1.名词解释:
资源包:点击 LuaFramework | Build XXX(平台名) Resource,框架会自动将自定义指定的资源打包到StreamingAssets文件夹,这个文件夹下的unity3d文件就是资源包,它是一种u3d自己的压缩格式,也被称为AssetBundle包。
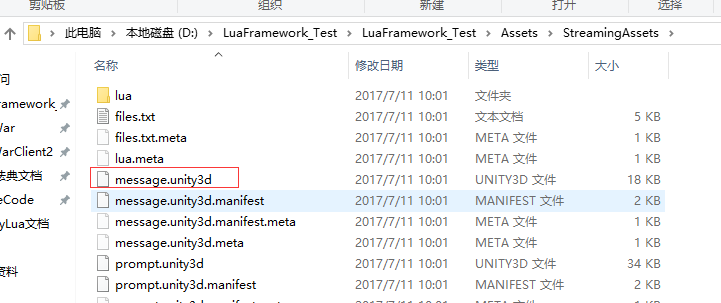
资源:资源经过打包成为资源包,如果在游戏里想用资源包里的内容的话,就需要先加载资源包到内存,然后解压这个资源包,获取资源包里包含的部分资源。
2.热更新涉及文件夹
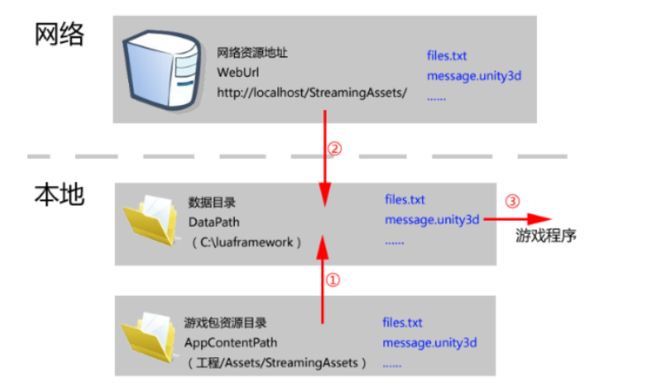
资源目录:apk文件(安卓安装文件)其实也是一种压缩格式,安装apk之后,安卓系统会为游戏自动创建一个资源目录,用来存放apk解压出来的东西。需要特别注意的一点是,资源目录里的东西是只能读不能写的,也就是说,我们不能随意地从网上下载文件替换资源目录里的资源,所以我们需要一个可读可写的目录以便我们用下载的资源替换原资源实现热更新。由于lua文件可以当作一种资源,所以lua里的代码也是可以热更新的。资源目录路径通过Util.AppContentPath()获取,安卓上大概是这样的:jar:file///data/app.com/com.XXX.XXX-1/base.apk!/assets/(root之后才能看到),用编辑器的话,是工程所在文件夹下的StreamingAssets。
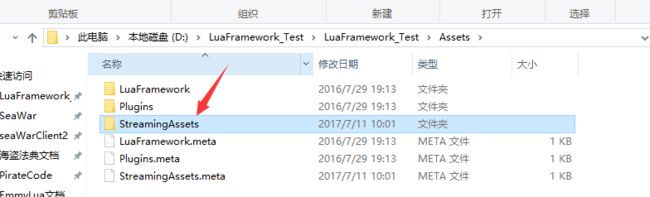
数据目录:安卓创建的用来存放游戏数据用的,可以随意读写。第一次打开游戏的时候,把资源包从资源目录复制到这里,此后每次启动游戏都会比较这里的文件和网络上的资源包,如果不一样的话,就下载下来替换这里的资源包。数据目录路径通过Util.DataPath获取,安卓上大概这样data/user/0/com.XXX.XXX-1/files/mygamename/(root之后才能看到),用编辑器的话,会创建在C盘。
网络资源目录:存放游戏资源的网址,游戏开启后,程序会从网络资源地址下载一些更新的文件到数据目录。版本控制是通过files.txt实现的,里面存放着资源文件的名称和md5码。程序会先下载“网络资源地址”上的files.txt,然后与“数据目录”中文件的md5码做比较,更新有变化的文件。
3.热更新的三个过程:
复制资源:将“游戏资源目录”的内容复制到“数据目录中”(只有在第一次打开游戏的时候会有此过程)
GameManager.OnExtractResource
1 IEnumerator OnExtractResource() { 2 string dataPath = Util.DataPath; //数据目录 3 string resPath = Util.AppContentPath(); //游戏包资源目录 4 5 if (Directory.Exists(dataPath)) Directory.Delete(dataPath, true); 6 Directory.CreateDirectory(dataPath); 7 8 string infile = resPath + "files.txt"; 9 string outfile = dataPath + "files.txt"; 10 if (File.Exists(outfile)) File.Delete(outfile); 11 12 string message = "正在解包文件:>files.txt"; 13 Debug.Log(infile); 14 Debug.Log(outfile); 15 if (Application.platform == RuntimePlatform.Android) { 16 WWW www = new WWW(infile); 17 yield return www; 18 19 if (www.isDone) { 20 File.WriteAllBytes(outfile, www.bytes); 21 } 22 yield return 0; 23 } else File.Copy(infile, outfile, true); 24 yield return new WaitForEndOfFrame(); 25 26 //释放所有文件到数据目录 27 string[] files = File.ReadAllLines(outfile); 28 foreach (var file in files) { 29 string[] fs = file.Split('|'); 30 infile = resPath + fs[0]; // 31 outfile = dataPath + fs[0]; 32 33 message = "正在解包文件:>" + fs[0]; 34 Debug.Log("正在解包文件:>" + infile); 35 facade.SendMessageCommand(NotiConst.UPDATE_MESSAGE, message); 36 37 string dir = Path.GetDirectoryName(outfile); 38 if (!Directory.Exists(dir)) Directory.CreateDirectory(dir); 39 40 if (Application.platform == RuntimePlatform.Android) { 41 WWW www = new WWW(infile); 42 yield return www; 43 44 if (www.isDone) { 45 File.WriteAllBytes(outfile, www.bytes); 46 } 47 yield return 0; 48 } else { 49 if (File.Exists(outfile)) { 50 File.Delete(outfile); 51 } 52 File.Copy(infile, outfile, true); 53 } 54 yield return new WaitForEndOfFrame(); 55 } 56 message = "解包完成!!!"; 57 facade.SendMessageCommand(NotiConst.UPDATE_MESSAGE, message); 58 yield return new WaitForSeconds(0.1f); 59 60 message = string.Empty; 61 //释放完成,开始启动更新资源 62 StartCoroutine(OnUpdateResource()); 63 }
下载资源:从网络比对下载资源
GameManager.OnUpdateResource
1 IEnumerator OnUpdateResource() { 2 if (!AppConst.UpdateMode) { 3 OnResourceInited(); 4 yield break; 5 } 6 string dataPath = Util.DataPath; //数据目录 7 string url = AppConst.WebUrl; 8 string message = string.Empty; 9 string random = DateTime.Now.ToString("yyyymmddhhmmss"); 10 string listUrl = url + "files.txt?v=" + random; 11 Debug.LogWarning("LoadUpdate---->>>" + listUrl); 12 13 WWW www = new WWW(listUrl); yield return www; 14 if (www.error != null) { 15 OnUpdateFailed(string.Empty); 16 yield break; 17 } 18 if (!Directory.Exists(dataPath)) { 19 Directory.CreateDirectory(dataPath); 20 } 21 File.WriteAllBytes(dataPath + "files.txt", www.bytes); 22 string filesText = www.text; 23 string[] files = filesText.Split('\n'); 24 25 for (int i = 0; i < files.Length; i++) { 26 if (string.IsNullOrEmpty(files[i])) continue; 27 string[] keyValue = files[i].Split('|'); 28 string f = keyValue[0]; 29 string localfile = (dataPath + f).Trim(); 30 string path = Path.GetDirectoryName(localfile); 31 if (!Directory.Exists(path)) { 32 Directory.CreateDirectory(path); 33 } 34 string fileUrl = url + f + "?v=" + random; 35 bool canUpdate = !File.Exists(localfile); 36 if (!canUpdate) { 37 string remoteMd5 = keyValue[1].Trim(); 38 string localMd5 = Util.md5file(localfile); 39 canUpdate = !remoteMd5.Equals(localMd5); 40 if (canUpdate) File.Delete(localfile); 41 } 42 if (canUpdate) { //本地缺少文件 43 Debug.Log(fileUrl); 44 message = "downloading>>" + fileUrl; 45 facade.SendMessageCommand(NotiConst.UPDATE_MESSAGE, message); 46 /* 47 www = new WWW(fileUrl); yield return www; 48 if (www.error != null) { 49 OnUpdateFailed(path); // 50 yield break; 51 } 52 File.WriteAllBytes(localfile, www.bytes); 53 */ 54 //这里都是资源文件,用线程下载 55 BeginDownload(fileUrl, localfile); 56 while (!(IsDownOK(localfile))) { yield return new WaitForEndOfFrame(); } 57 } 58 } 59 yield return new WaitForEndOfFrame(); 60 61 message = "更新完成!!"; 62 facade.SendMessageCommand(NotiConst.UPDATE_MESSAGE, message); 63 64 OnResourceInited(); 65 }
加载资源:加载资源包内的资源到内存
PanelManager.CreatePanel
1 public void CreatePanel(string name, LuaFunction func = null) { 2 string assetName = name + "Panel"; 3 string abName = name.ToLower() + AppConst.ExtName; 4 5 ResManager.LoadPrefab(abName, assetName, delegate(UnityEngine.Object[] objs) { 6 if (objs.Length == 0) return; 7 // Get the asset. 8 GameObject prefab = objs[0] as GameObject; 9 10 if (Parent.FindChild(name) != null || prefab == null) { 11 return; 12 } 13 GameObject go = Instantiate(prefab) as GameObject; 14 go.name = assetName; 15 go.layer = LayerMask.NameToLayer("Default"); 16 go.transform.SetParent(Parent); 17 go.transform.localScale = Vector3.one; 18 go.transform.localPosition = Vector3.zero; 19 go.AddComponent(); 20 21 if (func != null) func.Call(go); 22 Debug.LogWarning("CreatePanel::>> " + name + " " + prefab); 23 }); 24 }
4.可更新的loading界面
前提:
1)如果想更新,则必须把loading界面打包进资源包
2)在第一次打开游戏的时候,下载资源阶段之前,还没有loading界面的资源包,但是此刻无疑是必须显示loading的
3)生成loading界面的两种过程:
使用Resource.Load(不可热更新)
1 public void CreateLoadingPanelFromResource() 2 { 3 _MemoryPoolManager_ memMgr = AppFacade.Instance.GetManager<_MemoryPoolManager_>(ManagerName._Pool_); 4 5 Debug.Log("--------------------Resources加载loading--------------------------------"); 6 7 memMgr.AddPrefabPoolRS("LoadingPanel", 1, 1, true, null); 8 // memMgr.AddPrefabPoolAB ("loading", "LoadingPanel", 1, 1, true, null); 9 10 Transform loadingPanel = _MemoryPoolManager_.Spawn("LoadingPanel", true); 11 loadingPanel.SetParent(GameObject.Find("2DERoot/Resolution").transform); 12 loadingPanel.localPosition = Vector3.zero; 13 loadingPanel.localScale = new Vector3(1, 1, 1); 14 15 _slider = loadingPanel.FindChild("LoadingSlider").GetComponent(); 16 _text = loadingPanel.FindChild("LoadingSlider/Text").GetComponent (); 17 _text.gameObject.SetActive(true); 18 _text.transform.localPosition = Vector3.zero; 19 _slider.value = 5; 20 _text.text = "准备启动游戏"; 21 _isStartupLoading = true; 22 23 KeyFrameWork.MVC.SendEvent(FWConst.E_ShowLog, "Resources load finish");
使用加载AssetBundle资源包的方式
1 public IEnumerator CreateLoadingPanelFromAssetBundle() 2 { 3 string url = Util.GetRelativePath() + "loading.unity3d"; 4 Debug.Log("--------------------AssetBundle加载Loading--------------------------------" + url); 5 6 7 WWW www = WWW.LoadFromCacheOrDownload(url, 0, 0); 8 9 yield return www; 10 11 AssetBundle bundle = www.assetBundle; 12 GameObject asset = bundle.LoadAsset("LoadingPanel"); 13 Transform loadingPanel = Instantiate (asset).GetComponent (); 14 15 //memMgr.AddPrefabPoolRS("LoadingPanel", 1, 1, true, null); 16 //Transform loadingPanel = _MemoryPoolManager_.Spawn ("LoadingPanel", true); 17 18 19 20 loadingPanel.SetParent(GameObject.Find("2DERoot/Resolution").transform); 21 loadingPanel.localPosition = Vector3.zero; 22 loadingPanel.localScale = new Vector3(1, 1, 1); 23 loadingPanel.name = "LoadingPanel(Clone)001"; 24 25 26 _slider = loadingPanel.FindChild("LoadingSlider").GetComponent (); 27 _text = loadingPanel.FindChild("LoadingSlider/Text").GetComponent (); 28 _text.gameObject.SetActive(true); 29 _slider.value = 5; 30 31 _isStartupLoading = true; 32 33 //加载loading完成后再开始lua,保证lua能顺利接管loading 34 AppFacade.Instance.StartUp(); //启动游戏 35 36 KeyFrameWork.MVC.SendEvent(FWConst.E_ShowLog, "AssetBundle load finish"); 37 38 }
在游戏开始的时候使用判断(Directory.Exists(Util.DataPath) && Directory.Exists(Util.DataPath + "lua/") && File.Exists(Util.DataPath + "files.txt")来确定当前是否是第一次开启游戏,如果是,则使用Resource.Load,如果不是则使用加载AssetBundle资源包的方式。这里需要着重说下加载AssetBundle资源包时的顺序问题,由于ResourceManager需要Manifest关联文件(用以确定各个资源包之间的引用关系)进行初始化,所以ResourceManager初始化必在下载资源之后,而下载资源时无疑是需要显示loading的,故顺序是:加载AssetBundle资源包-》下载资源-》ResourceManager初始化。在这个顺序下,虽然ResourceManager有加载AssetBundle资源包的接口,但是不能用。而加载AssetBundle资源包是需要异步加载的,也就是说,显示loading需要1秒左右的时间(根据具体loading资源和手机硬件而定),若是需要在lua里接管loading(通过Find函数找到loading界面然后在lua里做一个引用),则必须在loading资源包加载完毕后才能执行lua接管loading的代码