7. Design a negative edge-triggered D-flipflop(D_FF) with synchronous clear, active high (D_FF clears only at a negative edge of clock when clear is high). Use behavioral statements only. (Hint: Output q of D_FF must be declared as reg). Design a clock with a period of 10 units and test the D_FF.
my answer:
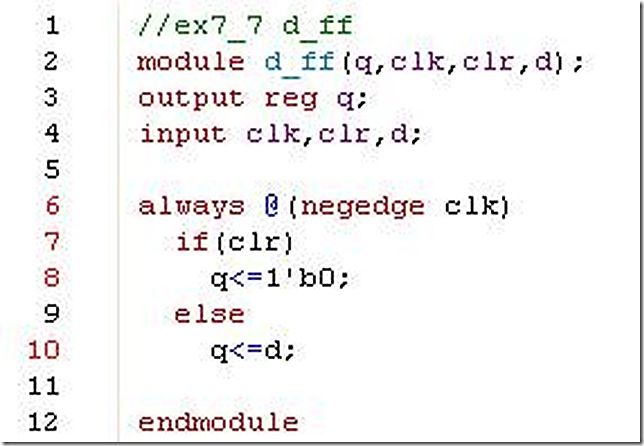
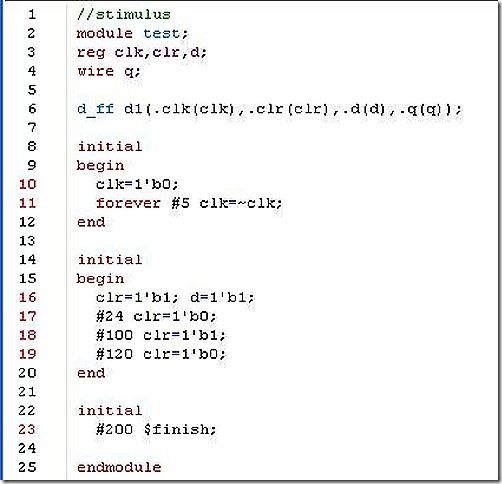
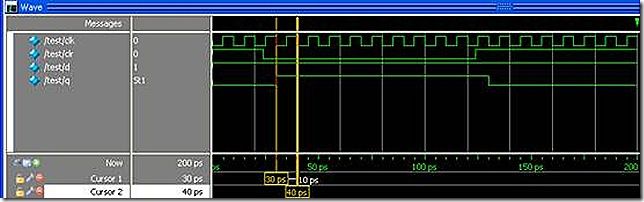
8. Design the D-flipflop in exercise 7 with asynchronous clear (D_FF clears whenever clear goes high. It does not wait for next negative edge). Test the D_FF.
my answer:
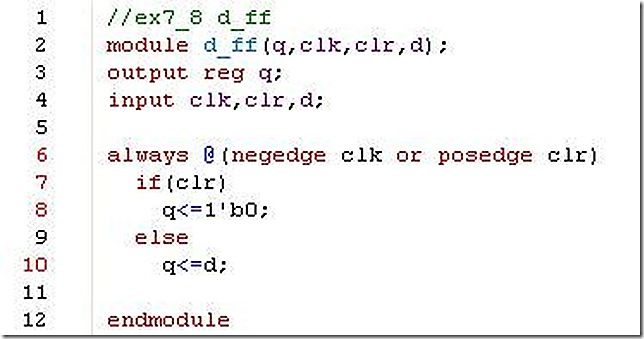
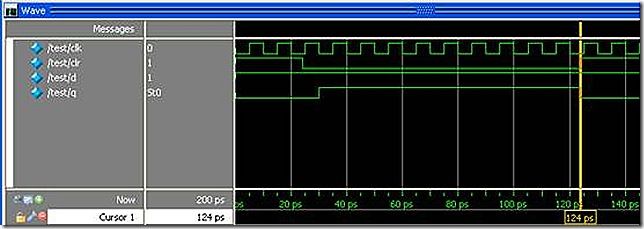
9. Using the wait statement, design a level-sensitive latch that takes clock and d as inputs and q as output. q=d whenever clock=1.
my answer:
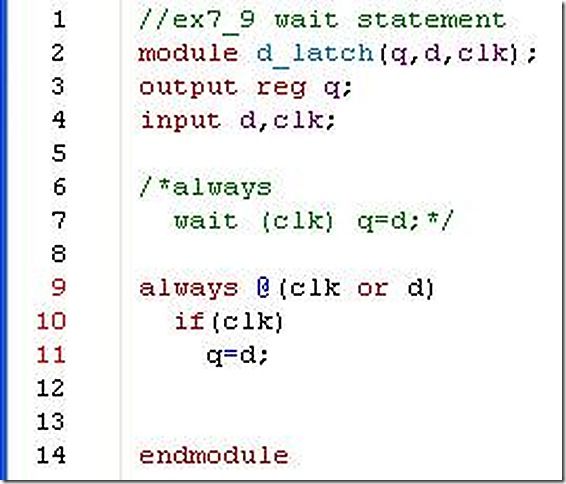
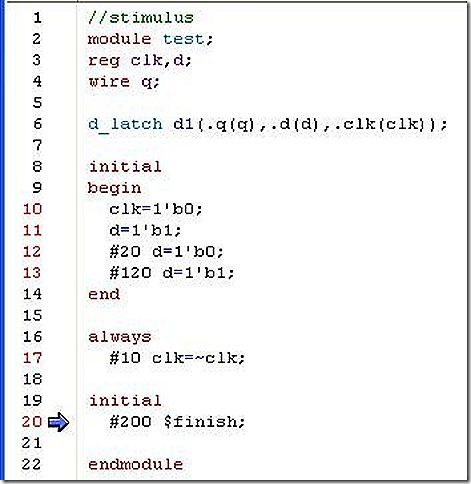

(p.s. 在modelsim 6.5b里测试,if可以仿真,wait会卡死。L).
10. Design the 4-to-1 multiplexer in eg 7-19 by using if and else statements. The port interface must remain the same.
my answer:
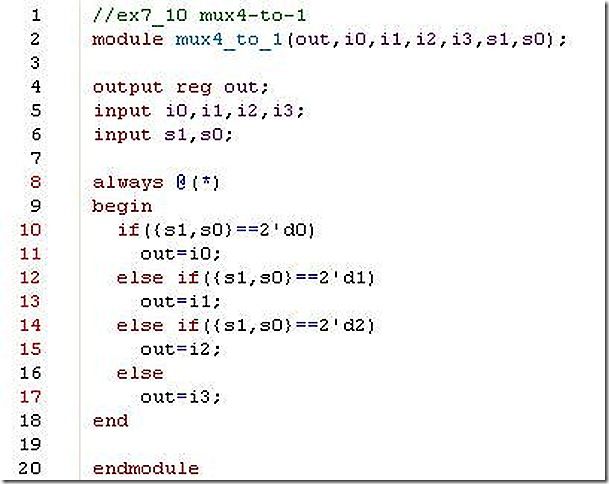
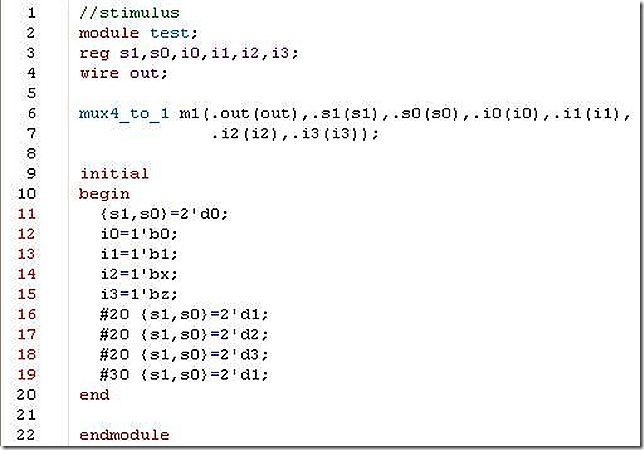
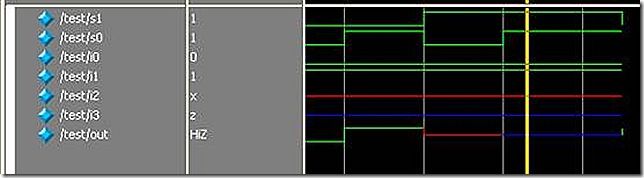
11. Design the traffic signal controller discussed in this chapter by using if and else statements.
my answer:
1
`define TRUE
1
'
b1;
2
3
`define FALSE
1
'
b0;
4
5
//
Delays
6
7
`define Y2RDELAY
3
//
Yellow to red delay
8
9
`define R2GDELAY
2
//
Red to green delay
10
11
module
sig_control
12
13
(hwy,cntry,X,clock,clear);
14
15
//
I/O ports
16
17
output
[
1
:
0
] hwy, cntry;
18
19
//
2-bit output for 3 states of signal
20
21
//
GREEN, YELLOW, RED
22
23
reg
[
1
:
0
] hwy,cntry;
24
25
//
declared output signals are registers
26
27
input
X;
28
29
//
if TRUE, indicates that there is car on
30
31
//
the country road, otherwise FALSE
32
33
input
clock,clear;
34
35
parameter
RED
=
2
'
d0,
36
37
YELLOW
=
2
'
d1,
38
39
GREEN
=
2
'
d2;
40
41
//
State definition HWY CONTRY
42
43
parameter
S0
=
3
'
d0, //GREEN RED
44
45
S1
=
3
'
d1, //YELLOW RED
46
47
S2
=
3
'
d2, //RED RED
48
49
S3
=
3
'
d3, //RED GREEN
50
51
S4
=
3
'
d4; //RED YELLOW
52
53
//
Internal state variables
54
55
reg
[
2
:
0
] state;
56
57
reg
[
2
:
0
] next_state;
58
59
//
state changes only at postive edge of clock
60
61
always
@(
posedge
clock)
62
63
if
(clear)
64
65
state
<=
S0;
//
Controller starts in S0 state
66
67
else
68
69
state
<=
next_state;
//
State change
70
71
//
Compute values of main signal and country signal
72
73
always
@(state)
74
75
begin
76
77
//
case(state)
78
79
//
S0: ;
//
No change, use default
80
81
if
(state
==
S1)
82
83
hwy
=
YELLOW;
84
85
else
if
(state
==
S2)
86
87
hwy
=
RED;
88
89
else
if
(state
==
S3)
90
91
begin
92
93
hwy
=
RED;
94
95
cntry
=
GREEN;
96
97
end
98
99
else
if
(state
==
S4)
100
101
begin
102
103
hwy
=
RED;
104
105
cntry
=
YELLOW;
106
107
end
108
109
else
110
111
begin
112
113
hwy
=
GREEN;
//
Default Light Assignment for Highway light
114
115
cntry
=
RED;
//
Default light Assignment for Country light
116
117
end
118
119
end
120
121
//
State machine using case statements
122
123
always
@(state
or
X)
124
125
begin
126
127
if
(state
==
S0)
128
129
if
(X)
130
131
next_state
=
S1;
132
133
else
134
135
next_state
=
S0;
136
137
else
if
(state
==
S1)
138
139
begin
//
delay some positive edges of clock
140
141
repeat
(`Y2RDELAY) @(
posedge
clock);
142
143
next_state
=
S2;
144
145
end
146
147
else
if
(state
==
S2)
148
149
begin
//
delay some positive edges of clock
150
151
repeat
(`R2GDELAY) @(
posedge
clock);
152
153
next_state
=
S3;
154
155
end
156
157
else
if
(state
==
S3)
158
159
if
(X)
160
161
next_state
=
S3;
162
163
else
164
165
next_state
=
S4;
166
167
else
168
169
begin
//
delay some positive edges of clock
170
171
repeat
(`Y2RDELAY) @(
posedge
clock);
172
173
next_state
=
S0;
174
175
end
176
177
//
default:next_state=S0;
178
179
//
endcase
180
181
end
182
183
endmodule