效果
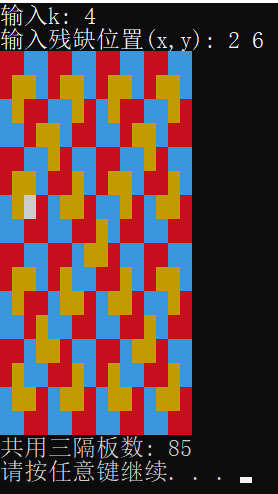
代码
#include
#include
using namespace std;
class ChessBoard {
private:
int **chessBoard;
int size;
int xd, yd;
int triangle;
public:
ChessBoard(int s, int x, int y) {
size = s;
chessBoard = new int*[size];
for (int i = 0; i < size; i++) {
chessBoard[i] = new int[size];
for (int j = 0; j < size; j++)
chessBoard[i][j] = 0;
}
xd = x, yd = y;
chessBoard[xd][yd] = -1;
triangle = 0;
}
int search(int lx, int ly, int rx, int ry, int len);
void cover(int lx, int ly, int rx, int ry, int len, int tri);
void display();
};
int ChessBoard::search(int lx, int ly, int rx, int ry, int len) {
int i, j;
bool flag = false;
for (i = lx; i <= rx; i++) {
for (j = ly; j <= ry; j++) {
if (chessBoard[i][j] != 0) {
flag = true;
break;
}
}
if (flag) break;
}
int ox = lx + len;
int oy = ly + len;
if (i >= ox && j >= oy)
return 4;
if (i < ox && j >= oy)
return 1;
if (i >= ox && j < oy)
return 3;
if (i < ox && j < oy)
return 2;
}
void ChessBoard::cover(int lx, int ly, int rx, int ry, int len, int color) {
if (len == 0)
return;
triangle++;
if (len >= 2) color = 1;
int quadrant = search(lx, ly, rx, ry, len);
if (quadrant == 1) {
chessBoard[lx + len][ly + len] = color;
chessBoard[lx + len][ly + len - 1] = color;
chessBoard[lx + len - 1][ly + len - 1] = color;
}
else if (quadrant == 2) {
chessBoard[lx + len - 1][ly + len] = color;
chessBoard[lx + len][ly + len] = color;
chessBoard[lx + len][ly + len - 1] = color;
}
else if (quadrant == 3) {
chessBoard[lx + len - 1][ly + len] = color;
chessBoard[lx + len - 1][ly + len - 1] = color;
chessBoard[lx + len][ly + len] = color;
}
else if (quadrant == 4) {
chessBoard[lx + len - 1][ly + len] = color;
chessBoard[lx + len - 1][ly + len - 1] = color;
chessBoard[lx + len][ly + len - 1] = color;
}
cover(lx, ly + len, lx + len - 1, ry, len / 2, 2);
cover(lx, ly, lx + len - 1, ly + len - 1, len / 2, 3);
cover(lx + len, ly, rx, ly + len - 1, len / 2, 2);
cover(lx + len, ly + len, rx, ry, len / 2, 3);
}
void ChessBoard::display() {
enum colour { black, blue, green, lake_blue, red, purple, yellow, white, grey, baby_blue };
int forecolour = white, backcolour;
HANDLE handle;
handle = GetStdHandle(STD_OUTPUT_HANDLE);
COORD coord;
for (int i = 0; i < size; i++) {
for (int j = 0; j < size; j++) {
coord.X = i;
coord.Y = j + 2;
SetConsoleCursorPosition(handle, coord);
if (chessBoard[i][j] == -1) backcolour = white;
else if (chessBoard[i][j] == 1) backcolour = yellow;
else if (chessBoard[i][j] == 2) backcolour = lake_blue;
else if (chessBoard[i][j] == 3) backcolour = red;
SetConsoleTextAttribute(handle, forecolour + backcolour * 0x10);
cout << " ";
}
cout << endl;
}
backcolour = black;
SetConsoleTextAttribute(handle, forecolour + backcolour * 0x10);
cout << "共用三隔板数: " << triangle << endl;
}
int main(void) {
int k, x, y;
cout << "输入k: ";
cin >> k;
cout << "输入残缺位置(x,y): ";
cin >> x >> y;
int size = pow(2, k);
ChessBoard chessBoard(size, x, y);
chessBoard.cover(0, 0, size - 1, size - 1, size / 2, 1);
chessBoard.display();
system("pause");
return 0;
}