from cv2 import cv2
import numpy as np
import camera_configs
cap = cv2.VideoCapture(0)
cap.set(3, 2560)
cap.set(4, 720)
cv2.namedWindow("depth")
cv2.namedWindow("left")
cv2.namedWindow("right")
cv2.moveWindow("left", 0, 0)
cv2.moveWindow("right", 600, 0)
cv2.namedWindow("config", cv2.WINDOW_NORMAL)
cv2.createTrackbar("num", "config", 0, 60, lambda x: None)
cv2.createTrackbar("blockSize", "config", 94, 255, lambda x: None)
cv2.createTrackbar("SpeckleWindowSize", "config", 1, 10, lambda x: None)
cv2.createTrackbar("SpeckleRange", "config", 1, 255, lambda x: None)
cv2.createTrackbar("UniquenessRatio", "config", 1, 255, lambda x: None)
cv2.createTrackbar("TextureThreshold", "config", 1, 255, lambda x: None)
cv2.createTrackbar("UniquenessRatio", "config", 1, 255, lambda x: None)
cv2.createTrackbar("MinDisparity", "config", 0, 255, lambda x: None)
cv2.createTrackbar("PreFilterCap", "config", 1, 62, lambda x: None)
cv2.createTrackbar("MaxDiff", "config", 1, 400, lambda x: None)
def callbackFunc(e, x, y, f, p):
if e == cv2.EVENT_LBUTTONDOWN:
print(threeD[y][x])
cv2.setMouseCallback("depth", callbackFunc, None)
frame_rate_calc = 1
freq = cv2.getTickFrequency()
font = cv2.FONT_HERSHEY_SIMPLEX
imageCount = 1
while True:
ret, frame = cap.read()
frame1 = frame[0:720, 0:1280]
frame2 = frame[0:720, 1280:2560]
if not ret:
print("camera is not connected!")
break
t1 = cv2.getTickCount()
img1_rectified = cv2.remap(frame1, camera_configs.left_map1, camera_configs.left_map2, cv2.INTER_LINEAR,
cv2.BORDER_CONSTANT)
img2_rectified = cv2.remap(frame2, camera_configs.right_map1, camera_configs.right_map2, cv2.INTER_LINEAR,
cv2.BORDER_CONSTANT)
imgL = cv2.cvtColor(img1_rectified, cv2.COLOR_BGR2GRAY)
imgR = cv2.cvtColor(img2_rectified, cv2.COLOR_BGR2GRAY)
out = np.hstack((img1_rectified, img2_rectified ))
for i in range(0, out.shape[0], 30):
cv2.line(out, (0, i), (out.shape[1], i), (0, 255, 0), 1)
num = cv2.getTrackbarPos("num", "config")
SpeckleWindowSize = cv2.getTrackbarPos("SpeckleWindowSize", "config")
SpeckleRange = cv2.getTrackbarPos("SpeckleRange", "config")
blockSize = cv2.getTrackbarPos("blockSize", "config")
UniquenessRatio = cv2.getTrackbarPos("UniquenessRatio", "config")
TextureThreshold = cv2.getTrackbarPos("TextureThreshold", "config")
MinDisparity = cv2.getTrackbarPos("MinDisparity", "config")
PreFilterCap = cv2.getTrackbarPos("PreFilterCap", "config")
MaxDiff = cv2.getTrackbarPos("MaxDiff", "config")
if blockSize % 2 == 0:
blockSize += 1
if blockSize < 5:
blockSize = 5
if PreFilterCap>63 :
PreFilterCap = 63
if PreFilterCap<1:
PreFilterCap = 1
stereo = cv2.StereoBM_create(
numDisparities=16 * num,
blockSize=blockSize,
)
stereo.setROI1(camera_configs.validPixROI1)
stereo.setROI2(camera_configs.validPixROI2)
stereo.setPreFilterCap(PreFilterCap)
stereo.setMinDisparity(MinDisparity)
stereo.setTextureThreshold(TextureThreshold)
stereo.setUniquenessRatio(UniquenessRatio)
stereo.setSpeckleWindowSize(SpeckleWindowSize)
stereo.setSpeckleRange(SpeckleRange)
stereo.setDisp12MaxDiff(MaxDiff)
disparity = stereo.compute(imgL, imgR)
disp = cv2.normalize(disparity, disparity, alpha=0, beta=255, norm_type=cv2.NORM_MINMAX, dtype=cv2.CV_8U)
threeD = cv2.reprojectImageTo3D(disparity.astype(np.float32) / 16., camera_configs.Q)
fakeColorDepth = cv2.applyColorMap(disp, cv2.COLORMAP_JET)
cv2.putText(frame1, "FPS: {0:.2f}".format(frame_rate_calc), (30, 50), font, 1, (255, 255, 0), 2, cv2.LINE_AA)
interrupt = cv2.waitKey(10)
if interrupt & 0xFF == 27:
break
if interrupt & 0xFF == ord('s'):
cv2.imwrite('images/left' + '.jpg', frame1)
cv2.imwrite('images/right' + '.jpg', frame2)
cv2.imwrite('images/img1_rectified' + '.jpg', img1_rectified)
cv2.imwrite('images/img2_rectified' + '.jpg', img2_rectified)
cv2.imwrite('images/depth' + '.jpg', disp)
cv2.imwrite('images/fakeColor' + '.jpg', fakeColorDepth)
cv2.imwrite('mages/epipolar' + '.jpg', out)
cv2.imshow("depth", disp)
cv2.imshow("fakeColor", fakeColorDepth)
img_medianBlur = cv2.medianBlur(disp, 25)
img_medianBlur_fakeColorDepth = cv2.applyColorMap(img_medianBlur, cv2.COLORMAP_JET)
img_GaussianBlur = cv2.GaussianBlur(disp, (7, 7), 0)
img_Blur = cv2.blur(disp, (5, 5))
cv2.imshow("img_GaussianBlur", img_GaussianBlur)
cv2.imshow("img_medianBlur_fakeColorDepth", img_medianBlur_fakeColorDepth)
cv2.imshow("img_Blur", img_Blur)
cv2.imshow("img_medianBlur", img_medianBlur)
t2 = cv2.getTickCount()
time1 = (t2 - t1) / freq
frame_rate_calc = 1 / time1
cv2.putText(out, "FPS: {0:.2f}".format(frame_rate_calc), (30, 50), font, 1, (255, 255, 0), 2, cv2.LINE_AA)
cv2.imshow("epipolar lines", out)
cap.release()
cv2.destroyAllWindows()
能够显示FPS
通过调整滑块,使得具有良好的视差图。
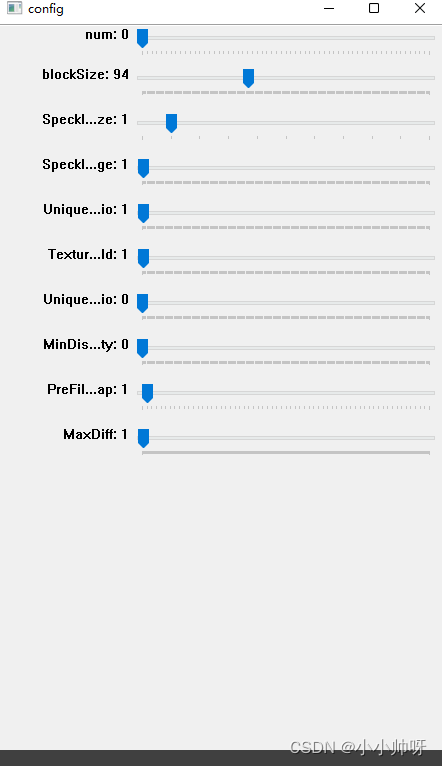