最近在做一个项目,需要用到拖动图片,旋转效果,两点放大缩小。
首先拖动图片
布局,主要就是一个button做测试
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
>
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="@string/hello"
/>
<Button
android:id="@+id/btn"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/bt"
/>
</LinearLayout>
package com.oppo.examples;
import android.app.Activity;
import android.os.Bundle;
import android.util.DisplayMetrics;
import android.util.Log;
import android.view.MotionEvent;
import android.view.View;
import android.view.View.OnTouchListener;
import android.widget.Button;
import android.widget.Toast;
public class DraftTest extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
DisplayMetrics dm = getResources().getDisplayMetrics();
final int screenWidth = dm.widthPixels;
final int screenHeight = dm.heightPixels - 50;
final Button b=(Button)findViewById(R.id.btn);
b.setOnTouchListener(new OnTouchListener(){
int lastX, lastY;
@Override
public boolean onTouch(View v, MotionEvent event) {
// TODO Auto-generated method stub
int ea=event.getAction();
Log.i("TAG", "Touch:"+ea);
//Toast.makeText(DraftTest.this, "位置:"+x+","+y, Toast.LENGTH_SHORT).show();
switch(ea){
case MotionEvent.ACTION_DOWN:
lastX = (int) event.getRawX();
lastY = (int) event.getRawY();
break;
/**
* layout(l,t,r,b)
* l Left position, relative to parent
t Top position, relative to parent
r Right position, relative to parent
b Bottom position, relative to parent
* */
case MotionEvent.ACTION_MOVE:
int dx =(int)event.getRawX() - lastX;
int dy =(int)event.getRawY() - lastY;
int left = v.getLeft() + dx;
int top = v.getTop() + dy;
int right = v.getRight() + dx;
int bottom = v.getBottom() + dy;
if(left < 0){
left = 0;
right = left + v.getWidth();
}
if(right > screenWidth){
right = screenWidth;
left = right - v.getWidth();
}
if(top < 0){
top = 0;
bottom = top + v.getHeight();
}
if(bottom > screenHeight){
bottom = screenHeight;
top = bottom - v.getHeight();
}
v.layout(left, top, right, bottom);
Log.i("", "position:" + left +", " + top + ", " + right + ", " + bottom);
lastX = (int) event.getRawX();
lastY = (int) event.getRawY();
break;
case MotionEvent.ACTION_UP:
break;
}
return false;
}});
}
}
代码主要实现 public boolean onTouch(View v, MotionEvent event) 方法,对MotionEvent 的各种状态进行定义 与距离的判断,ACTION_DOWN,ACTION_MOVE,ACTION_UP
这里是资源源代码:http://download.csdn.net/detail/zhangty0223/5787251
android Bitmap围绕一个点进行旋转
在项目中需要使用定位功能,也就是一个点围绕一个圆心进行旋转,查看了canvas的函数也就只有一个 canvas.drawBitmap(bitmap, matrix, paint)通过使用Matrix来实现旋转,这里实现一个demo,功能就是小原点顺时针绘制一个原然后逆时针撤销这个圆,通过Timer来实现。代码如下:
Surface.java这个是实现绘制图形的类
- package com.example.test;
-
- import java.util.Timer;
- import java.util.TimerTask;
-
- import android.annotation.SuppressLint;
- import android.content.Context;
- import android.graphics.Bitmap;
- import android.graphics.BitmapFactory;
- import android.graphics.Canvas;
- import android.graphics.Color;
- import android.graphics.Matrix;
- import android.graphics.Paint;
- import android.graphics.PixelFormat;
- import android.graphics.PorterDuff.Mode;
- import android.os.Handler;
- import android.os.Message;
- import android.util.Log;
- import android.view.SurfaceHolder;
- import android.view.SurfaceView;
- import android.view.SurfaceHolder.Callback;
-
- @SuppressLint("HandlerLeak")
- class Surface extends SurfaceView implements Callback{
-
- Bitmap bmp;
- private Paint mPaint;
- private SurfaceHolder mHolder;
- public Surface(Context context) {
- super(context);
- mHolder = getHolder();
- mHolder.addCallback(this);
- mPaint = new Paint();
- mPaint.setColor(Color.GREEN);
- mPaint.setAntiAlias(true);
- setFocusable(true);
- setFocusableInTouchMode(true);
-
-
- bmp = BitmapFactory.decodeResource(getResources(), R.drawable.camera_r_local);
- bmp = Bitmap.createScaledBitmap(bmp, 15, 15, false);
- }
-
- public Handler handler = new Handler(){
- @Override
- public void handleMessage(Message msg) {
- switch (msg.what) {
- case 0:
- canvas_ok();
- break;
- }
- super.handleMessage(msg);
- }
-
- };
-
- public void canvas_ok(){
- Canvas canvas = null;
- try {
- canvas = mHolder.lockCanvas();
- canvas.drawColor(Color.TRANSPARENT, Mode.CLEAR);
-
- Matrix matrix = new Matrix();
- matrix.postTranslate(130, 0);
- matrix.postRotate(degree, 130 ,130);
- canvas.drawBitmap(bmp, matrix, mPaint);
-
- } catch (Exception e) {
- }finally {
- if(canvas != null) {
- mHolder.unlockCanvasAndPost(canvas);
- }
- }
- }
-
- int degree = 0;
- Timer timer = new Timer();
- boolean flag = true;
- @Override
- public void surfaceCreated(SurfaceHolder holder) {
- canvas_ok();
- timer.schedule(new TimerTask() {
- @Override
- public void run() {
- if (flag) {
- degree++;
- if (degree == 360) {
- flag = false;
- }
- }else {
- degree--;
- if(degree == -360 || degree == 0){
- flag = true;
- }
- }
- Log.e("flag", degree+" : "+flag);
- handler.sendEmptyMessage(0);
- }
- }, 50, 50);
- }
-
-
- @Override
- public void surfaceChanged(SurfaceHolder holder, int format, int width,
- int height) {
-
- }
-
-
- @Override
- public void surfaceDestroyed(SurfaceHolder holder) {
- if (timer != null) {
- timer.cancel();
- timer = null;
- }
- }
- }
Main7.java是主类
- package com.example.test;
-
- import android.app.Activity;
- import android.os.Bundle;
- import android.widget.RelativeLayout;
-
- public class Main7 extends Activity{
- Surface surface;
- RelativeLayout.LayoutParams laParams;
- @Override
- protected void onCreate(Bundle savedInstanceState) {
- super.onCreate(savedInstanceState);
- surface = new Surface(this);
- laParams = new RelativeLayout.LayoutParams(260, 260);
- setContentView(surface, laParams);
- }
-
- }
效果图如下:
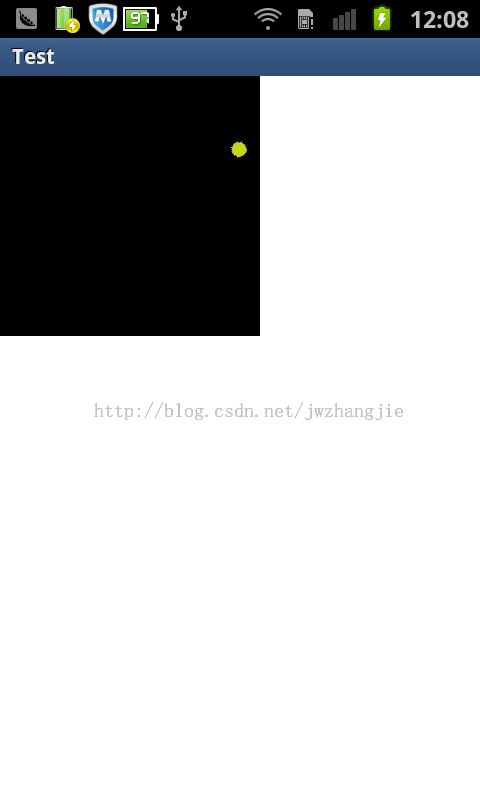
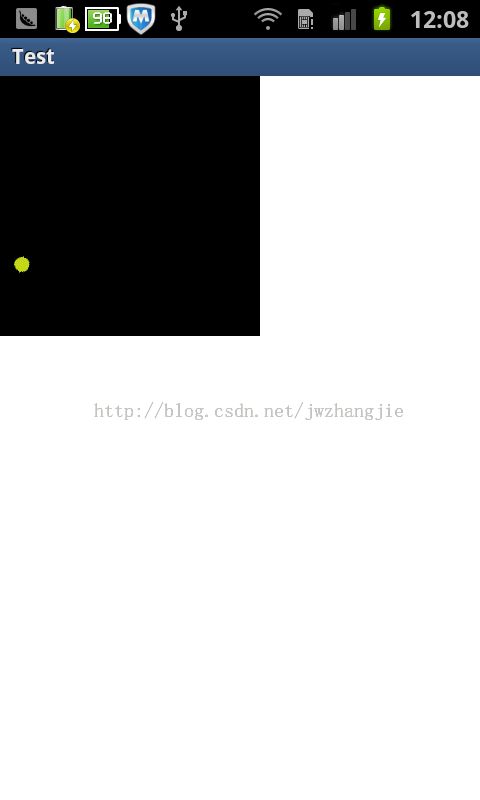
两点放大缩小图片
功能有:
- 以触摸点为中心放大(这个是网上其他的代码没有的)
- 边界控制(这个是网上其他的代码没有的)
- 双击放大或缩小(主要考虑到电阻屏)
- 多点触摸放大和缩小
这个模块已经通过了测试,并且用户也使用有一段时间了,是属于比较稳定的了。
下面贴上代码及使用方法(没有写测试项目,大家见谅):
ImageControl.cs 类似一个用户自定义的ImageView控件。用法将在下面的代码中贴出。
- import android.content.Context;
- import android.graphics.Bitmap;
- import android.graphics.Matrix;
- import android.util.AttributeSet;
- import android.util.FloatMath;
- import android.view.MotionEvent;
- import android.widget.ImageView;
-
- public class ImageControl extends ImageView {
- public ImageControl(Context context) {
- super(context);
-
- }
-
- public ImageControl(Context context, AttributeSet attrs) {
- super(context, attrs);
-
- }
-
- public ImageControl(Context context, AttributeSet attrs, int defStyle) {
- super(context, attrs, defStyle);
-
- }
-
-
- Matrix imgMatrix = null;
-
- static final int DOUBLE_CLICK_TIME_SPACE = 300;
- static final int DOUBLE_POINT_DISTANCE = 10;
- static final int NONE = 0;
- static final int DRAG = 1;
- static final int ZOOM = 2;
- private int mode = NONE;
-
- float bigScale = 3f;
- Boolean isBig = false;
- long lastClickTime = 0;
- float startDistance;
- float endDistance;
-
- float topHeight;
- Bitmap primaryBitmap = null;
-
- float contentW;
- float contentH;
-
- float primaryW;
- float primaryH;
-
- float scale;
- Boolean isMoveX = true;
- Boolean isMoveY = true;
- float startX;
- float startY;
- float endX;
- float endY;
- float subX;
- float subY;
- float limitX1;
- float limitX2;
- float limitY1;
- float limitY2;
- ICustomMethod mCustomMethod = null;
-
-
-
-
-
-
-
-
-
-
-
-
-
- public void imageInit(Bitmap bitmap, int contentW, int contentH,
- int topHeight, ICustomMethod iCustomMethod) {
- this.primaryBitmap = bitmap;
- this.contentW = contentW;
- this.contentH = contentH;
- this.topHeight = topHeight;
- mCustomMethod = iCustomMethod;
- primaryW = primaryBitmap.getWidth();
- primaryH = primaryBitmap.getHeight();
- float scaleX = (float) contentW / primaryW;
- float scaleY = (float) contentH / primaryH;
- scale = scaleX < scaleY ? scaleX : scaleY;
- if (scale < 1 && 1 / scale < bigScale) {
- bigScale = (float) (1 / scale + 0.5);
- }
-
- imgMatrix = new Matrix();
- subX = (contentW - primaryW * scale) / 2;
- subY = (contentH - primaryH * scale) / 2;
- this.setImageBitmap(primaryBitmap);
- this.setScaleType(ScaleType.MATRIX);
- imgMatrix.postScale(scale, scale);
- imgMatrix.postTranslate(subX, subY);
- this.setImageMatrix(imgMatrix);
- }
-
-
-
-
-
-
- public void mouseDown(MotionEvent event) {
- mode = NONE;
- startX = event.getRawX();
- startY = event.getRawY();
- if (event.getPointerCount() == 1) {
-
- if (event.getEventTime() - lastClickTime < DOUBLE_CLICK_TIME_SPACE) {
- changeSize(startX, startY);
- } else if (isBig) {
- mode = DRAG;
- }
- }
-
- lastClickTime = event.getEventTime();
- }
-
-
-
-
-
-
- public void mousePointDown(MotionEvent event) {
- startDistance = getDistance(event);
- if (startDistance > DOUBLE_POINT_DISTANCE) {
- mode = ZOOM;
- } else {
- mode = NONE;
- }
- }
-
-
-
-
-
-
- public void mouseMove(MotionEvent event) {
- if ((mode == DRAG) && (isMoveX || isMoveY)) {
- float[] XY = getTranslateXY(imgMatrix);
- float transX = 0;
- float transY = 0;
- if (isMoveX) {
- endX = event.getRawX();
- transX = endX - startX;
- if ((XY[0] + transX) <= limitX1) {
- transX = limitX1 - XY[0];
- }
- if ((XY[0] + transX) >= limitX2) {
- transX = limitX2 - XY[0];
- }
- }
- if (isMoveY) {
- endY = event.getRawY();
- transY = endY - startY;
- if ((XY[1] + transY) <= limitY1) {
- transY = limitY1 - XY[1];
- }
- if ((XY[1] + transY) >= limitY2) {
- transY = limitY2 - XY[1];
- }
- }
-
- imgMatrix.postTranslate(transX, transY);
- startX = endX;
- startY = endY;
- this.setImageMatrix(imgMatrix);
- } else if (mode == ZOOM && event.getPointerCount() > 1) {
- endDistance = getDistance(event);
- float dif = endDistance - startDistance;
- if (Math.abs(endDistance - startDistance) > DOUBLE_POINT_DISTANCE) {
- if (isBig) {
- if (dif < 0) {
- changeSize(0, 0);
- mode = NONE;
- }
- } else if (dif > 0) {
- float x = event.getX(0) / 2 + event.getX(1) / 2;
- float y = event.getY(0) / 2 + event.getY(1) / 2;
- changeSize(x, y);
- mode = NONE;
- }
- }
- }
- }
-
-
-
-
- public void mouseUp() {
- mode = NONE;
- }
-
-
-
-
-
-
-
-
-
- private void changeSize(float x, float y) {
- if (isBig) {
-
- imgMatrix.reset();
- imgMatrix.postScale(scale, scale);
- imgMatrix.postTranslate(subX, subY);
- isBig = false;
- } else {
- imgMatrix.postScale(bigScale, bigScale);
- float transX = -((bigScale - 1) * x);
- float transY = -((bigScale - 1) * (y - topHeight));
- float currentWidth = primaryW * scale * bigScale;
- float currentHeight = primaryH * scale * bigScale;
-
- if (currentHeight > contentH) {
- limitY1 = -(currentHeight - contentH);
- limitY2 = 0;
- isMoveY = true;
- float currentSubY = bigScale * subY;
-
- if (-transY < currentSubY) {
- transY = -currentSubY;
- }
-
- if (currentSubY + transY < limitY1) {
- transY = -(currentHeight + currentSubY - contentH);
- }
- } else {
-
- isMoveY = false;
- }
-
- if (currentWidth > contentW) {
- limitX1 = -(currentWidth - contentW);
- limitX2 = 0;
- isMoveX = true;
- float currentSubX = bigScale * subX;
- if (-transX < currentSubX) {
- transX = -currentSubX;
- }
- if (currentSubX + transX < limitX1) {
- transX = -(currentWidth + currentSubX - contentW);
- }
- } else {
- isMoveX = false;
- }
-
- imgMatrix.postTranslate(transX, transY);
- isBig = true;
- }
-
- this.setImageMatrix(imgMatrix);
- if (mCustomMethod != null) {
- mCustomMethod.customMethod(isBig);
- }
- }
-
-
-
-
-
-
-
-
- private float[] getTranslateXY(Matrix matrix) {
- float[] values = new float[9];
- matrix.getValues(values);
- float[] floats = new float[2];
- floats[0] = values[Matrix.MTRANS_X];
- floats[1] = values[Matrix.MTRANS_Y];
- return floats;
- }
-
-
-
-
-
-
-
- private float getDistance(MotionEvent event) {
- float x = event.getX(0) - event.getX(1);
- float y = event.getY(0) - event.getY(1);
- return FloatMath.sqrt(x * x + y * y);
- }
-
-
-
-
- public interface ICustomMethod {
- public void customMethod(Boolean currentStatus);
- }
- }
ImageVewActivity.cs 这个用于测试的Activity
在上面的代码中,需要注意两点。一Activity中要重写onTouchEvent方法,将触摸事件传递到ImageControl,这点类似于WPF中的路由事件机制。二初始化imgControl即imgControl.imageInit,注意其中的参数。最后一个参数类似于C#中的委托,我这里使用接口来实现,在放大缩小的切换时要执行的操作都卸载这个方法中。
common_image_view.xml 布局文件
- <?xml version="1.0" encoding="utf-8"?>
- <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
- android:id="@+id/rl"
- android:layout_width="fill_parent"
- android:layout_height="fill_parent" >
-
- <ejiang.boiler.ImageControl
- android:id="@+id/common_imageview_imageControl1"
- android:layout_width="fill_parent"
- android:layout_height="fill_parent"
- android:src="@drawable/ic_launcher" />
-
- <LinearLayout
- android:id="@+id/common_imageview_llTitle"
- style="@style/reportTitle1"
- android:layout_alignParentLeft="true"
- android:layout_alignParentTop="true" >
-
- <TextView
- android:id="@+id/common_imageview_title"
- style="@style/title2"
- android:layout_width="fill_parent"
- android:layout_height="wrap_content"
- android:layout_weight="1"
- android:text="报告" />
- </LinearLayout>
-
- </RelativeLayout>
源码给大家学习。。。
http://download.csdn.net/detail/zhangty0223/5787291
谢谢!