基础图元的绘图过程
1. 画直线
private void Form_Paint(object sender, PaintEventArgs e)
{
Graphics g = this.CreateGraphics();
PointF p1 = new PointF(100, 100);
PointF p2 = new PointF(300, 300);
Pen pen =new Pen(Brushes.Red);
g.DrawLine(pen, p1, p2);
}
绘制效果,左上角为起点。
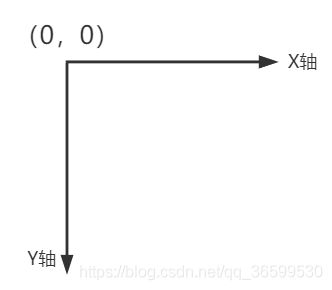
2. 画三角形
private void Form_Paint(object sender, PaintEventArgs e)
{
Graphics g = this.CreateGraphics();
Pen pen =new Pen(Brushes.Black);
PointF[] points = { new PointF(100, 100), new PointF(200, 100), new PointF(200, 300)
g.DrawPolygon(pen, points);
}
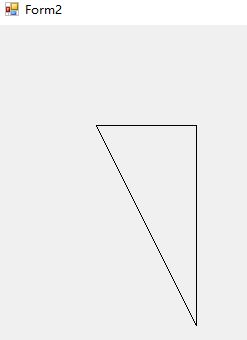
3. 画弧线
private void Form_Paint(object sender, PaintEventArgs e)
{
Graphics g = this.CreateGraphics();
Pen pen =new Pen(Brushes.Blue);
SizeF size = new SizeF(300, 300);
PointF p = new PointF(0, 0);
RectangleF rec = new RectangleF(p, size);
g.DrawArc(pen, rec, 0, 120);
}
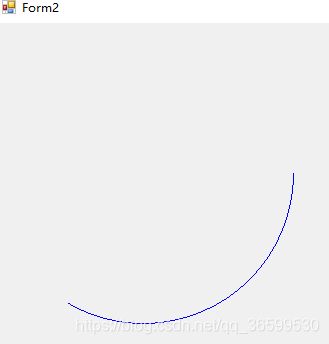
4. 画棱柱
private void Form_Paint(object sender, PaintEventArgs e)
{
Graphics g = this.CreateGraphics();
float width =150;
float height =150;
float x1 = 150;
float y1 = 150;
Pen pen =new Pen(Brushes.DarkGreen);
PointF[] points = { new PointF(x1, y1),
new PointF(x1 , y1 + height),
new PointF(x1 + 0.6f*width, y1+height),
new PointF(x1 + 0.6f*width, y1),
new PointF(x1 , y1),
new PointF(x1 - 0.2f * width, y1 - width*0.4f),
new PointF(x1 + 0.3f * width, y1 - width*0.8f),
new PointF(x1 + 0.8f * width, y1-width*0.4f),
new PointF(x1 + 0.6f*width, y1),
new PointF(x1 + 0.6f*width, y1+height),
new PointF(x1 + 0.8f*width, y1+height-width*0.4f),
new PointF(x1 + 0.8f * width, y1-width*0.4f),
new PointF(x1 + 0.6f*width, y1),
new PointF(x1 , y1),
new PointF(x1 , y1 + height),
new PointF(x1-0.2f*width , y1 + height-width*0.4f),
new PointF(x1 - 0.2f * width, y1 - width*0.4f)
};
g.DrawPolygon(pen, points);
pen.DashStyle = System.Drawing.Drawing2D.DashStyle.Dot;
PointF c = new PointF(x1 + 0.3f * width, y1 - width * 0.8f + height);
g.DrawLine(pen, c, points[6]);
g.DrawLine(pen, c, points[10]);
g.DrawLine(pen, c, points[15]);
}
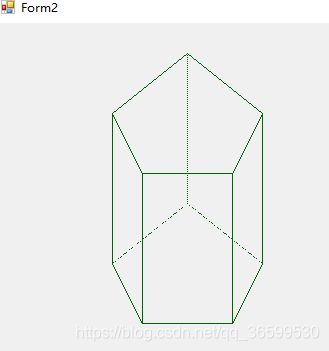
5. 画直尺
protected void ScaleMark(Graphics gra, float x1, float y1, float width, float height, int scale, bool isDown = false, float size = 9)
{
float xOffset = x1;
float yOffset = y1;
float offset = 0;
Pen pen = new Pen(Brushes.DarkGreen);
StringFormat strfmt = new StringFormat();
strfmt.Alignment = StringAlignment.Center;
for (int i = 0; i <= scale; i++)
{
offset += width / scale;
if (i % 10 == 0)
{
gra.DrawLine(pen,
new PointF(xOffset + offset, yOffset),
new PointF(xOffset + offset, yOffset + (isDown == false ? (10 + height / 5) : (-height / 5 - 10))));
gra.DrawString((i / 10).ToString(), new Font("宋体", size), Brushes.Black,
new PointF(xOffset + offset, yOffset + (isDown == false ? (+10 + height / 5) : (-20 - height / 5))),
strfmt);
}
else if (i % 5 == 0)
{
gra.DrawLine(pen,
new PointF(xOffset + offset, yOffset),
new PointF(xOffset + offset, yOffset + (isDown == false ? (7 + height / 8) : (-7 - height / 8))));
}
else
{
gra.DrawLine(pen,
new PointF(xOffset + offset, yOffset),
new PointF(xOffset + offset, yOffset + (isDown == false ? (+5 + height / 10) : (-5 - height / 10))));
}
}
}
private void Form_Paint(object sender, PaintEventArgs e)
{
Graphics g = this.CreateGraphics();
float x1 = 150;
float y1 = 150;
float size = 50;
Pen pen =new Pen(Brushes.DarkGreen);
float width = size * 10;
float height = size;
g.DrawPolygon(pen, new PointF[]
{
new PointF(x1,y1),
new PointF(x1+ width, y1),
new PointF(x1 + width, y1 + height),
new PointF(x1,y1 + height)
});
ScaleMark(g, x1 + 1, y1 , width - size / 2, height, 100, false);
}
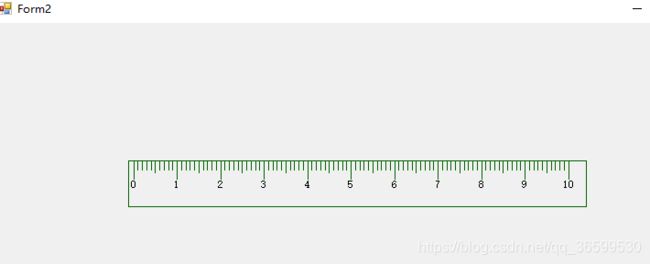
还要更多的绘图,读者可以自己试试,利用单纯的基础图形通过一定规则组合成较复杂的图形。