- 目标要求:自己初始化日期相关的数组并从小到大排序。
- 错误代码:
public class TestDateBubbleSort {
public static void main (String [] args) {
Date[] date = new Date[5];
date[0] = new Date(2006,5,4);
date[1] = new Date(2006,7,4);
date[2] = new Date(2008,5,4);
date[3] = new Date(2004,5,9);
date[4] = new Date(2004,5,4);
bubbleSort(date);
for(int i=0;i<date.length;i++) {
System.out.println(date[i]);
}
}
public static void bubbleSort(Date[] date) {
int len = date.length;
for (int i=len-1; i>=1; i--) {
for (int j = 0; j<=i-1; j++) {
if (date[j].compare(date[j+1]) > 0) {
Date temp = date[j];
date[j] = date[j+1];
date[j+1] = temp;
}
}
}
}
class Date {
int year,month,day;
Date (int year,int month,int day) {
this.year = year;
this.month = month;
this.day = day;
}
public int compare (Date date) {
return year > date.year ? 1
:year < date.year? -1
:month > date.month ? 1
:month < date.month ? -1
:day > date.day ? 1
:day < date.day ? -1 : 0;
}
public String toString () {
return "Year-Month-Day:" + year + "-" + month + "-" + day;
}
}
}
- 报错情况截图:
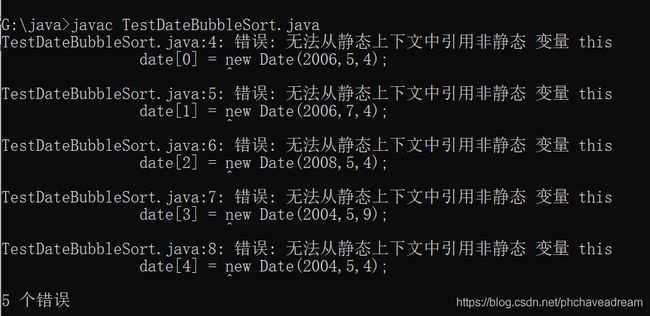
- 报错原因:
静态方法可以通过所在类直接调用而不需要实例化对象,非静态成员变量则是一个对象的属性它只有在有实例化对象时才存在的,所以在静态方法中是不可以调用非静态变量!这里的出错是因为将Date类置于TestDateBubbleSort类中,并在静态方法中调用。
- 解决方案一(调整Date类位置):
public class TestDateBubbleSort {
public static void main (String [] args) {
Date[] date = new Date[5];
date[0] = new Date(2006,5,4);
date[1] = new Date(2006,7,4);
date[2] = new Date(2008,5,4);
date[3] = new Date(2004,5,9);
date[4] = new Date(2004,5,4);
bubbleSort(date);
for(int i=0;i<date.length;i++) {
System.out.println(date[i]);
}
}
public static void bubbleSort(Date[] date) {
int len = date.length;
for (int i=len-1; i>=1; i--) {
for (int j = 0; j<=i-1; j++) {
if (date[j].compare(date[j+1]) > 0) {
Date temp = date[j];
date[j] = date[j+1];
date[j+1] = temp;
}
}
}
}
}
class Date {
int year,month,day;
Date (int year,int month,int day) {
this.year = year;
this.month = month;
this.day = day;
}
public int compare (Date date) {
return year > date.year ? 1
:year < date.year? -1
:month > date.month ? 1
:month < date.month ? -1
:day > date.day ? 1
:day < date.day ? -1 : 0;
}
public String toString () {
return "Year-Month-Day:" + year + "-" + month + "-" + day;
}
}
- 方案一效果图:
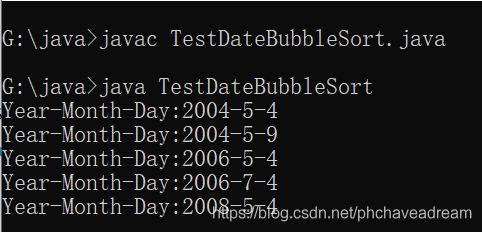
- 方案二(静态方法main中使用外部类的对象访问非静态成员):
public class TestDateBubbleSort {
public static void main (String [] args) {
TestDateBubbleSort.Date[] date = new TestDateBubbleSort.Date[5];
date[0] = new TestDateBubbleSort().new Date(2006,5,4);
date[1] = new TestDateBubbleSort().new Date(2006,7,4);
date[2] = new TestDateBubbleSort().new Date(2008,5,4);
date[3] = new TestDateBubbleSort().new Date(2004,5,9);
date[4] = new TestDateBubbleSort().new Date(2004,5,4);
bubbleSort(date);
for(int i=0;i<date.length;i++) {
System.out.println(date[i]);
}
}
public static void bubbleSort(Date[] date) {
int len = date.length;
for (int i=len-1; i>=1; i--) {
for (int j = 0; j<=i-1; j++) {
if (date[j].compare(date[j+1]) > 0) {
Date temp = date[j];
date[j] = date[j+1];
date[j+1] = temp;
}
}
}
}
class Date {
int year,month,day;
Date (int year,int month,int day) {
this.year = year;
this.month = month;
this.day = day;
}
public int compare (Date date) {
return year > date.year ? 1
:year < date.year? -1
:month > date.month ? 1
:month < date.month ? -1
:day > date.day ? 1
:day < date.day ? -1 : 0;
}
public String toString () {
return "Year-Month-Day:" + year + "-" + month + "-" + day;
}
}
}
- 方案二效果图:
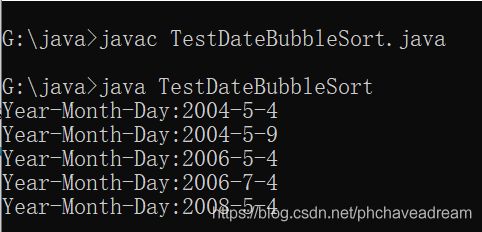
- (不推荐)方案三(将Date类转变为静态类)
public class TestDateBubbleSort {
public static void main (String [] args) {
Date[] date = new Date[5];
date[0] = new Date(2006,5,4);
date[1] = new Date(2006,7,4);
date[2] = new Date(2008,5,4);
date[3] = new Date(2004,5,9);
date[4] = new Date(2004,5,4);
bubbleSort(date);
for(int i=0;i<date.length;i++) {
System.out.println(date[i]);
}
}
public static void bubbleSort(Date[] date) {
int len = date.length;
for (int i=len-1; i>=1; i--) {
for (int j = 0; j<=i-1; j++) {
if (date[j].compare(date[j+1]) > 0) {
Date temp = date[j];
date[j] = date[j+1];
date[j+1] = temp;
}
}
}
}
static class Date {
int year,month,day;
Date (int year,int month,int day) {
this.year = year;
this.month = month;
this.day = day;
}
public int compare (Date date) {
return year > date.year ? 1
:year < date.year? -1
:month > date.month ? 1
:month < date.month ? -1
:day > date.day ? 1
:day < date.day ? -1 : 0;
}
public String toString () {
return "Year-Month-Day:" + year + "-" + month + "-" + day;
}
}
}
- 方案三效果图:
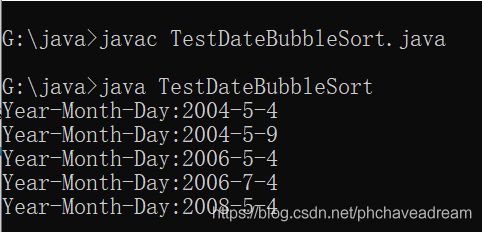
特别鸣谢以下大佬提供的帮助和灵感:
大佬1
大佬2
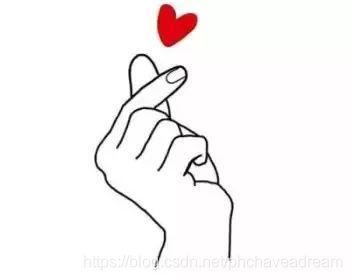