
- 新建 head 表,字段如下
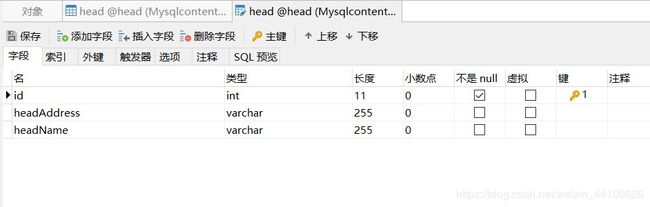
- 文件目录
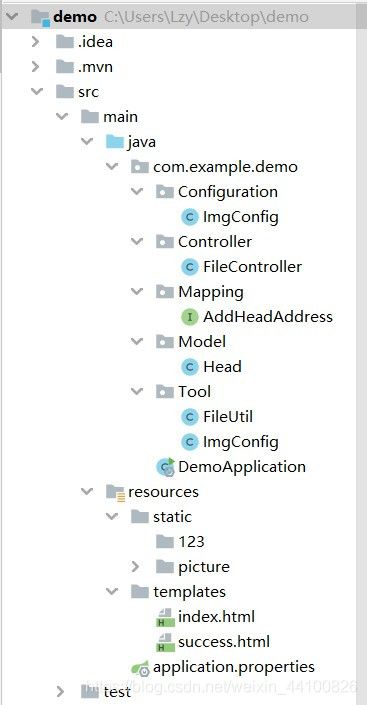
- maven依赖
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.2.4.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.example</groupId>
<artifactId>demo</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>demo</name>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web-services</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.session</groupId>
<artifactId>spring-session-core</artifactId>
</dependency>
<!--mybatis-->
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>1.3.1</version>
</dependency>
<!--整合mysql-->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
<!--json @responseBody/@requestBody-->
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>fastjson</artifactId>
<version>1.2.54</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
<exclusions>
<exclusion>
<groupId>org.junit.vintage</groupId>
<artifactId>junit-vintage-engine</artifactId>
</exclusion>
</exclusions>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
- 建立两个HTML页面,一个为index上传页面,一个为success展示图片页面
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>上传图片展示区title>
head>
<body>
选择图片:
<form enctype="multipart/form-data" method="post" action="/doUpload">
<input type="file" name="file"/>
<input type="submit" value="上传">
form>
<span th:text="${error}">span>
body>
html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>上传成功title>
head>
<body>
上传成功
<form method="post" action="/showHead">
你要查询的id
<input name="id" type="text">
<button name="doShow" type="submit" value="查询">查询button>
form>
<div th:each="head:${byId}">
<span th:text="${head.getId()}">span><br>
<span th:text="${head.getHeadAddress()}">span><br>
<img th:src="@{'/picture/'+${head.getHeadName()}}">img>
div>
body>
html>
- 创建一个配置类,接入虚拟路径(解决重启服务器才显示图片的问题)
@Configuration
public class ImgConfig implements WebMvcConfigurer {
@Override
public void addResourceHandlers(ResourceHandlerRegistry registry) {
registry.addResourceHandler("/picture/**").addResourceLocations("file:" + "C:/Users/Lzy/Desktop/demo/src/main/resources/static/picture/");
}
}
- 控制器Controller,注释写得十分清楚
- 防止文件名相同而出现二义性,使用 UUID自动生成的密钥 与 文件名称 进行拼接
@Controller
public class FileController {
@Autowired
private AddHeadAddress addHeadAddress;
@GetMapping("/")
public String goUploadImg(){
return "index";
}
@PostMapping(value = "/doUpload")
public String uploadImg(
@RequestParam("file") MultipartFile file,
Model model){
if ((file.getOriginalFilename().isEmpty() )) {
model.addAttribute("error","error");
return "index";
}else{
Head head = new Head();
String fileName = file.getOriginalFilename();
String hToken = UUID.randomUUID().toString();
String HeadName = hToken + fileName;
String filePath = "C:\\Users\\Lzy\\Desktop\\demo\\src\\main\\resources\\static\\picture\\";
String fileAddress = filePath + HeadName;
try{
FileUtil.uploadFile(file.getBytes(),filePath,HeadName);
head.setHeadAddress(fileAddress);
head.setHeadName(HeadName);
addHeadAddress.insert(head);
} catch (Exception e){
}
return "success";
}
}
@GetMapping("/showHead")
public String toShow(){
return "success";
}
@PostMapping(value = "/showHead")
public String showHead(
@RequestParam("id") Integer id,
Model model
){
List<Head> byId = addHeadAddress.getById(id);
model.addAttribute("byId",byId);
return "success";
}
}
- Mapping,数据持久化操作
@Mapper@Repository
public interface AddHeadAddress {
@Insert("insert into head(headAddress,headName) values (#{headAddress},#{headName})")
void insert(Head head);
@Select("SELECT * FROM head where (id) = (#{id})")
List<Head> getById(@Param("id") Integer id);
}
- Model层,与数据库的表字段一一对应
public class Head {
public String headAddress;
public Integer id;
public String headName;
public String getHeadName() {
return headName;
}
public void setHeadName(String headName) {
this.headName = headName;
}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getHeadAddress() {
return headAddress;
}
public void setHeadAddress(String headAddress) {
this.headAddress = headAddress;
}
}
- FileConfig,上传图片的工具类
public class FileUtil {
public static void uploadFile(byte[] file, String filePath, String fileName) throws Exception {
File targetFile = new File(filePath);
if(!targetFile.exists()){
targetFile.mkdirs();
}
FileOutputStream out = new FileOutputStream(filePath+fileName);
out.write(file);
out.flush();
out.close();
}
}
四、github链接