#include
#include
#include
using namespace std;
#define OK 1
#define ERROR 0
typedef int Status ;
typedef int Elemtype;
typedef struct LNode
{
Elemtype data;
struct LNode * next;
}LNode, *LinkList;
void InitList(LinkList &head)
{
head = (LinkList)malloc(sizeof(LNode));
head->next = NULL;
}
void CreatList(LinkList &head)
{
printf("开始头插");
printf("链表开始创建以0结尾");
int x;
LinkList s;
scanf_s("%d",&x);
system("cls");
while (x != 0)
{
s = (LinkList)malloc(sizeof(LNode));
s->data = x;
s->next = head->next;
head->next = s;
scanf_s("%d",&x);
system("cls");
}
}
void CreatList_tail (LinkList &head)
{
LinkList s;
LinkList p = head;
int x;
printf("开始尾插");
printf("链表开始创建以0结尾");
scanf_s("%d", &x);
system("cls");
while (x != 0)
{
s = (LinkList)malloc(sizeof(LNode));
s->data = x;
s->next = NULL;
if (head->next==NULL )
{
head->next = s;
}
else
{
p->next = s;
}
p = s;
scanf_s("%d", &x);
system("cls");
}
}
void print(LinkList &head)
{
LinkList p=head;
if (!head->next)
{
printf("ERROR");
}
else
{
while (p->next != NULL)
{
p = p->next;
printf("%d\t", p->data);
}
}
}
LinkList Locate(LinkList head,int i)
{
if (i < 0)
return NULL;
LinkList p=head;
int k=0;
while (p != NULL && k < i)
{
p = p->next;
k++;
}
return p;
}
void InsertList(LinkList &head,int i,Elemtype e)
{
if (i < 0)
printf("error\n");
else
{
LinkList s = (LinkList)malloc(sizeof(LNode));
s->data = e;
s->next = NULL;
LinkList p = Locate(head,i-1);
if (p)
{
s->next = p->next;
p->next = s;
}
else printf("ERROR");
}
}
void DeleteList(LinkList &head,int i)
{
LinkList p = Locate(head, i - 1);
if (i < 0)
printf("error\n");
else
{
if (p == NULL)
printf("ERROR");
else
p->next = p->next->next;
}
}
void ChangeList(LinkList &head,int i,Elemtype e)
{
if (i < 0)
printf("ERROR");
else
{
LinkList p = Locate(head,i);
if (p != NULL)
{
p->data = e;
}
else printf("ERROR");
}
}
int main()
{
LinkList head = NULL;
InitList(head);
printf("您打算用头插还是尾插,头插请按1,尾插请按2\n");
int tag;
int turn = 0;
do{
scanf_s("%d", &tag);
if (tag == 1)
{
CreatList(head);
turn = 1;
}
else if (tag == 2)
{
CreatList_tail(head);
turn = 1;
}
else
{
printf("输入值不合法,重新输入");
}
} while (turn = 0);
print(head);
printf("链表建立完毕\n");
int loc, val;
char m;
LinkList value;
while (1)
{
print(head);
printf("\n插入请按A,删除值请按B,定位请按C,修改请按D");
cin >> m;
switch (m)
{
case 'A':
printf("开始插入,请输入插入值和位置");
scanf_s("%d,%d", &val, &loc);
InsertList(head, loc, val);
system("cls");
break;
case 'B':
printf("开始删除,请输入删除位置");
scanf_s("%d", &loc);
DeleteList(head, loc);
system("cls");
break;
case 'C':
printf("请输入位");
scanf_s("%d", &val);
printf("当前定位值如下");
value = Locate(head,val);
printf("%d\n",value->data);
break;
case 'D':
printf("您想更改哪个位置,请输入更改值和位置");
scanf_s("%d,%d", &val, &loc);
ChangeList(head, loc, val);
system("cls");
break;
default:
exit(0);
}
}
return 0;
}
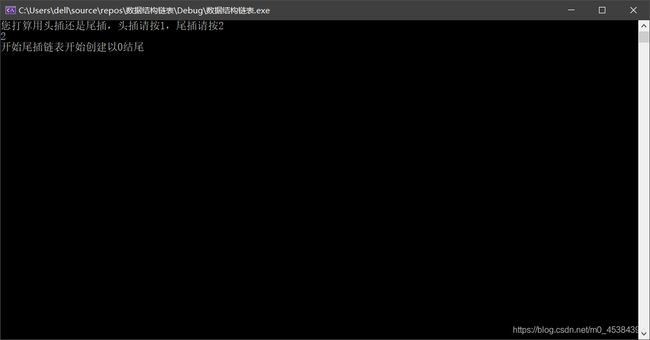
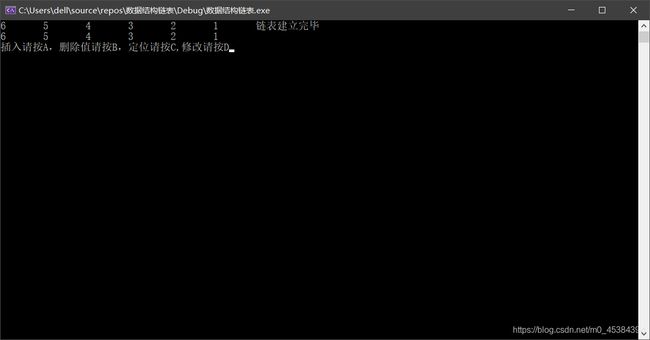
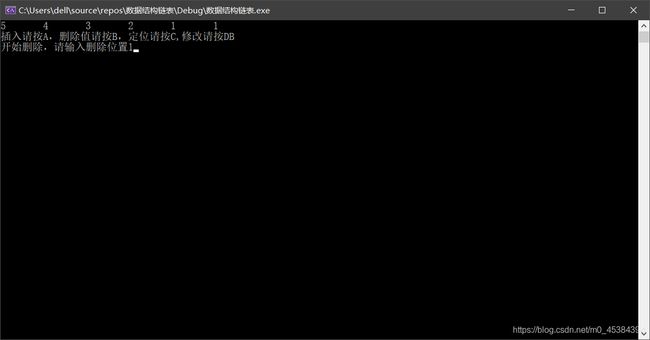