映入pom依赖:
org.apache.poi
poi-ooxml
4.0.1
org.apache.poi
poi
4.0.1
Ecel的信息实体类:
@Data
public class ExcelCellInfo {
private String fileName;
//表头
private String shellTitle;
//各个列的表头
private List heardList;
//需要填充的数据信息,map中的是每一个title-value
private List
模板生成工具类:
public class ExcelCreatUtil {
static List analysisExcelCell(Map> map){
List list = map.entrySet().stream().map(item ->{
String shellTitle = item.getKey();
Map info = item.getValue();
List cellTitle = info.containsKey("title") ? (List)info.get("title") : Lists.newArrayList();
List
测试接口:
/**
* 导出空白模板
* @param response 返回数据
*/
@GetMapping("/export")
public Object exportBlackList(HttpServletResponse response){
Map> info = new HashMap<>();
Map map1 = Maps.newHashMap();
map1.put("1-title1","1-1title1-1111");
map1.put("1-title2","1-1title2-2222");
map1.put("1-title3","1-1title3-3333");
map1.put("2-title1","2-2title1-1111");
map1.put("2-title2","2-2title2-2222");
map1.put("2-title3","2-2title3-3333");
Map map2 = Maps.newHashMap();
map2.put("1-title1","1-1title1-aaaa");
map2.put("1-title2","1-1title2-bbbb");
map2.put("1-title3","1-1title3-cccc");
map2.put("2-title1","2-2title1-eeee");
map2.put("2-title2","2-2title2-dddd");
map2.put("2-title3","2-2title3-ffff");
List> data = new ArrayList<>();
data.add(map1);
data.add(map2);
Map sheet1 = new HashMap<>();
List liat1 = Lists.newArrayList("1-title1", "1-title2", "1-title3");
sheet1.put("title",liat1);
sheet1.put("data", data);
info.put("sheet1",sheet1);
Map sheet2 = new HashMap<>();
List liat2 = Lists.newArrayList("2-title1", "2-title2", "2-title3");
sheet2.put("title",liat2);
sheet2.put("data", data);
info.put("sheet2",sheet2);
if(MapUtils.isEmpty(info)){
return "表格信息为空,请输入表格信息";
}
List list = ExcelCreatUtil.analysisExcelCell(info);
return new ExcelCreatUtil().creatExcel(response, list, "测试");
}
使用浏览器测试下载:
http://localhost:8080/demo/excel/export
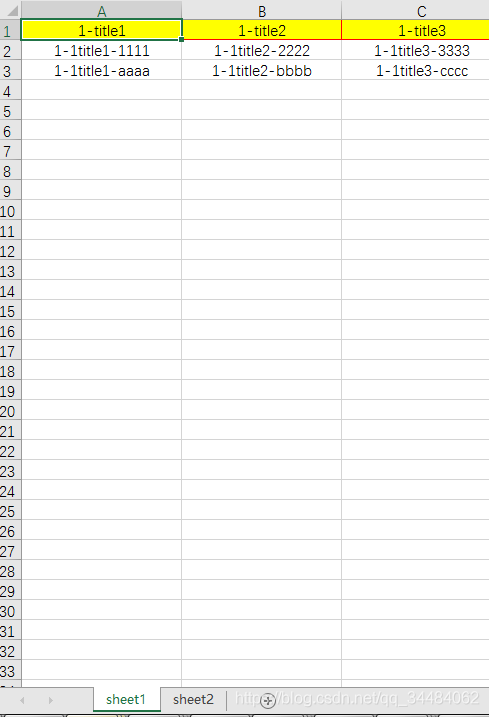