文章目录
- 013 unity工程结构
- 014 封装C#微信中间件
- 14.1、封装中间件简介
- 14.2、WeChatComponent.cs文件的编写
- 015 支付宝中间件的封装
- 15.1、AliComponent.cs文件的编写
- 016 完成demo入口场景
- 017 微信登录
- 17.1、插入EventSystem响应单击事件
- 17.2、将WeChatComponent放入场景编写WeChatLoginTest.cs文件
- 17.3、将WeChatLoginTest文件挂载到camera
- 018 微信分享
- 18.1、编写FileComponent.cs文件
- 18.2、编写WeChatShareTest.cs文件
- 019 实现微信支付
- 19.1、camera挂载WeChatPayTest.cs文件
- 19.2、编写WeChatPayTest.cs文件
- 020 实现支付宝支付
- 20.1、camera挂载AliPayTest.cs文件
- 20.2、工程内挂载AliComponent预置体
- 20.3、编写AliPayTest.cs文件
- 021 打包操作
- 21.1、填写公司名和产品名
- 21.2、设置包名
- 21.3、入口场景放最上面
- 所有程序源码本人博客下载资源页面均有下载
013 unity工程结构
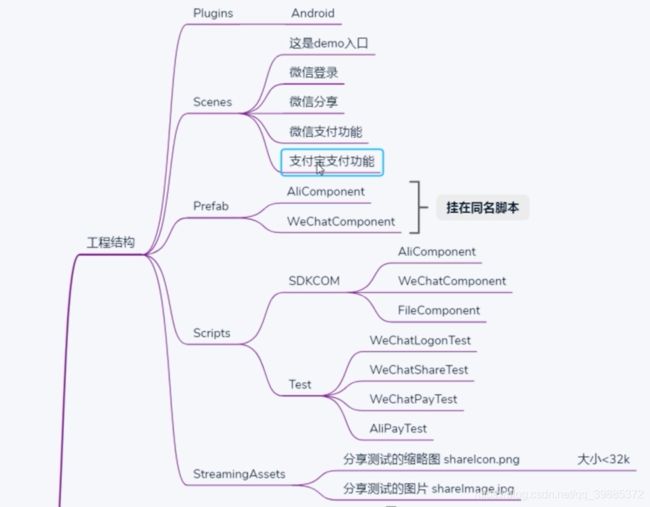
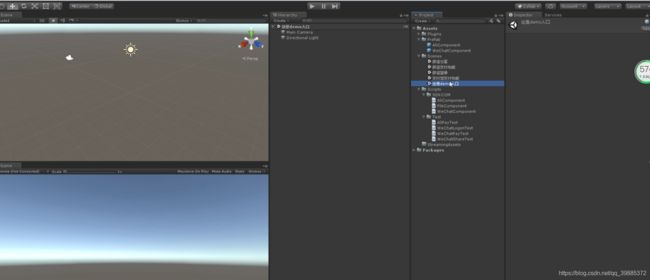
014 封装C#微信中间件
14.1、封装中间件简介
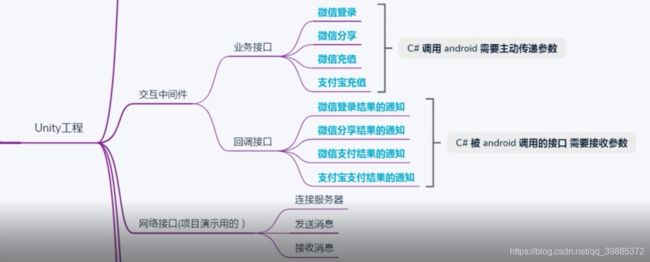
14.2、WeChatComponent.cs文件的编写
using System.Collections;
using System.Collections.Generic;
using System.Runtime.InteropServices;
using UnityEngine;
public class WeChatComponent : MonoBehaviour
{
public string WXAppID = "wxc0d38c38f13506d4";
public string WXAppSecret = "9c789be0809b1353f2fdd08e6fbaae95";
public bool isRegisterToWechat = false;
AndroidJavaClass javaClass;
AndroidJavaObject javaActive;
public string javaClassStr = "com.unity3d.player.UnityPlayer";
public string javaActiveStr = "currentActivity";
public string WeChatCallObjName = "WeChatComponent";
public delegate void WeChatLogonCallback(WeChatUserData userData);
public delegate void WeChatShareCallback(string result);
public delegate void WeChatPayCallback(int state);
public WeChatLogonCallback weChatLogonCallback;
public WeChatShareCallback weChatShareTextCallback, weChatShareImageCallback, weChatShareWebPageCallback;
public WeChatPayCallback weChatPayCallback;
#if UNITY_EDITOR
#elif UNITY_ANDROID
#elif UNITY_IPHONE
#endif
void Start()
{
#if UNITY_EDITOR
#elif UNITY_ANDROID
javaClass = new AndroidJavaClass(javaClassStr);
javaActive = javaClass.GetStatic<AndroidJavaObject>(javaActiveStr);
#elif UNITY_IPHONE
#endif
RegisterAppWechat();
}
public void RegisterAppWechat()
{
#if UNITY_EDITOR
#elif UNITY_ANDROID
if (!isRegisterToWechat)
{
javaActive.Call("WechatInit", WXAppID);
}
isRegisterToWechat=true;
#elif UNITY_IPHONE
#else
return;
#endif
}
public bool IsWechatInstalled()
{
#if UNITY_EDITOR
return false;
#elif UNITY_ANDROID
return javaActive.Call<bool>("IsWechatInstalled");
#elif UNITY_IPHONE
#else
return false;
#endif
}
public void WeChatLogon(string state)
{
#if UNITY_EDITOR
#elif UNITY_ANDROID
object[] objs = new object[] { WXAppID, state, WeChatCallObjName, "LogonCallback" };
javaActive.Call("LoginWechat", objs);
#elif UNITY_IPHONE
#endif
}
public void WeChatPay(string appid, string mchid, string prepayid, string noncestr, string timestamp, string sign)
{
#if UNITY_EDITOR
#elif UNITY_ANDROID
object[] objs = new object[] { appid, mchid, prepayid, noncestr, timestamp, sign, WeChatCallObjName, "WechatPayCallback" };
javaActive.Call("WeChatPayReq", objs);
#elif UNITY_IPHONE
#endif
}
public void WeChatShare_WebPage(int scene, string url, string title, string content, byte[] thumb)
{
#if UNITY_EDITOR
#elif UNITY_ANDROID
object[] objs = new object[] { scene, url, title,content, thumb,WeChatCallObjName,"ShareWebPage_Callback"};
javaActive.Call("WXShareWebPage", objs);
#elif UNITY_IPHONE
#endif
}
public void WeChatShare_Image(int scene, byte[] imgData, byte[] thumbData)
{
#if UNITY_EDITOR
#elif UNITY_ANDROID
object[] objs = new object[] { scene, imgData, thumbData, WeChatCallObjName, "ShareImage_Callback" };
javaActive.Call("WXShareImage", objs);
#elif UNITY_IPHONE
#endif
}
public void LogonCallback(string str)
{
if (str != "用户拒绝授权" && str != "用户取消授权")
{
Debug.Log("微信登录,用户已授权:" + str);
StartCoroutine(GetWeChatUserData(WXAppID, WXAppSecret, str));
}
if (str == "用户拒绝授权" || str == "用户取消授权")
{
Debug.Log("微信登录," + str);
weChatLogonCallback(null);
}
}
public void ShareImage_Callback(string code)
{
switch (code)
{
case "ERR_OK":
Debug.Log("分享成功");
break;
case "ERR_AUTH_DENIED":
case "ERR_USER_CANCEL":
Debug.Log("用户取消分享");
break;
default:
break;
}
weChatShareImageCallback(code);
}
public void ShareWebPage_Callback(string code)
{
switch (code)
{
case "ERR_OK":
Debug.Log("wx:网页分享成功");
break;
case "ERR_AUTH_DENIED":
case "ERR_USER_CANCEL":
Debug.Log("wx:用户取消分享网页");
break;
default:
break;
}
weChatShareWebPageCallback(code);
}
public void WechatPayCallback(string retCode)
{
int state = int.Parse(retCode);
switch (state)
{
case -2:
Debug.Log("支付取消");
break;
case -1:
Debug.Log("支付失败");
break;
case 0:
Debug.Log("支付成功");
break;
}
weChatPayCallback(state);
}
IEnumerator GetWeChatUserData(string appid, string secret, string code)
{
string url = "https://api.weixin.qq.com/sns/oauth2/access_token?appid="
+ appid + "&secret=" + secret + "&code=" + code + "&grant_type=authorization_code";
WWW www = new WWW(url);
yield return www;
if (www.error != null)
{
Debug.Log("微信登录请求令牌失败:" + www.error);
}
else
{
Debug.Log("微信登录请求令牌成功:" + www.text);
WeChatData weChatData = JsonUtility.FromJson<WeChatData>(www.text);
if (weChatData == null)
{
yield break;
}
else
{
string getuserurl = "https://api.weixin.qq.com/sns/userinfo?access_token="
+ weChatData.access_token + "&openid=" + weChatData.openid;
WWW getuser = new WWW(getuserurl);
yield return getuser;
if (getuser.error != null)
{
Debug.Log("向微信请求用户公开的信息,出现异常:" + getuser.error);
}
else
{
WeChatUserData weChatUserData = JsonUtility.FromJson<WeChatUserData>(getuser.text);
if (weChatUserData == null)
{
Debug.Log("error:" + "用户信息反序列化异常");
yield break;
}
else
{
Debug.Log("用户信息获取成功:" + getuser.text);
Debug.Log("openid:" + weChatUserData.openid + ";nickname:" + weChatUserData.nickname);
string wxOpenID = weChatUserData.openid;
string wxNickname = weChatUserData.nickname;
int sex = weChatUserData.sex;
weChatLogonCallback(weChatUserData);
}
}
}
}
}
}
[System.Serializable]
public class WeChatData
{
public string access_token;
public string expires_in;
public string refresh_token;
public string openid;
public string scope;
}
[System.Serializable]
public class WeChatUserData
{
public string openid;
public string nickname;
public int sex;
public string province;
public string city;
public string country;
public string headimgurl;
public string[] privilege;
public string unionid;
}
015 支付宝中间件的封装
15.1、AliComponent.cs文件的编写
using System.Collections;
using System.Collections.Generic;
using System.Runtime.InteropServices;
using UnityEngine;
public class AliComponent : MonoBehaviour
{
string aliSDKCallObjName = "AliComponent";
AndroidJavaClass javaClass;
AndroidJavaObject javaActive;
string javaClassStr = "com.mafeng.alinewsdk.AliSDKActivity";
string javaActiveStr = "currentActivity";
#if UNITY_EDITOR
#elif UNITY_ANDROID
#elif UNITY_IPHONE
#endif
void Start()
{
#if UNITY_EDITOR
#elif UNITY_ANDROID
javaActive = new AndroidJavaObject(javaClassStr);
#elif UNITY_IPHONE
#endif
}
public void AliPay(string OrderInfo)
{
#if UNITY_EDITOR
#elif UNITY_ANDROID
object[] objs = new object[] { OrderInfo,aliSDKCallObjName, "AliPayCallback" };
javaActive.Call("AliPay", objs);
#elif UNITY_IPHONE
#endif
}
public delegate void AliSDKAction(string result);
public AliSDKAction aliPayCallBack;
public void AliPayCallback(string result)
{
aliPayCallBack(result);
if (result == "支付成功")
{
Debug.Log("支付宝支付成功");
}
else
{
Debug.Log("支付宝支付失败");
}
}
}
016 完成demo入口场景
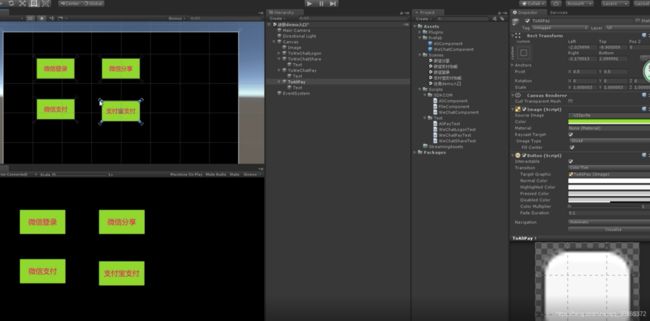
16.1、ToDemoScene.cs文件的编写
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
using UnityEngine.UI;
public class ToDemoScene : MonoBehaviour
{
void Start()
{
var btns = transform.GetComponentsInChildren<Button>();
for (int i = 0; i < btns.Length; i++)
{
string btnName = btns[i].name;
btns[i].onClick.AddListener(() =>
{
switch (btnName)
{
case "ToWeChatLogon":
SceneManager.LoadScene("微信登录");
break;
case "ToWeChatShare":
SceneManager.LoadScene("微信分享");
break;
case "ToWeChatPay":
SceneManager.LoadScene("微信支付功能");
break;
case "ToAliPay":
SceneManager.LoadScene("支付宝支付功能");
break;
default:
break;
}
});
}
}
}
017 微信登录
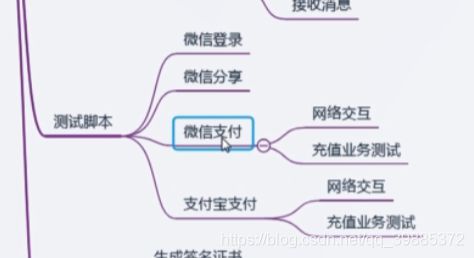
17.1、插入EventSystem响应单击事件
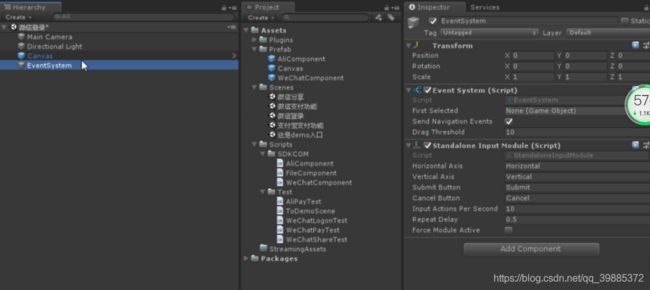
17.2、将WeChatComponent放入场景编写WeChatLoginTest.cs文件
using System;
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
using UnityEngine.UI;
public class WeChatLogonTest : MonoBehaviour
{
WeChatComponent weChatComponent;
void Start()
{
weChatComponent = GameObject.Find("WeChatComponent").GetComponent<WeChatComponent>();
transform.Find("WechatLogonBtn").GetComponent<Button>().onClick.AddListener(TestWechatLogon);
transform.Find("ReturnBtn").GetComponent<Button>().onClick.AddListener(() =>
{
SceneManager.LoadScene("这是demo入口");
});
}
private void TestWechatLogon()
{
weChatComponent.weChatLogonCallback += WeChatLogonCallback;
if (weChatComponent.IsWechatInstalled())
{
weChatComponent.WeChatLogon("mafeng");
}
else
{
}
}
private void WeChatLogonCallback(WeChatUserData userData)
{
if (userData != null)
{
transform.Find("tipsWindow/content").GetComponent<Text>().text = "登录成功,用户的OpenID:" + userData.openid + '\n'
+ "昵称:" + userData.nickname + '\n'
+ "性别:" + userData.sex;
Debug.Log("头像链接:" + userData.headimgurl);
}
else
{
transform.Find("tipsWindow/content").GetComponent<Text>().text = "用户拒绝授权或者取消登录";
}
transform.Find("tipsWindow").gameObject.SetActive(true);
weChatComponent.weChatLogonCallback -= WeChatLogonCallback;
}
}
17.3、将WeChatLoginTest文件挂载到camera
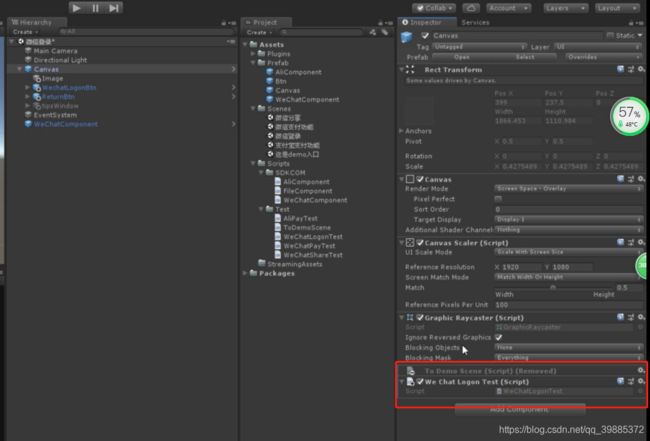
018 微信分享
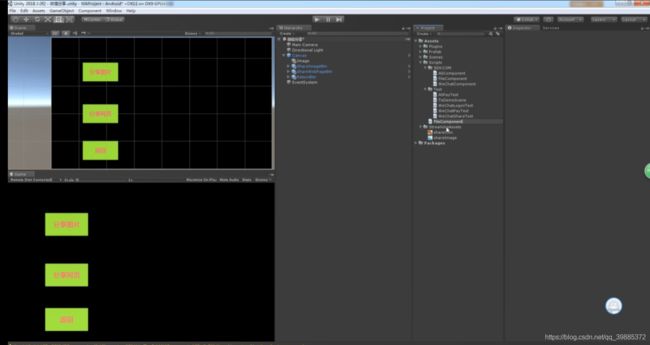
18.1、编写FileComponent.cs文件
using System.Collections;
using System.Collections.Generic;
using System.IO;
using UnityEngine;
public class FileComponent
{
public static string GetStreamingAssets(string path, bool ifwwwLoad)
{
string tempPath = "";
if (ifwwwLoad)
{
if (Application.platform == RuntimePlatform.IPhonePlayer)
{
tempPath = "file://" + Application.streamingAssetsPath + "/" + path;
}
else
{
tempPath = Application.streamingAssetsPath + "/" + path;
}
}
else
{
Debug.Log("现在并不支持通过File类去访问 如果发现可以的同学,请告诉我哦");
}
return tempPath;
}
public static string GetPersistentdatapath(string path, bool ifwwwLoad)
{
string temppath = "";
if (ifwwwLoad)
{
temppath = "file://" + Application.persistentDataPath + "/" + path;
}
else
{
temppath = Application.persistentDataPath + "/" + path;
}
return temppath;
}
}
18.2、编写WeChatShareTest.cs文件
using System;
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
using UnityEngine.UI;
public class WeChatShareTest : MonoBehaviour
{
WeChatComponent weChatComponent;
void Start()
{
weChatComponent = GameObject.Find("WeChatComponent").GetComponent<WeChatComponent>();
transform.Find("ShareImageBtn").GetComponent<Button>().onClick.AddListener(ShareImageToWeChat);
transform.Find("ShareWebPageBtn").GetComponent<Button>().onClick.AddListener(ShareWebPageToWeChat);
transform.Find("ReturnBtn").GetComponent<Button>().onClick.AddListener(() => {
SceneManager.LoadScene("这是demo入口");
});
}
private void WeChatShareWebPageCallback(string result)
{
transform.Find("contentText").GetComponent<Text>().text = "分享结果网页结果:" + result;
weChatComponent.weChatShareWebPageCallback -= WeChatShareWebPageCallback;
}
private void WeChatShareImageCallback(string result)
{
transform.Find("contentText").GetComponent<Text>().text = "分享结果图片结果:" + result;
weChatComponent.weChatShareImageCallback -= WeChatShareImageCallback;
}
public void ShareImageToWeChat()
{
weChatComponent.weChatShareImageCallback += WeChatShareImageCallback;
StartCoroutine(ShareImageToWeChat(0));
}
public void ShareWebPageToWeChat()
{
Debug.Log("分享网页");
weChatComponent.weChatShareWebPageCallback += WeChatShareWebPageCallback;
StartCoroutine(ShareWebPageToWeChat(0, @"https://www.baidu.com/", "百度一下,你就知道了",
"全球最大的中文搜索引擎、致力于让网民更便捷地获取信息."));
}
Texture2D miniTexture, mainTexture;
byte[] thumbData, mainImageData;
private IEnumerator ShareImageToWeChat(int scene)
{
if (miniTexture == null)
{
Debug.Log("分享图片");
string miniImagePath = FileComponent.GetStreamingAssets("shareIcon.png",true);
WWW loadMini = new WWW(miniImagePath);
yield return loadMini;
if (loadMini != null && string.IsNullOrEmpty(loadMini.error))
{
miniTexture = loadMini.texture;
thumbData = miniTexture.EncodeToPNG();
}
else
{
Debug.Log("error 获取不到分享的缩略图:" + loadMini.error);
}
}
if (mainTexture == null)
{
string imagePath = FileComponent.GetStreamingAssets("shareImage.jpg",true);
WWW loadMainImage = new WWW(imagePath);
yield return loadMainImage;
if (loadMainImage != null && string.IsNullOrEmpty(loadMainImage.error))
{
mainTexture = loadMainImage.texture;
mainImageData = mainTexture.EncodeToJPG();
}
else
{
Debug.Log("error 获取不到分享的图片:" + loadMainImage.error);
}
}
weChatComponent.WeChatShare_Image(scene, mainImageData, thumbData);
}
private IEnumerator ShareWebPageToWeChat(int scene, string url, string title, string content)
{
if (miniTexture == null)
{
string miniImagePath = FileComponent.GetStreamingAssets("shareIcon.png",true);
WWW loadMini = new WWW(miniImagePath);
yield return loadMini;
if (loadMini != null && string.IsNullOrEmpty(loadMini.error))
{
miniTexture = loadMini.texture;
thumbData = miniTexture.EncodeToPNG();
}
else
{
Debug.Log("error 获取不到分享的缩略图:" + loadMini.error);
}
}
weChatComponent.WeChatShare_WebPage(scene, url, title, content, thumbData);
}
}
019 实现微信支付
19.1、camera挂载WeChatPayTest.cs文件
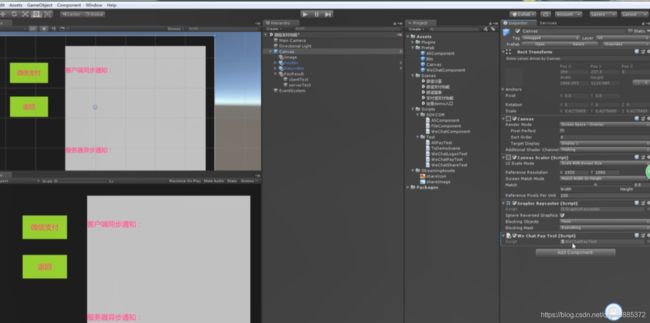
19.2、编写WeChatPayTest.cs文件
using System;
using System.Collections;
using System.Collections.Generic;
using System.Net;
using System.Net.Sockets;
using System.Text;
using System.Threading;
using UnityEngine;
using UnityEngine.SceneManagement;
using UnityEngine.UI;
public class WeChatPayTest : MonoBehaviour
{
private EndPoint remoteEndPoint;
Socket tcpSocket;
public string serverIP = "193.112.30.89";
public int serverPort = 7577;
Thread receiveThread, sendThread;
public string appid;
public string mch_id;
public string prepayid;
public string noncestr;
public string timestamp;
public string packageValue;
public string sign;
public string aliPreOrder;
public string sendMsgType = "WeChatPay";
WeChatComponent weChatComponent;
Queue<string> netMsg = new Queue<string>();
void Start()
{
weChatComponent = GameObject.Find("WeChatComponent").GetComponent<WeChatComponent>();
weChatComponent.weChatPayCallback += WeChatPayCallback;
transform.Find("ReturnBtn").GetComponent<Button>().onClick.AddListener(() =>
{
SceneManager.LoadScene("这是demo入口");
});
transform.Find("PayBtn").GetComponent<Button>().onClick.AddListener(()=> {
RequestPayment(sendMsgType, "1001", 1);
}
);
tcpSocket = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp);
remoteEndPoint = new IPEndPoint(IPAddress.Parse(serverIP), serverPort);
tcpSocket.Connect(remoteEndPoint);
receiveThread = new Thread(Receivemsg);
receiveThread.Start();
receiveThread.IsBackground = true;
}
private void WeChatPayCallback(int state)
{
string result = "";
switch (state)
{
case 0:
result = "成功";
break;
case 1:
result = "失败";
break;
case 2:
result = "取消";
break;
default:
break;
}
transform.Find("PayResult/clientText").GetComponent<Text>().text += "...收到微信客户端的支付结果:" + result;
transform.Find("PayResult").gameObject.SetActive(true);
}
public void RequestPayment(string msgType, string goodsID, int buyCount)
{
SendToServer(msgType + "," + goodsID + "," + buyCount);
}
public void Update()
{
if (netMsg.Count > 0)
{
string msg = netMsg.Dequeue();
string[] msgList = msg.Split(',');
switch (msgList[0])
{
case "WeChatPay":
Debug.Log("WeChatPay");
WechatPay(msgList);
break;
case "sendProps":
transform.Find("PayResult/serverText").GetComponent<Text>().text += "...现在收到服务器发放的道具";
transform.Find("PayResult").gameObject.SetActive(true);
string sendMsg = "getProps" + msgList[1] + "," + msgList[2] + "," + msgList[3];
SendToServer(sendMsg);
break;
default:
break;
}
}
}
private void WechatPay(string[] parameter)
{
Debug.Log("获取到微信支付参数:");
appid = parameter[1];
mch_id = parameter[2];
prepayid = parameter[3];
noncestr = parameter[4];
timestamp = parameter[5];
packageValue = parameter[6];
sign = parameter[7];
Debug.Log("执行微信支付");
weChatComponent.WeChatPay(appid, mch_id, prepayid, noncestr, timestamp, sign);
}
private void Receivemsg()
{
byte[] data = new byte[1024];
while (true)
{
int length = tcpSocket.ReceiveFrom(data, ref remoteEndPoint);
if (length > 0)
{
string message = Encoding.UTF8.GetString(data, 0, length);
netMsg.Enqueue(message);
Debug.Log("收到的服务器消息是:" + message);
}
}
}
public void SendToServer(string str)
{
string sendMsg = str;
sendThread = new Thread(() => {
byte[] data = Encoding.UTF8.GetBytes(sendMsg);
tcpSocket.SendTo(data, remoteEndPoint);
sendThread.Abort();
});
sendThread.Start();
}
void Close()
{
if (tcpSocket != null)
{
tcpSocket.Close();
}
sendThread.Abort();
receiveThread.Abort();
}
private void OnApplicationQuit()
{
Close();
}
private void OnDestroy()
{
Close();
}
}
020 实现支付宝支付
20.1、camera挂载AliPayTest.cs文件
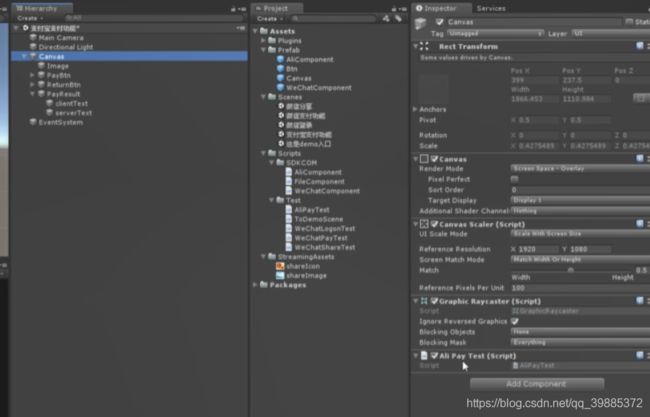
20.2、工程内挂载AliComponent预置体
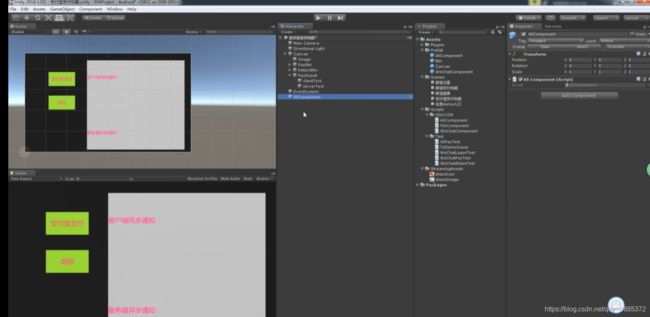
20.3、编写AliPayTest.cs文件
using System;
using System.Collections;
using System.Collections.Generic;
using System.Net;
using System.Net.Sockets;
using System.Text;
using System.Threading;
using UnityEngine;
using UnityEngine.SceneManagement;
using UnityEngine.UI;
public class AliPayTest : MonoBehaviour
{
private EndPoint remoteEndPoint;
Socket tcpSocket;
public string serverIP = "193.112.30.89";
public int serverPort = 7577;
Thread receiveThread, sendThread;
public string sendMsgType = "AliPay";
AliComponent aliComponent;
Queue<string> netMsg = new Queue<string>();
void Start()
{
aliComponent = GameObject.Find("AliComponent").GetComponent<AliComponent>();
aliComponent.aliPayCallBack += AliPayCallback;
transform.Find("ReturnBtn").GetComponent<Button>().onClick.AddListener(() =>
{
SceneManager.LoadScene("这是demo入口");
});
transform.Find("PayBtn").GetComponent<Button>().onClick.AddListener(() => {
RequestPayment(sendMsgType, "1001", 1);
}
);
tcpSocket = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp);
remoteEndPoint = new IPEndPoint(IPAddress.Parse(serverIP), serverPort);
tcpSocket.Connect(remoteEndPoint);
receiveThread = new Thread(Receivemsg);
receiveThread.Start();
receiveThread.IsBackground = true;
}
private void AliPayCallback(string result)
{
if (result == "支付成功")
{
Debug.Log("支付宝支付成功");
}
else
{
Debug.Log("支付宝支付失败");
}
transform.Find("PayResult/clientText").GetComponent<Text>().text += "...收到支付宝支付结果:" + result;
transform.Find("PayResult").gameObject.SetActive(true);
}
public void RequestPayment(string msgType, string goodsID, int buyCount)
{
SendToServer(msgType + "," + goodsID + "," + buyCount);
}
public void Update()
{
if (netMsg.Count > 0)
{
string msg = netMsg.Dequeue();
string[] msgList = msg.Split(',');
switch (msgList[0])
{
case "AliPay":
Debug.Log("AliPay");
AliPay(msgList);
break;
case "sendProps":
transform.Find("PayResult/serverText").GetComponent<Text>().text += "...现在收到服务器发放的道具";
transform.Find("PayResult").gameObject.SetActive(true);
string sendMsg = "getProps" + msgList[1] + "," + msgList[2] + "," + msgList[3];
SendToServer(sendMsg);
break;
default:
break;
}
}
}
private void AliPay(string[] parameter)
{
Debug.Log("执行支付宝支付");
aliComponent.AliPay(parameter[1]);
}
private void Receivemsg()
{
byte[] data = new byte[1024];
while (true)
{
int length = tcpSocket.ReceiveFrom(data, ref remoteEndPoint);
if (length > 0)
{
string message = Encoding.UTF8.GetString(data, 0, length);
netMsg.Enqueue(message);
Debug.Log("收到的服务器消息是:" + message);
}
}
}
public void SendToServer(string str)
{
string sendMsg = str;
sendThread = new Thread(() => {
byte[] data = Encoding.UTF8.GetBytes(sendMsg);
tcpSocket.SendTo(data, remoteEndPoint);
sendThread.Abort();
});
sendThread.Start();
}
void Close()
{
if (tcpSocket != null)
{
tcpSocket.Close();
}
sendThread.Abort();
receiveThread.Abort();
}
private void OnApplicationQuit()
{
Close();
}
private void OnDestroy()
{
Close();
}
}
021 打包操作
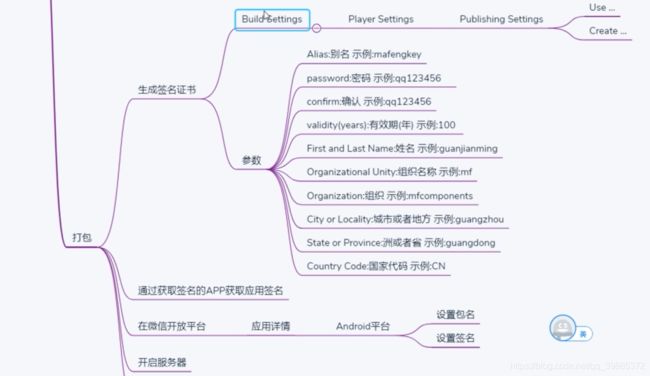
21.1、填写公司名和产品名
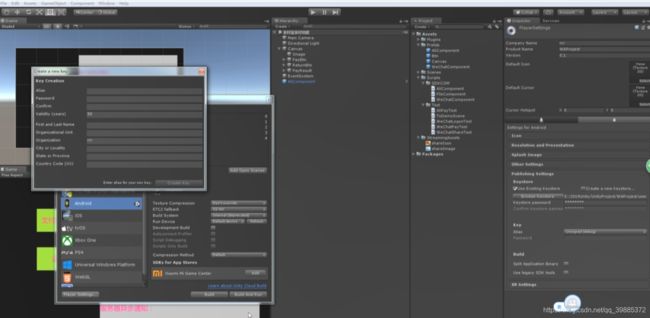
21.2、设置包名
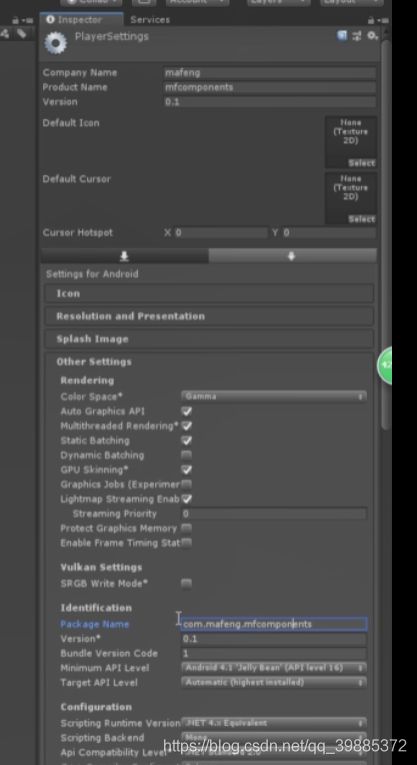
21.3、入口场景放最上面
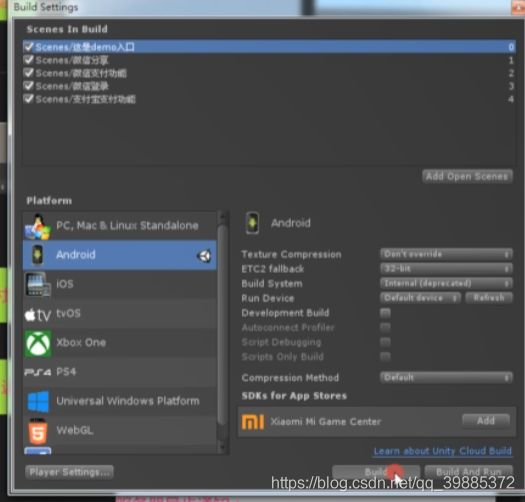
所有程序源码本人博客下载资源页面均有下载