需求:
1.一个android端的service后台运行的程序,作为socket的服务器端;用于接收Pc client端发来的命令,来处理数据后,把结果发给PC client
2.PC端程序,作为socket的客户端,用于给android手机端发操作命令
难点分析:
1.手机一定要有adb模式,即插上USB线时马上提示的对话框选adb。好多对手机的操作都可以用adb直接作。
不过,我发现LG GW880就没有,要去下载个
2.android默认手机端的IP为“127.0.0.1”
3.要想联通PC与android手机的sokcet,一定要用adb forward 来作下端口转发才能连上socket.
- Runtime.getRuntime().exec("adb forward tcp:12580 tcp:10086");
- Thread.sleep(3000);
4.android端的service程序Install到手机上容易,但是还要有方法来从PC的client端来启动手机上的service ,这个办法可以通过PC端adb命令来发一个Broastcast,手机端再写个接收BroastcastReceive来接收这个Broastcast,在这个BroastcastReceive来启动service
pc端命令:
- Runtime.getRuntime().exec(
- "adb shell am broadcast -a NotifyServiceStart");
android端的代码:ServiceBroadcastReceiver.java
- package com.otheri.service;
-
- import android.content.BroadcastReceiver;
- import android.content.Context;
- import android.content.Intent;
- import android.util.Log;
-
- public class ServiceBroadcastReceiver extends BroadcastReceiver {
- private static String START_ACTION = "NotifyServiceStart";
- private static String STOP_ACTION = "NotifyServiceStop";
-
- @Override
- public void onReceive(Context context, Intent intent) {
- Log.d(androidService.TAG, Thread.currentThread().getName() + "---->"
- + "ServiceBroadcastReceiver onReceive");
-
- String action = intent.getAction();
- if (START_ACTION.equalsIgnoreCase(action)) {
- context.startService(new Intent(context, androidService.class));
-
- Log.d(androidService.TAG, Thread.currentThread().getName() + "---->"
- + "ServiceBroadcastReceiver onReceive start end");
- } else if (STOP_ACTION.equalsIgnoreCase(action)) {
- context.stopService(new Intent(context, androidService.class));
- Log.d(androidService.TAG, Thread.currentThread().getName() + "---->"
- + "ServiceBroadcastReceiver onReceive stop end");
- }
- }
-
- }
5.由于是USB连接,所以socket就可以设计为一但连接就一直联通,即在new socket和开完out,in流后,就用个while(true){}来循环PC端和android端的读和写
android的代码:
- public void run() {
- Log.d(androidService.TAG, Thread.currentThread().getName() + "---->"
- + "a client has connected to server!");
- BufferedOutputStream out;
- BufferedInputStream in;
- try {
-
- String currCMD = "";
- out = new BufferedOutputStream(client.getOutputStream());
- in = new BufferedInputStream(client.getInputStream());
-
- androidService.ioThreadFlag = true;
- while (androidService.ioThreadFlag) {
- try {
- if (!client.isConnected()) {
- break;
- }
-
-
- Log.v(androidService.TAG, Thread.currentThread().getName()
- + "---->" + "will read......");
-
- currCMD = readCMDFromSocket(in);
- Log.v(androidService.TAG, Thread.currentThread().getName()
- + "---->" + "**currCMD ==== " + currCMD);
-
-
- if (currCMD.equals("1")) {
- out.write("OK".getBytes());
- out.flush();
- } else if (currCMD.equals("2")) {
- out.write("OK".getBytes());
- out.flush();
- } else if (currCMD.equals("3")) {
- out.write("OK".getBytes());
- out.flush();
- } else if (currCMD.equals("4")) {
-
- try {
- out.write("service receive OK".getBytes());
- out.flush();
- } catch (IOException e) {
- e.printStackTrace();
- }
-
-
- byte[] filelength = new byte[4];
- byte[] fileformat = new byte[4];
- byte[] filebytes = null;
-
-
- filebytes = receiveFileFromSocket(in, out, filelength,
- fileformat);
-
-
-
- try {
-
- File file = FileHelper.newFile("R0013340.JPG");
- FileHelper.writeFile(file, filebytes, 0,
- filebytes.length);
- } catch (IOException e) {
- e.printStackTrace();
- }
- } else if (currCMD.equals("exit")) {
-
- }
- } catch (Exception e) {
-
-
-
-
-
-
- Log.e(androidService.TAG, Thread.currentThread().getName()
- + "---->" + "read write error111111");
- }
- }
- out.close();
- in.close();
- } catch (Exception e) {
- Log.e(androidService.TAG, Thread.currentThread().getName()
- + "---->" + "read write error222222");
- e.printStackTrace();
- } finally {
- try {
- if (client != null) {
- Log.v(androidService.TAG, Thread.currentThread().getName()
- + "---->" + "client.close()");
- client.close();
- }
- } catch (IOException e) {
- Log.e(androidService.TAG, Thread.currentThread().getName()
- + "---->" + "read write error333333");
- e.printStackTrace();
- }
- }
6.如果是在PC端和android端的读写操作来while(true){}循环,这样socket流的结尾不好判断,不能用“-1”来判断,因为“-1”是只有在socket关闭时才作为判断结尾。
7.socket在out.write(bytes);时,要是数据太大时,超过socket的缓存,socket自动分包发送,所以对方就一定要用循环来多次读。最好的办法就是服务器和客户端协议好,比如发文件时,先写过来一个要发送的文件的大小,然后再发送文件;对方用这个大小,来循环读取数据。
android端接收数据的代码:
-
-
-
-
-
-
-
-
-
-
- public static byte[] receiveFileFromSocket(InputStream in,
- OutputStream out, byte[] filelength, byte[] fileformat) {
- byte[] filebytes = null;
- try {
- int filelen = MyUtil.bytesToInt(filelength);
- String strtmp = "read file length ok:" + filelen;
- out.write(strtmp.getBytes("utf-8"));
- out.flush();
-
- filebytes = new byte[filelen];
- int pos = 0;
- int rcvLen = 0;
- while ((rcvLen = in.read(filebytes, pos, filelen - pos)) > 0) {
- pos += rcvLen;
- }
- Log.v(androidService.TAG, Thread.currentThread().getName()
- + "---->" + "read file OK:file size=" + filebytes.length);
- out.write("read file ok".getBytes("utf-8"));
- out.flush();
- } catch (Exception e) {
- Log.v(androidService.TAG, Thread.currentThread().getName()
- + "---->" + "receiveFileFromSocket error");
- e.printStackTrace();
- }
- return filebytes;
- }
8.socket的最重要的机制就是读写采用的是阻塞的方式,如果客户端作为命令发起者,服务器端作为接收者的话,只有当客户端client用out.writer()写到输出流里后,即流中有数据service的read才会执行,不然就会一直停在read()那里等数据。
9.还要让服务器端可以同时连接多个client,即服务器端用new thread()来作数据读取操作。
源码:
客户端(pc端):
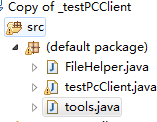
testPcClient.java
android服务器端:
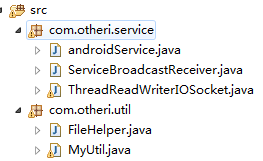
主类androidService.java
- package com.otheri.service;
-
- import java.io.File;
- import java.io.IOException;
- import java.net.ServerSocket;
- import java.net.Socket;
-
- import android.app.Service;
- import android.content.BroadcastReceiver;
- import android.content.Context;
- import android.content.Intent;
- import android.content.IntentFilter;
- import android.os.IBinder;
- import android.util.Log;
-
-
-
-
-
-
- public class androidService extends Service {
- public static final String TAG = "TAG";
- public static Boolean mainThreadFlag = true;
- public static Boolean ioThreadFlag = true;
- ServerSocket serverSocket = null;
- final int SERVER_PORT = 10086;
- File testFile;
- private sysBroadcastReceiver sysBR;
-
- @Override
- public void onCreate() {
- super.onCreate();
- Log.v(TAG, Thread.currentThread().getName() + "---->" + " onCreate");
-
- sysRegisterReceiver();
- new Thread() {
- public void run() {
- doListen();
- };
- }.start();
- }
-
- private void doListen() {
- Log.d(TAG, Thread.currentThread().getName() + "---->"
- + " doListen() START");
- serverSocket = null;
- try {
- Log.d(TAG, Thread.currentThread().getName() + "---->"
- + " doListen() new serverSocket");
- serverSocket = new ServerSocket(SERVER_PORT);
-
- boolean mainThreadFlag = true;
- while (mainThreadFlag) {
- Log.d(TAG, Thread.currentThread().getName() + "---->"
- + " doListen() listen");
-
- Socket client = serverSocket.accept();
-
- new Thread(new ThreadReadWriterIOSocket(this, client)).start();
- }
- } catch (IOException e1) {
- Log.v(androidService.TAG, Thread.currentThread().getName()
- + "---->" + "new serverSocket error");
- e1.printStackTrace();
- }
- }
-
-
- private void sysRegisterReceiver() {
- Log.v(TAG, Thread.currentThread().getName() + "---->"
- + "sysRegisterReceiver");
- sysBR = new sysBroadcastReceiver();
-
- IntentFilter filter1 = new IntentFilter();
-
- filter1.addAction("android.intent.action.PACKAGE_ADDED");
- filter1.addDataScheme("package");
- filter1.addAction("android.intent.action.PACKAGE_REMOVED");
- filter1.addDataScheme("package");
- registerReceiver(sysBR, filter1);
- }
-
-
- private class sysBroadcastReceiver extends BroadcastReceiver {
-
- @Override
- public void onReceive(Context context, Intent intent) {
- String action = intent.getAction();
- if (action.equalsIgnoreCase("android.intent.action.PACKAGE_ADDED")) {
-
- } else if (action
- .equalsIgnoreCase("android.intent.action.PACKAGE_REMOVED")) {
-
- }
- Log.v(TAG, Thread.currentThread().getName() + "---->"
- + "sysBroadcastReceiver onReceive");
- }
- }
-
- @Override
- public void onDestroy() {
- super.onDestroy();
-
-
- mainThreadFlag = false;
- ioThreadFlag = false;
-
- try {
- Log.v(TAG, Thread.currentThread().getName() + "---->"
- + "serverSocket.close()");
- serverSocket.close();
- } catch (IOException e) {
- e.printStackTrace();
- }
- Log.v(TAG, Thread.currentThread().getName() + "---->"
- + "**************** onDestroy****************");
- }
-
- @Override
- public void onStart(Intent intent, int startId) {
- Log.d(TAG, Thread.currentThread().getName() + "---->" + " onStart()");
- super.onStart(intent, startId);
-
- }
-
- @Override
- public IBinder onBind(Intent arg0) {
- Log.d(TAG, " onBind");
- return null;
- }
-
- }
用于接收PC发来的Broastcast并启动主类service的ServiceBroadcastReceiver.java
- package com.otheri.service;
-
- import android.content.BroadcastReceiver;
- import android.content.Context;
- import android.content.Intent;
- import android.util.Log;
-
- public class ServiceBroadcastReceiver extends BroadcastReceiver {
- private static String START_ACTION = "NotifyServiceStart";
- private static String STOP_ACTION = "NotifyServiceStop";
-
- @Override
- public void onReceive(Context context, Intent intent) {
- Log.d(androidService.TAG, Thread.currentThread().getName() + "---->"
- + "ServiceBroadcastReceiver onReceive");
-
- String action = intent.getAction();
- if (START_ACTION.equalsIgnoreCase(action)) {
- context.startService(new Intent(context, androidService.class));
-
- Log.d(androidService.TAG, Thread.currentThread().getName() + "---->"
- + "ServiceBroadcastReceiver onReceive start end");
- } else if (STOP_ACTION.equalsIgnoreCase(action)) {
- context.stopService(new Intent(context, androidService.class));
- Log.d(androidService.TAG, Thread.currentThread().getName() + "---->"
- + "ServiceBroadcastReceiver onReceive stop end");
- }
- }
-
- }
用于新socket连接的读写线程类ThreadReadWriterIOSocket.java
- package com.otheri.service;
-
- import java.io.BufferedInputStream;
- import java.io.BufferedOutputStream;
- import java.io.ByteArrayOutputStream;
- import java.io.File;
- import java.io.IOException;
- import java.io.InputStream;
- import java.io.OutputStream;
- import java.net.Socket;
-
- import android.content.Context;
- import android.util.Log;
-
- import com.otheri.util.FileHelper;
- import com.otheri.util.MyUtil;
-
-
-
-
-
-
-
- public class ThreadReadWriterIOSocket implements Runnable {
- private Socket client;
- private Context context;
-
- ThreadReadWriterIOSocket(Context context, Socket client) {
-
- this.client = client;
- this.context = context;
- }
-
- @Override
- public void run() {
- Log.d(androidService.TAG, Thread.currentThread().getName() + "---->"
- + "a client has connected to server!");
- BufferedOutputStream out;
- BufferedInputStream in;
- try {
-
- String currCMD = "";
- out = new BufferedOutputStream(client.getOutputStream());
- in = new BufferedInputStream(client.getInputStream());
-
- androidService.ioThreadFlag = true;
- while (androidService.ioThreadFlag) {
- try {
- if (!client.isConnected()) {
- break;
- }
-
-
- Log.v(androidService.TAG, Thread.currentThread().getName()
- + "---->" + "will read......");
-
- currCMD = readCMDFromSocket(in);
- Log.v(androidService.TAG, Thread.currentThread().getName()
- + "---->" + "**currCMD ==== " + currCMD);
-
-
- if (currCMD.equals("1")) {
- out.write("OK".getBytes());
- out.flush();
- } else if (currCMD.equals("2")) {
- out.write("OK".getBytes());
- out.flush();
- } else if (currCMD.equals("3")) {
- out.write("OK".getBytes());
- out.flush();
- } else if (currCMD.equals("4")) {
-
- try {
- out.write("service receive OK".getBytes());
- out.flush();
- } catch (IOException e) {
- e.printStackTrace();
- }
-
-
- byte[] filelength = new byte[4];
- byte[] fileformat = new byte[4];
- byte[] filebytes = null;
-
-
- filebytes = receiveFileFromSocket(in, out, filelength,
- fileformat);
-
-
-
- try {
-
- File file = FileHelper.newFile("R0013340.JPG");
- FileHelper.writeFile(file, filebytes, 0,
- filebytes.length);
- } catch (IOException e) {
- e.printStackTrace();
- }
- } else if (currCMD.equals("exit")) {
-
- }
- } catch (Exception e) {
-
-
-
-
-
-
- Log.e(androidService.TAG, Thread.currentThread().getName()
- + "---->" + "read write error111111");
- }
- }
- out.close();
- in.close();
- } catch (Exception e) {
- Log.e(androidService.TAG, Thread.currentThread().getName()
- + "---->" + "read write error222222");
- e.printStackTrace();
- } finally {
- try {
- if (client != null) {
- Log.v(androidService.TAG, Thread.currentThread().getName()
- + "---->" + "client.close()");
- client.close();
- }
- } catch (IOException e) {
- Log.e(androidService.TAG, Thread.currentThread().getName()
- + "---->" + "read write error333333");
- e.printStackTrace();
- }
- }
- }
-
-
-
-
-
-
-
-
-
-
-
- public static byte[] receiveFileFromSocket(InputStream in,
- OutputStream out, byte[] filelength, byte[] fileformat) {
- byte[] filebytes = null;
- try {
- in.read(filelength);
- int filelen = MyUtil.bytesToInt(filelength);
- String strtmp = "read file length ok:" + filelen;
- out.write(strtmp.getBytes("utf-8"));
- out.flush();
-
- filebytes = new byte[filelen];
- int pos = 0;
- int rcvLen = 0;
- while ((rcvLen = in.read(filebytes, pos, filelen - pos)) > 0) {
- pos += rcvLen;
- }
- Log.v(androidService.TAG, Thread.currentThread().getName()
- + "---->" + "read file OK:file size=" + filebytes.length);
- out.write("read file ok".getBytes("utf-8"));
- out.flush();
- } catch (Exception e) {
- Log.v(androidService.TAG, Thread.currentThread().getName()
- + "---->" + "receiveFileFromSocket error");
- e.printStackTrace();
- }
- return filebytes;
- }
-
-
- public static String readCMDFromSocket(InputStream in) {
- int MAX_BUFFER_BYTES = 2048;
- String msg = "";
- byte[] tempbuffer = new byte[MAX_BUFFER_BYTES];
- try {
- int numReadedBytes = in.read(tempbuffer, 0, tempbuffer.length);
- msg = new String(tempbuffer, 0, numReadedBytes, "utf-8");
- tempbuffer = null;
- } catch (Exception e) {
- Log.v(androidService.TAG, Thread.currentThread().getName()
- + "---->" + "readFromSocket error");
- e.printStackTrace();
- }
-
- return msg;
- }
- }
后面是两个辅助类:
- package com.otheri.util;
-
- import java.io.BufferedInputStream;
- import java.io.File;
- import java.io.FileInputStream;
- import java.io.FileOutputStream;
- import java.io.IOException;
-
- import com.otheri.service.androidService;
-
- import android.util.Log;
-
- public class FileHelper {
-
-
- private static String FILEPATH = "/sdcard";
-
-
-
- public static File newFile(String fileName) {
- File file = null;
- try {
- file = new File(FILEPATH, fileName);
- file.delete();
- file.createNewFile();
- } catch (IOException e) {
-
- e.printStackTrace();
- }
- return file;
- }
-
- public static void writeFile(File file, byte[] data, int offset, int count)
- throws IOException {
-
- FileOutputStream fos = new FileOutputStream(file, true);
- fos.write(data, offset, count);
- fos.flush();
- fos.close();
- }
-
- public static byte[] readFile(String fileName) throws IOException {
- File file = new File(FILEPATH, fileName);
- file.createNewFile();
- FileInputStream fis = new FileInputStream(file);
- BufferedInputStream bis = new BufferedInputStream(fis);
- int leng = bis.available();
- Log.v(androidService.TAG, "filesize = " + leng);
- byte[] b = new byte[leng];
- bis.read(b, 0, leng);
-
-
-
- bis.close();
- return b;
-
- }
- }
- package com.otheri.util;
-
- import java.io.InputStream;
-
- import android.util.Log;
-
- import com.otheri.service.androidService;
-
- public class MyUtil {
-
- public static int bytesToInt(byte[] bytes) {
- int addr = bytes[0] & 0xFF;
- addr |= ((bytes[1] << 8) & 0xFF00);
- addr |= ((bytes[2] << 16) & 0xFF0000);
- addr |= ((bytes[3] << 24) & 0xFF000000);
- return addr;
- }
-
-
- public static byte[] intToByte(int i) {
- byte[] abyte0 = new byte[4];
- abyte0[0] = (byte) (0xff & i);
- abyte0[1] = (byte) ((0xff00 & i) >> 8);
- abyte0[2] = (byte) ((0xff0000 & i) >> 16);
- abyte0[3] = (byte) ((0xff000000 & i) >> 24);
- return abyte0;
- }
-
-
-
-
- }