1.需求:需要请示查询数据库的大表情况,以便进行拆分表
2.方案:a. 云商的DBA 表空间分析 b.自己写sql查询【这里以自己动手写为案例】
3.思路:查询出排名前15的数据库表的行数,数据容量,索引容量
4.代码部分
from email.header import Header
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
from email import encoders
from email.mime.base import MIMEBase
import smtplib, os
import pymysql, xlwt
import time, datetime
class CreateExcel(object):
'''查询数据库并生成Excel文档'''
def __init__(self, mysql_info):
self.mysql_info = mysql_info
self.conn = pymysql.connect(host = self.mysql_info['host'], port = self.mysql_info['port'],
user = self.mysql_info['user'], passwd = self.mysql_info['passwd'],
db = self.mysql_info['db'], charset='utf8')
self.cursor = self.conn.cursor()
def getUserData(self, sql):
self.cursor.execute(sql)
table_desc = self.cursor.description
result = self.cursor.fetchall()
if not result:
print('没数据。')
print('数据库查询完毕'.center(30, '#'))
return result, table_desc
def writeToExcel(self, data, filename):
result, fileds = data
wbk = xlwt.Workbook(encoding='utf-8')
sheet1 = wbk.add_sheet('sheet1', cell_overwrite_ok=True)
for filed in range(len(fileds)):
sheet1.write(0, filed, fileds[filed][0])
for row in range(1, len(result)+1):
for col in range(0, len(fileds)):
sheet1.write(row, col, result[row-1][col])
wbk.save(filename)
def close(self):
self.cursor.close()
self.conn.close()
print('关闭数据库连接'.center(30, '#'))
class SendMail(object):
'''将Excel作为附件发送邮件'''
def __init__(self, email_info):
self.email_info = email_info
self.smtp = smtplib.SMTP_SSL(self.email_info['server'], self.email_info['port'])
self._attachements = []
self._from = ''
def login(self):
self._from = self.email_info['user']
self.smtp.login(self.email_info['user'], self.email_info['password'])
def add_attachment(self):
file_path = self.email_info['file_path']
with open(file_path, 'rb') as file:
filename = os.path.split(file_path)[1]
mime = MIMEBase('application', 'octet-stream', filename=filename)
mime.add_header('Content-Disposition', 'attachment', filename=('gbk', '', filename))
mime.add_header('Content-ID', '<0>')
mime.add_header('X-Attachment-Id', '0')
mime.set_payload(file.read())
encoders.encode_base64(mime)
self._attachements.append(mime)
def sendMail(self):
msg = MIMEMultipart()
contents = MIMEText(self.email_info['content'], 'plain', 'utf-8')
msg['From'] = self.email_info['user']
msg['To'] = self.email_info['to']
msg['Subject'] = self.email_info['subject']
for att in self._attachements:
msg.attach(att)
msg.attach(contents)
try:
self.smtp.sendmail(self._from, self.email_info['to'].split(','), msg.as_string())
print('邮件发送成功,请注意查收'.center(30, '#'))
print("%s:success"%nowdate)
except Exception as e:
print('Error:', e)
print("%s:error"%nowdate)
def close(self):
self.smtp.quit()
print('logout'.center(30, '#'))
if __name__ == '__main__':
nowdate=datetime.date.today()
oneday=datetime.timedelta(days=1)
yesterday=nowdate-oneday
mysql_dict = {
'host': '192.168.1.204',
'port': 3306,
'user': 'read',
'passwd': 'Read@123',
'db': 'example-db'
}
email_dict = {
"user": "[email protected]",
"to": "[email protected],[email protected]",
"server": "mail.wisepool.com.cn",
'port': 465,
"username": "[email protected]",
"password": "1234",
"subject": "生产数据库按容量排序情况-%s"%nowdate,
"content": '数据见附件--运维小组',
'file_path': '%s-example.xls'%nowdate
}
not_email_dict = {
"user": "[email protected]",
"to": "[email protected],[email protected]",
"server": "mail.wisepool.com.cn",
'port': 465,
"username": "[email protected]",
"password": "1234",
"subject": "生产数据库按容量排序情况-%s"%nowdate,
"content": '%s暂无数据导出---运维小组'%nowdate,
}
sql ='''select
table_schema as '数据库',
table_name as '表名',
table_rows as '记录数',
truncate(data_length/1024/1024, 2) as '数据容量(MB)',
truncate(index_length/1024/1024, 2) as '索引容量(MB)'
from information_schema.tables
order by data_length desc, index_length desc limit 15;'''
create_excel = CreateExcel(mysql_dict)
sql_res = create_excel.getUserData(sql)
if not sql_res[0]:
sendmail = SendMail(not_email_dict)
sendmail.login()
sendmail.sendMail()
sendmail.close()
else:
create_excel.writeToExcel(sql_res,email_dict['file_path'])
create_excel.close()
sendmail = SendMail(email_dict)
sendmail.login()
sendmail.add_attachment()
sendmail.sendMail()
sendmail.close()
5.效果图
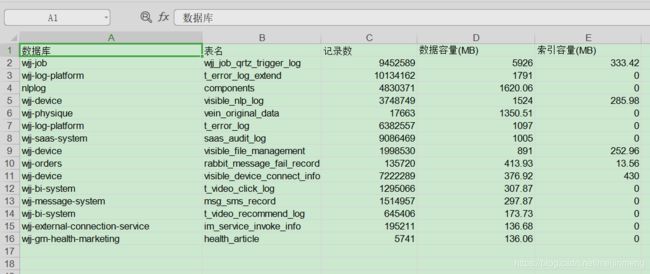