一、BaseExecuteDao接口
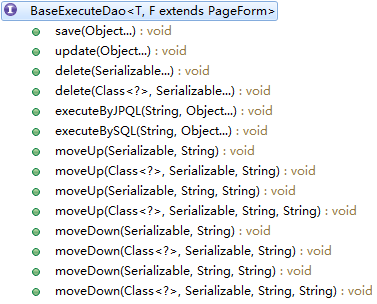
/**
*
* 通用的操作类型方法接口
*
* @param <T> 后台实体
* @param <F> 前台Form(包含分页信息)
* @author chendejiang
*/
public interface BaseExecuteDao<T,F extends PageForm> {
/**
* 保存一个或多个实体
* @param entities 一个或多个实体
*/
public void save(Object... entities);
/***
* 更新一个或多个实体
* @param entities 一个或多个实体
*/
public void update(Object... entities);
/***
* 根据一个或多个主键删除实体
* @param ids 一个或多个主键
*/
public void delete(Serializable... ids);
/**
* 根据一个或多个主键删除指定实体
* @param entityClass 实体Bean的类型。如User.class
* @param ids 一个或多个主键
*/
public void delete(Class<?> entityClass,Serializable... ids);
/**
* 执行JPQL语句
* @param jpql JPQL语句
* @param params
*/
public void executeByJPQL(String jpql,Object... params);
/**
* 执行SQL语句
* @param sql SQL语句
* @param params
*/
public void executeBySQL(String sql,Object... params);
/**
* 上移
* @param id 主键
* @param sortPropertyName 排序的实体属性名
*/
public void moveUp(Serializable id,String sortPropertyName);
/**
* 上移
* @param id 主键
* @param entityClass 实体Bean的类型。如User.class
* @param sortPropertyName 排序的实体属性名
*/
public void moveUp(Class<?> entityClass,Serializable id,String sortPropertyName);
/**
* 上移
* @param id 主键
* @param sortPropertyName 排序的实体属性名
* @param parentPropertyName 父节点属性名
*/
public void moveUp(Serializable id,String sortPropertyName,String parentPropertyName);
/**
* 上移
* @param entityClass 实体Bean的类型。如User.class
* @param id 主键
* @param sortPropertyName 排序的实体属性名
* @param parentPropertyName 父节点属性名
*/
public void moveUp(Class<?> entityClass,Serializable id,String sortPropertyName,String parentPropertyName);
/**
* 下移
* @param id 主键
* @param sortPropertyName 排序的实体属性名
*/
public void moveDown(Serializable id,String sortPropertyName);
/**
* 下移
* @param id 主键
* @param entityClass 实体Bean的类型。如User.class
* @param sortPropertyName 排序的实体属性名
*/
public void moveDown(Class<?> entityClass,Serializable id,String sortPropertyName);
/**
* 下移
* @param id 主键
* @param sortPropertyName 排序的实体属性名
* @param parentPropertyName 父节点属性名
*/
public void moveDown(Serializable id,String sortPropertyName,String parentPropertyName);
/**
* 下移
* @param entityClass 实体Bean的类型。如User.class
* @param id 主键
* @param sortPropertyName 排序的实体属性名
* @param parentPropertyName 父节点属性名
*/
public void moveDown(Class<?> entityClass,Serializable id,String sortPropertyName,String parentPropertyName);
}
二、BaseExecuteDaoImpl类
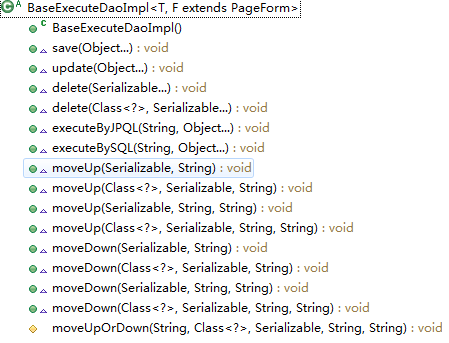
public abstract class BaseExecuteDaoImpl<T,F extends PageForm> extends BaseQueryDaoImpl<T, F> implements BaseExecuteDao<T,F> {
/* (non-Javadoc)
* @see com.nenglong.base.dao.BaseExecuteDao#save(java.lang.Object[])
*/
public void save(final Object... entities) {
if(entities!=null){
String idName;
Object value;
int i=-1;
for (Object entity : entities) {
em.persist(entity);
idName = getIdPropertyName(entity.getClass());
try {
value = PropertyUtils.getProperty(entity, idName);
PropertyUtils.setProperty(entities[++i], idName, value);//设置主键值
} catch (Exception e) {
e.printStackTrace();
}
}
}
}
/* (non-Javadoc)
* @see com.nenglong.base.dao.BaseExecuteDao#update(java.lang.Object[])
*/
public void update(final Object... entities) {
if(entities!=null){
Object bean;
String idName;
Object value;
for (Object entity : entities) {
bean = em.merge(entity);
idName = getIdPropertyName(entity.getClass());
try {
value = PropertyUtils.getProperty(bean, idName);
PropertyUtils.setProperty(entity, idName, value);//设置主键值
} catch (Exception e) {
e.printStackTrace();
}
}
}
}
/* (non-Javadoc)
* @see com.nenglong.base.dao.BaseExecuteDao#delete(java.io.Serializable[])
*/
public void delete(final Serializable... ids) {
delete(this.entityClass, ids);
}
/* (non-Javadoc)
* @see com.nenglong.base.dao.BaseExecuteDao#delete(java.lang.Class, java.io.Serializable[])
*/
public void delete(final Class<?> entityClass, final Serializable... ids) {
if(ids!=null){
for (Serializable id : ids) {
em.remove(em.getReference(entityClass, id));
}
}
}
/* (non-Javadoc)
* @see com.nenglong.base.dao.BaseExecuteDao#executeByJPQL(java.lang.String, java.lang.Object[])
*/
public void executeByJPQL(final String jpql, final Object... params) {
Query query = em.createQuery(convertJPQL(jpql, params));
setParameters(query, params);
query.executeUpdate();
}
/* (non-Javadoc)
* @see com.nenglong.base.dao.BaseExecuteDao#executeBySQL(java.lang.String, java.lang.Object[])
*/
public void executeBySQL(final String sql, final Object... params) {
Query query = em.createNativeQuery(sql);
setParameters(query, params);
query.executeUpdate();
}
/* (non-Javadoc)
* @see com.nenglong.base.dao.BaseExecuteDao#moveUp(java.io.Serializable, java.lang.String)
*/
public void moveUp(final Serializable id, final String sortPropertyName) {
moveUp(entityClass, id, sortPropertyName);
}
/* (non-Javadoc)
* @see com.nenglong.base.dao.BaseExecuteDao#moveUp(java.lang.Class, java.io.Serializable, java.lang.String)
*/
public void moveUp(final Class<?> entityClass, final Serializable id, final String sortPropertyName) {
moveUp(entityClass, id, sortPropertyName, null);
}
/* (non-Javadoc)
* @see com.nenglong.base.dao.BaseExecuteDao#moveUp(java.io.Serializable, java.lang.String, java.lang.String)
*/
public void moveUp(final Serializable id, final String sortPropertyName, final String parentPropertyName) {
moveUp(entityClass, id, sortPropertyName, parentPropertyName);
}
/* (non-Javadoc)
* @see com.nenglong.base.dao.BaseExecuteDao#moveUp(java.lang.Class, java.io.Serializable, java.lang.String, java.lang.String)
*/
public void moveUp(final Class<?> entityClass, final Serializable id, final String sortPropertyName, final String parentPropertyName) {
moveUpOrDown("UP", entityClass, id, sortPropertyName, parentPropertyName);
}
/* (non-Javadoc)
* @see com.nenglong.base.dao.BaseExecuteDao#moveDown(java.io.Serializable, java.lang.String)
*/
public void moveDown(final Serializable id, final String sortPropertyName) {
moveDown(entityClass, id, sortPropertyName);
}
/* (non-Javadoc)
* @see com.nenglong.base.dao.BaseExecuteDao#moveDown(java.lang.Class, java.io.Serializable, java.lang.String)
*/
public void moveDown(final Class<?> entityClass, final Serializable id, final String sortPropertyName) {
moveDown(entityClass, id, sortPropertyName, null);
}
/* (non-Javadoc)
* @see com.nenglong.base.dao.BaseExecuteDao#moveDown(java.io.Serializable, java.lang.String, java.lang.String)
*/
public void moveDown(final Serializable id, final String sortPropertyName, final String parentPropertyName) {
moveDown(entityClass, id, sortPropertyName, parentPropertyName);
}
/* (non-Javadoc)
* @see com.nenglong.base.dao.BaseExecuteDao#moveDown(java.lang.Class, java.io.Serializable, java.lang.String, java.lang.String)
*/
public void moveDown(final Class<?> entityClass, final Serializable id, final String sortPropertyName, final String parentPropertyName) {
moveUpOrDown("DOWN", entityClass, id, sortPropertyName, parentPropertyName);
}
/**
* 上移或下移
* @param move UP:下移;DOWN:下移
* @param id 主键
* @param entityClass 实体Bean的类型。如User.class
* @param sortPropertyName 排序的实体属性名
* @param parentPropertyName 父节点属性名
*/
protected void moveUpOrDown(final String move, final Class<?> entityClass, final Serializable id, final String sortPropertyName, final String parentPropertyName){
String jpql;
boolean isNotParent = StringUtils.isEmpty(parentPropertyName);
//jqpl格式参数。参数1:实体名;参数2:排序属性;参数3:条件属性(父节点属性)
if("DOWN".equals(move)){//下移。写查询上一条记录的JPQL语句
jpql = "from %1$s mod where mod.%2$s in (select min(mod1.%2$s) from %1$s mod1 where mod1.%2$s > ?)";
}else if("UP".equals(move)){//上移。写查询下一条记录的JPQL语句
jpql = "from %1$s mod where mod.%2$s in (select max(mod1.%2$s) from %1$s mod1 where mod1.%2$s < ?)";
}else {
return;
}
try {
List<Object> list = new LinkedList<Object>();//jqpl格式化的属性参数
List<Object> list2 = new LinkedList<Object>();//查询语句的参数值
String entityName = getEntityName(entityClass);
list.add(entityName);
list.add(sortPropertyName);
Object bean = find(entityClass,id);//查询当前记录对象
Object sort = PropertyUtils.getProperty(bean, sortPropertyName);//获取当前记录的排序值
list2.add(sort);
if(!isNotParent){//有父节点
Object obj = PropertyUtils.getProperty(bean, parentPropertyName);//获取当前记录的父节点值
list.add(parentPropertyName);
StringBuilder sb = new StringBuilder(jpql.substring(0, jpql.length()-1));
if(obj==null){
sb.append(" and mod1.%3$s is null) and mod.%3$s is null");
}else{
sb.append(" and mod1.%3$s = ?) and mod.%3$s =?");
list2.add(obj);
list2.add(obj);
}
jpql = sb.toString();
sb = null;
}
jpql = String.format(jpql,list.toArray());////jqpl格式化
list = null;
Object bean2 = getSingleResultByJPQL(jpql, list2.toArray());//如果为上移,则查询上一条记录对象;否则为下移,则查询下一条记录对象。
if(bean2!=null){
Object sort2 = PropertyUtils.getProperty(bean2, sortPropertyName);//如果为上移,则获取上一条记录的排序值;否则为下移,则获取下一条记录的排序值。
PropertyUtils.setProperty(bean, sortPropertyName, sort2);//如果为上移,则设置当前记录的排序值为上一条记录的排序值;否则为下移,则设置当前记录的排序值为下一条记录的排序值。
PropertyUtils.setProperty(bean2, sortPropertyName, sort);//如果为上移,则设置上一条记录的排序值为当前记录的排序值;否则为下移,则设置下一条记录的排序值为当前记录的排序值。
update(bean,bean2);//更新当前的排序顺序,更新上一条或下一条的排序顺序
}
} catch (Exception e) {
throw new RuntimeException(e);
}
}
}