1. 数值选择器(NumberPicker)
实例:选择您意向的价格范围
public class MainActivity extends AppCompatActivity {
NumberPicker np1, np2;
int minPrice = 25, maxPrice = 75;
@Override
protected void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
np1 = (NumberPicker) findViewById(R.id.np1);
np1.setMinValue(10);
np1.setMaxValue(50);
np1.setValue(minPrice);
np1.setOnValueChangedListener(new NumberPicker.OnValueChangeListener()
{
@Override
public void onValueChange(NumberPicker picker,
int oldVal, int newVal)
{
minPrice = newVal;
showSelectedPrice();
}
});
np2 = (NumberPicker) findViewById(R.id.np2);
np2.setMinValue(60);
np2.setMaxValue(100);
np2.setValue(maxPrice);
np2.setOnValueChangedListener(new NumberPicker.OnValueChangeListener()
{
@Override
public void onValueChange(NumberPicker picker, int oldVal,
int newVal)
{
maxPrice = newVal;
showSelectedPrice();
}
});
}
private void showSelectedPrice()
{
Toast.makeText(this, "您选择最低价格为:" + minPrice
+ ",最高价格为:" + maxPrice, Toast.LENGTH_SHORT)
.show();
}
}
<TableLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<TableRow
android:layout_width="match_parent"
android:layout_height="wrap_content">
<TextView
android:text="选择低价:"
android:layout_width="120dp"
android:layout_height="wrap_content" />
<NumberPicker
android:id="@+id/np1"
android:layout_width="match_parent"
android:layout_height="80dp"
android:focusable="true"
android:focusableInTouchMode="true" />
TableRow>
<TableRow
android:layout_width="match_parent"
android:layout_height="wrap_content">
<TextView
android:text="选择高价:"
android:layout_width="120dp"
android:layout_height="wrap_content" />
<NumberPicker
android:id="@+id/np2"
android:layout_width="match_parent"
android:layout_height="80dp"
android:focusable="true"
android:focusableInTouchMode="true" />
TableRow>
TableLayout>
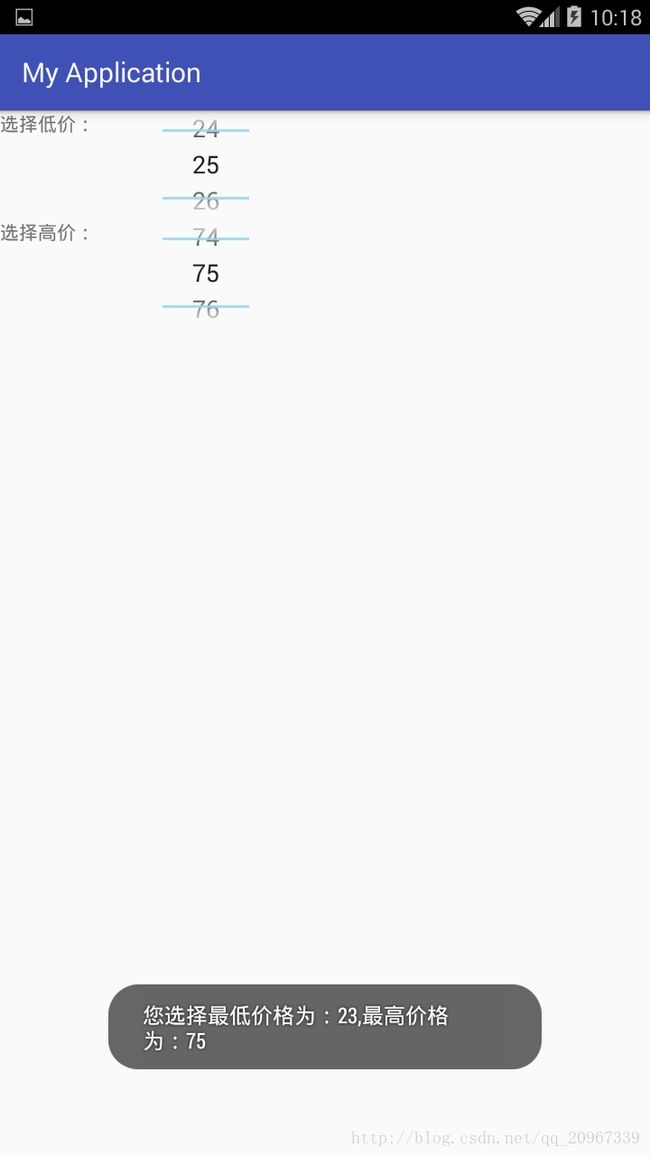
2. 搜索框(SearchView)
实例:搜索
public class MainActivity extends AppCompatActivity {
private SearchView sv;
private ListView lv;
private final String[] mStrings = { "aaaaa", "bbbbbb", "cccccc" };
@Override
protected void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
lv = (ListView) findViewById(R.id.lv);
lv.setAdapter(new ArrayAdapter(this,
android.R.layout.simple_list_item_1, mStrings));
lv.setTextFilterEnabled(true);
sv = (SearchView) findViewById(R.id.sv);
sv.setIconifiedByDefault(false);
sv.setSubmitButtonEnabled(true);
sv.setQueryHint("查找");
sv.setOnQueryTextListener(new SearchView.OnQueryTextListener()
{
@Override
public boolean onQueryTextChange(String newText)
{
if (TextUtils.isEmpty(newText))
{
lv.clearTextFilter();
}
else
{
lv.setFilterText(newText);
}
return true;
}
@Override
public boolean onQueryTextSubmit(String query)
{
Toast.makeText(MainActivity.this, "您的选择是:" + query
, Toast.LENGTH_SHORT).show();
return false;
}
});
}
}
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<SearchView
android:id="@+id/sv"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
<ListView
android:id="@+id/lv"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"/>
LinearLayout>
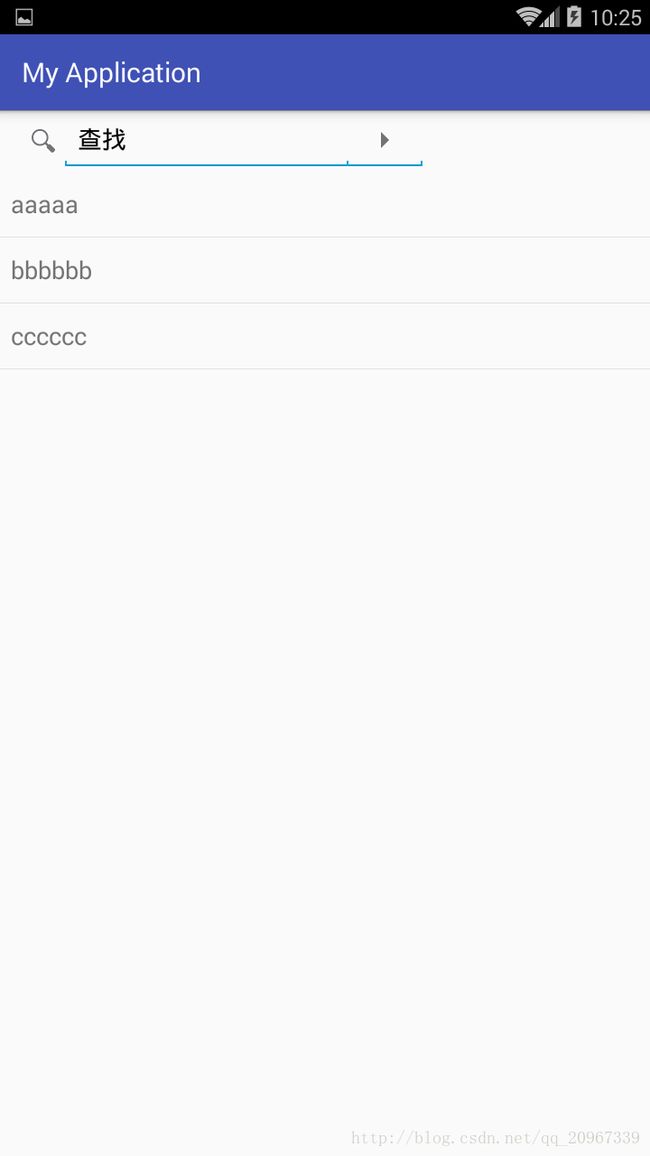
3. 选项卡(TabHost)
实例:通话记录界面
public class MainActivity extends TabActivity {
@Override
public void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
TabHost tabHost = getTabHost();
TabHost.TabSpec tab1 = tabHost.newTabSpec("tab1")
.setIndicator("已接电话")
.setContent(R.id.tab01);
tabHost.addTab(tab1);
TabHost.TabSpec tab2 = tabHost.newTabSpec("tab2")
.setIndicator("呼出电话", getResources()
.getDrawable(R.mipmap.ic_launcher))
.setContent(R.id.tab02);
tabHost.addTab(tab2);
TabHost.TabSpec tab3 = tabHost.newTabSpec("tab3")
.setIndicator("未接电话")
.setContent(R.id.tab03);
tabHost.addTab(tab3);
}
}
<TabHost
xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@android:id/tabhost"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_weight="1">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TabWidget
android:id="@android:id/tabs"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
<FrameLayout
android:id="@android:id/tabcontent"
android:layout_width="match_parent"
android:layout_height="match_parent">
<LinearLayout
android:id="@+id/tab01"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="女儿国国王 - 2012/12/12"
android:textSize="11pt" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="东海龙女 - 2012/12/18"
android:textSize="11pt" />
LinearLayout>
<LinearLayout
android:id="@+id/tab02"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="白骨精 - 2012/08/12"
android:textSize="11pt" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="蜘蛛精 - 2012/09/20"
android:textSize="11pt" />
LinearLayout>
<LinearLayout
android:id="@+id/tab03"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:textSize="11pt">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="孙悟空 - 2012/09/19"
android:textSize="11pt" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="猪八戒 - 2012/10/12"
android:textSize="11pt" />
LinearLayout>
FrameLayout>
LinearLayout>
TabHost>
实例:可垂直和水平滚动的视图
5.Notification
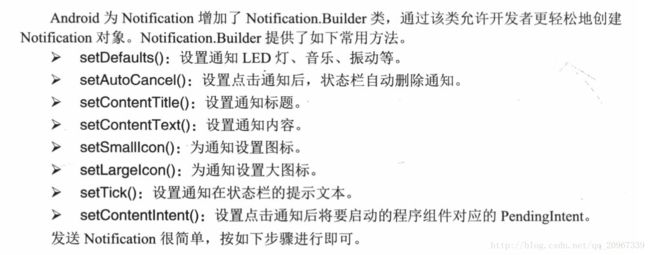
实例:加薪通知
public class MainActivity extends Activity {
static final int NOTIFICATION_ID = 0x123;
NotificationManager nm;
@Override
public void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
nm = (NotificationManager)
getSystemService(NOTIFICATION_SERVICE);
}
public void send(View source)
{
Intent intent = new Intent(MainActivity.this
, DrawView.class);
PendingIntent pi = PendingIntent.getActivity(
MainActivity.this, 0, intent, 0);
Notification notify = new Notification.Builder(this)
.setAutoCancel(true)
.setTicker("有新消息")
.setSmallIcon(R.drawable.bomb16)
.setContentTitle("一条新通知")
.setContentText("恭喜你,您加薪了,工资增加20%!")
.setSound(Uri.parse("android.resource://org.crazyit.ui/"
+ R.raw.msg))
.setWhen(System.currentTimeMillis())
.setContentIntent(pi)
.build();
nm.notify(NOTIFICATION_ID, notify);
}
public void del(View v)
{
nm.cancel(NOTIFICATION_ID);
}
}
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center_horizontal">
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="发送Notification"
android:onClick="send"
/>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="删除Notification"
android:onClick="del"
/>
LinearLayout>
public class DrawView extends Activity
{
@Override
public void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
setContentView(R.layout.other);
}
}
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center_horizontal"
android:orientation="vertical">
<ImageView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:src="@drawable/bomb5"
android:layout_gravity="center_horizontal"
/>
LinearLayout>
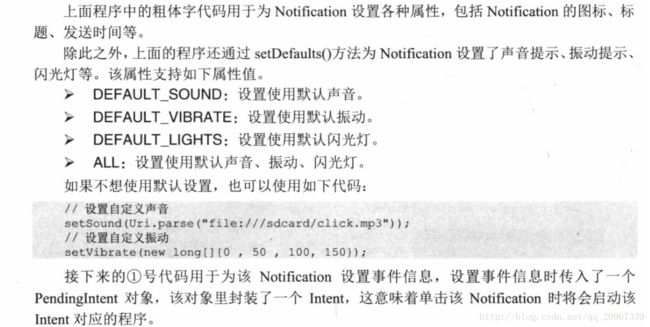
<uses-permission android:name="android.permission.FLASHLIGHT"/>
<uses-permission android:name="android.permission.VIBRATE"/>