参考 keras.backend - 云+社区 - 腾讯云
Keras backend API.
一、Functions
- abs(...): Element-wise absolute value.
- all(...): Bitwise reduction (logical AND).
- any(...): Bitwise reduction (logical OR).
- arange(...): Creates a 1D tensor containing a sequence of integers.
- argmax(...): Returns the index of the maximum value along an axis.
- argmin(...): Returns the index of the minimum value along an axis.
- backend(...): Publicly accessible method for determining the current backend.
- batch_dot(...): Batchwise dot product.
- batch_flatten(...): Turn a nD tensor into a 2D tensor with same 0th dimension.
- batch_get_value(...): Returns the value of more than one tensor variable.
- batch_normalization(...): Applies batch normalization on x given mean, var, beta and gamma.
- batch_set_value(...): Sets the values of many tensor variables at once.
- bias_add(...): Adds a bias vector to a tensor.
- binary_crossentropy(...): Binary crossentropy between an output tensor and a target tensor.
- cast(...): Casts a tensor to a different dtype and returns it.
- cast_to_floatx(...): Cast a Numpy array to the default Keras float type.
- categorical_crossentropy(...): Categorical crossentropy between an output tensor and a target tensor.
- clear_session(...): Destroys the current TF graph and creates a new one.
- clip(...): Element-wise value clipping.
- concatenate(...): Concatenates a list of tensors alongside the specified axis.
- constant(...): Creates a constant tensor.
- conv1d(...): 1D convolution.
- conv2d(...): 2D convolution.
- conv2d_transpose(...): 2D deconvolution (i.e.
- conv3d(...): 3D convolution.
- cos(...): Computes cos of x element-wise.
- count_params(...): Returns the static number of elements in a variable or tensor.
- ctc_batch_cost(...): Runs CTC loss algorithm on each batch element.
- ctc_decode(...): Decodes the output of a softmax.
- ctc_label_dense_to_sparse(...): Converts CTC labels from dense to sparse.
- cumprod(...): Cumulative product of the values in a tensor, alongside the specified axis.
- cumsum(...): Cumulative sum of the values in a tensor, alongside the specified axis.
- dot(...): Multiplies 2 tensors (and/or variables) and returns a tensor.
- dropout(...): Sets entries in
x
to zero at random, while scaling the entire tensor.
- dtype(...): Returns the dtype of a Keras tensor or variable, as a string.
- elu(...): Exponential linear unit.
- epsilon(...): Returns the value of the fuzz factor used in numeric expressions.
- equal(...): Element-wise equality between two tensors.
- eval(...): Evaluates the value of a variable.
- exp(...): Element-wise exponential.
- expand_dims(...): Adds a 1-sized dimension at index "axis".
- eye(...): Instantiate an identity matrix and returns it.
- flatten(...): Flatten a tensor.
- floatx(...): Returns the default float type, as a string.
- foldl(...): Reduce elems using fn to combine them from left to right.
- foldr(...): Reduce elems using fn to combine them from right to left.
- function(...): Instantiates a Keras function.
- gather(...): Retrieves the elements of indices
indices
in the tensor reference
.
- get_uid(...): Associates a string prefix with an integer counter in a TensorFlow graph.
- get_value(...): Returns the value of a variable.
- gradients(...): Returns the gradients of
loss
w.r.t. variables
.
- greater(...): Element-wise truth value of (x > y).
- greater_equal(...): Element-wise truth value of (x >= y).
- hard_sigmoid(...): Segment-wise linear approximation of sigmoid.
- image_data_format(...): Returns the default image data format convention.
- in_test_phase(...): Selects
x
in test phase, and alt
otherwise.
- in_top_k(...): Returns whether the
targets
are in the top k
predictions
.
- in_train_phase(...): Selects
x
in train phase, and alt
otherwise.
- int_shape(...): Returns the shape of tensor or variable as a tuple of int or None entries.
- is_keras_tensor(...): Returns whether
x
is a Keras tensor.
- is_sparse(...): Returns whether a tensor is a sparse tensor.
- l2_normalize(...): Normalizes a tensor wrt the L2 norm alongside the specified axis.
- learning_phase(...): Returns the learning phase flag.
- learning_phase_scope(...): Provides a scope within which the learning phase is equal to
value
.
- less(...): Element-wise truth value of (x < y).
- less_equal(...): Element-wise truth value of (x <= y).
- local_conv1d(...): Apply 1D conv with un-shared weights.
- local_conv2d(...): Apply 2D conv with un-shared weights.
- log(...): Element-wise log.
- manual_variable_initialization(...): Sets the manual variable initialization flag.
- map_fn(...): Map the function fn over the elements elems and return the outputs.
- max(...): Maximum value in a tensor.
- maximum(...): Element-wise maximum of two tensors.
- mean(...): Mean of a tensor, alongside the specified axis.
- min(...): Minimum value in a tensor.
- minimum(...): Element-wise minimum of two tensors.
- moving_average_update(...): Compute the moving average of a variable.
- name_scope(...): A context manager for use when defining a Python op.
- ndim(...): Returns the number of axes in a tensor, as an integer.
- normalize_batch_in_training(...): Computes mean and std for batch then apply batch_normalization on batch.
- not_equal(...): Element-wise inequality between two tensors.
- one_hot(...): Computes the one-hot representation of an integer tensor.
- ones(...): Instantiates an all-ones variable and returns it.
- ones_like(...): Instantiates an all-ones variable of the same shape as another tensor.
- permute_dimensions(...): Permutes axes in a tensor.
- placeholder(...): Instantiates a placeholder tensor and returns it.
- pool2d(...): 2D Pooling.
- pool3d(...): 3D Pooling.
- pow(...): Element-wise exponentiation.
- print_tensor(...): Prints
message
and the tensor value when evaluated.
- prod(...): Multiplies the values in a tensor, alongside the specified axis.
- random_binomial(...): Returns a tensor with random binomial distribution of values.
- random_normal(...): Returns a tensor with normal distribution of values.
- random_normal_variable(...): Instantiates a variable with values drawn from a normal distribution.
- random_uniform(...): Returns a tensor with uniform distribution of values.
- random_uniform_variable(...): Instantiates a variable with values drawn from a uniform distribution.
- relu(...): Rectified linear unit.
- repeat(...): Repeats a 2D tensor.
- repeat_elements(...): Repeats the elements of a tensor along an axis, like
np.repeat
.
- reset_uids(...): Resets graph identifiers.
- reshape(...): Reshapes a tensor to the specified shape.
- resize_images(...): Resizes the images contained in a 4D tensor.
- resize_volumes(...): Resizes the volume contained in a 5D tensor.
- reverse(...): Reverse a tensor along the specified axes.
- rnn(...): Iterates over the time dimension of a tensor.
- round(...): Element-wise rounding to the closest integer.
- separable_conv2d(...): 2D convolution with separable filters.
- set_epsilon(...): Sets the value of the fuzz factor used in numeric expressions.
- set_floatx(...): Sets the default float type.
- set_image_data_format(...): Sets the value of the image data format convention.
- set_learning_phase(...): Sets the learning phase to a fixed value.
- set_value(...): Sets the value of a variable, from a Numpy array.
- shape(...): Returns the symbolic shape of a tensor or variable.
- sigmoid(...): Element-wise sigmoid.
- sign(...): Element-wise sign.
- sin(...): Computes sin of x element-wise.
- softmax(...): Softmax of a tensor.
- softplus(...): Softplus of a tensor.
- softsign(...): Softsign of a tensor.
- sparse_categorical_crossentropy(...): Categorical crossentropy with integer targets.
- spatial_2d_padding(...): Pads the 2nd and 3rd dimensions of a 4D tensor.
- spatial_3d_padding(...): Pads 5D tensor with zeros along the depth, height, width dimensions.
- sqrt(...): Element-wise square root.
- square(...): Element-wise square.
- squeeze(...): Removes a 1-dimension from the tensor at index "axis".
- stack(...): Stacks a list of rank
R
tensors into a rank R+1
tensor.
- std(...): Standard deviation of a tensor, alongside the specified axis.
- stop_gradient(...): Returns
variables
but with zero gradient w.r.t. every other variable.
- sum(...): Sum of the values in a tensor, alongside the specified axis.
- switch(...): Switches between two operations depending on a scalar value.
- tanh(...): Element-wise tanh.
- temporal_padding(...): Pads the middle dimension of a 3D tensor.
- tile(...): Creates a tensor by tiling
x
by n
.
- to_dense(...): Converts a sparse tensor into a dense tensor and returns it.
- transpose(...): Transposes a tensor and returns it.
- truncated_normal(...): Returns a tensor with truncated random normal distribution of values.
- update(...)
- update_add(...): Update the value of
x
by adding increment
.
- update_sub(...): Update the value of
x
by subtracting decrement
.
- var(...): Variance of a tensor, alongside the specified axis.
- variable(...): Instantiates a variable and returns it.
- zeros(...): Instantiates an all-zeros variable and returns it.
- zeros_like(...): Instantiates an all-zeros variable of the same shape as another tensor.
二、重要的函数
1、keras.backend.arange
Creates a 1D tensor containing a sequence of integers.
tf.keras.backend.arange(
start,
stop=None,
step=1,
dtype='int32'
)
The function arguments use the same convention as Theano's arange: if only one argument is provided, it is in fact the "stop" argument and "start" is 0.
The default type of the returned tensor is 'int32'
to match TensorFlow's default.
Arguments:
start
: Start value.
stop
: Stop value.
step
: Difference between two successive values.
dtype
: Integer dtype to use.
Returns:
Example:
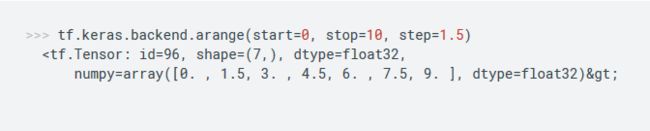
Compat aliases
- tf.compat.v1.keras.backend.arange
- tf.compat.v2.keras.backend.arange
2、keras.backend.reshape
Reshapes a tensor to the specified shape.
tf.keras.backend.reshape(
x,
shape
)
Arguments:
x
: Tensor or variable.
shape
: Target shape tuple.
Returns:
Example:
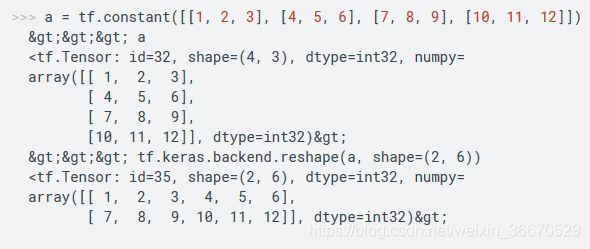
Compat aliases
- tf.compat.v1.keras.backend.reshape
- tf.compat.v2.keras.backend.reshape
3、keras.backend.variable
Instantiates a variable and returns it.
tf.keras.backend.variable(
value,
dtype=None,
name=None,
constraint=None
)
Arguments:
value
: Numpy array, initial value of the tensor.
dtype
: Tensor type.
name
: Optional name string for the tensor.
constraint
: Optional projection function to be applied to the variable after an optimizer update.
Returns:
- A variable instance (with Keras metadata included).
Examples:
import numpy as np
>>> from keras import backend as K
>>> val = np.array([[1, 2], [3, 4]])
>>> kvar = K.variable(value=val, dtype='float64', name='example_var')
>>> K.dtype(kvar)
'float64'
>>> print(kvar)
example_var
>>> kvar.eval()
array([[ 1., 2.],
[ 3., 4.]])
Compat aliases
- tf.compat.v1.keras.backend.variable
- tf.compat.v2.keras.backend.variable
4、keras.backend.cast
Casts a tensor to a different dtype and returns it.
tf.keras.backend.cast(
x,
dtype
)
You can cast a Keras variable but it still returns a Keras tensor.
Arguments:
x
: Keras tensor (or variable).
dtype
: String, either ('float16'
, 'float32'
, or 'float64'
).
Returns:
- Keras tensor with dtype
dtype
.
Examples:
Cast a float32 variable to a float64 tensor
import tensorflow as tf
>>> from tensorflow.keras import backend as K
>>> input = K.ones(shape=(1,3))
>>> print(input)
>>> cast_input = K.cast(input, dtype='float64')
>>> print(cast_input)
<tf.Variable 'Variable:0' shape=(1, 3) dtype=float32,
numpy=array([[1., 1., 1.]], dtype=float32)>
tf.Tensor([[1. 1. 1.]], shape=(1, 3), dtype=float64)
Compat aliases
- tf.compat.v1.keras.backend.cast
- tf.compat.v2.keras.backend.cast
5、keras.backend.greater
Element-wise truth value of (x > y).
tf.keras.backend.greater(
x,
y
)
Arguments:
x
: Tensor or variable.
y
: Tensor or variable.
Returns:
Compat aliases
- tf.compat.v1.keras.backend.greater
- tf.compat.v2.keras.backend.greater
6、keras.backend.gather
Retrieves the elements of indices indices
in the tensor reference
.
tf.keras.backend.gather(
reference,
indices
)
Arguments:
reference
: A tensor.
indices
: An integer tensor of indices.
Returns:
- A tensor of same type as
reference
.
Compat aliases
- tf.compat.v1.keras.backend.gather
- tf.compat.v2.keras.backend.gather
7、keras.backend.stack
Stacks a list of rank R
tensors into a rank R+1
tensor.
tf.keras.backend.stack(
x,
axis=0
)
Arguments:
x
: List of tensors.
axis
: Axis along which to perform stacking.
Returns:
Example:
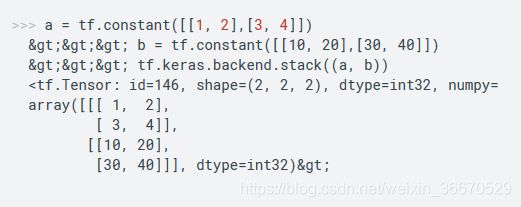
Compat aliases
- tf.compat.v1.keras.backend.stack
- tf.compat.v2.keras.backend.stack
8、keras.backend.shape
Returns the symbolic shape of a tensor or variable.
tf.keras.backend.shape(x)
Arguments:
Returns:
- A symbolic shape (which is itself a tensor).
Examples:
# TensorFlow example
>>> from keras import backend as K
>>> tf_session = K.get_session()
>>> val = np.array([[1, 2], [3, 4]])
>>> kvar = K.variable(value=val)
>>> input = keras.backend.placeholder(shape=(2, 4, 5))
>>> K.shape(kvar)
>>> K.shape(input)
# To get integer shape (Instead, you can use K.int_shape(x))
>>> K.shape(kvar).eval(session=tf_session)
array([2, 2], dtype=int32)
>>> K.shape(input).eval(session=tf_session)
array([2, 4, 5], dtype=int32)
Compat aliases
- tf.compat.v1.keras.backend.shape
- tf.compat.v2.keras.backend.shape
9、keras.backend.concatenate
Concatenates a list of tensors alongside the specified axis.
tf.keras.backend.concatenate(
tensors,
axis=-1
)
Arguments:
tensors
: list of tensors to concatenate.
axis
: concatenation axis.
Returns:
Example:
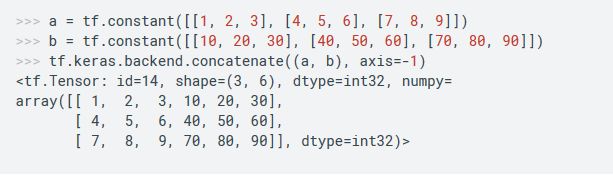
Compat aliases
- tf.compat.v1.keras.backend.concatenate
- tf.compat.v2.keras.backend.concatenate
10、keras.backend.max
Maximum value in a tensor.
tf.keras.backend.max(
x,
axis=None,
keepdims=False
)
Arguments:
x
: A tensor or variable.
axis
: An integer, the axis to find maximum values.
keepdims
: A boolean, whether to keep the dimensions or not. If keepdims
is False
, the rank of the tensor is reduced by 1. If keepdims
is True
, the reduced dimension is retained with length 1.
Returns:
A tensor with maximum values of x
.
Compat aliases
- tf.compat.v1.keras.backend.max
- tf.compat.v2.keras.backend.max