javascript constructor 详解
2010-04-26 22:19
请参考:[0] http://hi.baidu.com/maxwin2008/blog/item/2b3a663582c1ad1a90ef39e5.html
课外读物:
[1] http://joost.zeekat.nl/constructors-considered-mildly-confusing.html
[2] http://dhtmlkitchen.com/learn/js/enumeration/index.jsp
这个是和js里面对象相关的问题,首先要了解的是类(函数对象)、实例之间的关系,也就是prototype chain[0, 2]。
每个class都有一个prototype,当使用该class创建instance时就会将该instance的[[Prototype]]指向class的prototype。
有些时候的应用我们想知道一个instance是由那个class创建的,这时候就要用到constructor属性。
class的prototype里有一个notEnum[2]属性的constructor来指向class本身。
<script type="text/javascript"> function Base () {} Base.prototype.foo = function() { console.log('Base.foo()'); } var b = new Base(); console.log(Base.prototype.constructor); // Base() console.log(b.constructor === Base); // true </script>
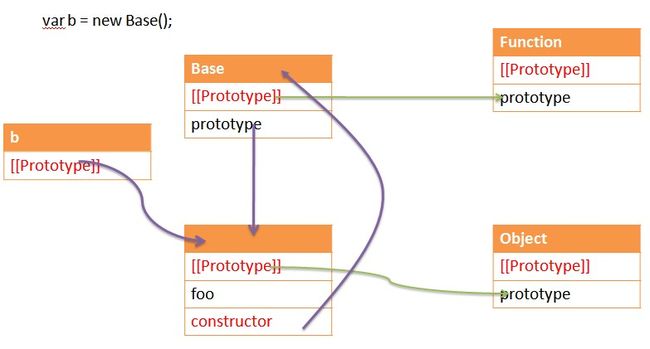
这一切行为都很正常,偏偏多了js oop。那么我们需要在class里定义一些属性和方法。这里有两种写法:
<script type="text/javascript"> function A() {} A.prototype.foo = function () { console.log('A.foo()'); } function B() {} B.prototype = { foo: function () { console.log('B.foo()'); } } </script> 需要清楚这两种方法的区别,第一种是往A.prototype里塞东西,原有的东西并没有被修改。
第二种则是让B.prototype大搬家,先前的东西全部丢掉了,其中就包含了constructor属性。
(注意:这里B.prototype里是没了constructor方法,但是b.constructor依然有值:Object()。因为prototype chain上直接查找到了Object.prototype.constructor)
<script type="text/javascript"> var a = new A(); console.log(a.constructor === A); // true var b = new B(); console.log(b.constructor === B); // false </script>
第二种方法导致constructor丢失了。如果写写简单的js,这个属性也用不到,没了就没了吧。但是这个属性对我们来说肯定是有用的,或者你对它感兴趣。
那就不能让这个属性就这样没了。
那也OK,我们都用第一中方法来定义class。但事情经常不尽人意,js里又需要通过prototype chain来模拟继承行为:
<script type="text/javascript"> function Derived() {} Derived.prototype = new Base(); Derived.prototype.foo = function() { console.log('Derived.foo'); } </script> 为了实现继承,我们必须让Derived的prototype搬家,这样就是上述的第二种行为,导致自己constructor没了,Derived生成的instance通过constructor来找自己的时候会找到自己的superclass Base(有违伦理啊...)。
那只好我们手动设置Derived的constructor属性:
<script type="text/javascript"> Derived.prototype.constructor = Derived; </script> 这样再使用的时候就基本OK了: <script type="text/javascript"> var d = new Derived(); console.log(d.constructor === Derived); // of course TRUE </script>
但是问题又来了,Derived.prototype.constructor = Derived 之后,原本这个constructor应该是指向Base的(单从Derived.prototype = new Base())来理解。
现在为了修正instance能找到自己的class,却把instance的class的superclass给丢了,真是顾此失彼...
<script type="text/javascript"> // class X function X() {} X.prototype.foo = function() {console.log('X.foo()');} // class Y extend X function Y() {} Y.prototype = new X(); Y.prototype.constructor = Y; Y.prototype.foo = function() {console.log('Y.foo()');} // class Z extend Y function Z() {} Z.prototype = new Y(); Z.prototype.constructor = Z; Z.prototype.foo = function() {console.log('Z.foo()');} var z = new Z(); console.log(z.constructor); // Z </script> 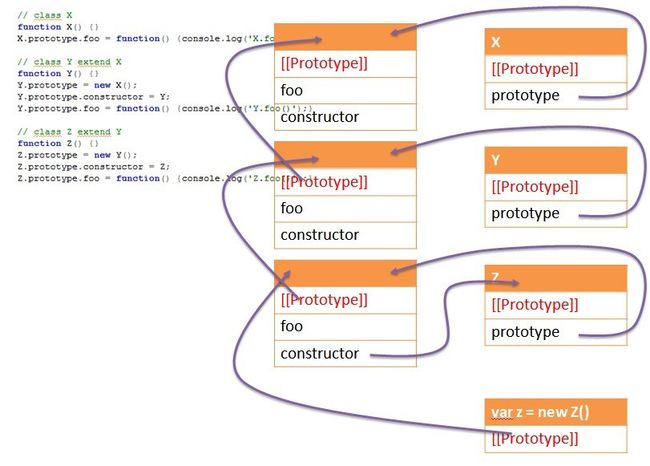 这里z可以知道通过constructor获取他的class Z,但是却没有方法获得它superclass的superclass Y。
在firefox中可以使用__proto__(这个就是[[Prototype]]的指针),但是ie并不支持。比较通用的方法是给每个class设置一个属性指向它的superclass
比如 Z.superclass = Y, Y.superclass = X。
|
constructor属性始终指向创建当前对象的构造函数。比如下面例子:
//
等价于 var foo = new Array(1, 56, 34, 12);
var
arr
=
[
1
,
56
,
34
,
12
]; console.log(arr.constructor
===
Array);
//
true
//
等价于 var foo = new Function();
var
Foo
=
function
() { }; console.log(Foo.constructor
===
Function);
//
true
//
由构造函数实例化一个obj对象
var
obj
=
new
Foo(); console.log(obj.constructor
===
Foo);
//
true
//
将上面两段代码合起来,就得到下面的结论
console.log(obj.constructor.constructor
===
Function);
//
true
但是当constructor遇到prototype时,有趣的事情就发生了。
我们知道每个函数都有一个默认的属性prototype,而这个prototype的constructor默认指向这个函数。如下例所示:
function
Person(name) {
this
.name
=
name; }; Person.prototype.getName
=
function
() {
return
this
.name; };
var
p
=
new
Person(
"
ZhangSan
"
); console.log(p.constructor
===
Person);
//
true
console.log(Person.prototype.constructor
===
Person);
//
true
//
将上两行代码合并就得到如下结果
console.log(p.constructor.prototype.constructor
===
Person);
//
true
当时当我们重新定义函数的prototype时(注意:和上例的区别,这里不是修改而是覆盖),constructor属性的行为就有点奇怪了,如下示例:
function
Person(name) {
this
.name
=
name; }; Person.prototype
=
{ getName:
function
() {
return
this
.name; } };
var
p
=
new
Person(
"
ZhangSan
"
); console.log(p.constructor
===
Person);
//
false
console.log(Person.prototype.constructor
===
Person);
//
false
console.log(p.constructor.prototype.constructor
===
Person);
//
false
为什么呢?
原来是因为覆盖Person.prototype时,等价于进行如下代码操作:
Person.prototype
=
new
Object({ getName:
function
() {
return
this
.name; } });
看到这里可能有童鞋会问为什么改prototype的值会让p.constructor 的值改变呢,其实单纯的function里没有constructor属性,但为什么可以访问,因为当访问一个不存在的属性的时候,JS会找改属性在原型链里存在与否,其实也就是JS继承的实现,结果发现原型链里有,所以,如上例,p.constructor跟p.prototype.constructor是同一个东西来的,不信你修改p.prototype.constructor就知道了,如下文,但你修改p.constructor就不会影响到p.prototype.consturctor,相当于重写了一个方法(add lonely 2011-9-28)
而constructor属性始终指向创建自身的构造函数,所以此时Person.prototype.constructor === Object,即是:
function
Person(name) {
this
.name
=
name; }; Person.prototype
=
{ getName:
function
() {
return
this
.name; } };
var
p
=
new
Person(
"
ZhangSan
"
); console.log(p.constructor
===
Object);
//
true
console.log(Person.prototype.constructor
===
Object);
//
true
console.log(p.constructor.prototype.constructor
===
Object);
//
true
怎么修正这种问题呢?方法也很简单,重新覆盖Person.prototype.constructor即可:
function
Person(name) {
this
.name
=
name; }; Person.prototype
=
new
Object({ getName:
function
() {
return
this
.name; } }); Person.prototype.constructor
=
Person;
var
p
=
new
Person(
"
ZhangSan
"
); console.log(p.constructor
===
Person);
//
true
console.log(Person.prototype.constructor
===
Person);
//
true
console.log(p.constructor.prototype.constructor
===
Person);
//
true