#include
#include
#include
#define LEFT_EDGE 1
#define RIGHT_EDGE 2
#define BOTTOM_EDGE 4
#define TOP_EDGE 8
void LineGL(int x0,int y0,int x1,int y1)
{
glBegin(GL_LINES);
glColor3f(1.0f,0.0f,0.0f); glVertex2f(x0,y0);
glColor3f(0.0f,1.0f,0.0f); glVertex2f(x1,y1); //虽然两个点的颜色设置不同,但是最终线段颜色是绿色
glEnd(); //因为glShadeModel(GL_FLAT)设置为最后一个顶点的颜色
} //决定整个图元的颜色。
struct Rectangle
{
float xmin,xmax,ymin,ymax;
};
Rectangle rect;
int x0,y0,x1,y1;
int CompCode(int x,int y,Rectangle rect)
{
int code=0x00; //此处是二进制
if(yrect.ymax)
code=code|8;
if(x>rect.xmax)
code=code|2;
if(x2) //total>2,说明此函数进入死循环,是cohen-Sutherland算法的意外情况
{
done=1;flag=1;
}
}
}while(!done);
if(accept) //线段位于窗口内或者线段剪裁过accept=1;
LineGL(x0,y0,x1,y1);
else
{
x0=0;y=0;x1=0;y1=0;
LineGL(x0,y0,x1,y1);
}
return accept;
}
void myDisplay()
{
glClear(GL_COLOR_BUFFER_BIT);
glColor3f(1.0f,0.0f,0.0f);
glRectf(rect.xmin,rect.ymin,rect.xmax,rect.ymax);
LineGL(x0,y0,x1,y1);
glFlush();
}
void Init()
{
glClearColor(0.0,0.0,0.0,0.0);
glShadeModel(GL_FLAT);
rect.xmin=100;
rect.xmax=300;
rect.ymin=100;
rect.ymax=300;
x0=150,y0=0,x1=50,y1=150;
printf("Press key 'c' to Clip!\nPress key 'r' to Restore!\n");
}
void Reshape(int w,int h)
{
glViewport(0,0,(GLsizei) w,(GLsizei) h);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
gluOrtho2D(0.0,(GLdouble) w,0.0,(GLdouble) h);
//printf("######Reshape\n");
}
void keyboard(unsigned char key,int x,int y)
{
switch (key)
{
case 'c':
cohensutherlandlineclip(rect,x0,y0,x1,y1);
glutPostRedisplay();
break;
case 'r':
Init();
glutPostRedisplay();
break;
case 'x':
exit(0);
break;
default:
break;
}
}
int main(int argc,char *argv[])
{
glutInit(&argc,argv);
glutInitDisplayMode(GLUT_RGB|GLUT_SINGLE);
glutInitWindowPosition(100,100);
glutInitWindowSize(640,480);
glutCreateWindow("Hello World");
Init();
glutDisplayFunc(myDisplay);
glutReshapeFunc(Reshape);
glutKeyboardFunc(&keyboard);//按下键盘后会调用该函数,键和鼠标点击位置作为变量传给keyboard函数
glutMainLoop();
return 0;
}
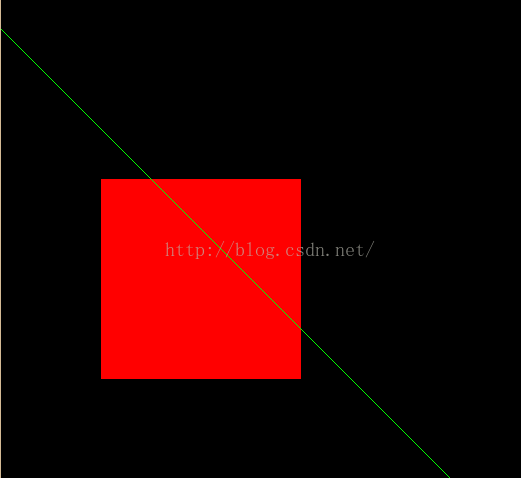
#include
#include
#include
#define LEFT_EDGE 1
#define RIGHT_EDGE 2
#define BOTTOM_EDGE 4
#define TOP_EDGE 8
float ymin=100;int ymax=300;int xmin=100;int xmax=300;
struct Linea
{
int x0;
int y0;
int x1;
int y1;
};
Linea l1={l1.x0=450,l1.y0=0,l1.x1=0,l1.y1=450};
Linea l2={l2.x0=450,l2.y0=200,l2.x1=0,l2.y1=200};
Linea l3={l3.x0=200,l3.y0=0,l3.x1=200,l3.y1=450};
void LineGL(Linea &li) //没有&,引用改变li的值
{
glBegin(GL_LINES);
glColor3f(1.0f,0.0f,0.0f); glVertex2f(li.x0,li.y0);
glColor3f(0.0f,1.0f,0.0f); glVertex2f(li.x1,li.y1); //虽然两个点的颜色设置不同,但是最终线段颜色是绿色
glEnd(); //因为glShadeModel(GL_FLAT)设置为最后一个顶点的颜色
} //决定整个图元的颜色。
int CompCode(int x,int y)
{
int code=0x00; //此处是二进制
if(yymax)
code=code|8;
if(x>xmax)
code=code|2;
if(x3) //total>3,执行两次后total又加一
{
done=1;flag=1;
}
}
}while(!done);
if(accept) //线段位于窗口内或者线段剪裁过accept=1;
{
LineGL(li);
}
else
{
li.x0=0;li.y0=0;li.x1=0;li.y1=0;
LineGL(li);
}
return accept;
}
void myDisplay()
{
glClear(GL_COLOR_BUFFER_BIT);
glColor3f(1.0f,0.0f,0.0f);
glBegin(GL_LINE_LOOP);
glVertex2f(100,100);
glVertex2f(300,100);
glVertex2f(300,300);
glVertex2f(100,300);
glEnd();
LineGL(l1);
LineGL(l2);
LineGL(l3);
glFlush();
}
void Init()
{
glClearColor(0.0,0.0,0.0,0.0);
glShadeModel(GL_FLAT);
printf("Press key 'c' to Clip!\nPress key 'r' to Restore!\n");
}
void Reshape(int w,int h)
{
glViewport(0,0,(GLsizei) w,(GLsizei) h);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
gluOrtho2D(0.0,(GLdouble) w,0.0,(GLdouble) h);
//printf("######Reshape\n");
}
void keyboard(unsigned char key,int x,int y)
{
switch (key)
{
case 'c':
cohensutherlandlineclip(l1);
cohensutherlandlineclip(l2);
cohensutherlandlineclip(l3);
glutPostRedisplay();
break;
case 'r':
l1.x0=450;l1.y0=0;l1.x1=0;l1.y1=450;
l2.x0=450;l2.y0=200;l2.x1=0;l2.y1=200;
l3.x0=200;l3.y0=0;l3.x1=200;l3.y1=450;
Init();
glutPostRedisplay();
break;
case 'x':
exit(0);
break;
default:
break;
}
}
int main(int argc,char *argv[])
{
glutInit(&argc,argv);
glutInitDisplayMode(GLUT_RGB|GLUT_SINGLE);
glutInitWindowPosition(100,100);
glutInitWindowSize(640,480);
glutCreateWindow("Hello World");
Init();
glutDisplayFunc(myDisplay);
glutReshapeFunc(Reshape);
glutKeyboardFunc(&keyboard);//按下键盘后会调用该函数,键和鼠标点击位置作为变量传给keyboard函数
glutMainLoop();
return 0;
}
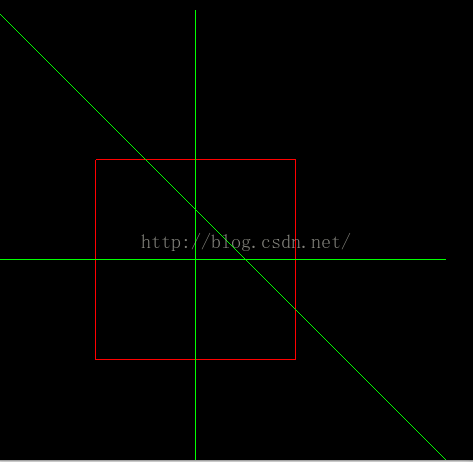