TensorFlow2.0 前向传播 张量基础的使用
1.导入与设置
import tensorflow as tf
from tensorflow import keras
from tensorflow.keras import datasets
print(tf.__version__)
gpus = tf.config.experimental.list_physical_devices("GPU")
for gpu in gpus:
tf.config.experimental.set_memory_growth(gpu, True)
print("物理GPU个数:", len(gpus))
logical_gpus = tf.config.experimental.list_logical_devices("GPU")
print("逻辑GPU个数:", len(logical_gpus))
2.加载数据与预处理
(x, y), _ = datasets.mnist.load_data()
print(x.shape)
print(y.shape)
x = tf.convert_to_tensor(x, dtype = tf.float32)
y = tf.convert_to_tensor(y, dtype = tf.int32)
print(x.dtype)
print(y.dtype)
print(tf.reduce_min(x), tf.reduce_max(x))
print(tf.reduce_min(y), tf.reduce_max(y))
x = x / 255.0
print(tf.reduce_min(x), tf.reduce_max(x))
3.数据集制作 变量初始化
train_db = tf.data.Dataset.from_tensor_slices((x, y)).batch(128)
train_iter = iter(train_db)
sample = next(train_iter)
print("batch:", sample[0].shape, sample[1].shape)
lr = 1e-3
w1 = tf.Variable(tf.random.truncated_normal([784, 256], stddev=0.1))
b1 = tf.Variable(tf.zeros([256]))
w2 = tf.Variable(tf.random.truncated_normal([256, 128], stddev=0.1))
b2 = tf.Variable(tf.zeros([128]))
w3 = tf.Variable(tf.random.truncated_normal([128, 10], stddev=0.1))
b3 = tf.Variable(tf.zeros([10]))
4.训练数据集 计算loss 计算梯度
for epoch in range(10):
for step, (x, y) in enumerate(train_db):
x = tf.reshape(x, [-1, 28*28])
with tf.GradientTape() as tape:
h1 = x @ w1 + tf.broadcast_to(b1, [x.shape[0], 256])
h1 = tf.nn.relu(h1)
h2 = h1 @ w2 + b2
h2 = tf.nn.relu(h2)
out = h2 @ w3 + b3
y_onehot = tf.one_hot(y, depth=10)
loss = tf.square(y_onehot - out)
loss = tf.reduce_mean(loss)
grads = tape.gradient(loss, [w1, b1, w2, b2, w3, b3])
w1.assign_sub(lr*grads[0])
b1.assign_sub(lr*grads[1])
w2.assign_sub(lr*grads[2])
b2.assign_sub(lr*grads[3])
w3.assign_sub(lr*grads[4])
b3.assign_sub(lr*grads[5])
if step % 100 == 0:
print("epoch=", epoch, "step=", step, " loss:", float(loss))
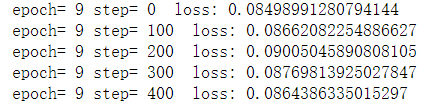